Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.ServiceModel.Activities / System / ServiceModel / Activities / CorrelationHandle.cs / 1305376 / CorrelationHandle.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.ServiceModel.Activities { using System; using System.Activities; using System.Collections.ObjectModel; using System.Runtime; using System.Runtime.DurableInstancing; using System.Runtime.Serialization; [DataContract] public class CorrelationHandle : Handle { internal static readonly string StaticExecutionPropertyName = typeof(CorrelationHandle).FullName; static readonly Type requestReplyCorrelationInitializerType = typeof(RequestReplyCorrelationInitializer); // CorrelationHandles to support Context/Durable Duplex // For processing the CallBackContextMessageProperty static readonly Type callbackCorrelationInitializerType = typeof(CallbackCorrelationInitializer); // This is for passing the Context information that we get in the reply message from the Server in the initial handshake // to the next Sendmessage activity from the client to the server static readonly Type contextCorrelationInitializerType = typeof(ContextCorrelationInitializer); //// To get to the same instance on the server side( between SendReply and following Receive) and on the client side( between Send and following send) //static readonly Type followingContextCorrelationInitializerType = typeof(FollowingContextCorrelationInitializer); CorrelationCallbackContext callbackContext; CorrelationContext context; // This is never null when it matters because the CorrelationHandle sets this during OnInitialize [DataMember] NoPersistHandle noPersistHandle; // This is never null when it matters because the CorrelationHandle sets this during OnInitialize [DataMember] BookmarkScopeHandle bookmarkScopeHandle; public CorrelationHandle() : base() { } internal CorrelationRequestContext RequestContext { get; private set; } internal CorrelationResponseContext ResponseContext { get; private set; } [DataMember(EmitDefaultValue = false)] internal CorrelationCallbackContext CallbackContext { get { return this.callbackContext; } set { Fx.Assert(this.callbackContext == null || this.callbackContext == value, "cannot set two different callback contexts"); this.callbackContext = value; } } [DataMember(EmitDefaultValue = false)] internal CorrelationContext Context { get { return this.context; } set { Fx.Assert(this.context == null || this.context == value, "cannot set two different callback contexts"); this.context = value; } } [DataMember(EmitDefaultValue = false)] internal BookmarkScope Scope { get; set; } protected override void OnInitialize(HandleInitializationContext context) { this.noPersistHandle = context.CreateAndInitializeHandle(); this.bookmarkScopeHandle = context.CreateAndInitializeHandle (); } protected override void OnUninitialize(HandleInitializationContext context) { context.UninitializeHandle(this.noPersistHandle); context.UninitializeHandle(this.bookmarkScopeHandle); } internal BookmarkScope EnsureBookmarkScope(NativeActivityContext executionContext) { if (this.Scope == null) { this.Scope = executionContext.DefaultBookmarkScope; } return this.Scope; } internal bool TryRegisterRequestContext(NativeActivityContext executionContext, CorrelationRequestContext requestContext) { Fx.Assert(requestContext != null, "requires a valid requestContext"); if(this.noPersistHandle == null) { return false; } if (this.RequestContext == null) { this.noPersistHandle.Enter(executionContext); this.RequestContext = requestContext; return true; } return object.ReferenceEquals(this.RequestContext, requestContext); } internal bool TryRegisterResponseContext(NativeActivityContext executionContext, CorrelationResponseContext responseContext) { Fx.Assert(responseContext != null, "requires a valid responseContext"); if (this.noPersistHandle == null) { return false; } if (this.ResponseContext == null) { this.noPersistHandle.Enter(executionContext); this.ResponseContext = responseContext; return true; } return object.ReferenceEquals(this.ResponseContext, responseContext); } internal bool TryAcquireRequestContext(NativeActivityContext executionContext, out CorrelationRequestContext requestContext) { if (this.RequestContext != null) { // We have a context, and we should disassociate it from the correlation handle this.noPersistHandle.Exit(executionContext); requestContext = this.RequestContext; this.RequestContext = null; return true; } else { requestContext = null; return false; } } internal bool TryAcquireResponseContext(NativeActivityContext executionContext, out CorrelationResponseContext responseContext) { if (this.ResponseContext != null) { // We have a context, and we should disassociate it from the correlation handle this.noPersistHandle.Exit(executionContext); responseContext = this.ResponseContext; this.ResponseContext = null; return true; } else { responseContext = null; return false; } } internal void InitializeBookmarkScope(NativeActivityContext context, InstanceKey instanceKey) { Fx.Assert(context != null, "executionContext cannot be null"); Fx.Assert(instanceKey != null, "instanceKey cannot be null"); if (this.Scope == null) { this.bookmarkScopeHandle.CreateBookmarkScope(context, instanceKey.Value); this.Scope = this.bookmarkScopeHandle.BookmarkScope; } else { if(this.Scope.IsInitialized) { if (this.Scope.Id != instanceKey.Value) { throw FxTrace.Exception.AsError( new InvalidOperationException(SR.CorrelationHandleInUse(this.Scope.Id, instanceKey.Value))); } } else { this.Scope.Initialize(context, instanceKey.Value); } } } internal bool IsInitalized() { if (this.Scope != null || this.CallbackContext != null || this.Context != null || this.ResponseContext != null || this.RequestContext != null) { return true; } return false; } internal static CorrelationHandle GetExplicitChannelCorrelation(NativeActivityContext context, Collection correlationInitializers) { return GetTypedCorrelationHandle(context, correlationInitializers, requestReplyCorrelationInitializerType); } internal static CorrelationHandle GetExplicitCallbackCorrelation(NativeActivityContext context, Collection correlationInitializers) { return GetTypedCorrelationHandle(context, correlationInitializers, callbackCorrelationInitializerType); } internal static CorrelationHandle GetExplicitContextCorrelation(NativeActivityContext context, Collection correlationInitializers) { return GetTypedCorrelationHandle(context, correlationInitializers, contextCorrelationInitializerType); } internal static CorrelationHandle GetTypedCorrelationHandle(NativeActivityContext context, Collection correlationInitializers, Type correlationInitializerType) { CorrelationHandle typedCorrelationHandle = null; if (correlationInitializers != null && correlationInitializers.Count > 0) { foreach (CorrelationInitializer correlation in correlationInitializers) { if (correlationInitializerType == correlation.GetType()) { typedCorrelationHandle = correlation.CorrelationHandle.Get(context); // We return the first handle we find break; } } } return typedCorrelationHandle; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
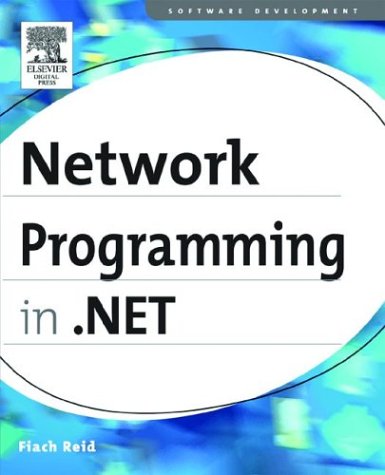
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AutomationPropertyInfo.cs
- ConfigPathUtility.cs
- PartialClassGenerationTaskInternal.cs
- TogglePattern.cs
- HttpStreamXmlDictionaryReader.cs
- MediaSystem.cs
- DocumentApplicationDocumentViewer.cs
- ThicknessAnimation.cs
- AuthenticationModulesSection.cs
- AdjustableArrowCap.cs
- DefaultAuthorizationContext.cs
- ConnectivityStatus.cs
- ConnectionConsumerAttribute.cs
- DaylightTime.cs
- ScrollEventArgs.cs
- RowType.cs
- CodeDomSerializerException.cs
- CheckedListBox.cs
- TypeName.cs
- IndexerReference.cs
- SafeProcessHandle.cs
- TextElementAutomationPeer.cs
- TreeNodeStyleCollection.cs
- TextHintingModeValidation.cs
- RuleRef.cs
- EntityProviderServices.cs
- DecoderBestFitFallback.cs
- ServiceObjectContainer.cs
- SqlFileStream.cs
- Atom10ItemFormatter.cs
- DefaultTextStore.cs
- EventLogSession.cs
- AssemblyResourceLoader.cs
- AutomationProperty.cs
- VisualBrush.cs
- UnmanagedMemoryStream.cs
- CommentAction.cs
- SingleAnimation.cs
- Module.cs
- DataGridLinkButton.cs
- DataGridViewTextBoxCell.cs
- HttpBrowserCapabilitiesBase.cs
- ByteAnimationBase.cs
- InstanceDescriptor.cs
- Int32CAMarshaler.cs
- MemberDomainMap.cs
- FormViewPageEventArgs.cs
- StdRegProviderWrapper.cs
- BamlResourceSerializer.cs
- CodeAttachEventStatement.cs
- StateChangeEvent.cs
- ListViewItem.cs
- FunctionDescription.cs
- WindowsImpersonationContext.cs
- MethodCallExpression.cs
- TypeSystem.cs
- SamlNameIdentifierClaimResource.cs
- VerticalAlignConverter.cs
- ObjectViewQueryResultData.cs
- ThemeInfoAttribute.cs
- ExpandableObjectConverter.cs
- FrameworkElementFactoryMarkupObject.cs
- SiteMapHierarchicalDataSourceView.cs
- ToolStripCodeDomSerializer.cs
- TextStore.cs
- NativeBuffer.cs
- CompilerInfo.cs
- TimeZoneInfo.cs
- StoragePropertyMapping.cs
- XmlILConstructAnalyzer.cs
- EventArgs.cs
- UpdateEventArgs.cs
- DataView.cs
- TypeDescriptorFilterService.cs
- InputProviderSite.cs
- UnitControl.cs
- CachedCompositeFamily.cs
- ValueProviderWrapper.cs
- RuntimeComponentFilter.cs
- Parameter.cs
- InputBindingCollection.cs
- ProcessModule.cs
- SqlMetaData.cs
- TextEvent.cs
- StyleConverter.cs
- GlyphRunDrawing.cs
- WebPartConnectionsDisconnectVerb.cs
- Expander.cs
- ScaleTransform3D.cs
- EventLogPropertySelector.cs
- FontStretches.cs
- Size3D.cs
- DataTable.cs
- ManipulationDeltaEventArgs.cs
- WindowsFormsHelpers.cs
- SapiAttributeParser.cs
- DataGridViewLinkColumn.cs
- _HTTPDateParse.cs
- ConsoleCancelEventArgs.cs
- DeclaredTypeElementCollection.cs