Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / Utils / ExternalCalls.cs / 1305376 / ExternalCalls.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Mapping.ViewGeneration.Structures; using System.Data.Common; using System.Data.Common.CommandTrees; using System.Data.Common.CommandTrees.ExpressionBuilder; using System.Data.Common.EntitySql; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Collections.Generic; using System.Linq; namespace System.Data.Mapping.ViewGeneration.Utils { // This class encapsulates "external" calls from view/UDF generation // to other System.Data.Entity features internal static class ExternalCalls { static internal bool IsReservedKeyword(string name) { return CqlLexer.IsReservedKeyword(name); } static internal DbCommandTree CompileView( string viewDef, StorageMappingItemCollection mappingItemCollection, ParserOptions.CompilationMode compilationMode) { Debug.Assert(!String.IsNullOrEmpty(viewDef), "!String.IsNullOrEmpty(viewDef)"); Debug.Assert(mappingItemCollection != null, "mappingItemCollection != null"); Debug.Assert(mappingItemCollection.EdmItemCollection != null, "mappingItemCollection.EdmItemCollection != null"); Debug.Assert(mappingItemCollection.StoreItemCollection != null, "mappingItemCollection.StoreItemCollection != null"); MetadataWorkspace workspace = new MetadataWorkspace(); workspace.RegisterItemCollection(mappingItemCollection.EdmItemCollection); workspace.RegisterItemCollection(mappingItemCollection.StoreItemCollection); workspace.RegisterItemCollection(mappingItemCollection); Perspective perspective = new TargetPerspective(workspace); ParserOptions parserOptions = new ParserOptions(); parserOptions.ParserCompilationMode = compilationMode; DbCommandTree expr = CqlQuery.Compile(viewDef, perspective, parserOptions, null); Debug.Assert(expr != null, "Compile returned empty tree?"); return expr; } ////// Compiles eSQL static internal DbLambda CompileFunctionDefinition( string functionFullName, string functionDefinition, IListand returns . /// Guarantees type match of lambda variables and . /// Passes thru all excepions coming from . /// functionParameters, EdmItemCollection edmItemCollection) { Debug.Assert(!String.IsNullOrEmpty(functionFullName), "!String.IsNullOrEmpty(functionFullName)"); Debug.Assert(!String.IsNullOrEmpty(functionDefinition), "!String.IsNullOrEmpty(functionDefinition)"); Debug.Assert(functionParameters != null, "functionParameters != null"); Debug.Assert(edmItemCollection != null, "edmItemCollection != null"); MetadataWorkspace workspace = new MetadataWorkspace(); workspace.RegisterItemCollection(edmItemCollection); Perspective perspective = new ModelPerspective(workspace); // Since we compile lambda expression and generate variables from the function parameter definitions, // the returned DbLambda will contain variable types that match function parameter types. DbLambda functionBody = CqlQuery.CompileQueryCommandLambda( functionDefinition, perspective, null /* use default parser options */, null /* parameters */, functionParameters.Select(pInfo => pInfo.TypeUsage.Variable(pInfo.Name))); Debug.Assert(functionBody != null, "functionBody != null"); return functionBody; } static internal ItemCollection GetItemCollection(MetadataWorkspace workspace, DataSpace space) { return workspace.GetItemCollection(space); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Mapping.ViewGeneration.Structures; using System.Data.Common; using System.Data.Common.CommandTrees; using System.Data.Common.CommandTrees.ExpressionBuilder; using System.Data.Common.EntitySql; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Collections.Generic; using System.Linq; namespace System.Data.Mapping.ViewGeneration.Utils { // This class encapsulates "external" calls from view/UDF generation // to other System.Data.Entity features internal static class ExternalCalls { static internal bool IsReservedKeyword(string name) { return CqlLexer.IsReservedKeyword(name); } static internal DbCommandTree CompileView( string viewDef, StorageMappingItemCollection mappingItemCollection, ParserOptions.CompilationMode compilationMode) { Debug.Assert(!String.IsNullOrEmpty(viewDef), "!String.IsNullOrEmpty(viewDef)"); Debug.Assert(mappingItemCollection != null, "mappingItemCollection != null"); Debug.Assert(mappingItemCollection.EdmItemCollection != null, "mappingItemCollection.EdmItemCollection != null"); Debug.Assert(mappingItemCollection.StoreItemCollection != null, "mappingItemCollection.StoreItemCollection != null"); MetadataWorkspace workspace = new