Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / Inlined / DecimalSumAggregationOperator.cs / 1305376 / DecimalSumAggregationOperator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // DecimalSumAggregationOperator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined sum aggregation and its enumerator, for decimals. /// internal sealed class DecimalSumAggregationOperator : InlinedAggregationOperator{ //---------------------------------------------------------------------------------------- // Constructs a new instance of a sum associative operator. // internal DecimalSumAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override decimal InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // We just reduce the elements in each output partition. decimal sum = 0.0m; while (enumerator.MoveNext()) { sum += enumerator.Current; } return sum; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new DecimalSumAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class DecimalSumAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator { private QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal DecimalSumAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the sum of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref decimal currentElement) { decimal element = default(decimal); TKey keyUnused = default(TKey); QueryOperatorEnumerator source = m_source; if (source.MoveNext(ref element, ref keyUnused)) { // We just scroll through the enumerator and accumulate the sum. decimal tempSum = 0.0m; int i = 0; do { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); tempSum += element; } while (source.MoveNext(ref element, ref keyUnused)); // The sum has been calculated. Now just return. currentElement = tempSum; return true; } return false; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // DecimalSumAggregationOperator.cs // // [....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined sum aggregation and its enumerator, for decimals. /// internal sealed class DecimalSumAggregationOperator : InlinedAggregationOperator{ //---------------------------------------------------------------------------------------- // Constructs a new instance of a sum associative operator. // internal DecimalSumAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override decimal InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // We just reduce the elements in each output partition. decimal sum = 0.0m; while (enumerator.MoveNext()) { sum += enumerator.Current; } return sum; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new DecimalSumAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class DecimalSumAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator { private QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal DecimalSumAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the sum of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref decimal currentElement) { decimal element = default(decimal); TKey keyUnused = default(TKey); QueryOperatorEnumerator source = m_source; if (source.MoveNext(ref element, ref keyUnused)) { // We just scroll through the enumerator and accumulate the sum. decimal tempSum = 0.0m; int i = 0; do { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); tempSum += element; } while (source.MoveNext(ref element, ref keyUnused)); // The sum has been calculated. Now just return. currentElement = tempSum; return true; } return false; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
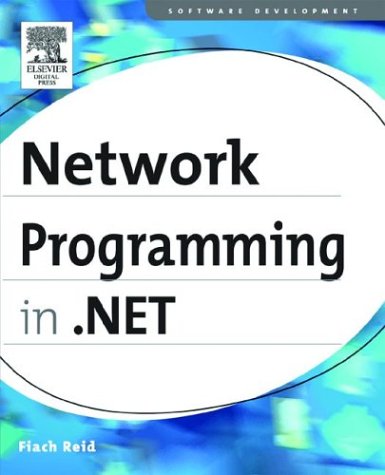
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RawStylusActions.cs
- TableChangeProcessor.cs
- SequentialOutput.cs
- TemplateControlCodeDomTreeGenerator.cs
- NamespaceExpr.cs
- ReadWriteObjectLock.cs
- ADMembershipUser.cs
- RectangleHotSpot.cs
- ProviderBase.cs
- LoadedOrUnloadedOperation.cs
- ConfigXmlWhitespace.cs
- ShapingWorkspace.cs
- QilInvokeEarlyBound.cs
- InkPresenter.cs
- RefreshPropertiesAttribute.cs
- TableRowGroupCollection.cs
- UnauthorizedWebPart.cs
- PersonalizationProvider.cs
- FrameworkContentElementAutomationPeer.cs
- DataObjectAttribute.cs
- XPathBinder.cs
- EntityDataSourceViewSchema.cs
- RootDesignerSerializerAttribute.cs
- TypeConverterAttribute.cs
- AuthenticationManager.cs
- AssemblyCollection.cs
- BaseCollection.cs
- WebPartsSection.cs
- Keywords.cs
- CatalogZone.cs
- InvalidComObjectException.cs
- AudioFormatConverter.cs
- TiffBitmapEncoder.cs
- GcSettings.cs
- Native.cs
- WebContext.cs
- PolyQuadraticBezierSegmentFigureLogic.cs
- MimeWriter.cs
- TemplatedWizardStep.cs
- DateTimeFormatInfo.cs
- OleDbSchemaGuid.cs
- GetRecipientRequest.cs
- AttachedAnnotation.cs
- XslException.cs
- OraclePermission.cs
- AsyncWaitHandle.cs
- TypeConverters.cs
- FlatButtonAppearance.cs
- BinaryWriter.cs
- ChannelSinkStacks.cs
- RunWorkerCompletedEventArgs.cs
- Encoder.cs
- NonBatchDirectoryCompiler.cs
- ResolveNameEventArgs.cs
- GraphicsPath.cs
- dataSvcMapFileLoader.cs
- TreeViewCancelEvent.cs
- CalendarDateRange.cs
- CLRBindingWorker.cs
- SchemaInfo.cs
- XmlSerializerSection.cs
- IncomingWebRequestContext.cs
- EventLogException.cs
- SecurityHelper.cs
- BaseDataList.cs
- StringUtil.cs
- ImageBrush.cs
- AssemblyInfo.cs
- NullReferenceException.cs
- MarkedHighlightComponent.cs
- SqlUnionizer.cs
- ExtensionSimplifierMarkupObject.cs
- TypeResolver.cs
- NativeStructs.cs
- HtmlTableRow.cs
- ColumnCollection.cs
- HashStream.cs
- DispatcherHooks.cs
- FileLoadException.cs
- HttpProfileBase.cs
- FunctionParameter.cs
- RequestQueue.cs
- WebPartZoneCollection.cs
- ArrangedElement.cs
- wpf-etw.cs
- KeyPressEvent.cs
- FlowLayoutPanel.cs
- DrawingBrush.cs
- ImpersonationContext.cs
- IOThreadTimer.cs
- TypeBuilder.cs
- ConfigXmlElement.cs
- Viewport3DVisual.cs
- ForeignKeyConstraint.cs
- NetMsmqBinding.cs
- DataColumnCollection.cs
- StringPropertyBuilder.cs
- _SingleItemRequestCache.cs
- AuthStoreRoleProvider.cs
- sqlcontext.cs