Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / documents / UIElementIsland.cs / 1305600 / UIElementIsland.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: UIElementIsland.cs // // Description: UIElement layout island. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; // Listusing System.Collections.ObjectModel; // ReadOnlyCollection using System.Windows; // UIElement using System.Windows.Media; // Visual namespace MS.Internal.Documents { /// /// UIElement layout island. /// internal class UIElementIsland : ContainerVisual, IContentHost, IDisposable { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Create an instance of a UIElementIsland. /// internal UIElementIsland(UIElement child) { SetFlags(true, VisualFlags.IsLayoutIslandRoot); _child = child; if (_child != null) { // Disconnect visual from its old parent, if necessary. Visual currentParent = VisualTreeHelper.GetParent(_child) as Visual; if (currentParent != null) { Invariant.Assert(currentParent is UIElementIsland, "Parent should always be a UIElementIsland."); ((UIElementIsland)currentParent).Dispose(); } // Notify the Visual layer that a new child appeared. Children.Add(_child); } } #endregion Constructors //-------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------- #region Internal Methods ////// Do layout of entire UIElement Island. /// /// Avalilable slot size of the UIElement Island. /// Whether horizontal autosizing is enabled. /// Whether vertical autosizing is enabled. ///The size of the UIElement Island. internal Size DoLayout(Size availableSize, bool horizontalAutoSize, bool verticalAutoSize) { Size islandSize = new Size(); if (_child != null) { // Get FlowDirection from logical parent and set it on UIElemntIsland // to get layout mirroring provided by the layout system. if (_child is FrameworkElement && ((FrameworkElement)_child).Parent != null) { SetValue(FrameworkElement.FlowDirectionProperty, ((FrameworkElement)_child).Parent.GetValue(FrameworkElement.FlowDirectionProperty)); } try { _layoutInProgress = true; // Measure UIElement _child.Measure(availableSize); // Arrange UIElement islandSize.Width = horizontalAutoSize ? _child.DesiredSize.Width : availableSize.Width; islandSize.Height = verticalAutoSize ? _child.DesiredSize.Height : availableSize.Height; _child.Arrange(new Rect(islandSize)); } finally { _layoutInProgress = false; } } return islandSize; } #endregion Internal Methods //-------------------------------------------------------------------- // // Internal Properties // //-------------------------------------------------------------------- #region Internal Properties ////// Root of UIElement island. /// internal UIElement Root { get { return _child; } } #endregion Internal Properties //------------------------------------------------------------------- // // Internal Events // //-------------------------------------------------------------------- #region Internal Events ////// Fired after DesiredSize for child UIElement has been changed. /// internal event DesiredSizeChangedEventHandler DesiredSizeChanged; #endregion Internal Events //------------------------------------------------------------------- // // Private Fields // //------------------------------------------------------------------- #region Private Fields private UIElement _child; // Hosted UIElement root. private bool _layoutInProgress; // Whether layout is in progress. #endregion Private Fields //------------------------------------------------------------------- // // IDisposable Members // //-------------------------------------------------------------------- #region IDisposable Members ////// public void Dispose() { if (_child != null) { Children.Clear(); _child = null; } GC.SuppressFinalize(this); } #endregion IDisposable Members //------------------------------------------------------------------- // // IContentHost Members // //-------------------------------------------------------------------- #region IContentHost Members ////// /// IInputElement IContentHost.InputHitTest(Point point) { // UIElementIsland only hosts UIElements, which can be found by the // normal hit-testing logic, so we don't need to provide our own // hit-testing implementation. return null; } ////// /// ReadOnlyCollection/// IContentHost.GetRectangles(ContentElement child) { return new ReadOnlyCollection (new List ()); } /// /// IEnumerator/// IContentHost.HostedElements { get { List hostedElements = new List (); if (_child != null) { hostedElements.Add(_child); } return hostedElements.GetEnumerator(); } } /// /// void IContentHost.OnChildDesiredSizeChanged(UIElement child) { Invariant.Assert(child == _child); if (!