Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CompMod / System / ComponentModel / TypeConverter.cs / 1 / TypeConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using Microsoft.Win32; using System.Collections; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Globalization; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.Runtime.Serialization.Formatters; using System.Security.Permissions; ////// [HostProtection(SharedState = true)] [System.Runtime.InteropServices.ComVisible(true)] public class TypeConverter { ///Converts the value of an object into a different data type. ////// public bool CanConvertFrom(Type sourceType) { return CanConvertFrom(null, sourceType); } ///Gets a value indicating whether this converter can convert an object in the /// given source type to the native type of the converter. ////// public virtual bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(InstanceDescriptor)) { return true; } return false; } ///Gets a value indicating whether this converter can /// convert an object in the given source type to the native type of the converter /// using the context. ////// public bool CanConvertTo(Type destinationType) { return CanConvertTo(null, destinationType); } ////// Gets a value /// indicating whether this converter can convert an object to the given destination /// type. /// ////// public virtual bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { return (destinationType == typeof(string)); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// public object ConvertFrom(object value) { return ConvertFrom(null, CultureInfo.CurrentCulture, value); } ///Converts the given value /// to the converter's native type. ////// public virtual object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { InstanceDescriptor id = value as InstanceDescriptor; if (id != null) { return id.Invoke(); } throw GetConvertFromException(value); } ///Converts the given object to the converter's native type. ////// Converts the given string to the converter's native type using the invariant culture. /// [SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands")] public object ConvertFromInvariantString(string text) { return ConvertFromString(null, CultureInfo.InvariantCulture, text); } ////// Converts the given string to the converter's native type using the invariant culture. /// public object ConvertFromInvariantString(ITypeDescriptorContext context, string text) { return ConvertFromString(context, CultureInfo.InvariantCulture, text); } ////// public object ConvertFromString(string text) { return ConvertFrom(null, null, text); } ///Converts the specified text into an object. ////// public object ConvertFromString(ITypeDescriptorContext context, string text) { return ConvertFrom(context, CultureInfo.CurrentCulture, text); } ///Converts the specified text into an object. ////// public object ConvertFromString(ITypeDescriptorContext context, CultureInfo culture, string text) { return ConvertFrom(context, culture, text); } ///Converts the specified text into an object. ////// public object ConvertTo(object value, Type destinationType) { return ConvertTo(null, null, value, destinationType); } ///Converts the given /// value object to the specified destination type using the arguments. ////// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // keep CultureInfo public virtual object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(string)) { if (value == null) { return String.Empty; } // Pre-whidbey we just did a ToString() here. To minimize the chance of a breaking change we // still send requests for the CurrentCulture to ToString() (which should return the same). if(culture != null && culture != CultureInfo.CurrentCulture) { // VSWhidbey 75433 - If the object is IFormattable, use this interface to convert to string // so we use the specified culture rather than the CurrentCulture like object.ToString() does. IFormattable formattable = value as IFormattable; if(formattable != null) { return formattable.ToString(/* format = */ null, /* formatProvider = */ culture); } } return value.ToString(); } throw GetConvertToException(value, destinationType); } ///Converts the given value object to /// the specified destination type using the specified context and arguments. ////// public string ConvertToInvariantString(object value) { return ConvertToString(null, CultureInfo.InvariantCulture, value); } ///Converts the