Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / Animation / Generated / Vector3DKeyFrameCollection.cs / 1 / Vector3DKeyFrameCollection.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; namespace System.Windows.Media.Animation { ////// This collection is used in conjunction with a KeyFrameVector3DAnimation /// to animate a Vector3D property value along a set of key frames. /// public class Vector3DKeyFrameCollection : Freezable, IList { #region Data private List_keyFrames; private static Vector3DKeyFrameCollection s_emptyCollection; #endregion #region Constructors /// /// Creates a new Vector3DKeyFrameCollection. /// public Vector3DKeyFrameCollection() : base() { _keyFrames = new List< Vector3DKeyFrame>(2); } #endregion #region Static Methods ////// An empty Vector3DKeyFrameCollection. /// public static Vector3DKeyFrameCollection Empty { get { if (s_emptyCollection == null) { Vector3DKeyFrameCollection emptyCollection = new Vector3DKeyFrameCollection(); emptyCollection._keyFrames = new List< Vector3DKeyFrame>(0); emptyCollection.Freeze(); s_emptyCollection = emptyCollection; } return s_emptyCollection; } } #endregion #region Freezable ////// Creates a freezable copy of this Vector3DKeyFrameCollection. /// ///The copy public new Vector3DKeyFrameCollection Clone() { return (Vector3DKeyFrameCollection)base.Clone(); } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new Vector3DKeyFrameCollection(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { Vector3DKeyFrameCollection sourceCollection = (Vector3DKeyFrameCollection) sourceFreezable; base.CloneCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< Vector3DKeyFrame>(count); for (int i = 0; i < count; i++) { Vector3DKeyFrame keyFrame = (Vector3DKeyFrame)sourceCollection._keyFrames[i].Clone(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { Vector3DKeyFrameCollection sourceCollection = (Vector3DKeyFrameCollection) sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< Vector3DKeyFrame>(count); for (int i = 0; i < count; i++) { Vector3DKeyFrame keyFrame = (Vector3DKeyFrame)sourceCollection._keyFrames[i].CloneCurrentValue(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { Vector3DKeyFrameCollection sourceCollection = (Vector3DKeyFrameCollection) sourceFreezable; base.GetAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< Vector3DKeyFrame>(count); for (int i = 0; i < count; i++) { Vector3DKeyFrame keyFrame = (Vector3DKeyFrame)sourceCollection._keyFrames[i].GetAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { Vector3DKeyFrameCollection sourceCollection = (Vector3DKeyFrameCollection) sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< Vector3DKeyFrame>(count); for (int i = 0; i < count; i++) { Vector3DKeyFrame keyFrame = (Vector3DKeyFrame)sourceCollection._keyFrames[i].GetCurrentValueAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetCurrentValueAsFrozenCore . ////// /// protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); for (int i = 0; i < _keyFrames.Count && canFreeze; i++) { canFreeze &= Freezable.Freeze(_keyFrames[i], isChecking); } return canFreeze; } #endregion #region IEnumerable ////// Returns an enumerator of the Vector3DKeyFrames in the collection. /// public IEnumerator GetEnumerator() { ReadPreamble(); return _keyFrames.GetEnumerator(); } #endregion #region ICollection ////// Returns the number of Vector3DKeyFrames in the collection. /// public int Count { get { ReadPreamble(); return _keyFrames.Count; } } ////// See public bool IsSynchronized { get { ReadPreamble(); return (IsFrozen || Dispatcher != null); } } ///ICollection.IsSynchronized . ////// See public object SyncRoot { get { ReadPreamble(); return ((ICollection)_keyFrames).SyncRoot; } } ///ICollection.SyncRoot . ////// Copies all of the Vector3DKeyFrames in the collection to an /// array. /// void ICollection.CopyTo(Array array, int index) { ReadPreamble(); ((ICollection)_keyFrames).CopyTo(array, index); } ////// Copies all of the Vector3DKeyFrames in the collection to an /// array of Vector3DKeyFrames. /// public void CopyTo(Vector3DKeyFrame[] array, int index) { ReadPreamble(); _keyFrames.CopyTo(array, index); } #endregion #region IList ////// Adds a Vector3DKeyFrame to the collection. /// int IList.Add(object keyFrame) { return Add((Vector3DKeyFrame)keyFrame); } ////// Adds a Vector3DKeyFrame to the collection. /// public int Add(Vector3DKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Add(keyFrame); WritePostscript(); return _keyFrames.Count - 1; } ////// Removes all Vector3DKeyFrames from the collection. /// public void Clear() { WritePreamble(); if (_keyFrames.Count > 0) { for (int i = 0; i < _keyFrames.Count; i++) { OnFreezablePropertyChanged(_keyFrames[i], null); } _keyFrames.Clear(); WritePostscript(); } } ////// Returns true of the collection contains the given Vector3DKeyFrame. /// bool IList.Contains(object keyFrame) { return Contains((Vector3DKeyFrame)keyFrame); } ////// Returns true of the collection contains the given Vector3DKeyFrame. /// public bool Contains(Vector3DKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.Contains(keyFrame); } ////// Returns the index of a given Vector3DKeyFrame in the collection. /// int IList.IndexOf(object keyFrame) { return IndexOf((Vector3DKeyFrame)keyFrame); } ////// Returns the index of a given Vector3DKeyFrame in the collection. /// public int IndexOf(Vector3DKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.IndexOf(keyFrame); } ////// Inserts a Vector3DKeyFrame into a specific location in the collection. /// void IList.Insert(int index, object keyFrame) { Insert(index, (Vector3DKeyFrame)keyFrame); } ////// Inserts a Vector3DKeyFrame into a specific location in the collection. /// public void Insert(int index, Vector3DKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Insert(index, keyFrame); WritePostscript(); } ////// Returns true if the collection is frozen. /// public bool IsFixedSize { get { ReadPreamble(); return IsFrozen; } } ////// Returns true if the collection is frozen. /// public bool IsReadOnly { get { ReadPreamble(); return IsFrozen; } } ////// Removes a Vector3DKeyFrame from the collection. /// void IList.Remove(object keyFrame) { Remove((Vector3DKeyFrame)keyFrame); } ////// Removes a Vector3DKeyFrame from the collection. /// public void Remove(Vector3DKeyFrame keyFrame) { WritePreamble(); if (_keyFrames.Contains(keyFrame)) { OnFreezablePropertyChanged(keyFrame, null); _keyFrames.Remove(keyFrame); WritePostscript(); } } ////// Removes the Vector3DKeyFrame at the specified index from the collection. /// public void RemoveAt(int index) { WritePreamble(); OnFreezablePropertyChanged(_keyFrames[index], null); _keyFrames.RemoveAt(index); WritePostscript(); } ////// Gets or sets the Vector3DKeyFrame at a given index. /// object IList.this[int index] { get { return this[index]; } set { this[index] = (Vector3DKeyFrame)value; } } ////// Gets or sets the Vector3DKeyFrame at a given index. /// public Vector3DKeyFrame this[int index] { get { ReadPreamble(); return _keyFrames[index]; } set { if (value == null) { throw new ArgumentNullException(String.Format(CultureInfo.InvariantCulture, "Vector3DKeyFrameCollection[{0}]", index)); } WritePreamble(); if (value != _keyFrames[index]) { OnFreezablePropertyChanged(_keyFrames[index], value); _keyFrames[index] = value; Debug.Assert(_keyFrames[index] != null); WritePostscript(); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; namespace System.Windows.Media.Animation { ////// This collection is used in conjunction with a KeyFrameVector3DAnimation /// to animate a Vector3D property value along a set of key frames. /// public class Vector3DKeyFrameCollection : Freezable, IList { #region Data private List_keyFrames; private static Vector3DKeyFrameCollection s_emptyCollection; #endregion #region Constructors /// /// Creates a new Vector3DKeyFrameCollection. /// public Vector3DKeyFrameCollection() : base() { _keyFrames = new List< Vector3DKeyFrame>(2); } #endregion #region Static Methods ////// An empty Vector3DKeyFrameCollection. /// public static Vector3DKeyFrameCollection Empty { get { if (s_emptyCollection == null) { Vector3DKeyFrameCollection emptyCollection = new Vector3DKeyFrameCollection(); emptyCollection._keyFrames = new List< Vector3DKeyFrame>(0); emptyCollection.Freeze(); s_emptyCollection = emptyCollection; } return s_emptyCollection; } } #endregion #region Freezable ////// Creates a freezable copy of this Vector3DKeyFrameCollection. /// ///The copy public new Vector3DKeyFrameCollection Clone() { return (Vector3DKeyFrameCollection)base.Clone(); } ////// Implementation of ///Freezable.CreateInstanceCore . ///The new Freezable. protected override Freezable CreateInstanceCore() { return new Vector3DKeyFrameCollection(); } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { Vector3DKeyFrameCollection sourceCollection = (Vector3DKeyFrameCollection) sourceFreezable; base.CloneCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< Vector3DKeyFrame>(count); for (int i = 0; i < count; i++) { Vector3DKeyFrame keyFrame = (Vector3DKeyFrame)sourceCollection._keyFrames[i].Clone(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { Vector3DKeyFrameCollection sourceCollection = (Vector3DKeyFrameCollection) sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< Vector3DKeyFrame>(count); for (int i = 0; i < count; i++) { Vector3DKeyFrame keyFrame = (Vector3DKeyFrame)sourceCollection._keyFrames[i].CloneCurrentValue(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable sourceFreezable) { Vector3DKeyFrameCollection sourceCollection = (Vector3DKeyFrameCollection) sourceFreezable; base.GetAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< Vector3DKeyFrame>(count); for (int i = 0; i < count; i++) { Vector3DKeyFrame keyFrame = (Vector3DKeyFrame)sourceCollection._keyFrames[i].GetAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable sourceFreezable) { Vector3DKeyFrameCollection sourceCollection = (Vector3DKeyFrameCollection) sourceFreezable; base.GetCurrentValueAsFrozenCore(sourceFreezable); int count = sourceCollection._keyFrames.Count; _keyFrames = new List< Vector3DKeyFrame>(count); for (int i = 0; i < count; i++) { Vector3DKeyFrame keyFrame = (Vector3DKeyFrame)sourceCollection._keyFrames[i].GetCurrentValueAsFrozen(); _keyFrames.Add(keyFrame); OnFreezablePropertyChanged(null, keyFrame); } } ///Freezable.GetCurrentValueAsFrozenCore . ////// /// protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); for (int i = 0; i < _keyFrames.Count && canFreeze; i++) { canFreeze &= Freezable.Freeze(_keyFrames[i], isChecking); } return canFreeze; } #endregion #region IEnumerable ////// Returns an enumerator of the Vector3DKeyFrames in the collection. /// public IEnumerator GetEnumerator() { ReadPreamble(); return _keyFrames.GetEnumerator(); } #endregion #region ICollection ////// Returns the number of Vector3DKeyFrames in the collection. /// public int Count { get { ReadPreamble(); return _keyFrames.Count; } } ////// See public bool IsSynchronized { get { ReadPreamble(); return (IsFrozen || Dispatcher != null); } } ///ICollection.IsSynchronized . ////// See public object SyncRoot { get { ReadPreamble(); return ((ICollection)_keyFrames).SyncRoot; } } ///ICollection.SyncRoot . ////// Copies all of the Vector3DKeyFrames in the collection to an /// array. /// void ICollection.CopyTo(Array array, int index) { ReadPreamble(); ((ICollection)_keyFrames).CopyTo(array, index); } ////// Copies all of the Vector3DKeyFrames in the collection to an /// array of Vector3DKeyFrames. /// public void CopyTo(Vector3DKeyFrame[] array, int index) { ReadPreamble(); _keyFrames.CopyTo(array, index); } #endregion #region IList ////// Adds a Vector3DKeyFrame to the collection. /// int IList.Add(object keyFrame) { return Add((Vector3DKeyFrame)keyFrame); } ////// Adds a Vector3DKeyFrame to the collection. /// public int Add(Vector3DKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Add(keyFrame); WritePostscript(); return _keyFrames.Count - 1; } ////// Removes all Vector3DKeyFrames from the collection. /// public void Clear() { WritePreamble(); if (_keyFrames.Count > 0) { for (int i = 0; i < _keyFrames.Count; i++) { OnFreezablePropertyChanged(_keyFrames[i], null); } _keyFrames.Clear(); WritePostscript(); } } ////// Returns true of the collection contains the given Vector3DKeyFrame. /// bool IList.Contains(object keyFrame) { return Contains((Vector3DKeyFrame)keyFrame); } ////// Returns true of the collection contains the given Vector3DKeyFrame. /// public bool Contains(Vector3DKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.Contains(keyFrame); } ////// Returns the index of a given Vector3DKeyFrame in the collection. /// int IList.IndexOf(object keyFrame) { return IndexOf((Vector3DKeyFrame)keyFrame); } ////// Returns the index of a given Vector3DKeyFrame in the collection. /// public int IndexOf(Vector3DKeyFrame keyFrame) { ReadPreamble(); return _keyFrames.IndexOf(keyFrame); } ////// Inserts a Vector3DKeyFrame into a specific location in the collection. /// void IList.Insert(int index, object keyFrame) { Insert(index, (Vector3DKeyFrame)keyFrame); } ////// Inserts a Vector3DKeyFrame into a specific location in the collection. /// public void Insert(int index, Vector3DKeyFrame keyFrame) { if (keyFrame == null) { throw new ArgumentNullException("keyFrame"); } WritePreamble(); OnFreezablePropertyChanged(null, keyFrame); _keyFrames.Insert(index, keyFrame); WritePostscript(); } ////// Returns true if the collection is frozen. /// public bool IsFixedSize { get { ReadPreamble(); return IsFrozen; } } ////// Returns true if the collection is frozen. /// public bool IsReadOnly { get { ReadPreamble(); return IsFrozen; } } ////// Removes a Vector3DKeyFrame from the collection. /// void IList.Remove(object keyFrame) { Remove((Vector3DKeyFrame)keyFrame); } ////// Removes a Vector3DKeyFrame from the collection. /// public void Remove(Vector3DKeyFrame keyFrame) { WritePreamble(); if (_keyFrames.Contains(keyFrame)) { OnFreezablePropertyChanged(keyFrame, null); _keyFrames.Remove(keyFrame); WritePostscript(); } } ////// Removes the Vector3DKeyFrame at the specified index from the collection. /// public void RemoveAt(int index) { WritePreamble(); OnFreezablePropertyChanged(_keyFrames[index], null); _keyFrames.RemoveAt(index); WritePostscript(); } ////// Gets or sets the Vector3DKeyFrame at a given index. /// object IList.this[int index] { get { return this[index]; } set { this[index] = (Vector3DKeyFrame)value; } } ////// Gets or sets the Vector3DKeyFrame at a given index. /// public Vector3DKeyFrame this[int index] { get { ReadPreamble(); return _keyFrames[index]; } set { if (value == null) { throw new ArgumentNullException(String.Format(CultureInfo.InvariantCulture, "Vector3DKeyFrameCollection[{0}]", index)); } WritePreamble(); if (value != _keyFrames[index]) { OnFreezablePropertyChanged(_keyFrames[index], value); _keyFrames[index] = value; Debug.Assert(_keyFrames[index] != null); WritePostscript(); } } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
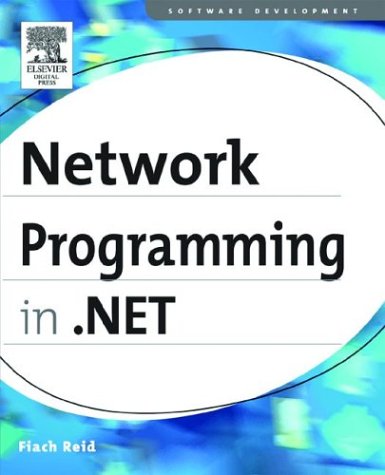
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XsltOutput.cs
- MetadataArtifactLoaderCompositeResource.cs
- MailFileEditor.cs
- StackBuilderSink.cs
- ValidatorUtils.cs
- ErasingStroke.cs
- DetailsViewPagerRow.cs
- ProxyManager.cs
- ConstraintCollection.cs
- UInt32Storage.cs
- WpfWebRequestHelper.cs
- Point3DAnimation.cs
- TextElementCollectionHelper.cs
- HttpModuleAction.cs
- SchemaLookupTable.cs
- ConsumerConnectionPointCollection.cs
- ProjectionAnalyzer.cs
- CharacterHit.cs
- TextMarkerSource.cs
- PictureBox.cs
- Attributes.cs
- OutputCache.cs
- SafeFileHandle.cs
- WsatServiceAddress.cs
- RuntimeEnvironment.cs
- TextRunTypographyProperties.cs
- Variant.cs
- BitmapDownload.cs
- ValidationError.cs
- DropSourceBehavior.cs
- SharedDp.cs
- HttpWebResponse.cs
- ShaderEffect.cs
- HttpEncoderUtility.cs
- _DigestClient.cs
- XmlSchemaIdentityConstraint.cs
- CompilerGlobalScopeAttribute.cs
- ActiveXContainer.cs
- RadioButton.cs
- FixedTextView.cs
- _CacheStreams.cs
- SocketPermission.cs
- _IPv4Address.cs
- COM2PropertyDescriptor.cs
- OutOfMemoryException.cs
- CodeSnippetTypeMember.cs
- EntityModelBuildProvider.cs
- PersonalizationProviderCollection.cs
- FileEnumerator.cs
- RuleSet.cs
- RelationshipType.cs
- ExternalException.cs
- WindowsComboBox.cs
- AttributeUsageAttribute.cs
- WebPartEditVerb.cs
- GridViewPageEventArgs.cs
- DBConcurrencyException.cs
- ConditionCollection.cs
- CustomTypeDescriptor.cs
- TextEffectCollection.cs
- DateTimeOffsetStorage.cs
- WebPartZoneCollection.cs
- FormattedTextSymbols.cs
- OrthographicCamera.cs
- HwndMouseInputProvider.cs
- EdmMember.cs
- SpellCheck.cs
- StaticFileHandler.cs
- BinaryUtilClasses.cs
- CatalogPartCollection.cs
- CultureTable.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- LinearQuaternionKeyFrame.cs
- smtpconnection.cs
- BinaryObjectWriter.cs
- AbstractDataSvcMapFileLoader.cs
- SoapInteropTypes.cs
- DNS.cs
- httpapplicationstate.cs
- xdrvalidator.cs
- AlignmentXValidation.cs
- DummyDataSource.cs
- SrgsRulesCollection.cs
- grammarelement.cs
- PageTheme.cs
- webeventbuffer.cs
- DelegateBodyWriter.cs
- ConfigurationManagerInternalFactory.cs
- _AcceptOverlappedAsyncResult.cs
- SoapReflector.cs
- EntityModelBuildProvider.cs
- Publisher.cs
- ResourceCodeDomSerializer.cs
- FilteredAttributeCollection.cs
- ComponentManagerBroker.cs
- PropertyDescriptor.cs
- TypeConverter.cs
- WindowsAuthenticationModule.cs
- DataReaderContainer.cs
- SmtpCommands.cs