Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / MIT / System / Web / UI / MobileControls / Design / BaseValidatorDesigner.cs / 1305376 / BaseValidatorDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.Design.MobileControls { using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.IO; using System.Web.UI; using System.Web.UI.Design; using System.Web.UI.WebControls; using System.Web.UI.MobileControls.Adapters; using System.Web.UI.Design.MobileControls.Adapters; ////// [ System.Security.Permissions.SecurityPermission(System.Security.Permissions.SecurityAction.Demand, Flags=System.Security.Permissions.SecurityPermissionFlag.UnmanagedCode) ] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] internal class BaseValidatorDesigner : MobileControlDesigner { private System.Web.UI.MobileControls.BaseValidator _baseValidator; ////// Provides /// a designer for controls derived from ValidatorBase. /// ////// /// /// The control element being designed. /// ////// Initializes the designer. /// ////// ////// This is called by the designer host to establish the component being /// designed. /// ///public override void Initialize(IComponent component) { Debug.Assert(component is System.Web.UI.MobileControls.BaseValidator, "BaseValidatorDesigner.Initialize - Invalid BaseValidator Control"); _baseValidator = (System.Web.UI.MobileControls.BaseValidator) component; base.Initialize(component); // remove the contained asp validator within mobile validator so that it won't // be persisted. for (int i = _baseValidator.Controls.Count - 1; i >= 0; i--) { Control child = _baseValidator.Controls[i]; if (child is System.Web.UI.WebControls.BaseValidator) { _baseValidator.Controls.RemoveAt(i); } } } /// /// ////// Gets the design time HTML of ValidatorBase controls. /// ////// protected override String GetDesignTimeNormalHtml() { Debug.Assert(_baseValidator.Text != null); String originalText = _baseValidator.ErrorMessage; ValidatorDisplay validatorDisplay = _baseValidator.Display; bool blankText = (validatorDisplay == ValidatorDisplay.None || (originalText.Trim().Length == 0 && _baseValidator.Text.Trim().Length == 0)); if (blankText) { _baseValidator.ErrorMessage = "[" + _baseValidator.ID + "]"; } DesignerTextWriter tw = new DesignerTextWriter(); _baseValidator.Adapter.Render(tw); if (blankText) { _baseValidator.ErrorMessage = originalText; } return tw.ToString(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// The design time /// HTML of the control. /// ///
Link Menu
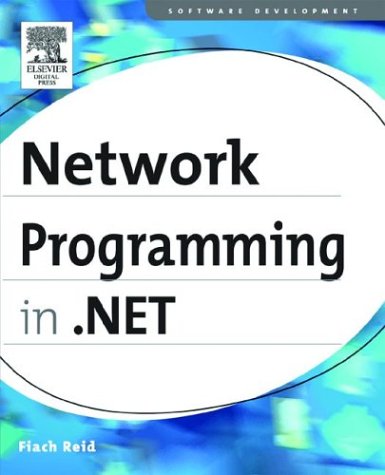
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ReferenceEqualityComparer.cs
- DataException.cs
- InfoCardService.cs
- DeclarativeConditionsCollection.cs
- HttpCachePolicy.cs
- ComponentResourceManager.cs
- IsolatedStorageFilePermission.cs
- OleServicesContext.cs
- MultiSelector.cs
- TraceLevelStore.cs
- LocationChangedEventArgs.cs
- HelpEvent.cs
- ToolStripLabel.cs
- JournalNavigationScope.cs
- X509Utils.cs
- DelayDesigner.cs
- SimpleTableProvider.cs
- MachineKeyConverter.cs
- PersonalizationDictionary.cs
- UserControlParser.cs
- WinInet.cs
- DiagnosticTraceSource.cs
- InputQueue.cs
- Transform.cs
- PrintPreviewGraphics.cs
- PackageFilter.cs
- BitVector32.cs
- GcHandle.cs
- SecurityIdentifierElement.cs
- ResolveNameEventArgs.cs
- SerializationHelper.cs
- ScrollItemPatternIdentifiers.cs
- ScrollableControl.cs
- Header.cs
- TextTreeText.cs
- GPRECT.cs
- TrackingQueryElement.cs
- Pair.cs
- InputProcessorProfiles.cs
- WebServiceData.cs
- SimpleBitVector32.cs
- FactoryMaker.cs
- Calendar.cs
- GridViewRowPresenterBase.cs
- ToolStripItem.cs
- SqlNotificationRequest.cs
- RegisteredDisposeScript.cs
- ButtonChrome.cs
- ServiceDescriptionSerializer.cs
- __Filters.cs
- Attributes.cs
- RemoteWebConfigurationHostServer.cs
- CellTreeNode.cs
- BuildManager.cs
- ToolStripHighContrastRenderer.cs
- SettingsProperty.cs
- AsymmetricKeyExchangeDeformatter.cs
- DoubleAnimation.cs
- SchemaElementDecl.cs
- SmtpDigestAuthenticationModule.cs
- PTUtility.cs
- GlyphRunDrawing.cs
- TextServicesCompartmentEventSink.cs
- CodeDOMUtility.cs
- MinimizableAttributeTypeConverter.cs
- Vector3DKeyFrameCollection.cs
- RenderData.cs
- OverflowException.cs
- EntityCommandCompilationException.cs
- StylusPointPropertyId.cs
- TemplateInstanceAttribute.cs
- PolygonHotSpot.cs
- OracleCommandBuilder.cs
- XmlSchemaSet.cs
- ToolStripDropDownMenu.cs
- SourceExpressionException.cs
- SafeFreeMibTable.cs
- WebPartAuthorizationEventArgs.cs
- LogSwitch.cs
- CommonDialog.cs
- PersonalizationState.cs
- XmlSignatureProperties.cs
- TypeRestriction.cs
- safex509handles.cs
- ArraySubsetEnumerator.cs
- GeneralTransform3DCollection.cs
- Terminate.cs
- LiteralControl.cs
- TableCell.cs
- PrinterResolution.cs
- DynamicValueConverter.cs
- WebPartsPersonalization.cs
- ZipIOFileItemStream.cs
- ChannelServices.cs
- RegisteredArrayDeclaration.cs
- LayoutManager.cs
- TableItemStyle.cs
- SmtpCommands.cs
- ChameleonKey.cs
- ThreadAbortException.cs