Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Services / Messaging / System / Messaging / MessageQueueCriteria.cs / 1305376 / MessageQueueCriteria.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Messaging { using System.Diagnostics; using System; using System.Messaging.Interop; using Microsoft.Win32; using System.ComponentModel; using System.Security.Permissions; using System.Globalization; //for CultureInfo ////// /// public class MessageQueueCriteria { private DateTime createdAfter; private DateTime createdBefore; private string label; private string machine; private DateTime modifiedAfter; private DateTime modifiedBefore; private Guid category; private CriteriaPropertyFilter filter = new CriteriaPropertyFilter(); private Restrictions restrictions; private Guid machineId; private static DateTime minDate = new DateTime(1970 , 1, 1); private static DateTime maxDate = new DateTime(2038 , 1, 19); ////// This class /// is used to filter MessageQueues when performing a /// query in the network, through MessageQueue.GetPublicQueues method. /// ////// /// Specifies the lower bound of the interval /// that will be used as the queue creation time /// search criteria. /// public DateTime CreatedAfter{ get { if (!this.filter.CreatedAfter) throw new InvalidOperationException(Res.GetString(Res.CriteriaNotDefined)); return this.createdAfter; } set { if (value < MessageQueueCriteria.minDate || value > MessageQueueCriteria.maxDate) throw new ArgumentException(Res.GetString(Res.InvalidDateValue, MessageQueueCriteria.minDate.ToString(CultureInfo.CurrentCulture), MessageQueueCriteria.maxDate.ToString(CultureInfo.CurrentCulture))); this.createdAfter = value; if (this.filter.CreatedBefore && this.createdAfter > this.createdBefore) this.createdBefore = this.createdAfter; this.filter.CreatedAfter = true; } } ////// /// Specifies the upper bound of the interval /// that will be used as the queue creation time /// search criteria. /// public DateTime CreatedBefore{ get { if (!this.filter.CreatedBefore) throw new InvalidOperationException(Res.GetString(Res.CriteriaNotDefined)); return this.createdBefore; } set { if (value < MessageQueueCriteria.minDate || value > MessageQueueCriteria.maxDate) throw new ArgumentException(Res.GetString(Res.InvalidDateValue, MessageQueueCriteria.minDate.ToString(CultureInfo.CurrentCulture), MessageQueueCriteria.maxDate.ToString(CultureInfo.CurrentCulture))); this.createdBefore = value; if (this.filter.CreatedAfter && this.createdAfter > this.createdBefore) this.createdAfter = this.createdBefore; this.filter.CreatedBefore = true; } } internal bool FilterMachine{ get{ return this.filter.MachineName; } } ////// /// Specifies the label that that will be used as /// the criteria to search queues in the network. /// public string Label{ get { if (!this.filter.Label) throw new InvalidOperationException(Res.GetString(Res.CriteriaNotDefined)); return this.label; } set { if (value == null) throw new ArgumentNullException("value"); this.label = value; this.filter.Label = true; } } ////// /// public string MachineName{ get { if (!this.filter.MachineName) throw new InvalidOperationException(Res.GetString(Res.CriteriaNotDefined)); return this.machine; } set { if (!SyntaxCheck.CheckMachineName(value)) throw new ArgumentException(Res.GetString(Res.InvalidProperty, "MachineName", value)); //SECREVIEW: Setting this property