Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / DataObjectCopyingEventArgs.cs / 1 / DataObjectCopyingEventArgs.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: DataObject.Copying event arguments // //--------------------------------------------------------------------------- using System; namespace System.Windows { ////// Arguments for the DataObject.Copying event. /// /// The DataObject.Copying event is raised when an editor /// has converted a content of selection into all appropriate /// clipboard data formats, collected them all in DataObject /// and is ready to put the objet onto the Clipboard. /// An application can inspect DataObject, change, remove or /// add some data formats into it and decide whether to proceed /// with the copying or cancel it. /// public sealed class DataObjectCopyingEventArgs : DataObjectEventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates an arguments for a DataObject.Copying event. /// This object created by editors executing a Copy and Drag. /// /// /// DataObject filled in with all appropriate data formats /// and ready for putting into the Clipboard /// or to used in dragging gesture. /// /// /// A flag indicating if this operation is part of drag/drop. /// Copying event is fired on drag start. /// public DataObjectCopyingEventArgs(IDataObject dataObject, bool isDragDrop) // : base(System.Windows.DataObject.CopyingEvent, isDragDrop) { if (dataObject == null) { throw new ArgumentNullException("dataObject"); } _dataObject = dataObject; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// DataObject filled in with all appropriate data formats /// and ready for putting into the Clipboard. /// public IDataObject DataObject { get { return _dataObject; } } #endregion Public Properties #region Protected Methods //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ ////// The mechanism used to call the type-specific handler on the target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { DataObjectCopyingEventHandler handler = (DataObjectCopyingEventHandler)genericHandler; handler(genericTarget, this); } #endregion Protected Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private IDataObject _dataObject; #endregion Private Fields } ////// The delegate to use for handlers that receive the DataObject.Copying event. /// ////// A handler for DataObject.Copying event. /// The handler is expected to inspect the content of a data object /// passed via event arguments (DataObjectCopyingEventArgs.DataObject) /// and add additional (custom) data format to it. /// It's also possible for the handler to change /// the contents of other data formats already put on DataObject /// or even remove some of those formats. /// All this happens before DataObject is put on /// the Clipboard (in copy operation) or before DragDrop /// process starts. /// The handler can cancel the whole copying event /// by calling DataObjectCopyingEventArgs.CancelCommand method. /// For the case of Copy a command will be cancelled, /// for the case of DragDrop a dragdrop process will be /// terminated in the beginning. /// public delegate void DataObjectCopyingEventHandler(object sender, DataObjectCopyingEventArgs e); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: DataObject.Copying event arguments // //--------------------------------------------------------------------------- using System; namespace System.Windows { ////// Arguments for the DataObject.Copying event. /// /// The DataObject.Copying event is raised when an editor /// has converted a content of selection into all appropriate /// clipboard data formats, collected them all in DataObject /// and is ready to put the objet onto the Clipboard. /// An application can inspect DataObject, change, remove or /// add some data formats into it and decide whether to proceed /// with the copying or cancel it. /// public sealed class DataObjectCopyingEventArgs : DataObjectEventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates an arguments for a DataObject.Copying event. /// This object created by editors executing a Copy and Drag. /// /// /// DataObject filled in with all appropriate data formats /// and ready for putting into the Clipboard /// or to used in dragging gesture. /// /// /// A flag indicating if this operation is part of drag/drop. /// Copying event is fired on drag start. /// public DataObjectCopyingEventArgs(IDataObject dataObject, bool