Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / CommonUI / System / Drawing / SolidBrush.cs / 1 / SolidBrush.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /*************************************************************************\ * * Copyright (c) 1998-1999, Microsoft Corp. All Rights Reserved. * * Module Name: * * SolidBrush.cs * * Abstract: * * COM+ wrapper for GDI+ SolidBrush objects * * Revision History: * * 12/15/1998 [....] * Created it. * \**************************************************************************/ namespace System.Drawing { using System.Runtime.InteropServices; using System.Diagnostics; using System; using Microsoft.Win32; using System.ComponentModel; using System.Drawing.Internal; ////// /// public sealed class SolidBrush : Brush, ISystemColorTracker { // GDI+ doesn't understand system colors, so we need to cache the value here private Color color = Color.Empty; private bool immutable; /** * Create a new solid fill brush object */ ////// Defines a brush made up of a single color. Brushes are /// used to fill graphics shapes such as rectangles, ellipses, pies, polygons, and paths. /// ////// /// public SolidBrush(Color color) { this.color = color; IntPtr brush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateSolidFill(this.color.ToArgb(), out brush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeBrush(brush); if (color.IsSystemColor) SystemColorTracker.Add(this); } internal SolidBrush(Color color, bool immutable) : this(color) { this.immutable = immutable; } ////// Initializes a new instance of the ///class of the specified /// color. /// /// Constructor to initialized this object from a GDI+ Brush native pointer. /// internal SolidBrush( IntPtr nativeBrush ) { Debug.Assert( nativeBrush != IntPtr.Zero, "Initializing native brush with null." ); SetNativeBrush( nativeBrush ); } ////// /// Creates an exact copy of this public override object Clone() { IntPtr cloneBrush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCloneBrush(new HandleRef(this, this.NativeBrush), out cloneBrush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); // We intentionally lose the "immutable" bit. return new SolidBrush(cloneBrush); } ///. /// protected override void Dispose(bool disposing) { if (!disposing) { immutable = false; } else if (immutable) { throw new ArgumentException(SR.GetString(SR.CantChangeImmutableObjects, "Brush")); } base.Dispose(disposing); } /// /// /// public Color Color { get { if (this.color == Color.Empty) { int colorARGB = 0; int status = SafeNativeMethods.Gdip.GdipGetSolidFillColor(new HandleRef(this, this.NativeBrush), out colorARGB); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); this.color = Color.FromArgb(colorARGB); } // GDI+ doesn't understand system colors, so we can't use GdipGetSolidFillColor in the general case return this.color; } set { if (immutable) { throw new ArgumentException(SR.GetString(SR.CantChangeImmutableObjects, "Brush")); } if( this.color != value ) { Color oldColor = this.color; InternalSetColor(value); // if (value.IsSystemColor && !oldColor.IsSystemColor) { SystemColorTracker.Add(this); } } } } // Sets the color even if the brush is considered immutable private void InternalSetColor(Color value) { int status = SafeNativeMethods.Gdip.GdipSetSolidFillColor(new HandleRef(this, this.NativeBrush), value.ToArgb()); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); this.color = value; } ////// The color of this ///. /// /// void ISystemColorTracker.OnSystemColorChanged() { if( this.NativeBrush != IntPtr.Zero ){ InternalSetColor(color); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
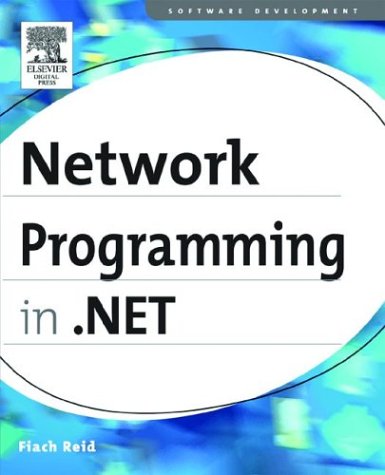
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesignerHelpers.cs
- NumericPagerField.cs
- MarkupExtensionParser.cs
- PrintPageEvent.cs
- PersonalizableAttribute.cs
- OrderedDictionary.cs
- _ShellExpression.cs
- ListDictionary.cs
- DateTimeFormat.cs
- SByteConverter.cs
- SharedUtils.cs
- InplaceBitmapMetadataWriter.cs
- DataObjectCopyingEventArgs.cs
- HashMembershipCondition.cs
- BindingManagerDataErrorEventArgs.cs
- HttpListenerRequestUriBuilder.cs
- ServiceBusyException.cs
- RoutedPropertyChangedEventArgs.cs
- PropertyEmitterBase.cs
- DrawingCollection.cs
- FieldMetadata.cs
- EncoderFallback.cs
- ScrollBarAutomationPeer.cs
- DataGridViewComboBoxColumnDesigner.cs
- RootCodeDomSerializer.cs
- MultipartContentParser.cs
- ComPlusDiagnosticTraceRecords.cs
- RelatedCurrencyManager.cs
- MetadataProperty.cs
- DetailsViewInsertEventArgs.cs
- ChannelAcceptor.cs
- TranslateTransform.cs
- RedirectionProxy.cs
- SelectionProcessor.cs
- basecomparevalidator.cs
- PageContentCollection.cs
- TypeConverterHelper.cs
- ErrorWebPart.cs
- VBIdentifierDesigner.xaml.cs
- _ContextAwareResult.cs
- ScrollData.cs
- StoreItemCollection.Loader.cs
- CollectionViewGroupRoot.cs
- PerformanceCounterLib.cs
- LogConverter.cs
- DataServiceProviderMethods.cs
- NavigatorInput.cs
- ActivityTypeDesigner.xaml.cs
- TypeNameParser.cs
- LocalizableResourceBuilder.cs
- PackageRelationship.cs
- ToolStripItemRenderEventArgs.cs
- UserCancellationException.cs
- InvalidChannelBindingException.cs
- DataGridColumnHeader.cs
- XhtmlBasicTextViewAdapter.cs
- ExpressionNode.cs
- CopyAction.cs
- StrokeRenderer.cs
- DesignBindingValueUIHandler.cs
- PropertyGrid.cs
- TextEncodedRawTextWriter.cs
- XmlSchemaValidator.cs
- StateManagedCollection.cs
- RemotingException.cs
- AssemblyFilter.cs
- DataGridViewDataConnection.cs
- OutputCacheProfileCollection.cs
- TokenizerHelper.cs
- GridViewUpdatedEventArgs.cs
- CodeConditionStatement.cs
- FontWeight.cs
- ScriptServiceAttribute.cs
- Int16Converter.cs
- WebPartTransformerCollection.cs
- ObjectSelectorEditor.cs
- milexports.cs
- NativeMethods.cs
- TextRangeSerialization.cs
- figurelengthconverter.cs
- BindingSource.cs
- DataGridViewCellPaintingEventArgs.cs
- HMACSHA256.cs
- sqlmetadatafactory.cs
- PointAnimationUsingPath.cs
- DateTimeConverter.cs
- SchemaImporterExtensionsSection.cs
- StyleXamlParser.cs
- DownloadProgressEventArgs.cs
- MenuScrollingVisibilityConverter.cs
- AdvancedBindingPropertyDescriptor.cs
- BookmarkInfo.cs
- TextBoxBase.cs
- HtmlInputImage.cs
- HtmlUtf8RawTextWriter.cs
- DynamicResourceExtensionConverter.cs
- NameService.cs
- ModulesEntry.cs
- WizardForm.cs
- ValidatorAttribute.cs