specified value to a culture-invariant string representation. ////// public string ConvertToInvariantString(ITypeDescriptorContext context, object value) { return ConvertToString(context, CultureInfo.InvariantCulture, value); } ///Converts the specified value to a culture-invariant string representation. ////// [SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands")] public string ConvertToString(object value) { return (string)ConvertTo(null, CultureInfo.CurrentCulture, value, typeof(string)); } ///Converts the specified value to a string representation. ////// public string ConvertToString(ITypeDescriptorContext context, object value) { return (string)ConvertTo(context, CultureInfo.CurrentCulture, value, typeof(string)); } ///Converts the specified value to a string representation. ////// public string ConvertToString(ITypeDescriptorContext context, CultureInfo culture, object value) { return (string)ConvertTo(context, culture, value, typeof(string)); } ///Converts the specified value to a string representation. ////// public object CreateInstance(IDictionary propertyValues) { return CreateInstance(null, propertyValues); } ///Re-creates an ///given a set of property values for the object. /// public virtual object CreateInstance(ITypeDescriptorContext context, IDictionary propertyValues) { return null; } ///Re-creates an ///given a set of property values for the /// object. /// protected Exception GetConvertFromException(object value) { string valueTypeName; if (value == null) { valueTypeName = SR.GetString(SR.ToStringNull); } else { valueTypeName = value.GetType().FullName; } throw new NotSupportedException(SR.GetString(SR.ConvertFromException, GetType().Name, valueTypeName)); } ////// Gets a suitable exception to throw when a conversion cannot /// be performed. /// ////// protected Exception GetConvertToException(object value, Type destinationType) { string valueTypeName; if (value == null) { valueTypeName = SR.GetString(SR.ToStringNull); } else { valueTypeName = value.GetType().FullName; } throw new NotSupportedException(SR.GetString(SR.ConvertToException, GetType().Name, valueTypeName, destinationType.FullName)); } ///Retrieves a suitable exception to throw when a conversion cannot /// be performed. ////// public bool GetCreateInstanceSupported() { return GetCreateInstanceSupported(null); } ///Gets a value indicating whether changing a value on this /// object requires a call to ////// to create a new value. /// public virtual bool GetCreateInstanceSupported(ITypeDescriptorContext context) { return false; } ///Gets a value indicating whether changing a value on this object requires a /// call to ///to create a new value, /// using the specified context. /// public PropertyDescriptorCollection GetProperties(object value) { return GetProperties(null, value); } ///Gets a collection of properties for the type of array specified by the value /// parameter. ////// public PropertyDescriptorCollection GetProperties(ITypeDescriptorContext context, object value) { return GetProperties(context, value, new Attribute[] {BrowsableAttribute.Yes}); } ///Gets a collection of /// properties for the type of array specified by the value parameter using the specified /// context. ////// public virtual PropertyDescriptorCollection GetProperties(ITypeDescriptorContext context, object value, Attribute[] attributes) { return null; } ///Gets a collection of properties for /// the type of array specified by the value parameter using the specified context and /// attributes. ////// public bool GetPropertiesSupported() { return GetPropertiesSupported(null); } ////// Gets a value indicating whether this object supports properties. /// ////// public virtual bool GetPropertiesSupported(ITypeDescriptorContext context) { return false; } ///Gets a value indicating /// whether this object supports properties using the /// specified context. ////// public ICollection GetStandardValues() { return GetStandardValues(null); } ///Gets a collection of standard values for the data type this type /// converter is designed for. ////// public virtual StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { return null; } ///Gets a collection of standard values for the data type this type converter is /// designed for. ////// public bool GetStandardValuesExclusive() { return GetStandardValuesExclusive(null); } ///Gets a value indicating whether the collection of standard