Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / ImageBrush.cs / 1 / ImageBrush.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: This file contains the implementation of ImageBrush. // The ImageBrush is a TileBrush which defines its tile content // by use of an ImageSource. // // History: // // 04/29/2003 : adsmith - Created it. // 01/19/2005 : timothyc - Removed SizeViewboxToContent. Moved UpdateResource // to the generated file. //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PresentationCore; using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// ImageBrush - This TileBrush defines its content as an Image /// public sealed partial class ImageBrush : TileBrush { #region Constructors ////// Default constructor for ImageBrush. The resulting Brush has no content. /// public ImageBrush() { // We do this so that the property, when read, is consistent - not that // this will every actually affect drawing. } ////// ImageBrush Constructor where the image is set to the parameter's value /// /// The image source. public ImageBrush(ImageSource image) { ImageSource = image; } #endregion Constructors #region Protected methods ////// This node can introduce graphness /// internal override bool CanIntroduceGraphness() { if (ImageSource == null) { // If we don't have an ImageSource, we can't introduce // graphness right now, if we get an image source later // we'll precompute again. return false; } else { if (ImageSource.CanIntroduceGraphness()) { return true; } else { return false; } } } ////// Obtains the current bounds of the brush's content /// /// Output bounds of content protected override void GetContentBounds(out Rect contentBounds) { // Note, only implemented for DrawingImages. contentBounds = Rect.Empty; DrawingImage di = ImageSource as DrawingImage; if (di != null) { Drawing drawing = di.Drawing; if (drawing != null) { contentBounds = drawing.Bounds; } } } #endregion Protected methods #region Realization Support ////// Precompute is called during the frame preparation phase. Derived classes /// typically check if the brush requires realizations during this phase. /// internal override void Precompute() { ImageSource imageSource = ImageSource; if (imageSource != null) { imageSource.Precompute(); _requiresRealizationUpdates = imageSource.RequiresRealizationUpdates; } else { _requiresRealizationUpdates = false; } } ////// Checks if realization updates are required for this resource. /// internal override bool RequiresRealizationUpdates { get { return _requiresRealizationUpdates; } } ////// Derived classes must override this method and update realizations on dependent /// resources if required. /// internal override void UpdateRealizations(Rect fillShapeBounds, RealizationContext ctx) { if (_requiresRealizationUpdates) { Matrix m; ImageSource imageSource = ImageSource; Debug.Assert(imageSource != null); // Otherwise _requiresRealizationUpdates would be false. GetTileBrushMapping(fillShapeBounds, out m); ctx.TransformStack.Push(ref m, true); imageSource.UpdateRealizations(ctx); ctx.TransformStack.Pop(); } } #endregion private bool _requiresRealizationUpdates; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: This file contains the implementation of ImageBrush. // The ImageBrush is a TileBrush which defines its tile content // by use of an ImageSource. // // History: // // 04/29/2003 : adsmith - Created it. // 01/19/2005 : timothyc - Removed SizeViewboxToContent. Moved UpdateResource // to the generated file. //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PresentationCore; using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// ImageBrush - This TileBrush defines its content as an Image /// public sealed partial class ImageBrush : TileBrush { #region Constructors ////// Default constructor for ImageBrush. The resulting Brush has no content. /// public ImageBrush() { // We do this so that the property, when read, is consistent - not that // this will every actually affect drawing. } ////// ImageBrush Constructor where the image is set to the parameter's value /// /// The image source. public ImageBrush(ImageSource image) { ImageSource = image; } #endregion Constructors #region Protected methods ////// This node can introduce graphness /// internal override bool CanIntroduceGraphness() { if (ImageSource == null) { // If