_layoutInProgress && DesiredSizeChanged != null) { DesiredSizeChanged(this, new DesiredSizeChangedEventArgs(child)); } } #endregion IContentHost Members } ////// /// DesiredSizeChanged event handler. /// internal delegate void DesiredSizeChangedEventHandler(object sender, DesiredSizeChangedEventArgs e); ////// Event arguments for the DesiredSizeChanged event. /// internal class DesiredSizeChangedEventArgs : EventArgs { ////// Constructor. /// /// UIElement for which DesiredSize has been changed. internal DesiredSizeChangedEventArgs(UIElement child) { _child = child; } ////// UIElement for which DesiredSize has been changed. /// internal UIElement Child { get { return _child; } } ////// UIElement for which DesiredSize has been changed. /// private readonly UIElement _child; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: UIElementIsland.cs // // Description: UIElement layout island. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; // Listusing System.Collections.ObjectModel; // ReadOnlyCollection using System.Windows; // UIElement using System.Windows.Media; // Visual namespace MS.Internal.Documents { /// /// UIElement layout island. /// internal class UIElementIsland : ContainerVisual, IContentHost, IDisposable { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Create an instance of a UIElementIsland. /// internal UIElementIsland(UIElement child) { SetFlags(true, VisualFlags.IsLayoutIslandRoot); _child = child; if (_child != null) { // Disconnect visual from its old parent, if necessary. Visual currentParent = VisualTreeHelper.GetParent(_child) as Visual; if (currentParent != null) { Invariant.Assert(currentParent is UIElementIsland, "Parent should always be a UIElementIsland."); ((UIElementIsland)currentParent).Dispose(); } // Notify the Visual layer that a new child appeared. Children.Add(_child); } } #endregion Constructors //-------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------- #region Internal Methods ////// Do layout of entire UIElement Island. /// /// Avalilable slot size of the UIElement Island. /// Whether horizontal autosizing is enabled. /// Whether vertical autosizing is enabled. ///The size of the UIElement Island. internal Size DoLayout(Size availableSize, bool horizontalAutoSize, bool verticalAutoSize) { Size islandSize = new Size(); if (_child != null) { // Get FlowDirection from logical parent and set it on UIElemntIsland // to get layout mirroring provided by the layout system. if (_child is FrameworkElement && ((FrameworkElement)_child).Parent != null) { SetValue(FrameworkElement.FlowDirectionProperty, ((FrameworkElement)_child).Parent.GetValue(FrameworkElement.FlowDirectionProperty)); } try { _layoutInProgress = true; // Measure UIElement _child.Measure(availableSize); // Arrange UIElement islandSize.Width = horizontalAutoSize ? _child.DesiredSize.Width : availableSize.Width; islandSize.Height = verticalAutoSize ? _child.DesiredSize.Height : availableSize.Height; _child.Arrange(new Rect(islandSize)); } finally { _layoutInProgress = false; } } return islandSize; } #endregion Internal Methods //-------------------------------------------------------------------- // // Internal Properties // //-------------------------------------------------------------------- #region Internal Properties ////// Root of UIElement island. /// internal UIElement Root { get { return _child; } } #endregion Internal Properties //------------------------------------------------------------------- // // Internal Events // //-------------------------------------------------------------------- #region Internal Events ////// Fired after DesiredSize for child UIElement has been changed. /// internal event DesiredSizeChangedEventHandler DesiredSizeChanged; #endregion Internal Events //------------------------------------------------------------------- // // Private Fields // //------------------------------------------------------------------- #region Private Fields private UIElement _child; // Hosted UIElement root. private bool _layoutInProgress; // Whether layout is in progress. #endregion Private Fields //------------------------------------------------------------------- // // IDisposable Members // //-------------------------------------------------------------------- #region