Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Base / System / Windows / Threading / DispatcherHooks.cs / 1 / DispatcherHooks.cs
using System.Security; using System.Security.Permissions; namespace System.Windows.Threading { ////// Additional information provided about a dispatcher. /// public sealed class DispatcherHooks { ////// An event indicating the the dispatcher has no more operations to process. /// ////// Note that this event will be raised by the dispatcher thread when /// there is no more pending work to do. /// /// Note also that this event could come before the last operation is /// invoked, because that is when we determine that the queue is empty. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _dispatcherInactive /// TreatAsSafe: link-demands /// public event EventHandler DispatcherInactive { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _dispatcherInactive += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _dispatcherInactive -= value; } } } ////// An event indicating that an operation was posted to the dispatcher. /// ////// Typically this is due to the BeginInvoke API, but the Invoke API can /// also cause this if any priority other than DispatcherPriority.Send is /// specified, or if the destination dispatcher is owned by a different /// thread. /// /// Note that any thread can post operations, so this event can be /// raised by any thread. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _operationPosted /// TreatAsSafe: link-demands /// public event DispatcherHookEventHandler OperationPosted { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _operationPosted += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _operationPosted -= value; } } } ////// An event indicating that an operation was completed. /// ////// Note that this event will be raised by the dispatcher thread after /// the operation has completed. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _operationCompleted /// TreatAsSafe: link-demands /// public event DispatcherHookEventHandler OperationCompleted { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _operationCompleted += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _operationCompleted -= value; } } } ////// An event indicating that the priority of an operation was changed. /// ////// Note that any thread can change the priority of operations, /// so this event can be raised by any thread. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _operationPriorityChanged /// TreatAsSafe: link-demands /// public event DispatcherHookEventHandler OperationPriorityChanged { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _operationPriorityChanged += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _operationPriorityChanged -= value; } } } ////// An event indicating that an operation was aborted. /// ////// Note that any thread can abort an operation, so this event /// can be raised by any thread. /// Callers must have UIPermission(PermissionState.Unrestricted) to call this API. /// ////// Critical: accesses _operationAborted /// TreatAsSafe: link-demands /// public event DispatcherHookEventHandler OperationAborted { [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] add { lock(_instanceLock) { _operationAborted += value; } } [SecurityCritical] [UIPermissionAttribute(SecurityAction.LinkDemand,Unrestricted=true)] remove { lock(_instanceLock) { _operationAborted -= value; } } } // Only we can create these things. internal DispatcherHooks() { } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseDispatcherInactive(Dispatcher dispatcher) { EventHandler dispatcherInactive = _dispatcherInactive; if(dispatcherInactive != null) { dispatcherInactive(dispatcher, EventArgs.Empty); } } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseOperationPosted(Dispatcher dispatcher, DispatcherOperation operation) { DispatcherHookEventHandler operationPosted = _operationPosted; if(operationPosted != null) { operationPosted(dispatcher, new DispatcherHookEventArgs(operation)); } } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseOperationCompleted(Dispatcher dispatcher, DispatcherOperation