MetadataWorkspace(); workspace.RegisterItemCollection(mappingItemCollection.EdmItemCollection); workspace.RegisterItemCollection(mappingItemCollection.StoreItemCollection); workspace.RegisterItemCollection(mappingItemCollection); Perspective perspective = new TargetPerspective(workspace); ParserOptions parserOptions = new ParserOptions(); parserOptions.ParserCompilationMode = compilationMode; DbCommandTree expr = CqlQuery.Compile(viewDef, perspective, parserOptions, null); Debug.Assert(expr != null, "Compile returned empty tree?"); return expr; } ////// Compiles eSQL static internal DbLambda CompileFunctionDefinition( string functionFullName, string functionDefinition, IListand returns . /// Guarantees type match of lambda variables and . /// Passes thru all excepions coming from . /// functionParameters, EdmItemCollection edmItemCollection) { Debug.Assert(!String.IsNullOrEmpty(functionFullName), "!String.IsNullOrEmpty(functionFullName)"); Debug.Assert(!String.IsNullOrEmpty(functionDefinition), "!String.IsNullOrEmpty(functionDefinition)"); Debug.Assert(functionParameters != null, "functionParameters != null"); Debug.Assert(edmItemCollection != null, "edmItemCollection != null"); MetadataWorkspace workspace = new MetadataWorkspace(); workspace.RegisterItemCollection(edmItemCollection); Perspective perspective = new ModelPerspective(workspace); // Since we compile lambda expression and generate variables from the function parameter definitions, // the returned DbLambda will contain variable types that match function parameter types. DbLambda functionBody = CqlQuery.CompileQueryCommandLambda( functionDefinition, perspective, null /* use default parser options */, null /* parameters */, functionParameters.Select(pInfo => pInfo.TypeUsage.Variable(pInfo.Name))); Debug.Assert(functionBody != null, "functionBody != null"); return functionBody; } static internal ItemCollection GetItemCollection(MetadataWorkspace workspace, DataSpace space) { return workspace.GetItemCollection(space); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
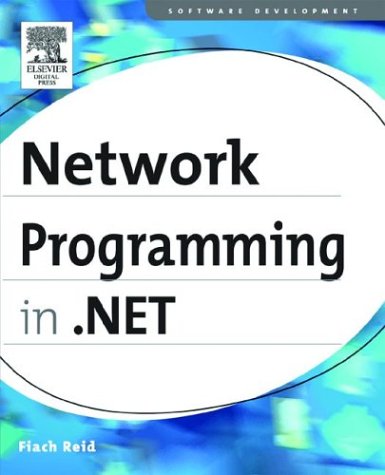
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OdbcEnvironment.cs
- SemaphoreSecurity.cs
- XmlSecureResolver.cs
- TreeViewDesigner.cs
- ServiceSecurityContext.cs
- JsonUriDataContract.cs
- CriticalFinalizerObject.cs
- ObjectViewListener.cs
- WSFederationHttpBinding.cs
- LogLogRecord.cs
- DataGridViewCellStyleConverter.cs
- ChineseLunisolarCalendar.cs
- EndpointDiscoveryMetadata11.cs
- FileDialog_Vista_Interop.cs
- Validator.cs
- PropertyStore.cs
- COM2ExtendedUITypeEditor.cs
- Semaphore.cs
- ScrollItemProviderWrapper.cs
- NetMsmqSecurityMode.cs
- XamlWrapperReaders.cs
- DecoratedNameAttribute.cs
- ParameterCollection.cs
- SpellCheck.cs
- XmlSchemaDatatype.cs
- CancellationTokenRegistration.cs
- SuppressMessageAttribute.cs
- TablePatternIdentifiers.cs
- ListSourceHelper.cs
- DispatcherExceptionFilterEventArgs.cs
- Panel.cs
- BlurEffect.cs
- DependencyPropertyDescriptor.cs
- TableCellAutomationPeer.cs
- ReadWriteSpinLock.cs
- WebPartUserCapability.cs
- ContentDisposition.cs
- ListViewItemSelectionChangedEvent.cs
- EDesignUtil.cs
- RelationshipNavigation.cs
- FixedSOMElement.cs
- IList.cs
- WebPartConnectionsCancelEventArgs.cs
- PartDesigner.cs
- AssociationTypeEmitter.cs
- dbenumerator.cs
- VarRemapper.cs
- PositiveTimeSpanValidator.cs
- ObjectSet.cs
- XmlSerializationGeneratedCode.cs
- TextTreeInsertUndoUnit.cs
- SqlReorderer.cs
- TrustDriver.cs
- NamedPermissionSet.cs
- LinkClickEvent.cs
- ProgressiveCrcCalculatingStream.cs
- ApplicationProxyInternal.cs
- ConfigPathUtility.cs
- VideoDrawing.cs
- CompiledRegexRunner.cs
- FontConverter.cs
- TextBoxDesigner.cs
- Ref.cs
- HTMLTextWriter.cs
- complextypematerializer.cs
- CompressionTransform.cs
- DbSourceParameterCollection.cs
- PlatformNotSupportedException.cs
- NotImplementedException.cs
- DataTableClearEvent.cs
- ScriptingWebServicesSectionGroup.cs
- Debug.cs
- PageAdapter.cs
- HierarchicalDataBoundControl.cs
- EntityDataSourceValidationException.cs
- DateTimePicker.cs
- InputMethodStateTypeInfo.cs
- MultiPageTextView.cs
- UIElementPropertyUndoUnit.cs
- SafeReversePInvokeHandle.cs
- SeekStoryboard.cs
- XmlSchemaCollection.cs
- MSAAEventDispatcher.cs
- CallbackHandler.cs
- DecimalConstantAttribute.cs
- WebPartHelpVerb.cs
- Vertex.cs
- OpenFileDialog.cs
- BooleanConverter.cs
- AppSettingsExpressionBuilder.cs
- CollectionViewSource.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- JobDuplex.cs
- XslTransform.cs
- SymbolMethod.cs
- CellRelation.cs
- FunctionUpdateCommand.cs
- BevelBitmapEffect.cs
- KeyTimeConverter.cs
- TemplateBuilder.cs