Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Media / Animation / Generated / ThicknessAnimationBase.cs / 1 / ThicknessAnimationBase.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- // Allow use of presharp: #pragma warning suppress#pragma warning disable 1634, 1691 using MS.Internal; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; using MS.Internal.PresentationFramework; using SR=System.Windows.SR; using SRID=System.Windows.SRID; namespace System.Windows.Media.Animation { /// /// /// public abstract class ThicknessAnimationBase : AnimationTimeline { #region Constructors ////// Creates a new ThicknessAnimationBase. /// protected ThicknessAnimationBase() : base() { } #endregion #region Freezable ////// Creates a copy of this ThicknessAnimationBase /// ///The copy public new ThicknessAnimationBase Clone() { return (ThicknessAnimationBase)base.Clone(); } #endregion #region IAnimation ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public override sealed object GetCurrentValue(object defaultOriginValue, object defaultDestinationValue, AnimationClock animationClock) { // Verify that object arguments are non-null since we are a value type if (defaultOriginValue == null) { throw new ArgumentNullException("defaultOriginValue"); } if (defaultDestinationValue == null) { throw new ArgumentNullException("defaultDestinationValue"); } return GetCurrentValue((Thickness)defaultOriginValue, (Thickness)defaultDestinationValue, animationClock); } ////// Returns the type of the target property /// public override sealed Type TargetPropertyType { get { ReadPreamble(); return typeof(Thickness); } } #endregion #region Methods ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public Thickness GetCurrentValue(Thickness defaultOriginValue, Thickness defaultDestinationValue, AnimationClock animationClock) { ReadPreamble(); if (animationClock == null) { throw new ArgumentNullException("animationClock"); } // We check for null above but presharp doesn't notice so we suppress the // warning here. #pragma warning suppress 6506 if (animationClock.CurrentState == ClockState.Stopped) { return defaultDestinationValue; } /* if (!AnimatedTypeHelpers.IsValidAnimationValueThickness(defaultDestinationValue)) { throw new ArgumentException( SR.Get( SRID.Animation_InvalidBaseValue, defaultDestinationValue, defaultDestinationValue.GetType(), GetType()), "defaultDestinationValue"); } */ return GetCurrentValueCore(defaultOriginValue, defaultDestinationValue, animationClock); } ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// protected abstract Thickness GetCurrentValueCore(Thickness defaultOriginValue, Thickness defaultDestinationValue, AnimationClock animationClock); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- // Allow use of presharp: #pragma warning suppress#pragma warning disable 1634, 1691 using MS.Internal; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; using MS.Internal.PresentationFramework; using SR=System.Windows.SR; using SRID=System.Windows.SRID; namespace System.Windows.Media.Animation { /// /// /// public abstract class ThicknessAnimationBase : AnimationTimeline { #region Constructors ////// Creates a new ThicknessAnimationBase. /// protected ThicknessAnimationBase() : base() { } #endregion #region Freezable ////// Creates a copy of this ThicknessAnimationBase /// ///The copy public new ThicknessAnimationBase Clone() { return (ThicknessAnimationBase)base.Clone(); } #endregion #region IAnimation ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public override sealed object GetCurrentValue(object defaultOriginValue, object defaultDestinationValue, AnimationClock animationClock) { // Verify that object arguments are non-null since we are a value type if (defaultOriginValue == null) { throw new ArgumentNullException("defaultOriginValue"); } if (defaultDestinationValue == null) { throw new ArgumentNullException("defaultDestinationValue"); } return GetCurrentValue((Thickness)defaultOriginValue, (Thickness)defaultDestinationValue, animationClock); } ////// Returns the type of the target property /// public override sealed Type TargetPropertyType { get { ReadPreamble(); return typeof(Thickness); } } #endregion #region Methods ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public Thickness GetCurrentValue(Thickness defaultOriginValue, Thickness defaultDestinationValue, AnimationClock animationClock) { ReadPreamble(); if (animationClock == null) { throw new ArgumentNullException("animationClock"); } // We check for null above but presharp doesn't notice so we suppress the // warning here. #pragma warning suppress 6506 if (animationClock.CurrentState == ClockState.Stopped) { return defaultDestinationValue; } /* if (!AnimatedTypeHelpers.IsValidAnimationValueThickness(defaultDestinationValue)) { throw new ArgumentException( SR.Get( SRID.Animation_InvalidBaseValue, defaultDestinationValue, defaultDestinationValue.GetType(), GetType()), "defaultDestinationValue"); } */ return GetCurrentValueCore(defaultOriginValue, defaultDestinationValue, animationClock); } ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// protected abstract Thickness GetCurrentValueCore(Thickness defaultOriginValue, Thickness defaultDestinationValue, AnimationClock animationClock); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
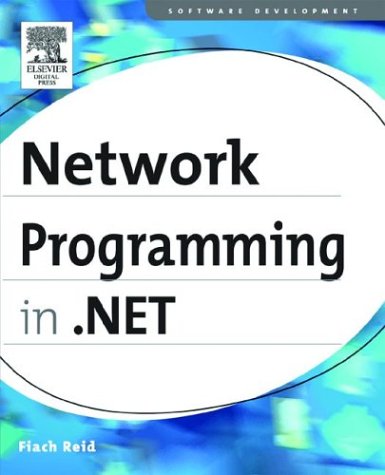
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataChangedEventManager.cs
- Enum.cs
- ArcSegment.cs
- FormatterServicesNoSerializableCheck.cs
- HttpFileCollection.cs
- ProcessModelInfo.cs
- SelectionWordBreaker.cs
- MemberDomainMap.cs
- Assembly.cs
- TextBox.cs
- XPathNavigatorException.cs
- SolidBrush.cs
- Part.cs
- ModelItemDictionary.cs
- ResourceFallbackManager.cs
- Propagator.Evaluator.cs
- LineServicesCallbacks.cs
- SimpleBitVector32.cs
- SqlServices.cs
- AdRotatorDesigner.cs
- SendKeys.cs
- ResolvePPIDRequest.cs
- BuildProviderUtils.cs
- SamlConditions.cs
- HttpCookie.cs
- CalloutQueueItem.cs
- QueryContext.cs
- _NetRes.cs
- ComboBoxAutomationPeer.cs
- XPathBinder.cs
- Delegate.cs
- ColumnMapVisitor.cs
- XmlParserContext.cs
- Timer.cs
- PointLightBase.cs
- PolicyManager.cs
- SspiSecurityTokenProvider.cs
- MediaPlayerState.cs
- CodeDOMUtility.cs
- CompleteWizardStep.cs
- ModuleConfigurationInfo.cs
- SamlAuthorizationDecisionStatement.cs
- ComplexBindingPropertiesAttribute.cs
- CorrelationScope.cs
- PathStreamGeometryContext.cs
- Intellisense.cs
- MULTI_QI.cs
- UnsafeNativeMethods.cs
- ErrorHandlerModule.cs
- RectAnimationBase.cs
- ExpressionBinding.cs
- MailWebEventProvider.cs
- ZoneLinkButton.cs
- SizeAnimationBase.cs
- RectangleHotSpot.cs
- xmlglyphRunInfo.cs
- WebPartVerbCollection.cs
- XmlSerializer.cs
- WebFormDesignerActionService.cs
- SqlTriggerContext.cs
- Rotation3D.cs
- DBDataPermission.cs
- EntityModelBuildProvider.cs
- UnauthorizedAccessException.cs
- QuaternionIndependentAnimationStorage.cs
- JsonReader.cs
- SystemSounds.cs
- SoapParser.cs
- BuildResult.cs
- ValidationErrorCollection.cs
- RenderDataDrawingContext.cs
- ByteFacetDescriptionElement.cs
- TaiwanLunisolarCalendar.cs
- MenuItemCollection.cs
- WebResponse.cs
- HMACSHA384.cs
- DataGridViewRowContextMenuStripNeededEventArgs.cs
- ExceptionValidationRule.cs
- XmlChildEnumerator.cs
- Graphics.cs
- ByteAnimation.cs
- WebPartZoneBase.cs
- CapabilitiesSection.cs
- Vector.cs
- TreeViewImageIndexConverter.cs
- BufferedGraphicsManager.cs
- ListItemParagraph.cs
- ObjectPropertyMapping.cs
- CacheSection.cs
- _ScatterGatherBuffers.cs
- LabelLiteral.cs
- ObservableCollection.cs
- SiteMembershipCondition.cs
- ActivityFunc.cs
- ServerValidateEventArgs.cs
- CqlLexerHelpers.cs
- RawMouseInputReport.cs
- DataGrid.cs
- XmlResolver.cs
- EnvironmentPermission.cs