shouldn't demmand any permissions, // the machine id will only be used internally. MessageQueuePermission permission = new MessageQueuePermission(PermissionState.Unrestricted); permission.Assert(); try { this.machineId = MessageQueue.GetMachineId(value); } finally { MessageQueuePermission.RevertAssert(); } this.machine = value; this.filter.MachineName = true; } } ////// Specifies the machine name that will be used /// as the criteria to search queues in the network. /// ////// /// Specifies the lower bound of the interval /// that will be used as the queue modified time /// search criteria. /// public DateTime ModifiedAfter{ get { if (!this.filter.ModifiedAfter) throw new InvalidOperationException(Res.GetString(Res.CriteriaNotDefined)); return this.modifiedAfter; } set { if (value < MessageQueueCriteria.minDate || value > MessageQueueCriteria.maxDate) throw new ArgumentException(Res.GetString(Res.InvalidDateValue, MessageQueueCriteria.minDate.ToString(CultureInfo.CurrentCulture), MessageQueueCriteria.maxDate.ToString(CultureInfo.CurrentCulture))); this.modifiedAfter = value; if (this.filter.ModifiedBefore && this.modifiedAfter > this.modifiedBefore) this.modifiedBefore = this.modifiedAfter; this.filter.ModifiedAfter = true; } } ////// /// Specifies the upper bound of the interval /// that will be used as the queue modified time /// search criteria. /// public DateTime ModifiedBefore{ get { if (!this.filter.ModifiedBefore) throw new InvalidOperationException(Res.GetString(Res.CriteriaNotDefined)); return this.modifiedBefore; } set { if (value < MessageQueueCriteria.minDate || value > MessageQueueCriteria.maxDate) throw new ArgumentException(Res.GetString(Res.InvalidDateValue, MessageQueueCriteria.minDate.ToString(CultureInfo.CurrentCulture), MessageQueueCriteria.maxDate.ToString(CultureInfo.CurrentCulture))); this.modifiedBefore = value; if (this.filter.ModifiedAfter && this.modifiedAfter > this.modifiedBefore) this.modifiedAfter = this.modifiedBefore; this.filter.ModifiedBefore = true; } } ////// internal Restrictions.MQRESTRICTION Reference{ get{ int size = 0; if (this.filter.CreatedAfter) ++ size; if (this.filter.CreatedBefore) ++ size; if (this.filter.Label) ++ size; if (this.filter.ModifiedAfter) ++ size; if (this.filter.ModifiedBefore) ++ size; if (this.filter.Category) ++ size; restrictions = new Restrictions(size); if (this.filter.CreatedAfter) restrictions.AddI4(NativeMethods.QUEUE_PROPID_CREATE_TIME, Restrictions.PRGT, ConvertTime(this.createdAfter)); if (this.filter.CreatedBefore) restrictions.AddI4(NativeMethods.QUEUE_PROPID_CREATE_TIME, Restrictions.PRLE, ConvertTime(this.createdBefore)); if (this.filter.Label) restrictions.AddString(NativeMethods.QUEUE_PROPID_LABEL, Restrictions.PREQ, this.label); if (this.filter.ModifiedAfter) restrictions.AddI4(NativeMethods.QUEUE_PROPID_MODIFY_TIME, Restrictions.PRGT, ConvertTime(this.modifiedAfter)); if (this.filter.ModifiedBefore) restrictions.AddI4(NativeMethods.QUEUE_PROPID_MODIFY_TIME, Restrictions.PRLE, ConvertTime(this.modifiedBefore)); if (this.filter.Category) restrictions.AddGuid(NativeMethods.QUEUE_PROPID_TYPE, Restrictions.PREQ, this.category); return this.restrictions.GetRestrictionsRef(); } } /// /// /// Specifies the Category that will be used /// as the criteria to search queues in the network. /// public Guid Category{ get { if (!this.filter.Category) throw new