values returned from /// ///is an exclusive list. /// public virtual bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } ///Gets a value indicating whether the collection of standard values returned from /// ///is an exclusive /// list of possible values, using the specified context. /// public bool GetStandardValuesSupported() { return GetStandardValuesSupported(null); } ////// Gets a value indicating whether this object supports a standard set of values /// that can be picked from a list. /// ////// public virtual bool GetStandardValuesSupported(ITypeDescriptorContext context) { return false; } ///Gets a value indicating /// whether this object /// supports a standard set of values that can be picked /// from a list using the specified context. ////// public bool IsValid(object value) { return IsValid(null, value); } ////// Gets /// a value indicating whether the given value object is valid for this type. /// ////// public virtual bool IsValid(ITypeDescriptorContext context, object value) { return true; } ///Gets /// a value indicating whether the given value object is valid for this type. ////// protected PropertyDescriptorCollection SortProperties(PropertyDescriptorCollection props, string[] names) { props.Sort(names); return props; } ///Sorts a collection of properties. ////// protected abstract class SimplePropertyDescriptor : PropertyDescriptor { private Type componentType; private Type propertyType; ////// An ////// class that provides /// properties for objects that do not have /// properties. /// /// protected SimplePropertyDescriptor(Type componentType, string name, Type propertyType) : this(componentType, name, propertyType, new Attribute[0]) { } ////// Initializes a new instance of the ////// class. /// /// protected SimplePropertyDescriptor(Type componentType, string name, Type propertyType, Attribute[] attributes) : base(name, attributes) { this.componentType = componentType; this.propertyType = propertyType; } ////// Initializes a new instance of the ///class. /// /// public override Type ComponentType { get { return componentType; } } ////// Gets the type of the component this property description /// is bound to. /// ////// public override bool IsReadOnly { get { return Attributes.Contains(ReadOnlyAttribute.Yes); } } ////// Gets a /// value indicating whether this property is read-only. /// ////// public override Type PropertyType { get { return propertyType; } } ////// Gets the type of the property. /// ////// public override bool CanResetValue(object component) { DefaultValueAttribute attr = (DefaultValueAttribute)Attributes[typeof(DefaultValueAttribute)]; if (attr == null) { return false; } return (attr.Value.Equals(GetValue(component))); } ///Gets a value indicating whether resetting the component /// will change the value of the component. ////// public override void ResetValue(object component) { DefaultValueAttribute attr = (DefaultValueAttribute)Attributes[typeof(DefaultValueAttribute)]; if (attr != null) { SetValue(component, attr.Value); } } ///Resets the value for this property /// of the component. ////// public override bool ShouldSerializeValue(object component) { return false; } } ///Gets a value /// indicating whether the value of this property needs to be persisted. ////// public class StandardValuesCollection : ICollection { private ICollection values; private Array valueArray; ///Represents a collection of values. ////// public StandardValuesCollection(ICollection values) { if (values == null) { values = new object[0]; } Array a = values as Array; if (a != null) { valueArray = a; } this.values = values; } ////// Initializes a new instance of the ////// class. /// /// public int Count { get { if (valueArray != null) { return valueArray.Length; } else { return values.Count; } } } ////// Gets the number of objects in the collection. /// ////// public object this[int index] { get { if (valueArray != null) { return valueArray.GetValue(index); } IList list = values as IList; if (list != null) { return list[index]; } // No other choice but to enumerate the collection. // valueArray = new object[values.Count]; values.CopyTo(valueArray, 0); return valueArray.GetValue(index); } } ///Gets the object at the specified index number. ////// public void CopyTo(Array array, int index) { values.CopyTo(array, index); } ///Copies the contents of this collection to an array. ////// public IEnumerator GetEnumerator() { return