Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / BuildTasks / Microsoft / Build / Tasks / Windows / FileClassifier.cs / 1 / FileClassifier.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: An MSBuild task that classify the input files to different // categories based on the input item's attributes. // // Spec: http://avalon/app/Compilation/Avalon-MSBUILD%20Targets.doc // // History: // 06/20/03: weibz rewrite and moved over to WCP tree // //--------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Globalization; using System.Diagnostics; using System.Reflection; using System.Resources; using System.Runtime.InteropServices; using Microsoft.Build.Framework; using Microsoft.Build.Utilities; using MS.Utility; using MS.Internal.Tasks; // Since we disable PreSharp warnings in this file, PreSharp warning is unknown to C# compiler. // We first need to disable warnings about unknown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 namespace Microsoft.Build.Tasks.Windows { #region FileClassifier Task class ////// The File Classification task puts all the input baml files, image files into different /// output resource groups, such as Resources for Main assembly and Resources for satellite /// assembly. /// public sealed class FileClassifier : Task { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constrcutor /// public FileClassifier() : base(SR.ResourceManager) { // set default values for some non-required input items _culture = null; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// ITask Execute method /// ///public override bool Execute() { bool ret = false; ArrayList mainEmbeddedList = new ArrayList( ); ArrayList satelliteEmbeddedList = new ArrayList( ); ArrayList clrEmbeddedResourceList = new ArrayList(); ArrayList clrSatelliteEmbeddedResourceList = new ArrayList(); try { TaskHelper.DisplayLogo(Log, SR.Get(SRID.FileClassifierTask)); ret = VerifyTaskInputs(); if (ret != false) { // Do the real work to classify input files. ret = DoFileClassifier(mainEmbeddedList, satelliteEmbeddedList); if (ret == true && CLRResourceFiles != null && CLRResourceFiles.Length > 0) { // Generate the output CLR embedded resource list. ret = CLRResourceFileClassifier(ref clrEmbeddedResourceList, ref clrSatelliteEmbeddedResourceList); } // move the arraylist to the TaskItem array. if (ret == true) { MainEmbeddedFiles = GetTaskItemListFromArrayList(mainEmbeddedList); SatelliteEmbeddedFiles = GetTaskItemListFromArrayList(satelliteEmbeddedList); CLREmbeddedResource = GetTaskItemListFromArrayList(clrEmbeddedResourceList); CLRSatelliteEmbeddedResource = GetTaskItemListFromArrayList(clrSatelliteEmbeddedResourceList); } } } catch (Exception e) { // PreSharp Complaint 6500 - do not handle null-ref or SEH exceptions. if (e is NullReferenceException || e is SEHException) { throw; } else { string message; string errorId; errorId = Log.ExtractMessageCode(e.Message, out message); if (String.IsNullOrEmpty(errorId)) { errorId = UnknownErrorID; message = SR.Get(SRID.UnknownBuildError, message); } Log.LogError(null, errorId, null, null, 0, 0, 0, 0, message, null); } return false; } #pragma warning disable 6500 catch // Non-CLS compliant errors { Log.LogErrorWithCodeFromResources(SRID.NonClsError); return false; } #pragma warning restore 6500 return ret; } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties /// /// SourceFiles: List of Items thatare to be classified /// [Required] public ITaskItem [] SourceFiles { get { return _sourceFiles; } set { _sourceFiles = value; } } ////// Can have values (exe, or dll) /// [Required] public string OutputType { get { return _outputType; } set { _outputType = value; } } ////// Culture of the build. Can be null if the build is non-localizable /// public string Culture { get { return _culture != null ? _culture.ToLower(CultureInfo.InvariantCulture) : null; } set { _culture = value; } } ////// The CLR resource file list. /// In Project file, those files will be define by type CLRResource. /// such as: ///- ///
