Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / TrustUi / MS / Internal / documents / Application / RestrictedTransactionalPackage.cs / 1 / RestrictedTransactionalPackage.cs
//------------------------------------------------------------------------------ //// Copyright (C) Microsoft Corporation. All rights reserved. // //// This class wraps TransactionalPackage, ensuring that only parts with // approved content types can be written. // // // History: // 04/12/2006: [....]: Created as a wrapper for TransactionalPackage to // limit which content types of parts can be written. //----------------------------------------------------------------------------- using System; using System.IO; using System.IO.Packaging; using System.Security; using System.Security.Permissions; using System.Windows.TrustUI; using MS.Internal; namespace MS.Internal.Documents.Application { ////// This class wraps TransactionalPackage, ensuring that only approved /// part content types can be written. /// internal sealed class RestrictedTransactionalPackage : TransactionalPackage { #region Constructors //------------------------------------------------------------------------- // Constructors //------------------------------------------------------------------------- ////// Requires an existing open Package; and returns a package which will /// capture changes with out applying them to the original. /// See the class description for details. /// ////// /// Package package = new RestrictedTransactionalPackage( /// Package.Open(source, FileMode.Open, FileAccess.Read)); /// /// An open package. ////// Critical: /// - Calls TransactionalPackage(Stream) which is critical. /// NotSafe: /// - only our caller knows where the stream came from, it must be from /// the user specified location /// [SecurityCritical] internal RestrictedTransactionalPackage(Stream original) : base(original) { } #endregion Constructors ////// /// /// Critical: /// 1) Accesses the value of the TempPackage, which is SecurityCriticalDataForSet. /// 2) Calls TransactionalPackage.MergeChanges which is SecurityCritical. /// NotSafe: /// - only the caller participating in constructing the stream can validate /// that this is an XpsDocument stream /// [SecurityCritical] internal override void MergeChanges(Stream target) { if (target == null) { throw new ArgumentNullException("target"); } if (TempPackage.Value != null) { foreach (PackagePart part in TempPackage.Value.GetParts()) { // Ensure that all parts being modified are permitted. if ((part != null) && (!IsValidContentType(part.ContentType))) { throw new NotSupportedException(SR.Get(SRID.PackagePartTypeNotWritable)); } } base.MergeChanges(target); } } ////// Creates a new PackagePart. /// ////// When creating a new PackagePart we must: /// a) ensure the part does not exist in package /// b) ensure there is a writable package /// c) create a temp part /// d) update active part reference to the temp part /// /// What if a PackagePart with the same Uri already exists? /// Package.CreatePart checks for this. /// /// Do we need to worry about updating relationships and other parts? /// Relationships are a part and are thus intrinsically handled. /// /// Uri for the part to create. /// Content type string. /// Compression options. ///A new PackagePart. protected override PackagePart CreatePartCore( Uri partUri, string contentType, CompressionOption compressionOption) { // Ensure that modifying this contentType is permitted. if (!IsValidContentType(contentType)) { throw new ArgumentException(SR.Get(SRID.PackagePartTypeNotWritable), "contentType"); } return base.CreatePartCore(partUri, contentType, compressionOption); } ////// Verifies that parts of the the given contentType are allowed to be modified. /// /// The content type of the part being modified. ///True if modification is allowed, false otherwise. /// Critical: /// 1) This code makes the actual security decision as to whether a specific /// content type can be written. /// TreatAsSafe: /// 1) The list of content types is a hardcoded constant list of strings that /// is only maintained here. The only data being used is the string content /// type to check. [SecurityCritical, SecurityTreatAsSafe] private bool IsValidContentType(string contentType) { // Check that the contentType is a valid string, and of one of the approved // contentTypes. // // The approved content types come from the Package-wide content types list // in the XPS Specification and Reference Guide. // Internally available at [....]