values.GetEnumerator(); } ////// Gets an enumerator for this collection. /// ////// /// Retrieves the count of objects in the collection. /// int ICollection.Count { get { return Count; } } ////// /// Determines if this collection is synchronized. /// The ValidatorCollection is not synchronized for /// speed. Also, since it is read-only, there is /// no need to synchronize it. /// bool ICollection.IsSynchronized { get { return false; } } ////// /// Retrieves the synchronization root for this /// collection. Because we are not synchronized, /// this returns null. /// object ICollection.SyncRoot { get { return null; } } ////// /// Copies the contents of this collection to an array. /// void ICollection.CopyTo(Array array, int index) { CopyTo(array, index); } ////// /// Retrieves a new enumerator that can be used to /// iterate over the values in this collection. /// IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.ComponentModel { using Microsoft.Win32; using System.Collections; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Globalization; using System.Runtime.InteropServices; using System.Runtime.Remoting; using System.Runtime.Serialization.Formatters; using System.Security.Permissions; ////// [HostProtection(SharedState = true)] [System.Runtime.InteropServices.ComVisible(true)] public class TypeConverter { ///Converts the value of an object into a different data type. ////// public bool CanConvertFrom(Type sourceType) { return CanConvertFrom(null, sourceType); } ///Gets a value indicating whether this converter can convert an object in the /// given source type to the native type of the converter. ////// public virtual bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(InstanceDescriptor)) { return true; } return false; } ///Gets a value indicating whether this converter can /// convert an object in the given source type to the native type of the converter /// using the context. ////// public bool CanConvertTo(Type destinationType) { return CanConvertTo(null, destinationType); } ////// Gets a value /// indicating whether this converter can convert an object to the given destination /// type. /// ////// public virtual bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { return (destinationType == typeof(string)); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// public object ConvertFrom(object value) { return ConvertFrom(null, CultureInfo.CurrentCulture, value); } ///Converts the given value /// to the converter's native type. ////// public virtual object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { InstanceDescriptor id = value as InstanceDescriptor; if (id != null) { return id.Invoke(); } throw GetConvertFromException(value); } ///Converts the given object to the converter's native type. ////// Converts the given string to the converter's native type using the invariant culture. /// [SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands")] public object ConvertFromInvariantString(string text) { return ConvertFromString(null, CultureInfo.InvariantCulture, text); } ////// Converts the given string to the converter's native type using the invariant culture. /// public object ConvertFromInvariantString(ITypeDescriptorContext context, string text) { return ConvertFromString(context, CultureInfo.InvariantCulture, text); } ////// public object ConvertFromString(string text) { return ConvertFrom(null, null, text); } ///Converts the specified text into an object. ////// public object ConvertFromString(ITypeDescriptorContext context, string text) { return ConvertFrom(context, CultureInfo.CurrentCulture, text); } ///Converts the specified text into an object. ////// public object ConvertFromString(ITypeDescriptorContext context, CultureInfo culture, string text) { return ConvertFrom(context, culture, text); } ///Converts the specified text into an object. ////// public object ConvertTo(object value, Type destinationType) { return ConvertTo(null, null, value, destinationType); } ///Converts the given /// value object to the specified destination type using the arguments. ////// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters")] // keep CultureInfo public virtual object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(string)) { if (value == null) { return String.Empty; } // Pre-whidbey we just did a ToString() here. To minimize the chance of a breaking change we // still send requests