public ITaskItem[] CLRResourceFiles { get { return _clrResourceFiles; } set { _clrResourceFiles = value; } } /// /// Output Item list for the CLR resources that will be saved in /// the main assembly. /// ///[Output] public ITaskItem[] CLREmbeddedResource { get { if (_clrEmbeddedResource == null) _clrEmbeddedResource = new TaskItem[0]; return _clrEmbeddedResource; } set { _clrEmbeddedResource = value; } } /// /// Output Item list for the CLR resources that will be saved in /// the satellite assembly. /// ///[Output] public ITaskItem[] CLRSatelliteEmbeddedResource { get { if (_clrSatelliteEmbeddedResource == null) _clrSatelliteEmbeddedResource = new TaskItem[0]; return _clrSatelliteEmbeddedResource; } set { _clrSatelliteEmbeddedResource = value; } } /// /// MainEmbeddedFiles /// /// Non-localizable resources which will be embedded into the Main assembly. /// [Output] public ITaskItem [] MainEmbeddedFiles { get { if (_mainEmbeddedFiles == null) _mainEmbeddedFiles = new TaskItem[0]; return _mainEmbeddedFiles; } set { _mainEmbeddedFiles = value; } } ////// SatelliteEmbeddedFiles /// /// Localizable files which are embedded to the Satellite assembly for the /// culture which is set in Culture property.. /// [Output] public ITaskItem [] SatelliteEmbeddedFiles { get { if (_satelliteEmbeddedFiles == null) _satelliteEmbeddedFiles = new TaskItem[0]; return _satelliteEmbeddedFiles; } set { _satelliteEmbeddedFiles = value; } } #endregion Public Properties //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Protected Methods // //----------------------------------------------------- //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods // // Verify all the propety values set from project file. // If any input value is set wrongly, report appropriate build error // and return false. // private bool VerifyTaskInputs() { bool bValidInput = true; // // Verify different property values // // OutputType is marked as Required, it should never be null or emtpy. // otherwise, the task should have been failed before the code goes here. string targetType = OutputType.ToLowerInvariant( ); switch (targetType) { case SharedStrings.Library : case SharedStrings.Module : case SharedStrings.WinExe : case SharedStrings.Exe : break; default : Log.LogErrorWithCodeFromResources(SRID.TargetIsNotSupported, targetType); bValidInput = false; break; } // SourceFiles property is marked as Required. // MSBUILD Engine should have checked the setting for this property // so don't need to recheck here. if (TaskHelper.IsValidCultureName(Culture) == false) { Log.LogErrorWithCodeFromResources(SRID.InvalidCulture, Culture); bValidInput = false; } return bValidInput; } //// Do the real work to classify input files. // // // //private bool DoFileClassifier(ArrayList mainEmbeddedList, ArrayList satelliteEmbeddedList ) { bool ret = true; for (int i = 0; i < SourceFiles.Length; i++) { ITaskItem inputItem = SourceFiles[i]; ITaskItem outputItem = new TaskItem( ); outputItem.ItemSpec = inputItem.ItemSpec; if (IsItemLocalizable(inputItem) == false) { // This file will be embedded into main assembly. mainEmbeddedList.Add(outputItem); } else { // This is localizable item. // Put it in Satellite Embedded OutputItem. satelliteEmbeddedList.Add(outputItem); } // Done for this input item handling. } return ret; } // // Get the ClrEmbeddedResourceList and ClrSatelliteEmebeddedResourceList // from