operation) { DispatcherHookEventHandler operationCompleted = _operationCompleted; if(operationCompleted != null) { operationCompleted(dispatcher, new DispatcherHookEventArgs(operation)); } } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseOperationPriorityChanged(Dispatcher dispatcher, DispatcherOperation operation) { DispatcherHookEventHandler operationPriorityChanged = _operationPriorityChanged; if(operationPriorityChanged != null) { operationPriorityChanged(dispatcher, new DispatcherHookEventArgs(operation)); } } ////// Critical: accesses _operationAborted /// TreatAsSafe: no exposure /// [SecurityCritical, SecurityTreatAsSafe] internal void RaiseOperationAborted(Dispatcher dispatcher, DispatcherOperation operation) { DispatcherHookEventHandler operationAborted = _operationAborted; if(operationAborted != null) { operationAborted(dispatcher, new DispatcherHookEventArgs(operation)); } } private object _instanceLock = new object(); ////// Do not expose to partially trusted code. /// [SecurityCritical] private EventHandler _dispatcherInactive; ////// Do not expose to partially trusted code. /// [SecurityCritical] private DispatcherHookEventHandler _operationPosted; ////// Do not expose to partially trusted code. /// [SecurityCritical] private DispatcherHookEventHandler _operationCompleted; ////// Do not expose to partially trusted code. /// [SecurityCritical] private DispatcherHookEventHandler _operationPriorityChanged; ////// Do not expose to partially trusted code. /// [SecurityCritical] private DispatcherHookEventHandler _operationAborted; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
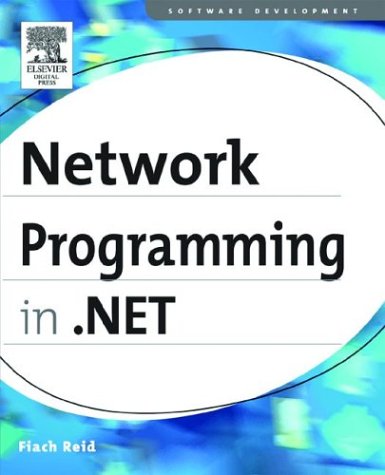
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SrgsElement.cs
- Point3DCollectionValueSerializer.cs
- ToolstripProfessionalRenderer.cs
- RecognizerInfo.cs
- TailPinnedEventArgs.cs
- ToolStripControlHost.cs
- TimeZoneNotFoundException.cs
- StringConverter.cs
- DataGridViewAdvancedBorderStyle.cs
- RectAnimation.cs
- NetTcpBinding.cs
- GatewayDefinition.cs
- PropertyGrid.cs
- SynchronousChannel.cs
- SiteMapNodeItemEventArgs.cs
- TextEvent.cs
- BuildProviderCollection.cs
- TableDetailsCollection.cs
- AppDomainManager.cs
- ChangeProcessor.cs
- ExpressionNode.cs
- Debug.cs
- DeploymentExceptionMapper.cs
- WeakReference.cs
- InvalidTimeZoneException.cs
- ObjectStateManagerMetadata.cs
- Peer.cs
- Pointer.cs
- ElementProxy.cs
- CodeStatementCollection.cs
- Vector3DCollection.cs
- ListControl.cs
- DiagnosticTrace.cs
- CompiledIdentityConstraint.cs
- StickyNoteHelper.cs
- SamlAuthorityBinding.cs
- EncodingDataItem.cs
- RectIndependentAnimationStorage.cs
- SchemaImporterExtensionElementCollection.cs
- InvalidOleVariantTypeException.cs
- WebPartDescription.cs
- Pair.cs
- Mappings.cs
- HttpModuleActionCollection.cs
- ThicknessAnimationUsingKeyFrames.cs
- Int32RectConverter.cs
- CodeObjectCreateExpression.cs
- HttpMethodConstraint.cs
- RuleEngine.cs
- WindowsEditBoxRange.cs
- EventLogPermissionEntry.cs
- OutputCacheProfile.cs
- CodeTypeDeclaration.cs
- HtmlWindowCollection.cs
- EventWaitHandle.cs
- Section.cs
- ReliabilityContractAttribute.cs
- PersistenceException.cs
- PassportAuthentication.cs
- ByteStreamGeometryContext.cs
- SqlEnums.cs
- WebPageTraceListener.cs
- SrgsElementList.cs
- URLIdentityPermission.cs
- StorageScalarPropertyMapping.cs
- Brush.cs
- SQlBooleanStorage.cs
- SettingsBase.cs
- MaterialGroup.cs
- DataColumnSelectionConverter.cs
- DecoderFallbackWithFailureFlag.cs
- PrintPreviewDialog.cs
- ProcessModelSection.cs
- NavigationHelper.cs
- HostProtectionException.cs
- ThreadSafeList.cs
- BitVector32.cs
- BlurBitmapEffect.cs
- GenericEnumConverter.cs
- OdbcUtils.cs
- StreamWriter.cs
- TextTreeInsertUndoUnit.cs
- BlockUIContainer.cs
- BaseTemplateParser.cs
- SoapAttributeAttribute.cs
- FatalException.cs
- ValuePatternIdentifiers.cs
- XmlSchemaComplexContentRestriction.cs
- ActivationWorker.cs
- ObjectPersistData.cs
- Int32Collection.cs
- RangeValidator.cs
- LinearGradientBrush.cs
- ToolStripSettings.cs
- MonthCalendar.cs
- WebPartEventArgs.cs
- RequestTimeoutManager.cs
- SatelliteContractVersionAttribute.cs
- DragSelectionMessageFilter.cs
- FixedSchema.cs