isDragDrop) // : base(System.Windows.DataObject.CopyingEvent, isDragDrop) { if (dataObject == null) { throw new ArgumentNullException("dataObject"); } _dataObject = dataObject; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// DataObject filled in with all appropriate data formats /// and ready for putting into the Clipboard. /// public IDataObject DataObject { get { return _dataObject; } } #endregion Public Properties #region Protected Methods //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ ////// The mechanism used to call the type-specific handler on the target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { DataObjectCopyingEventHandler handler = (DataObjectCopyingEventHandler)genericHandler; handler(genericTarget, this); } #endregion Protected Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private IDataObject _dataObject; #endregion Private Fields } ////// The delegate to use for handlers that receive the DataObject.Copying event. /// ////// A handler for DataObject.Copying event. /// The handler is expected to inspect the content of a data object /// passed via event arguments (DataObjectCopyingEventArgs.DataObject) /// and add additional (custom) data format to it. /// It's also possible for the handler to change /// the contents of other data formats already put on DataObject /// or even remove some of those formats. /// All this happens before DataObject is put on /// the Clipboard (in copy operation) or before DragDrop /// process starts. /// The handler can cancel the whole copying event /// by calling DataObjectCopyingEventArgs.CancelCommand method. /// For the case of Copy a command will be cancelled, /// for the case of DragDrop a dragdrop process will be /// terminated in the beginning. /// public delegate void DataObjectCopyingEventHandler(object sender, DataObjectCopyingEventArgs e); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
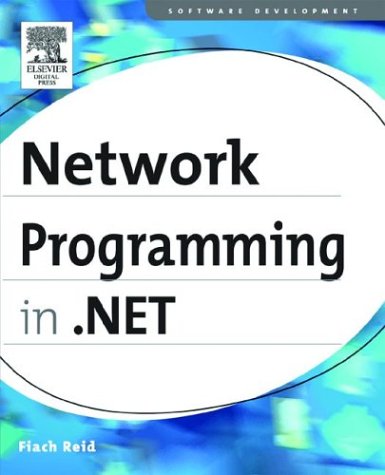
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Button.cs
- ObjectStateFormatter.cs
- HttpRequestCacheValidator.cs
- QEncodedStream.cs
- LocalServiceSecuritySettingsElement.cs
- SizeAnimationClockResource.cs
- CheckBoxRenderer.cs
- SplitterPanel.cs
- FixedPage.cs
- PrimitiveType.cs
- FragmentNavigationEventArgs.cs
- RC2CryptoServiceProvider.cs
- CodeArgumentReferenceExpression.cs
- webclient.cs
- AssemblyHash.cs
- TextTreeText.cs
- SystemTcpConnection.cs
- MSHTMLHost.cs
- Interlocked.cs
- CryptoProvider.cs
- Schedule.cs
- ToolStripLocationCancelEventArgs.cs
- Overlapped.cs
- ApplicationDirectory.cs
- RegexRunnerFactory.cs
- XmlNodeChangedEventArgs.cs
- ListViewInsertionMark.cs
- WebPartConnectionsCloseVerb.cs
- X509Utils.cs
- SecurityUtils.cs
- ExceptionNotification.cs
- ObjectStateFormatter.cs
- SetterBaseCollection.cs
- CustomErrorsSectionWrapper.cs
- TrueReadOnlyCollection.cs
- CodeNamespace.cs
- PerformanceCounterManager.cs
- WinEventQueueItem.cs
- DataTemplateSelector.cs
- UTF8Encoding.cs
- XmlSchemaChoice.cs
- PtsContext.cs
- ValidationPropertyAttribute.cs
- DependencyObjectType.cs
- CalculatedColumn.cs
- BaseTemplateBuildProvider.cs
- RuntimeDelegateArgument.cs
- CommonGetThemePartSize.cs
- PolicyUtility.cs
- ProgressBarBrushConverter.cs
- TextTreeExtractElementUndoUnit.cs
- HtmlElementErrorEventArgs.cs
- SmiEventSink_Default.cs
- SelectionRangeConverter.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- CodeParameterDeclarationExpressionCollection.cs
- LinkLabel.cs
- NetSectionGroup.cs
- FusionWrap.cs
- BaseValidatorDesigner.cs
- KeyBinding.cs
- ReachFixedPageSerializerAsync.cs
- TextModifierScope.cs
- ImageCodecInfo.cs
- NotConverter.cs
- ToolZone.cs
- MouseWheelEventArgs.cs
- TriState.cs
- AppDomainResourcePerfCounters.cs
- ProvidePropertyAttribute.cs
- CodeSubDirectoriesCollection.cs
- KernelTypeValidation.cs
- HiddenFieldPageStatePersister.cs
- UdpRetransmissionSettings.cs
- LinkedResource.cs
- XmlNamedNodeMap.cs
- XmlSchemaFacet.cs
- MessageBox.cs
- ErrorHandler.cs
- XmlUtil.cs
- SafeRightsManagementPubHandle.cs
- MobileTextWriter.cs
- IDReferencePropertyAttribute.cs
- SimpleWebHandlerParser.cs
- PartialCachingControl.cs
- storepermissionattribute.cs
- PersonalizationProvider.cs
- SmiMetaDataProperty.cs
- RoleManagerEventArgs.cs
- ButtonBase.cs
- SQLSingle.cs
- DataGridViewCellContextMenuStripNeededEventArgs.cs
- CatalogZoneBase.cs
- FactoryId.cs
- RMEnrollmentPage1.cs
- userdatakeys.cs
- BitSet.cs
- TextElementCollectionHelper.cs
- QueryContinueDragEvent.cs
- DecimalFormatter.cs