Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Base / MS / Internal / ComponentModel / DependencyPropertyAttribute.cs / 1 / DependencyPropertyAttribute.cs
namespace MS.Internal.ComponentModel { using System; using System.Windows; ////// This attribute is synthesized by our DependencyObjectProvider /// to relate a property descriptor back to a dependency property. /// [AttributeUsage(AttributeTargets.Method)] internal sealed class DependencyPropertyAttribute : Attribute { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates a new DependencyPropertyAttribute for the given dependency property. /// internal DependencyPropertyAttribute(DependencyProperty dependencyProperty, bool isAttached) { if (dependencyProperty == null) throw new ArgumentNullException("dependencyProperty"); _dp = dependencyProperty; _isAttached = isAttached; } #endregion Constructors //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Override of Object.Equals that returns true when the dependency /// property contained within each attribute is the same. /// public override bool Equals(object value) { DependencyPropertyAttribute da = value as DependencyPropertyAttribute; if (da != null && object.ReferenceEquals(da._dp, _dp) && da._isAttached == _isAttached) { return true; } return false; } ////// Override of Object.GetHashCode(); /// public override int GetHashCode() { return _dp.GetHashCode(); } #endregion Public Methods //------------------------------------------------------ // // Public Operators // //----------------------------------------------------- //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// Overrides Attribute.TypeId to be unique with respect to /// other dependency property attributes.c /// public override object TypeId { get { return typeof(DependencyPropertyAttribute); } } #endregion Public Properties //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Returns whether the dependency property is an attached /// property. /// internal bool IsAttached { get { return _isAttached; } } ////// Returns the dependency property instance this attribute is /// associated with. /// internal DependencyProperty DependencyProperty { get { return _dp; } } #endregion Internal Properties //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private DependencyProperty _dp; private bool _isAttached; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace MS.Internal.ComponentModel { using System; using System.Windows; ////// This attribute is synthesized by our DependencyObjectProvider /// to relate a property descriptor back to a dependency property. /// [AttributeUsage(AttributeTargets.Method)] internal sealed class DependencyPropertyAttribute : Attribute { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates a new DependencyPropertyAttribute for the given dependency property. /// internal DependencyPropertyAttribute(DependencyProperty dependencyProperty, bool isAttached) { if (dependencyProperty == null) throw new ArgumentNullException("dependencyProperty"); _dp = dependencyProperty; _isAttached = isAttached; } #endregion Constructors //----------------------------------------------------- // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Override of Object.Equals that returns true when the dependency /// property contained within each attribute is the same. /// public override bool Equals(object value) { DependencyPropertyAttribute da = value as DependencyPropertyAttribute; if (da != null && object.ReferenceEquals(da._dp, _dp) && da._isAttached == _isAttached) { return true; } return false; } ////// Override of Object.GetHashCode(); /// public override int GetHashCode() { return _dp.GetHashCode(); } #endregion Public Methods //------------------------------------------------------ // // Public Operators // //----------------------------------------------------- //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// Overrides Attribute.TypeId to be unique with respect to /// other dependency property attributes.c /// public override object TypeId { get { return typeof(DependencyPropertyAttribute); } } #endregion Public Properties //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Internal Properties // //----------------------------------------------------- #region Internal Properties ////// Returns whether the dependency property is an attached /// property. /// internal bool IsAttached { get { return _isAttached; } } ////// Returns the dependency property instance this attribute is /// associated with. /// internal DependencyProperty DependencyProperty { get { return _dp; } } #endregion Internal Properties //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private DependencyProperty _dp; private bool _isAttached; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
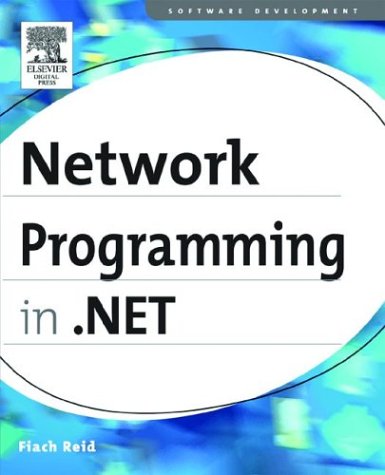
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- IpcPort.cs
- XmlBindingWorker.cs
- FileInfo.cs
- CodeStatementCollection.cs
- ActivityStateRecord.cs
- DLinqAssociationProvider.cs
- PerCallInstanceContextProvider.cs
- DictionaryMarkupSerializer.cs
- PagesChangedEventArgs.cs
- BitmapSizeOptions.cs
- KeyProperty.cs
- DocumentEventArgs.cs
- GenericTypeParameterConverter.cs
- GridViewHeaderRowPresenterAutomationPeer.cs
- AlphabeticalEnumConverter.cs
- TextServicesCompartmentEventSink.cs
- ParserStreamGeometryContext.cs
- BindingContext.cs
- SqlVisitor.cs
- ColumnResult.cs
- HyperLinkColumn.cs
- DataGridViewCellLinkedList.cs
- SizeAnimationClockResource.cs
- TableCellCollection.cs
- Matrix3D.cs
- SimplePropertyEntry.cs
- CreateUserWizardStep.cs
- SinglePhaseEnlistment.cs
- ProcessModule.cs
- CalendarItem.cs
- listviewsubitemcollectioneditor.cs
- Point.cs
- CompositionTarget.cs
- WebExceptionStatus.cs
- ValidatingPropertiesEventArgs.cs
- ApplicationActivator.cs
- AuthenticationManager.cs
- MemberPath.cs
- FlowDocument.cs
- XmlSchemaSimpleTypeList.cs
- XmlAnyElementAttribute.cs
- InvalidCastException.cs
- SqlDataSourceCache.cs
- DecimalConstantAttribute.cs
- ErrorHandler.cs
- WebScriptMetadataFormatter.cs
- ClientFormsAuthenticationCredentials.cs
- ScriptRef.cs
- BaseCollection.cs
- SchemaImporter.cs
- BulletDecorator.cs
- PrimitiveRenderer.cs
- DeviceSpecificChoiceCollection.cs
- ByteConverter.cs
- InvokeProviderWrapper.cs
- StrokeSerializer.cs
- ProtocolsConfigurationEntry.cs
- ProfileSettings.cs
- ZoneButton.cs
- X509CertificateCollection.cs
- Int32CAMarshaler.cs
- XmlLanguage.cs
- Rule.cs
- MouseWheelEventArgs.cs
- Int16.cs
- EnumerationRangeValidationUtil.cs
- Renderer.cs
- SmtpLoginAuthenticationModule.cs
- StateDesigner.Helpers.cs
- FlowDocumentPaginator.cs
- storagemappingitemcollection.viewdictionary.cs
- EmptyElement.cs
- ControlPaint.cs
- ListControlDesigner.cs
- Variable.cs
- WebEventCodes.cs
- xdrvalidator.cs
- _ProxyChain.cs
- DBConnectionString.cs
- Rect3DValueSerializer.cs
- ArgIterator.cs
- InvokeAction.cs
- ParseHttpDate.cs
- ForwardPositionQuery.cs
- VarInfo.cs
- HttpListenerException.cs
- FlagsAttribute.cs
- Range.cs
- ComponentCommands.cs
- HttpClientCertificate.cs
- UIHelper.cs
- SafeMILHandle.cs
- DelegateSerializationHolder.cs
- SiteMapProvider.cs
- PerformanceCounter.cs
- RuleInfoComparer.cs
- CardSpaceSelector.cs
- RouteParameter.cs
- CustomPopupPlacement.cs
- QueryableDataSource.cs