for the CurrentCulture to ToString() (which should return the same). if(culture != null && culture != CultureInfo.CurrentCulture) { // VSWhidbey 75433 - If the object is IFormattable, use this interface to convert to string // so we use the specified culture rather than the CurrentCulture like object.ToString() does. IFormattable formattable = value as IFormattable; if(formattable != null) { return formattable.ToString(/* format = */ null, /* formatProvider = */ culture); } } return value.ToString(); } throw GetConvertToException(value, destinationType); } ///Converts the given value object to /// the specified destination type using the specified context and arguments. ////// public string ConvertToInvariantString(object value) { return ConvertToString(null, CultureInfo.InvariantCulture, value); } ///Converts the specified value to a culture-invariant string representation. ////// public string ConvertToInvariantString(ITypeDescriptorContext context, object value) { return ConvertToString(context, CultureInfo.InvariantCulture, value); } ///Converts the specified value to a culture-invariant string representation. ////// [SuppressMessage("Microsoft.Security", "CA2122:DoNotIndirectlyExposeMethodsWithLinkDemands")] public string ConvertToString(object value) { return (string)ConvertTo(null, CultureInfo.CurrentCulture, value, typeof(string)); } ///Converts the specified value to a string representation. ////// public string ConvertToString(ITypeDescriptorContext context, object value) { return (string)ConvertTo(context, CultureInfo.CurrentCulture, value, typeof(string)); } ///Converts the specified value to a string representation. ////// public string ConvertToString(ITypeDescriptorContext context, CultureInfo culture, object value) { return (string)ConvertTo(context, culture, value, typeof(string)); } ///Converts the specified value to a string representation. ////// public object CreateInstance(IDictionary propertyValues) { return CreateInstance(null, propertyValues); } ///Re-creates an ///given a set of property values for the object. /// public virtual object CreateInstance(ITypeDescriptorContext context, IDictionary propertyValues) { return null; } ///Re-creates an ///given a set of property values for the /// object. /// protected Exception GetConvertFromException(object value) { string valueTypeName; if (value == null) { valueTypeName = SR.GetString(SR.ToStringNull); } else { valueTypeName = value.GetType().FullName; } throw new NotSupportedException(SR.GetString(SR.ConvertFromException, GetType().Name, valueTypeName)); } ////// Gets a suitable exception to throw when a conversion cannot /// be performed. /// ////// protected Exception GetConvertToException(object value, Type destinationType) { string valueTypeName; if (value == null) { valueTypeName = SR.GetString(SR.ToStringNull); } else { valueTypeName = value.GetType().FullName; } throw new NotSupportedException(SR.GetString(SR.ConvertToException, GetType().Name, valueTypeName, destinationType.FullName)); } ///Retrieves a suitable exception to throw when a conversion cannot /// be performed. ////// public bool GetCreateInstanceSupported() { return GetCreateInstanceSupported(null); } ///Gets a value indicating whether changing a value on this /// object requires a call to ////// to create a new value. /// public virtual bool GetCreateInstanceSupported(ITypeDescriptorContext context) { return false; } ///Gets a value indicating whether changing a value on this object requires a /// call to ///to create a new value, /// using the specified context. /// public PropertyDescriptorCollection GetProperties(object value) { return GetProperties(null, value); } ///Gets a collection of properties for the type of array specified by the value /// parameter. ////// public PropertyDescriptorCollection GetProperties(ITypeDescriptorContext context, object value) { return GetProperties(context, value, new Attribute[] {BrowsableAttribute.Yes}); } ///Gets a collection of /// properties for the type of array specified by the value parameter using the specified /// context. ////// public virtual PropertyDescriptorCollection GetProperties(ITypeDescriptorContext context, object value, Attribute[] attributes) { return null; } ///Gets a collection of properties for /// the type of array specified by the value parameter using the specified context and /// attributes. ////// public bool GetPropertiesSupported() { return