Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Media / Animation / ControllableStoryboardAction.cs / 1 / ControllableStoryboardAction.cs
/****************************************************************************\ * * File: ControllableStoryboardAction.cs * * This object includes a named Storyboard references. When triggered, the * name is resolved and passed to a derived class method to perform the * actual action. * * Copyright (C) by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System.ComponentModel; // DefaultValueAttribute using System.Diagnostics; // Debug.Assert using System.Windows.Documents; // TableTemplate using System.Windows.Markup; // INameScope namespace System.Windows.Media.Animation { ////// A controllable storyboard action associates a trigger action with a /// Storyboard. The association from this object is a string that is the name /// of the Storyboard in a resource dictionary. /// public abstract class ControllableStoryboardAction : TriggerAction { ////// Internal constructor - nobody is supposed to ever create an instance /// of this class. Use a derived class instead. /// internal ControllableStoryboardAction() { } ////// Name to use for resolving the storyboard reference needed. This /// points to a BeginStoryboard instance, and we're controlling that one. /// [DefaultValue(null)] public string BeginStoryboardName { get { return _beginStoryboardName; } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "ControllableStoryboardAction")); } // Null is allowed to wipe out existing name - as long as another // valid name is set before Invoke time. _beginStoryboardName = value; } } internal sealed override void Invoke( FrameworkElement fe, FrameworkContentElement fce, Style targetStyle, FrameworkTemplate frameworkTemplate, Int64 layer ) { Debug.Assert( fe != null || fce != null, "Caller of internal function failed to verify that we have a FE or FCE - we have neither." ); Debug.Assert( targetStyle != null || frameworkTemplate != null, "This function expects to be called when the associated action is inside a Style/Template. But it was not given a reference to anything." ); INameScope nameScope = null; if( targetStyle != null ) { nameScope = targetStyle; } else { Debug.Assert( frameworkTemplate != null ); nameScope = frameworkTemplate; } Invoke( fe, fce, GetStoryboard( fe, fce, nameScope ) ); } internal sealed override void Invoke( FrameworkElement fe ) { Debug.Assert( fe != null, "Invoke needs an object as starting point"); Invoke( fe, null, GetStoryboard( fe, null, null ) ); } internal virtual void Invoke( FrameworkElement containingFE, FrameworkContentElement containingFCE, Storyboard storyboard ) { } // Find a Storyboard object for this StoryboardAction to act on, using the // given BeginStoryboardName to find a BeginStoryboard instance and use // its Storyboard object reference. private Storyboard GetStoryboard( FrameworkElement fe, FrameworkContentElement fce, INameScope nameScope ) { if( BeginStoryboardName == null ) { throw new InvalidOperationException(SR.Get(SRID.Storyboard_BeginStoryboardNameRequired)); } BeginStoryboard keyedBeginStoryboard = Storyboard.ResolveBeginStoryboardName( BeginStoryboardName, nameScope, fe, fce ); Storyboard storyboard = keyedBeginStoryboard.Storyboard; if( storyboard == null ) { throw new InvalidOperationException(SR.Get(SRID.Storyboard_BeginStoryboardNoStoryboard, BeginStoryboardName)); } return storyboard; } private string _beginStoryboardName = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /****************************************************************************\ * * File: ControllableStoryboardAction.cs * * This object includes a named Storyboard references. When triggered, the * name is resolved and passed to a derived class method to perform the * actual action. * * Copyright (C) by Microsoft Corporation. All rights reserved. * \***************************************************************************/ using System.ComponentModel; // DefaultValueAttribute using System.Diagnostics; // Debug.Assert using System.Windows.Documents; // TableTemplate using System.Windows.Markup; // INameScope namespace System.Windows.Media.Animation { ////// A controllable storyboard action associates a trigger action with a /// Storyboard. The association from this object is a string that is the name /// of the Storyboard in a resource dictionary. /// public abstract class ControllableStoryboardAction : TriggerAction { ////// Internal constructor - nobody is supposed to ever create an instance /// of this class. Use a derived class instead. /// internal ControllableStoryboardAction() { } ////// Name to use for resolving the storyboard reference needed. This /// points to a BeginStoryboard instance, and we're controlling that one. /// [DefaultValue(null)] public