Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / Utils / Boolean / IdentifierService.cs / 1599186 / IdentifierService.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Collections.ObjectModel; using System.Globalization; using System.Linq; using System.Runtime.CompilerServices; namespace System.Data.Common.Utils.Boolean { ////// Services related to different identifier types for Boolean expressions. /// internal abstract class IdentifierService{ #region Static members internal static readonly IdentifierService Instance = GetIdentifierService(); [MethodImpl(MethodImplOptions.NoInlining | MethodImplOptions.NoOptimization)] private static IdentifierService GetIdentifierService() { Type identifierType = typeof(T_Identifier); if (identifierType.IsGenericType && identifierType.GetGenericTypeDefinition() == typeof(DomainConstraint<,>)) { // initialize a domain constraint literal service Type[] genericArguments = identifierType.GetGenericArguments(); Type variableType = genericArguments[0]; Type elementType = genericArguments[1]; return (IdentifierService )Activator.CreateInstance( typeof(DomainConstraintIdentifierService<,>).MakeGenericType(identifierType, variableType, elementType)); } else { // initialize a generic literal service for all other identifier types return new GenericIdentifierService(); } } #endregion #region Constructors private IdentifierService() { } #endregion #region Service methods /// /// Returns negation of the given literal. /// internal abstract LiteralNegateLiteral(Literal literal); /// /// Creates a new conversion context. /// internal abstract ConversionContextCreateConversionContext(); /// /// Performs local simplification appropriate to the current identifier. /// internal abstract BoolExprLocalSimplify(BoolExpr expression); #endregion private class GenericIdentifierService : IdentifierService { internal override Literal NegateLiteral(Literal literal) { // just invert the sign return new Literal (literal.Term, !literal.IsTermPositive); } internal override ConversionContext CreateConversionContext() { return new GenericConversionContext (); } internal override BoolExpr LocalSimplify(BoolExpr expression) { return expression.Accept(Simplifier .Instance); } } private class DomainConstraintIdentifierService : IdentifierService > { internal override Literal > NegateLiteral(Literal > literal) { // negate the literal by inverting the range, rather than changing the sign // of the literal TermExpr > term = new TermExpr >( literal.Term.Identifier.InvertDomainConstraint()); return new Literal >(term, literal.IsTermPositive); } internal override ConversionContext > CreateConversionContext() { return new DomainConstraintConversionContext (); } internal override BoolExpr > LocalSimplify(BoolExpr > expression) { expression = NegationPusher.EliminateNot (expression); return expression.Accept(Simplifier >.Instance); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Diagnostics; using System.Collections.ObjectModel; using System.Globalization; using System.Linq; using System.Runtime.CompilerServices; namespace System.Data.Common.Utils.Boolean { ////// Services related to different identifier types for Boolean expressions. /// internal abstract class IdentifierService{ #region Static members internal static readonly IdentifierService Instance = GetIdentifierService(); [MethodImpl(MethodImplOptions.NoInlining | MethodImplOptions.NoOptimization)] private static IdentifierService GetIdentifierService() { Type identifierType = typeof(T_Identifier); if (identifierType.IsGenericType && identifierType.GetGenericTypeDefinition() == typeof(DomainConstraint<,>)) { // initialize a domain constraint literal service Type[] genericArguments = identifierType.GetGenericArguments(); Type variableType = genericArguments[0]; Type elementType = genericArguments[1]; return (IdentifierService )Activator.CreateInstance( typeof(DomainConstraintIdentifierService<,>).MakeGenericType(identifierType, variableType, elementType)); } else { // initialize a generic literal service for all other identifier types return new GenericIdentifierService(); } } #endregion #region Constructors private IdentifierService() { } #endregion #region Service methods /// /// Returns negation of the given literal. /// internal abstract LiteralNegateLiteral(Literal literal); /// /// Creates a new conversion context. /// internal abstract ConversionContextCreateConversionContext(); /// /// Performs local simplification appropriate to the current identifier. /// internal abstract BoolExprLocalSimplify(BoolExpr expression); #endregion private class GenericIdentifierService : IdentifierService { internal override Literal NegateLiteral(Literal literal) { // just invert the sign return new Literal (literal.Term, !literal.IsTermPositive); } internal override ConversionContext CreateConversionContext() { return new GenericConversionContext (); } internal override BoolExpr LocalSimplify(BoolExpr expression) { return expression.Accept(Simplifier .Instance); } } private class DomainConstraintIdentifierService : IdentifierService > { internal override Literal > NegateLiteral(Literal > literal) { // negate the literal by inverting the range, rather than changing the sign // of the literal TermExpr > term = new TermExpr >( literal.Term.Identifier.InvertDomainConstraint()); return new Literal >(term, literal.IsTermPositive); } internal override ConversionContext > CreateConversionContext() { return new DomainConstraintConversionContext (); } internal override BoolExpr > LocalSimplify(BoolExpr > expression) { expression = NegationPusher.EliminateNot (expression); return expression.Accept(Simplifier >.Instance); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
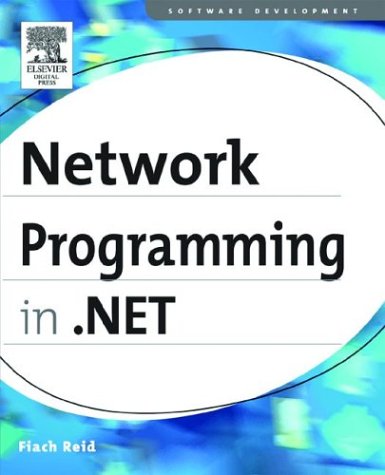
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RelationshipFixer.cs
- XmlSchemaSubstitutionGroup.cs
- FormsAuthenticationModule.cs
- XPathParser.cs
- ThicknessAnimationBase.cs
- TraceContextEventArgs.cs
- RenderDataDrawingContext.cs
- RandomNumberGenerator.cs
- UnSafeCharBuffer.cs
- ButtonBaseAdapter.cs
- TextElement.cs
- UpDownBase.cs
- DataGridViewRowErrorTextNeededEventArgs.cs
- selecteditemcollection.cs
- CursorConverter.cs
- BaseDataBoundControl.cs
- Point3DCollection.cs
- OdbcConnectionString.cs
- CalendarTable.cs
- FileLevelControlBuilderAttribute.cs
- SQLCharsStorage.cs
- Focus.cs
- SingleTagSectionHandler.cs
- ApplicationException.cs
- InfoCardTrace.cs
- invalidudtexception.cs
- QueryExecutionOption.cs
- __ComObject.cs
- FixedSchema.cs
- Repeater.cs
- SymbolMethod.cs
- CreateUserErrorEventArgs.cs
- WriteFileContext.cs
- RightsManagementEncryptionTransform.cs
- PeerNodeTraceRecord.cs
- DataBindingCollection.cs
- Misc.cs
- InternalConfigEventArgs.cs
- CalendarKeyboardHelper.cs
- ProcessInputEventArgs.cs
- DataGridItemCollection.cs
- PathData.cs
- ThemeDictionaryExtension.cs
- PeerObject.cs
- AssemblyName.cs
- PerfService.cs
- InputElement.cs
- AnimationClock.cs
- ReadOnlyDataSource.cs
- Decimal.cs
- DataServiceEntityAttribute.cs
- InstanceDataCollection.cs
- RequestedSignatureDialog.cs
- ResourceDisplayNameAttribute.cs
- ErrorWrapper.cs
- SqlUserDefinedTypeAttribute.cs
- XPathParser.cs
- DbConnectionOptions.cs
- CodeCompileUnit.cs
- WebExceptionStatus.cs
- CustomErrorsSectionWrapper.cs
- DashStyle.cs
- RepeaterDataBoundAdapter.cs
- SqlServer2KCompatibilityCheck.cs
- StorageScalarPropertyMapping.cs
- FileAuthorizationModule.cs
- DataRowChangeEvent.cs
- IPGlobalProperties.cs
- TextDecoration.cs
- Contracts.cs
- TreeViewDataItemAutomationPeer.cs
- DataDocumentXPathNavigator.cs
- Socket.cs
- PrivilegedConfigurationManager.cs
- RuntimeConfig.cs
- securitycriticaldataClass.cs
- TreeNodeBinding.cs
- CompositionTarget.cs
- BitmapFrameEncode.cs
- ElementsClipboardData.cs
- DetailsViewDesigner.cs
- XmlTextAttribute.cs
- BindingsCollection.cs
- BoundColumn.cs
- File.cs
- StringDictionaryWithComparer.cs
- SHA384Managed.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- NullableConverter.cs
- ZoneMembershipCondition.cs
- XmlException.cs
- XmlDocument.cs
- FilteredXmlReader.cs
- Component.cs
- DeclarationUpdate.cs
- OleDbError.cs
- EngineSite.cs
- DescendantOverDescendantQuery.cs
- Path.cs
- WebBrowserContainer.cs