/ // Externally available at http://www.microsoft.com/xps/ return ((!string.IsNullOrEmpty(contentType)) && ((contentType.Equals( @"application/xml", StringComparison.OrdinalIgnoreCase)) || // Core Properties Part (contentType.Equals( @"application/vnd.openxmlformats-package.core-properties+xml", StringComparison.OrdinalIgnoreCase)) || // Digital Signature Certificate Part (contentType.Equals( @"application/vnd.openxmlformats-package.digital-signature-certificate", StringComparison.OrdinalIgnoreCase)) || // Digital Signature Origin Part (contentType.Equals( @"application/vnd.openxmlformats-package.digital-signature-origin", StringComparison.OrdinalIgnoreCase)) || // Digital Signature XML Signature Part (contentType.Equals( @"application/vnd.openxmlformats-package.digital-signature-xmlsignature+xml", StringComparison.OrdinalIgnoreCase)) || // Relationships Part (contentType.Equals( @"application/vnd.openxmlformats-package.relationships+xml", StringComparison.OrdinalIgnoreCase)) )); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
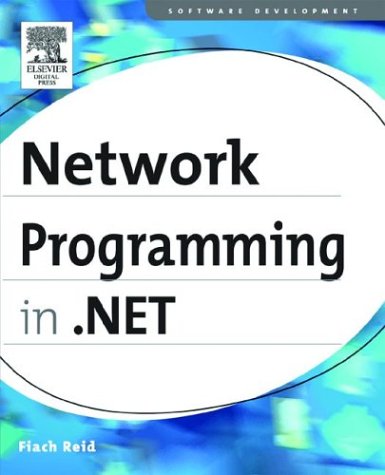
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XamlTemplateSerializer.cs
- GetRecipientRequest.cs
- XmlElementCollection.cs
- AdRotator.cs
- MetadataSource.cs
- Cursors.cs
- GradientStopCollection.cs
- PageAsyncTask.cs
- Assembly.cs
- CheckBox.cs
- CompilationUnit.cs
- XmlArrayAttribute.cs
- InstanceData.cs
- RangeBaseAutomationPeer.cs
- LogicalCallContext.cs
- HitTestResult.cs
- LogWriteRestartAreaState.cs
- ControlUtil.cs
- SafeHandles.cs
- SqlTriggerAttribute.cs
- ExeContext.cs
- HtmlInputCheckBox.cs
- KeyFrames.cs
- DataRecordInternal.cs
- ScaleTransform.cs
- IdentifierService.cs
- X509CertificateCollection.cs
- DataPointer.cs
- Hash.cs
- WebPartVerb.cs
- WebPartMenuStyle.cs
- DelegateBodyWriter.cs
- XamlValidatingReader.cs
- TdsEnums.cs
- TypeRestriction.cs
- Win32SafeHandles.cs
- coordinatorfactory.cs
- ToolStripSettings.cs
- login.cs
- ScrollableControl.cs
- GPRECT.cs
- ToolStripRendererSwitcher.cs
- PropertyDescriptorComparer.cs
- WeakReadOnlyCollection.cs
- DebuggerAttributes.cs
- TextRangeSerialization.cs
- DesignerDeviceConfig.cs
- Cloud.cs
- ImageAutomationPeer.cs
- KnownTypeHelper.cs
- HostedTransportConfigurationBase.cs
- FloatUtil.cs
- PlatformNotSupportedException.cs
- ResourceSet.cs
- TypeConverterAttribute.cs
- Byte.cs
- GeneralTransformCollection.cs
- DictionaryItemsCollection.cs
- QuaternionKeyFrameCollection.cs
- NetworkInformationPermission.cs
- HttpCapabilitiesSectionHandler.cs
- CodeExpressionCollection.cs
- FixedSOMTable.cs
- ViewValidator.cs
- DesignerTransactionCloseEvent.cs
- AttributeCollection.cs
- entityreference_tresulttype.cs
- SplitterCancelEvent.cs
- ExternalCalls.cs
- GeometryGroup.cs
- HtmlInputFile.cs
- Listener.cs
- ClientRuntime.cs
- Keyboard.cs
- ColorMatrix.cs
- HwndMouseInputProvider.cs
- BamlLocalizableResourceKey.cs
- TabPage.cs
- PreApplicationStartMethodAttribute.cs
- HtmlTextViewAdapter.cs
- QilName.cs
- ObjectItemLoadingSessionData.cs
- FileRecordSequenceCompletedAsyncResult.cs
- HttpHeaderCollection.cs
- DrawingAttributeSerializer.cs
- ElementMarkupObject.cs
- PropertyChangedEventArgs.cs
- ZipIOBlockManager.cs
- DataKey.cs
- ImplicitInputBrush.cs
- LinqDataSourceInsertEventArgs.cs
- WebZone.cs
- SearchForVirtualItemEventArgs.cs
- MULTI_QI.cs
- ForeignKeyConstraint.cs
- PageCatalogPart.cs
- SizeIndependentAnimationStorage.cs
- ConvertEvent.cs
- IDispatchConstantAttribute.cs
- EpmContentDeSerializerBase.cs