IDisposable Members ////// public void Dispose() { if (_child != null) { Children.Clear(); _child = null; } GC.SuppressFinalize(this); } #endregion IDisposable Members //------------------------------------------------------------------- // // IContentHost Members // //-------------------------------------------------------------------- #region IContentHost Members ////// /// IInputElement IContentHost.InputHitTest(Point point) { // UIElementIsland only hosts UIElements, which can be found by the // normal hit-testing logic, so we don't need to provide our own // hit-testing implementation. return null; } ////// /// ReadOnlyCollection/// IContentHost.GetRectangles(ContentElement child) { return new ReadOnlyCollection (new List ()); } /// /// IEnumerator/// IContentHost.HostedElements { get { List hostedElements = new List (); if (_child != null) { hostedElements.Add(_child); } return hostedElements.GetEnumerator(); } } /// /// void IContentHost.OnChildDesiredSizeChanged(UIElement child) { Invariant.Assert(child == _child); if (!_layoutInProgress && DesiredSizeChanged != null) { DesiredSizeChanged(this, new DesiredSizeChangedEventArgs(child)); } } #endregion IContentHost Members } ////// /// DesiredSizeChanged event handler. /// internal delegate void DesiredSizeChangedEventHandler(object sender, DesiredSizeChangedEventArgs e); ////// Event arguments for the DesiredSizeChanged event. /// internal class DesiredSizeChangedEventArgs : EventArgs { ////// Constructor. /// /// UIElement for which DesiredSize has been changed. internal DesiredSizeChangedEventArgs(UIElement child) { _child = child; } ////// UIElement for which DesiredSize has been changed. /// internal UIElement Child { get { return _child; } } ////// UIElement for which DesiredSize has been changed. /// private readonly UIElement _child; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
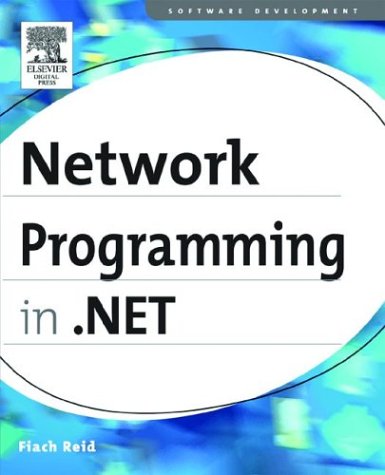
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AuthenticationService.cs
- StylusPointDescription.cs
- QuaternionIndependentAnimationStorage.cs
- RoleService.cs
- PersonalizationProvider.cs
- OdbcParameter.cs
- BaseTreeIterator.cs
- DecoderNLS.cs
- SqlDataAdapter.cs
- FieldCollectionEditor.cs
- NavigatorOutput.cs
- input.cs
- SQLResource.cs
- ImmutableObjectAttribute.cs
- EntityViewGenerationConstants.cs
- ConfigXmlComment.cs
- CategoryEditor.cs
- ErrorFormatterPage.cs
- CodeExpressionCollection.cs
- OpCopier.cs
- SharedUtils.cs
- DesignOnlyAttribute.cs
- DependencyObjectType.cs
- WebPartExportVerb.cs
- BCLDebug.cs
- UIElementCollection.cs
- OleDbConnection.cs
- EditorZoneDesigner.cs
- ImageEditor.cs
- GiveFeedbackEvent.cs
- MetricEntry.cs
- AdCreatedEventArgs.cs
- EntityCommandCompilationException.cs
- WebServiceMethodData.cs
- EntitySetBaseCollection.cs
- StylusPointPropertyUnit.cs
- SqlInfoMessageEvent.cs
- XmlSerializationWriter.cs
- Hyperlink.cs
- DirectoryInfo.cs
- SystemIPGlobalStatistics.cs
- ButtonChrome.cs
- PagesChangedEventArgs.cs
- HttpServerUtilityBase.cs
- ColumnMapProcessor.cs
- HostingEnvironmentSection.cs
- EngineSiteSapi.cs
- ObjectDisposedException.cs
- DefaultBindingPropertyAttribute.cs
- AttachedAnnotationChangedEventArgs.cs
- ListViewVirtualItemsSelectionRangeChangedEvent.cs
- FileStream.cs
- ConfigXmlCDataSection.cs
- SafeMemoryMappedFileHandle.cs
- ReadOnlyCollectionBase.cs
- AnchoredBlock.cs
- SecondaryIndexDefinition.cs
- SchemaReference.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- JournalEntryListConverter.cs
- Timer.cs
- HelpEvent.cs
- contentDescriptor.cs
- WindowsToolbar.cs
- WebPartTransformerCollection.cs
- IMembershipProvider.cs
- AnnotationHelper.cs
- TreeWalkHelper.cs
- TextElement.cs
- RemoveStoryboard.cs
- AnnotationMap.cs
- BackgroundFormatInfo.cs
- MultipleViewPatternIdentifiers.cs
- CryptoProvider.cs
- GridViewRowPresenterBase.cs
- _ChunkParse.cs
- ConnectionManager.cs
- WebPartManagerInternals.cs
- ConstructorNeedsTagAttribute.cs
- ListViewDataItem.cs
- SimplePropertyEntry.cs
- ProcessModule.cs
- HorizontalAlignConverter.cs
- MdiWindowListStrip.cs
- SimpleHandlerFactory.cs
- XmlBoundElement.cs
- DesignerLoader.cs
- ChannelReliableSession.cs
- XmlEncoding.cs
- ExtentKey.cs
- DependencySource.cs
- WebRequestModulesSection.cs
- DataGridViewBand.cs
- MonthChangedEventArgs.cs
- AlignmentYValidation.cs
- ManagedIStream.cs
- SystemColorTracker.cs
- XsdBuilder.cs
- log.cs
- HttpDigestClientElement.cs