InvalidOperationException(Res.GetString(Res.CriteriaNotDefined)); return this.category; } set { this.category = value; this.filter.Category = true; } } ////// /// Resets all the current instance settings. /// public void ClearAll() { this.filter.ClearAll(); } ////// private int ConvertTime(DateTime time) { time = time.ToUniversalTime(); return (int)(time - MessageQueueCriteria.minDate).TotalSeconds; } /// /// private class CriteriaPropertyFilter{ public bool CreatedAfter; public bool CreatedBefore; public bool Label; public bool MachineName; public bool ModifiedAfter; public bool ModifiedBefore; public bool Category; public void ClearAll() { this.CreatedAfter = false; this.CreatedBefore = false; this.Label = false; this.MachineName = false; this.ModifiedAfter = false; this.ModifiedBefore = false; this.Category = false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
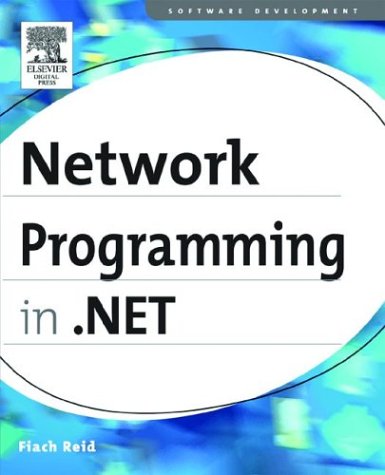
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FontEmbeddingManager.cs
- AsyncPostBackTrigger.cs
- DocumentGridContextMenu.cs
- ThousandthOfEmRealPoints.cs
- Error.cs
- Number.cs
- FileClassifier.cs
- FlagsAttribute.cs
- TrayIconDesigner.cs
- BitmapCodecInfo.cs
- XmlDictionaryWriter.cs
- TemplateComponentConnector.cs
- SqlProfileProvider.cs
- LineSegment.cs
- CaseInsensitiveHashCodeProvider.cs
- QilStrConcatenator.cs
- DataSvcMapFileSerializer.cs
- InputElement.cs
- Expr.cs
- DropSource.cs
- HybridObjectCache.cs
- Binding.cs
- ActivationServices.cs
- KeysConverter.cs
- ObjectComplexPropertyMapping.cs
- ConsumerConnectionPointCollection.cs
- M3DUtil.cs
- SmiEventSink_DeferedProcessing.cs
- NativeMethods.cs
- XPathConvert.cs
- PrintDialog.cs
- ErrorEventArgs.cs
- ConnectionStringEditor.cs
- PrintControllerWithStatusDialog.cs
- DataControlFieldHeaderCell.cs
- codemethodreferenceexpression.cs
- AlgoModule.cs
- InternalResources.cs
- DataRowExtensions.cs
- MasterPageCodeDomTreeGenerator.cs
- WsatServiceCertificate.cs
- AssociatedControlConverter.cs
- ADRoleFactoryConfiguration.cs
- Point3DCollectionConverter.cs
- SourceFileInfo.cs
- SafeNativeMethodsMilCoreApi.cs
- ThrowHelper.cs
- ProgressBarRenderer.cs
- ParseElementCollection.cs
- SqlStream.cs
- HttpCookiesSection.cs
- ToolZone.cs
- CatalogZoneBase.cs
- ObjectDataSourceDisposingEventArgs.cs
- JsonReader.cs
- PackUriHelper.cs
- OrderPreservingPipeliningSpoolingTask.cs
- ShortcutKeysEditor.cs
- BrowserCapabilitiesCodeGenerator.cs
- NameTable.cs
- XmlAttributeHolder.cs
- EncoderNLS.cs
- ToolStripStatusLabel.cs
- SortedList.cs
- WindowsAltTab.cs
- CodeDirectoryCompiler.cs
- FileResponseElement.cs
- ListViewItemSelectionChangedEvent.cs
- SafePEFileHandle.cs
- EntityRecordInfo.cs
- HealthMonitoringSectionHelper.cs
- RevocationPoint.cs
- ColumnWidthChangingEvent.cs
- FixedDSBuilder.cs
- TextServicesHost.cs
- DirectoryNotFoundException.cs
- TypeViewSchema.cs
- EventHandlerList.cs
- RequestCacheEntry.cs
- TransferRequestHandler.cs
- Stackframe.cs
- ToolZone.cs
- StringValidatorAttribute.cs
- HashJoinQueryOperatorEnumerator.cs
- FixedTextContainer.cs
- PageAsyncTaskManager.cs
- CompilerGlobalScopeAttribute.cs
- MetabaseServerConfig.cs
- RequestCacheManager.cs
- TypeElement.cs
- Touch.cs
- SequentialOutput.cs
- EpmSyndicationContentSerializer.cs
- DataGridItemAttachedStorage.cs
- DesignerForm.cs
- EntityDataSource.cs
- DiagnosticTraceSource.cs
- BlurEffect.cs
- XmlTypeMapping.cs
- xml.cs