the input CLRResourceFiles. // private bool CLRResourceFileClassifier(ref ArrayList clrEmbeddedResourceList, ref ArrayList clrSatelliteEmbeddedResourceList) { bool ret = true; for (int i = 0; i < CLRResourceFiles.Length; i++) { ITaskItem inputItem = CLRResourceFiles[i]; ITaskItem outputItem = new TaskItem(); outputItem.ItemSpec = inputItem.ItemSpec; if (IsItemLocalizable(inputItem) == true) { clrSatelliteEmbeddedResourceList.Add(outputItem); } else { clrEmbeddedResourceList.Add(outputItem); } } return ret; } // // Check if the item is localizable or not. // // //private bool IsItemLocalizable(ITaskItem fileItem) { bool isLocalizable = false; // if the default culture is not set, by default all // the items are not localizable. if (Culture != null && Culture.Equals("") == false) { string localizableString; // Default culture is set, by default the item is localizable // unless it is set as false in the Localizable attribute. isLocalizable = true; localizableString = fileItem.GetMetadata(SharedStrings.Localizable); if (localizableString != null && String.Compare(localizableString, "false", StringComparison.OrdinalIgnoreCase) ==0 ) { isLocalizable = false; } } return isLocalizable; } // // Return TaskItem list from ArrayList // private ITaskItem[] GetTaskItemListFromArrayList(ArrayList inputList) { ITaskItem[] taskItemList = new TaskItem[0]; if (inputList != null && inputList.Count > 0) { taskItemList = (ITaskItem[])inputList.ToArray(typeof(ITaskItem)); } return taskItemList; } #endregion Private Methods //----------------------------------------------------- // // Private Properties // //----------------------------------------------------- //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields private string _outputType; private string _culture; private ITaskItem [] _sourceFiles; private ITaskItem [] _clrResourceFiles; private ITaskItem [] _mainEmbeddedFiles; private ITaskItem [] _satelliteEmbeddedFiles; private ITaskItem [] _clrEmbeddedResource; private ITaskItem [] _clrSatelliteEmbeddedResource; private const string UnknownErrorID = "FC1000"; #endregion Private Fields } #endregion FileClassifier Task class } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: An MSBuild task that classify the input files to different // categories based on the input item's attributes. // // Spec: http://avalon/app/Compilation/Avalon-MSBUILD%20Targets.doc // // History: // 06/20/03: weibz rewrite and moved over to WCP tree // //--------------------------------------------------------------------------- using System; using System.IO; using System.Collections; using System.Globalization; using System.Diagnostics; using System.Reflection; using System.Resources; using System.Runtime.InteropServices; using Microsoft.Build.Framework; using Microsoft.Build.Utilities; using MS.Utility; using MS.Internal.Tasks; // Since we disable PreSharp warnings in this file, PreSharp warning is unknown to C# compiler. // We first need to disable warnings about unknown message numbers and unknown pragmas. #pragma warning disable 1634, 1691 namespace Microsoft.Build.Tasks.Windows { #region FileClassifier Task class ////// The File Classification task puts all the input baml files, image files into different /// output resource groups, such as Resources for Main assembly and Resources for satellite /// assembly. /// public sealed class FileClassifier : Task { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Constrcutor /// public FileClassifier() : base(SR.ResourceManager) { // set default values for some non-required input items _culture = null; } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// ITask Execute method /// ///public override bool Execute() { bool