string BeginStoryboardName { get { return _beginStoryboardName; } set { if (IsSealed) { throw new InvalidOperationException(SR.Get(SRID.CannotChangeAfterSealed, "ControllableStoryboardAction")); } // Null is allowed to wipe out existing name - as long as another // valid name is set before Invoke time. _beginStoryboardName = value; } } internal sealed override void Invoke( FrameworkElement fe, FrameworkContentElement fce, Style targetStyle, FrameworkTemplate frameworkTemplate, Int64 layer ) { Debug.Assert( fe != null || fce != null, "Caller of internal function failed to verify that we have a FE or FCE - we have neither." ); Debug.Assert( targetStyle != null || frameworkTemplate != null, "This function expects to be called when the associated action is inside a Style/Template. But it was not given a reference to anything." ); INameScope nameScope = null; if( targetStyle != null ) { nameScope = targetStyle; } else { Debug.Assert( frameworkTemplate != null ); nameScope = frameworkTemplate; } Invoke( fe, fce, GetStoryboard( fe, fce, nameScope ) ); } internal sealed override void Invoke( FrameworkElement fe ) { Debug.Assert( fe != null, "Invoke needs an object as starting point"); Invoke( fe, null, GetStoryboard( fe, null, null ) ); } internal virtual void Invoke( FrameworkElement containingFE, FrameworkContentElement containingFCE, Storyboard storyboard ) { } // Find a Storyboard object for this StoryboardAction to act on, using the // given BeginStoryboardName to find a BeginStoryboard instance and use // its Storyboard object reference. private Storyboard GetStoryboard( FrameworkElement fe, FrameworkContentElement fce, INameScope nameScope ) { if( BeginStoryboardName == null ) { throw new InvalidOperationException(SR.Get(SRID.Storyboard_BeginStoryboardNameRequired)); } BeginStoryboard keyedBeginStoryboard = Storyboard.ResolveBeginStoryboardName( BeginStoryboardName, nameScope, fe, fce ); Storyboard storyboard = keyedBeginStoryboard.Storyboard; if( storyboard == null ) { throw new InvalidOperationException(SR.Get(SRID.Storyboard_BeginStoryboardNoStoryboard, BeginStoryboardName)); } return storyboard; } private string _beginStoryboardName = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
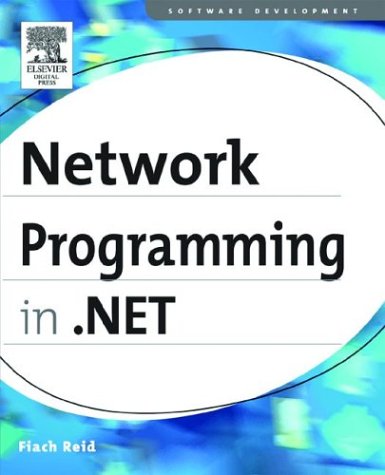
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SpinLock.cs
- ConnectionManagementElement.cs
- EventlogProvider.cs
- DictionaryEntry.cs
- CorrelationInitializer.cs
- Propagator.Evaluator.cs
- CriticalHandle.cs
- CheckBox.cs
- RootBrowserWindowAutomationPeer.cs
- Transform.cs
- ClusterSafeNativeMethods.cs
- LostFocusEventManager.cs
- XmlReaderDelegator.cs
- RegexCompiler.cs
- ApplicationId.cs
- ResXFileRef.cs
- FontSource.cs
- DataGridRelationshipRow.cs
- PrePostDescendentsWalker.cs
- AdapterDictionary.cs
- TextTreeTextBlock.cs
- Parameter.cs
- DataGrid.cs
- FileUpload.cs
- SafeUserTokenHandle.cs
- ProgressBar.cs
- WebResponse.cs
- MULTI_QI.cs
- TypedReference.cs
- EditingCommands.cs
- HandlerBase.cs
- ScrollBarAutomationPeer.cs
- DefaultBindingPropertyAttribute.cs
- MD5CryptoServiceProvider.cs
- TriggerBase.cs
- StateDesignerConnector.cs
- SimpleHandlerBuildProvider.cs
- PersonalizationProvider.cs
- IDQuery.cs
- RegisteredDisposeScript.cs
- Convert.cs
- LinqDataSourceValidationException.cs
- InheritedPropertyDescriptor.cs
- ReflectEventDescriptor.cs
- StateManagedCollection.cs
- DataBoundControlAdapter.cs
- InstancePersistenceException.cs
- ToolStripItemCollection.cs
- TableItemStyle.cs
- InheritanceContextChangedEventManager.cs
- FakeModelItemImpl.cs
- NamespaceCollection.cs
- CacheRequest.cs
- SemaphoreFullException.cs
- typedescriptorpermissionattribute.cs
- UserControlBuildProvider.cs
- SerialPort.cs
- DiagnosticsElement.cs
- SqlCaseSimplifier.cs
- ObjectListFieldCollection.cs
- ToolBarButton.cs
- GenericRootAutomationPeer.cs
- XmlExtensionFunction.cs
- ColumnHeader.cs
- ModifyActivitiesPropertyDescriptor.cs
- UserNameSecurityTokenProvider.cs
- StringUtil.cs
- Margins.cs
- DataGridViewImageCell.cs
- XmlSiteMapProvider.cs
- SoapReflectionImporter.cs
- XmlBinaryReader.cs
- _KerberosClient.cs
- ReadOnlyDictionary.cs
- XamlReaderHelper.cs
- TableLayoutStyle.cs
- DeclaredTypeValidatorAttribute.cs
- MenuItemCollectionEditorDialog.cs
- RoutedEventArgs.cs
- EndpointAddressMessageFilterTable.cs
- ObjectView.cs
- MimeMapping.cs
- MarginCollapsingState.cs
- FilteredAttributeCollection.cs
- MimeTypeAttribute.cs
- DataObjectEventArgs.cs
- BoolExpr.cs
- XmlNamespaceDeclarationsAttribute.cs
- DeploymentSectionCache.cs
- OracleCommandBuilder.cs
- DataGridViewTextBoxColumn.cs
- HierarchicalDataBoundControl.cs
- PenThread.cs
- InvalidOperationException.cs
- MsmqDiagnostics.cs
- InfoCardServiceInstallComponent.cs
- WebSysDescriptionAttribute.cs
- MobileControlDesigner.cs
- Substitution.cs
- RegexTree.cs