Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / fx / src / Data / System / Data / Sql / SqlUserDefinedTypeAttribute.cs / 1 / SqlUserDefinedTypeAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All Rights Reserved. // Information Contained Herein is Proprietary and Confidential. // //[....] //[....] //daltudov //[....] //beysims //[....] //vadimt //venkar //[....] //----------------------------------------------------------------------------- namespace Microsoft.SqlServer.Server { using System; using System.Data.Common; public enum Format { //: byte Unknown = 0, Native = 1, UserDefined = 2, #if WINFSFunctionality // Only applies to WinFS Structured = 4 #endif } // This custom attribute indicates that the given type is // a SqlServer udt. The properties on the attribute reflect the // physical attributes that will be used when the type is registered // with SqlServer. [AttributeUsage(AttributeTargets.Class | AttributeTargets.Struct, AllowMultiple=false, Inherited=true)] public sealed class SqlUserDefinedTypeAttribute: Attribute { private int m_MaxByteSize; private bool m_IsFixedLength; private bool m_IsByteOrdered; private Format m_format; private string m_fName; // The maximum value for the maxbytesize field, in bytes. internal const int YukonMaxByteSizeValue = 8000; private String m_ValidationMethodName = null; // A required attribute on all udts, used to indicate that the // given type is a udt, and its storage format. public SqlUserDefinedTypeAttribute(Format format) { switch(format) { case Format.Unknown: throw ADP.NotSupportedUserDefinedTypeSerializationFormat((Microsoft.SqlServer.Server.Format)format, "format"); case Format.Native: case Format.UserDefined: #if WINFSFunctionality case Format.Structured: #endif this.m_format = format; break; default: throw ADP.InvalidUserDefinedTypeSerializationFormat((Microsoft.SqlServer.Server.Format)format); } } // The maximum size of this instance, in bytes. Does not have to be // specified for Native serialization. The maximum value // for this property is specified by MaxByteSizeValue. public int MaxByteSize { get { return this.m_MaxByteSize; } set { if (value < -1) { throw ADP.ArgumentOutOfRange("MaxByteSize"); } this.m_MaxByteSize = value; } } // Are all instances of this udt the same size on disk? public bool IsFixedLength { get { return this.m_IsFixedLength; } set { this.m_IsFixedLength = value; } } // Is this type byte ordered, i.e. is the on disk representation // consistent with the ordering semantics for this type? // If true, the binary representation of the type will be used // in comparison by SqlServer. This property enables indexing on the // udt and faster comparisons. public bool IsByteOrdered { get { return this.m_IsByteOrdered; } set { this.m_IsByteOrdered = value; } } // The on-disk format for this type. public Format Format { get { return this.m_format; } } // An Optional method used to validate this UDT // Signature: bool <ValidationMethodName>(); public String ValidationMethodName { get { return this.m_ValidationMethodName; } set { this.m_ValidationMethodName = value; } } public string Name { get { return m_fName; } set { m_fName = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
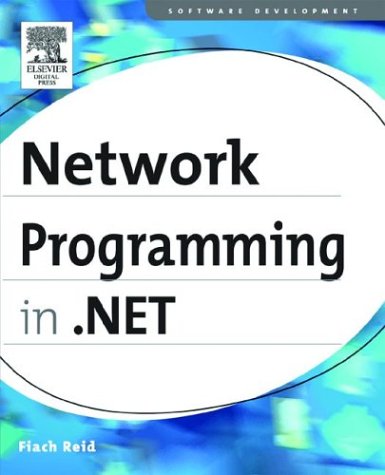
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- clipboard.cs
- TraceRecord.cs
- BigIntegerStorage.cs
- ToolStripPanelRow.cs
- ImageList.cs
- FacetChecker.cs
- ShimAsPublicXamlType.cs
- CodeSnippetExpression.cs
- ListBindableAttribute.cs
- TemplateBamlTreeBuilder.cs
- HandlerFactoryWrapper.cs
- WebServiceErrorEvent.cs
- updatecommandorderer.cs
- PageCache.cs
- QuaternionValueSerializer.cs
- RemoteCryptoSignHashRequest.cs
- DesignerOptionService.cs
- StringComparer.cs
- XmlAnyAttributeAttribute.cs
- StylusOverProperty.cs
- Repeater.cs
- TreeNodeBinding.cs
- TextBoxAutomationPeer.cs
- FontNameEditor.cs
- CharacterShapingProperties.cs
- CachedPathData.cs
- XmlDocumentFragment.cs
- ResourceDisplayNameAttribute.cs
- _SSPIWrapper.cs
- MasterPageParser.cs
- ConstNode.cs
- SimpleTypeResolver.cs
- sqlnorm.cs
- ZipIOZip64EndOfCentralDirectoryLocatorBlock.cs
- EntityDescriptor.cs
- TreeView.cs
- IPCCacheManager.cs
- InstanceLockQueryResult.cs
- RequiredFieldValidator.cs
- StorageConditionPropertyMapping.cs
- CodeBlockBuilder.cs
- DataGridViewTextBoxEditingControl.cs
- XmlSchemaAttributeGroupRef.cs
- MatrixUtil.cs
- XmlSchemaExternal.cs
- DbgUtil.cs
- Inflater.cs
- XamlStackWriter.cs
- ControlEvent.cs
- DbBuffer.cs
- AttributeEmitter.cs
- Rfc2898DeriveBytes.cs
- AddInToken.cs
- RectValueSerializer.cs
- SafeRsaProviderHandle.cs
- TriggerBase.cs
- RadialGradientBrush.cs
- CheckBox.cs
- ObjectDataSourceFilteringEventArgs.cs
- SQLRoleProvider.cs
- EntitySqlQueryCacheKey.cs
- TypeTypeConverter.cs
- TreeNodeConverter.cs
- TextSerializer.cs
- WinFormsComponentEditor.cs
- WinFormsComponentEditor.cs
- WindowsScrollBarBits.cs
- AutomationProperty.cs
- RectAnimation.cs
- SortedSetDebugView.cs
- Drawing.cs
- XDeferredAxisSource.cs
- FunctionNode.cs
- PerfCounterSection.cs
- Figure.cs
- ValidationResult.cs
- WebServiceErrorEvent.cs
- HttpHandlersSection.cs
- IItemProperties.cs
- StorageAssociationTypeMapping.cs
- PeerDuplexChannelListener.cs
- LinqTreeNodeEvaluator.cs
- TypeConverterValueSerializer.cs
- SelfIssuedAuthAsymmetricKey.cs
- MailMessageEventArgs.cs
- ReverseQueryOperator.cs
- SqlFormatter.cs
- ToolstripProfessionalRenderer.cs
- DetailsViewUpdateEventArgs.cs
- XmlArrayItemAttribute.cs
- ImageSourceConverter.cs
- StrokeFIndices.cs
- Rule.cs
- Identity.cs
- SystemIcons.cs
- RefType.cs
- ImageField.cs
- CorrelationHandle.cs
- SqlReferenceCollection.cs
- Errors.cs