ret = false; ArrayList mainEmbeddedList = new ArrayList( ); ArrayList satelliteEmbeddedList = new ArrayList( ); ArrayList clrEmbeddedResourceList = new ArrayList(); ArrayList clrSatelliteEmbeddedResourceList = new ArrayList(); try { TaskHelper.DisplayLogo(Log, SR.Get(SRID.FileClassifierTask)); ret = VerifyTaskInputs(); if (ret != false) { // Do the real work to classify input files. ret = DoFileClassifier(mainEmbeddedList, satelliteEmbeddedList); if (ret == true && CLRResourceFiles != null && CLRResourceFiles.Length > 0) { // Generate the output CLR embedded resource list. ret = CLRResourceFileClassifier(ref clrEmbeddedResourceList, ref clrSatelliteEmbeddedResourceList); } // move the arraylist to the TaskItem array. if (ret == true) { MainEmbeddedFiles = GetTaskItemListFromArrayList(mainEmbeddedList); SatelliteEmbeddedFiles = GetTaskItemListFromArrayList(satelliteEmbeddedList); CLREmbeddedResource = GetTaskItemListFromArrayList(clrEmbeddedResourceList); CLRSatelliteEmbeddedResource = GetTaskItemListFromArrayList(clrSatelliteEmbeddedResourceList); } } } catch (Exception e) { // PreSharp Complaint 6500 - do not handle null-ref or SEH exceptions. if (e is NullReferenceException || e is SEHException) { throw; } else { string message; string errorId; errorId = Log.ExtractMessageCode(e.Message, out message); if (String.IsNullOrEmpty(errorId)) { errorId = UnknownErrorID; message = SR.Get(SRID.UnknownBuildError, message); } Log.LogError(null, errorId, null, null, 0, 0, 0, 0, message, null); } return false; } #pragma warning disable 6500 catch // Non-CLS compliant errors { Log.LogErrorWithCodeFromResources(SRID.NonClsError); return false; } #pragma warning restore 6500 return ret; } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties /// /// SourceFiles: List of Items thatare to be classified /// [Required] public ITaskItem [] SourceFiles { get { return _sourceFiles; } set { _sourceFiles = value; } } ////// Can have values (exe, or dll) /// [Required] public string OutputType { get { return _outputType; } set { _outputType = value; } } ////// Culture of the build. Can be null if the build is non-localizable /// public string Culture { get { return _culture != null ? _culture.ToLower(CultureInfo.InvariantCulture) : null; } set { _culture = value; } } ////// The CLR resource file list. /// In Project file, those files will be define by type CLRResource. /// such as: ///- ///
public ITaskItem[] CLRResourceFiles { get { return _clrResourceFiles; } set { _clrResourceFiles = value; } } /// /// Output Item list for the CLR resources that will be saved in /// the main assembly. /// ///[Output] public ITaskItem[] CLREmbeddedResource { get { if (_clrEmbeddedResource == null) _clrEmbeddedResource = new TaskItem[0]; return _clrEmbeddedResource; } set { _clrEmbeddedResource = value; } } /// /// Output Item list for the CLR resources that will be saved in /// the satellite assembly. /// ///[Output] public ITaskItem[] CLRSatelliteEmbeddedResource { get { if (_clrSatelliteEmbeddedResource == null) _clrSatelliteEmbeddedResource = new TaskItem[0]; return _clrSatelliteEmbeddedResource; } set { _clrSatelliteEmbeddedResource = value; } } /// /// MainEmbeddedFiles /// /// Non-localizable resources which will be embedded into the Main assembly. /// [Output] public ITaskItem [] MainEmbeddedFiles { get { if (_mainEmbeddedFiles == null) _mainEmbeddedFiles = new TaskItem[0]; return _mainEmbeddedFiles; } set { _mainEmbeddedFiles = value; } } ////// SatelliteEmbeddedFiles /// /// Localizable files which are embedded to the Satellite assembly for the /// culture which is set in Culture property.. /// [Output] public ITaskItem [] SatelliteEmbeddedFiles { get { if (_satelliteEmbeddedFiles == null) _satelliteEmbeddedFiles = new TaskItem[0]; return _satelliteEmbeddedFiles; } set { _satelliteEmbeddedFiles = value; } } #endregion Public Properties //----------------------------------------------------- // // Public Events // //------------------------------------------------------ //----------------------------------------------------- // // Protected Methods // //----------------------------------------------------- //----------------------------------------------------- // // Internal Methods // //------------------------------------------------------ #region Internal Methods #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ //------------------------------------------------------ // // Internal Events // //----------------------------------------------------- //------------------------------------------------------ // // Private Methods // //----------------------------------------------------- #region Private Methods // // Verify all the propety values set from project file. // If any input value is set wrongly, report appropriate build error // and return false. // private bool VerifyTaskInputs() { bool bValidInput = true; // // Verify different property values // // OutputType is marked as Required, it should never be null or emtpy. // otherwise, the task should have been failed before the code goes here. string targetType = OutputType.ToLowerInvariant( ); switch (targetType) { case SharedStrings.Library : case SharedStrings.Module : case SharedStrings.WinExe : case SharedStrings.Exe : break; default : Log.LogErrorWithCodeFromResources(SRID.TargetIsNotSupported, targetType); bValidInput = false; break; } // SourceFiles property is marked as Required. // MSBUILD Engine should have checked the setting for this property // so don't need to recheck here. if (TaskHelper.IsValidCultureName(Culture) == false) { Log.LogErrorWithCodeFromResources(SRID.InvalidCulture, Culture); bValidInput = false; } return bValidInput; } //// Do the real work to classify input files. // // // //private bool DoFileClassifier(ArrayList mainEmbeddedList, ArrayList satelliteEmbeddedList ) { bool ret = true; for (int i = 0; i < SourceFiles.Length; i++) { ITaskItem inputItem = SourceFiles[i]; ITaskItem outputItem = new TaskItem( ); outputItem.ItemSpec = inputItem.ItemSpec; if (IsItemLocalizable(inputItem) == false) { // This file will be embedded into main assembly. mainEmbeddedList.Add(outputItem); } else { // This is localizable item. // Put it in Satellite Embedded OutputItem. satelliteEmbeddedList.Add(outputItem); } // Done for this input item handling. } return ret; } // // Get the ClrEmbeddedResourceList and ClrSatelliteEmebeddedResourceList // from the input CLRResourceFiles. // private bool CLRResourceFileClassifier(ref ArrayList clrEmbeddedResourceList, ref ArrayList clrSatelliteEmbeddedResourceList) { bool ret = true; for (int i = 0; i < CLRResourceFiles.Length; i++) { ITaskItem inputItem = CLRResourceFiles[i]; ITaskItem outputItem = new TaskItem(); outputItem.ItemSpec = inputItem.ItemSpec; if (IsItemLocalizable(inputItem) == true) { clrSatelliteEmbeddedResourceList.Add(outputItem); } else { clrEmbeddedResourceList.Add(outputItem); } } return ret; } // // Check if the item is localizable or not. // // //private bool IsItemLocalizable(ITaskItem fileItem) { bool isLocalizable = false; // if the default culture is not set, by default all // the items are not localizable. if (Culture != null && Culture.Equals("") == false) { string localizableString; // Default culture is set, by default the item is localizable // unless it is set as false in the Localizable attribute. isLocalizable = true; localizableString = fileItem.GetMetadata(SharedStrings.Localizable); if (localizableString != null && String.Compare(localizableString, "false", StringComparison.OrdinalIgnoreCase) ==0 ) { isLocalizable = false; } } return isLocalizable; } // // Return TaskItem list from ArrayList // private ITaskItem[] GetTaskItemListFromArrayList(ArrayList inputList) { ITaskItem[] taskItemList = new TaskItem[0]; if (inputList != null && inputList.Count > 0) { taskItemList = (ITaskItem[])inputList.ToArray(typeof(ITaskItem)); } return taskItemList; } #endregion Private Methods //----------------------------------------------------- // // Private Properties // //----------------------------------------------------- //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields private string _outputType; private string _culture; private ITaskItem [] _sourceFiles; private ITaskItem [] _clrResourceFiles; private ITaskItem [] _mainEmbeddedFiles; private ITaskItem [] _satelliteEmbeddedFiles; private ITaskItem [] _clrEmbeddedResource; private ITaskItem [] _clrSatelliteEmbeddedResource; private const string UnknownErrorID = "FC1000"; #endregion Private Fields } #endregion FileClassifier Task class } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
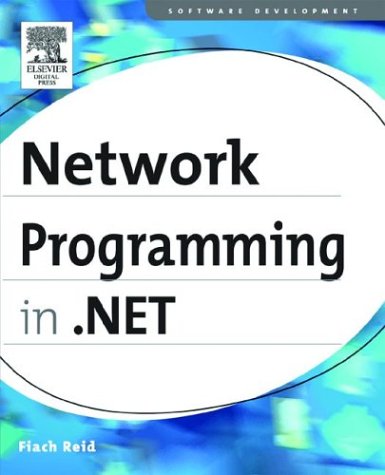
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlBinaryReader.cs
- FixedSOMTable.cs
- BitmapEffectCollection.cs
- SqlUserDefinedAggregateAttribute.cs
- FrameworkReadOnlyPropertyMetadata.cs
- MsmqIntegrationValidationBehavior.cs
- SpecialFolderEnumConverter.cs
- StoreContentChangedEventArgs.cs
- XsdBuilder.cs
- SQLSingle.cs
- ComponentGlyph.cs
- CharUnicodeInfo.cs
- WorkflowMarkupElementEventArgs.cs
- errorpatternmatcher.cs
- ResizeGrip.cs
- ToolStripDropDownItemDesigner.cs
- DocumentAutomationPeer.cs
- InstanceKeyCompleteException.cs
- FormsAuthenticationConfiguration.cs
- DrawingCollection.cs
- RestrictedTransactionalPackage.cs
- EntityContainerAssociationSet.cs
- TextBoxLine.cs
- ButtonChrome.cs
- SortDescription.cs
- GroupQuery.cs
- ScriptingJsonSerializationSection.cs
- EditableRegion.cs
- OdbcDataAdapter.cs
- UnsignedPublishLicense.cs
- NegotiateStream.cs
- HttpPostedFile.cs
- RuntimeEnvironment.cs
- FindCriteria.cs
- PixelFormats.cs
- DesignerVerb.cs
- KeyInterop.cs
- TreeView.cs
- XmlSerializationReader.cs
- GrabHandleGlyph.cs
- OracleParameterBinding.cs
- WebPartUtil.cs
- IconBitmapDecoder.cs
- EntityTransaction.cs
- ApplicationDirectoryMembershipCondition.cs
- ScriptingProfileServiceSection.cs
- CellQuery.cs
- XmlReturnWriter.cs
- Constraint.cs
- HMACSHA512.cs
- HierarchicalDataBoundControlAdapter.cs
- Crc32.cs
- XmlCompatibilityReader.cs
- ShaderEffect.cs
- TypeName.cs
- XsdCachingReader.cs
- LinkLabel.cs
- XmlWellformedWriter.cs
- VScrollProperties.cs
- GPStream.cs
- XPathScanner.cs
- HebrewCalendar.cs
- ConfigurationStrings.cs
- FormViewModeEventArgs.cs
- RegexInterpreter.cs
- SafeNativeMethods.cs
- VirtualDirectoryMapping.cs
- ReliableMessagingVersionConverter.cs
- AppDomainProtocolHandler.cs
- ProjectionCamera.cs
- MergeFailedEvent.cs
- RenderingEventArgs.cs
- SingleAnimationBase.cs
- NativeMethodsOther.cs
- ToolboxComponentsCreatingEventArgs.cs
- EntitySqlException.cs
- UnsafeNativeMethods.cs
- Propagator.cs
- WebEventCodes.cs
- SchemaContext.cs
- XmlSignificantWhitespace.cs
- SmtpFailedRecipientsException.cs
- TablePattern.cs
- UriGenerator.cs
- VariantWrapper.cs
- XmlSchemaAll.cs
- LineProperties.cs
- DBParameter.cs
- ZipIOFileItemStream.cs
- TextRange.cs
- SimpleWebHandlerParser.cs
- ContentPresenter.cs
- AccessViolationException.cs
- CalendarButton.cs
- SerializationHelper.cs
- BitmapScalingModeValidation.cs
- AmbientLight.cs
- SystemPens.cs
- QueuePathDialog.cs
- SplineKeyFrames.cs