GetPropertiesSupported(null); } ////// Gets a value indicating whether this object supports properties. /// ////// public virtual bool GetPropertiesSupported(ITypeDescriptorContext context) { return false; } ///Gets a value indicating /// whether this object supports properties using the /// specified context. ////// public ICollection GetStandardValues() { return GetStandardValues(null); } ///Gets a collection of standard values for the data type this type /// converter is designed for. ////// public virtual StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { return null; } ///Gets a collection of standard values for the data type this type converter is /// designed for. ////// public bool GetStandardValuesExclusive() { return GetStandardValuesExclusive(null); } ///Gets a value indicating whether the collection of standard values returned from /// ///is an exclusive list. /// public virtual bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } ///Gets a value indicating whether the collection of standard values returned from /// ///is an exclusive /// list of possible values, using the specified context. /// public bool GetStandardValuesSupported() { return GetStandardValuesSupported(null); } ////// Gets a value indicating whether this object supports a standard set of values /// that can be picked from a list. /// ////// public virtual bool GetStandardValuesSupported(ITypeDescriptorContext context) { return false; } ///Gets a value indicating /// whether this object /// supports a standard set of values that can be picked /// from a list using the specified context. ////// public bool IsValid(object value) { return IsValid(null, value); } ////// Gets /// a value indicating whether the given value object is valid for this type. /// ////// public virtual bool IsValid(ITypeDescriptorContext context, object value) { return true; } ///Gets /// a value indicating whether the given value object is valid for this type. ////// protected PropertyDescriptorCollection SortProperties(PropertyDescriptorCollection props, string[] names) { props.Sort(names); return props; } ///Sorts a collection of properties. ////// protected abstract class SimplePropertyDescriptor : PropertyDescriptor { private Type componentType; private Type propertyType; ////// An ////// class that provides /// properties for objects that do not have /// properties. /// /// protected SimplePropertyDescriptor(Type componentType, string name, Type propertyType) : this(componentType, name, propertyType, new Attribute[0]) { } ////// Initializes a new instance of the ////// class. /// /// protected SimplePropertyDescriptor(Type componentType, string name, Type propertyType, Attribute[] attributes) : base(name, attributes) { this.componentType = componentType; this.propertyType = propertyType; } ////// Initializes a new instance of the ///class. /// /// public override Type ComponentType { get { return componentType; } } ////// Gets the type of the component this property description /// is bound to. /// ////// public override bool IsReadOnly { get { return Attributes.Contains(ReadOnlyAttribute.Yes); } } ////// Gets a /// value indicating whether this property is read-only. /// ////// public override Type PropertyType { get { return propertyType; } } ////// Gets the type of the property. /// ////// public override bool CanResetValue(object component) { DefaultValueAttribute attr = (DefaultValueAttribute)Attributes[typeof(DefaultValueAttribute)]; if (attr == null) { return false; } return (attr.Value.Equals(GetValue(component))); } ///Gets a value indicating whether resetting the component /// will change the value of the component. ////// public override void ResetValue(object component) { DefaultValueAttribute attr = (DefaultValueAttribute)Attributes[typeof(DefaultValueAttribute)]; if (attr != null) { SetValue(component, attr.Value); } } ///Resets the value for this property /// of the component. ////// public override bool ShouldSerializeValue(object component) { return false; } } ///Gets a value /// indicating whether the value of this property needs to be persisted. ////// public class StandardValuesCollection : ICollection { private ICollection values; private Array valueArray; ///Represents a collection of values. ////// public StandardValuesCollection(ICollection values) { if (values == null) { values = new object[0]; } Array a = values as Array; if (a != null) { valueArray = a; } this.values = values; } ////// Initializes a new instance of the ////// class. /// /// public int Count { get { if (valueArray != null) { return valueArray.Length; } else { return values.Count; } } } ////// Gets the number of objects in the collection. /// ////// public object this[int index] { get { if (valueArray != null) { return valueArray.GetValue(index); } IList list = values as IList; if (list != null) { return list[index]; } // No other choice but to enumerate the collection. // valueArray = new object[values.Count]; values.CopyTo(valueArray, 0); return valueArray.GetValue(index); } } ///Gets the object at the specified index number. ////// public void CopyTo(Array array, int index) { values.CopyTo(array, index); } ///Copies the contents of this collection to an array. ////// public IEnumerator GetEnumerator() { return values.GetEnumerator(); } ////// Gets an enumerator for this collection. /// ////// /// Retrieves the count of objects in the collection. /// int ICollection.Count { get { return Count; } } ////// /// Determines if this collection is synchronized. /// The ValidatorCollection is not synchronized for /// speed. Also, since it is read-only, there is /// no need to synchronize it. /// bool ICollection.IsSynchronized { get { return false; } } ////// /// Retrieves the synchronization root for this /// collection. Because we are not synchronized, /// this returns null. /// object ICollection.SyncRoot { get { return null; } } ////// /// Copies the contents of this collection to an array. /// void ICollection.CopyTo(Array array, int index) { CopyTo(array, index); } ////// /// Retrieves a new enumerator that can be used to /// iterate over the values in this collection. /// IEnumerator IEnumerable.GetEnumerator() { return GetEnumerator(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
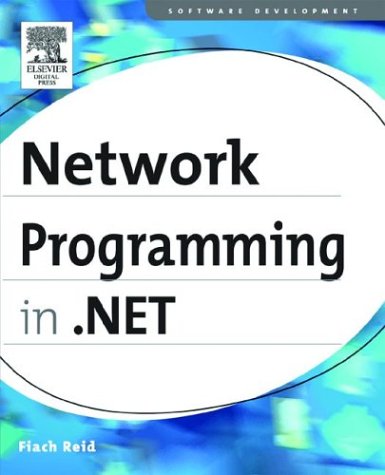
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MsmqInputMessage.cs
- SplashScreen.cs
- UnicastIPAddressInformationCollection.cs
- CertificateManager.cs
- OwnerDrawPropertyBag.cs
- Match.cs
- PenLineJoinValidation.cs
- CorePropertiesFilter.cs
- Point3DCollection.cs
- CommandManager.cs
- DiscreteKeyFrames.cs
- PersianCalendar.cs
- ClaimTypes.cs
- TextParentUndoUnit.cs
- ToolStripTextBox.cs
- EventDescriptor.cs
- LambdaCompiler.Generated.cs
- StreamInfo.cs
- RemotingException.cs
- DataRowCollection.cs
- KeyToListMap.cs
- _AuthenticationState.cs
- ExclusiveHandleList.cs
- Byte.cs
- WindowsAuthenticationModule.cs
- ConfigXmlWhitespace.cs
- XmlSerializerAssemblyAttribute.cs
- PriorityQueue.cs
- ExceptionHandler.cs
- SwitchAttribute.cs
- VerticalAlignConverter.cs
- Matrix.cs
- controlskin.cs
- Parallel.cs
- ContractNamespaceAttribute.cs
- HttpListenerTimeoutManager.cs
- MultiView.cs
- DataGridViewUtilities.cs
- CacheHelper.cs
- ToolStripAdornerWindowService.cs
- CodeTypeReferenceExpression.cs
- NotifyIcon.cs
- sqlinternaltransaction.cs
- SqlDataReaderSmi.cs
- DataSetMappper.cs
- MissingSatelliteAssemblyException.cs
- WSSecurityOneDotZeroReceiveSecurityHeader.cs
- UrlMappingsSection.cs
- DataRowChangeEvent.cs
- LeftCellWrapper.cs
- LineMetrics.cs
- ContentFilePart.cs
- QuotedPairReader.cs
- ComPlusInstanceContextInitializer.cs
- OpenFileDialog.cs
- cookiecollection.cs
- ConstNode.cs
- PreservationFileWriter.cs
- ButtonBase.cs
- Window.cs
- Single.cs
- ProxyGenerationError.cs
- RightsManagementEncryptionTransform.cs
- ConfigurationManagerHelperFactory.cs
- CustomAttributeBuilder.cs
- XmlSchemaCompilationSettings.cs
- TimeManager.cs
- _NegoState.cs
- DbConnectionPoolIdentity.cs
- ToolStripDropDownClosedEventArgs.cs
- ListBindingConverter.cs
- PanelDesigner.cs
- RightsManagementEncryptedStream.cs
- TemplateControlBuildProvider.cs
- ObjectStateEntryDbUpdatableDataRecord.cs
- TemplateEditingVerb.cs
- ExpressionReplacer.cs
- PeerNameRecordCollection.cs
- DependencyObjectType.cs
- PropertyHelper.cs
- MissingManifestResourceException.cs
- KnownAssembliesSet.cs
- StatusBarDrawItemEvent.cs
- Misc.cs
- ItemsPanelTemplate.cs
- HitTestDrawingContextWalker.cs
- Animatable.cs
- AbsoluteQuery.cs
- SubclassTypeValidatorAttribute.cs
- ListBindingConverter.cs
- FileUpload.cs
- Base64Stream.cs
- DocumentsTrace.cs
- Knowncolors.cs
- GridEntry.cs
- StringToken.cs
- PartialTrustVisibleAssemblyCollection.cs
- ConnectionOrientedTransportManager.cs
- ImageFormatConverter.cs
- ItemChangedEventArgs.cs