we don't have an ImageSource, we can't introduce // graphness right now, if we get an image source later // we'll precompute again. return false; } else { if (ImageSource.CanIntroduceGraphness()) { return true; } else { return false; } } } ////// Obtains the current bounds of the brush's content /// /// Output bounds of content protected override void GetContentBounds(out Rect contentBounds) { // Note, only implemented for DrawingImages. contentBounds = Rect.Empty; DrawingImage di = ImageSource as DrawingImage; if (di != null) { Drawing drawing = di.Drawing; if (drawing != null) { contentBounds = drawing.Bounds; } } } #endregion Protected methods #region Realization Support ////// Precompute is called during the frame preparation phase. Derived classes /// typically check if the brush requires realizations during this phase. /// internal override void Precompute() { ImageSource imageSource = ImageSource; if (imageSource != null) { imageSource.Precompute(); _requiresRealizationUpdates = imageSource.RequiresRealizationUpdates; } else { _requiresRealizationUpdates = false; } } ////// Checks if realization updates are required for this resource. /// internal override bool RequiresRealizationUpdates { get { return _requiresRealizationUpdates; } } ////// Derived classes must override this method and update realizations on dependent /// resources if required. /// internal override void UpdateRealizations(Rect fillShapeBounds, RealizationContext ctx) { if (_requiresRealizationUpdates) { Matrix m; ImageSource imageSource = ImageSource; Debug.Assert(imageSource != null); // Otherwise _requiresRealizationUpdates would be false. GetTileBrushMapping(fillShapeBounds, out m); ctx.TransformStack.Push(ref m, true); imageSource.UpdateRealizations(ctx); ctx.TransformStack.Pop(); } } #endregion private bool _requiresRealizationUpdates; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
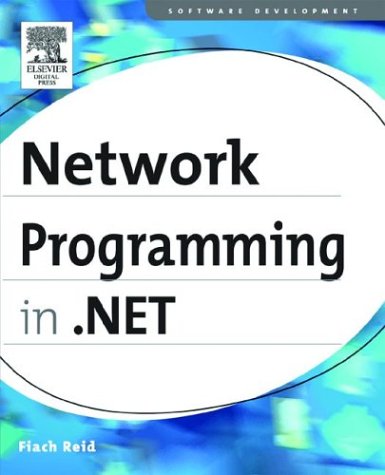
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MappingSource.cs
- Exceptions.cs
- AndCondition.cs
- DataObjectEventArgs.cs
- XmlSchemaGroupRef.cs
- CompilerInfo.cs
- ViewBase.cs
- StringSource.cs
- ParseChildrenAsPropertiesAttribute.cs
- ValidatedControlConverter.cs
- XmlSerializer.cs
- ProgressiveCrcCalculatingStream.cs
- PrintingPermission.cs
- InputLanguageProfileNotifySink.cs
- WebRequest.cs
- InputReportEventArgs.cs
- PixelShader.cs
- SqlXml.cs
- SignatureDescription.cs
- ValidatorCompatibilityHelper.cs
- CommandManager.cs
- XmlWriterTraceListener.cs
- SqlRetyper.cs
- ConfigXmlDocument.cs
- SQLInt32.cs
- ForEachDesigner.xaml.cs
- IApplicationTrustManager.cs
- RegexNode.cs
- CodeAttributeDeclaration.cs
- ExternalCalls.cs
- XmlILConstructAnalyzer.cs
- localization.cs
- TransformCollection.cs
- UriGenerator.cs
- TableLayoutSettingsTypeConverter.cs
- FontFamily.cs
- ApplicationSecurityManager.cs
- DiscoveryDocumentSearchPattern.cs
- SettingsPropertyCollection.cs
- ServerValidateEventArgs.cs
- FixedSOMPageElement.cs
- EllipseGeometry.cs
- XPathNavigator.cs
- DataServiceException.cs
- WorkflowHostingEndpoint.cs
- PathSegment.cs
- ServiceHost.cs
- Size3D.cs
- UiaCoreTypesApi.cs
- ClientProxyGenerator.cs
- ElementHost.cs
- EventManager.cs
- RoleManagerEventArgs.cs
- ToolStripItemDataObject.cs
- TextSerializer.cs
- JavaScriptSerializer.cs
- SafeRightsManagementPubHandle.cs
- SoapInteropTypes.cs
- dbdatarecord.cs
- MethodCallConverter.cs
- EdmError.cs
- DocumentCollection.cs
- IndexedGlyphRun.cs
- _ConnectionGroup.cs
- Hyperlink.cs
- XmlDataDocument.cs
- BrowserDefinition.cs
- WindowsTab.cs
- PopupControlService.cs
- iisPickupDirectory.cs
- OracleDateTime.cs
- __Error.cs
- BindingNavigator.cs
- followingquery.cs
- ClientOptions.cs
- _OverlappedAsyncResult.cs
- SerializationException.cs
- AuthorizationContext.cs
- oledbmetadatacollectionnames.cs
- HttpListenerTimeoutManager.cs
- SponsorHelper.cs
- FormViewUpdatedEventArgs.cs
- ExpressionStringBuilder.cs
- PointConverter.cs
- NamespaceDecl.cs
- DbProviderFactories.cs
- ConfigXmlReader.cs
- SHA1Managed.cs
- BrowserInteropHelper.cs
- ControlBindingsConverter.cs
- FacetEnabledSchemaElement.cs
- ArrayList.cs
- _ScatterGatherBuffers.cs
- CompositeDataBoundControl.cs
- MetadataCollection.cs
- ResolvedKeyFrameEntry.cs
- SiteMapDataSource.cs
- WorkflowServiceHostFactory.cs
- Row.cs
- ListBox.cs