Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / MS / Internal / IO / Packaging / CorePropertiesFilter.cs / 1 / CorePropertiesFilter.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implements indexing filters for core properties. // Invoked by PackageFilter and EncryptedPackageFilter // for filtering core properties in Package and // EncryptedPackageEnvelope respectively. // // History: // 07/18/2005: ArindamB: Initial implementation //--------------------------------------------------------------------------- using System; using System.Windows; using System.IO.Packaging; using MS.Internal.Interop; using System.Runtime.InteropServices; using System.Globalization; namespace MS.Internal.IO.Packaging { #region CorePropertiesFilter ////// Class for indexing filters for core properties. /// Implements IManagedFilter to extract property chunks and values /// from CoreProperties. /// internal class CorePropertiesFilter : IManagedFilter { #region Nested types ////// Represents a property chunk. /// private class PropertyChunk : ManagedChunk { internal PropertyChunk(uint chunkId, Guid guid, uint propId) : base( chunkId, CHUNK_BREAKTYPE.CHUNK_EOS, new ManagedFullPropSpec(guid, propId), (uint)CultureInfo.InvariantCulture.LCID, CHUNKSTATE.CHUNK_VALUE ) { } } #endregion Nested types #region Constructor ////// Constructor. /// /// core properties to filter internal CorePropertiesFilter(PackageProperties coreProperties) { if (coreProperties == null) { throw new ArgumentNullException("coreProperties"); } _coreProperties = coreProperties; } #endregion Constructor #region IManagedFilter methods ////// Initialzes the session for this filter. /// /// usage flags /// array of Managed FULLPROPSPEC structs to restrict responses ///IFILTER_FLAGS_NONE. Return value is effectively ignored by the caller. public IFILTER_FLAGS Init(IFILTER_INIT grfFlags, ManagedFullPropSpec[] aAttributes) { // NOTE: Methods parameters have already been validated by XpsFilter. _grfFlags = grfFlags; _aAttributes = aAttributes; // Each call to Init() creates a new enumerator // with parameters corresponding to current Init() call. _corePropertyEnumerator = new CorePropertyEnumerator( _coreProperties, _grfFlags, _aAttributes); return IFILTER_FLAGS.IFILTER_FLAGS_NONE; } ////// Returns description of the next chunk. /// ///Chunk descriptor if there is a next chunk, else null. public ManagedChunk GetChunk() { // No GetValue() call pending from this point on. _pendingGetValue = false; // // Move to the next core property that exists and has a value // and create a chunk descriptor out of it. // if (!CorePropertyEnumerator.MoveNext()) { // End of chunks. return null; } ManagedChunk chunk = new PropertyChunk( AllocateChunkID(), CorePropertyEnumerator.CurrentGuid, CorePropertyEnumerator.CurrentPropId ); // GetValue() call pending from this point on // for the current GetChunk call. _pendingGetValue = true; return chunk; } ////// Gets text content corresponding to current chunk. /// /// ////// Not supported in indexing of core properties. public string GetText(int bufferCharacterCount) { throw new COMException(SR.Get(SRID.FilterGetTextNotSupported), (int)FilterErrorCode.FILTER_E_NO_TEXT); } ////// Gets the property value corresponding to current chunk. /// ///Property value public object GetValue() { // If GetValue() is already called for current chunk, // return error with FILTER_E_NO_MORE_VALUES. if (!_pendingGetValue) { throw new COMException(SR.Get(SRID.FilterGetValueAlreadyCalledOnCurrentChunk), (int)FilterErrorCode.FILTER_E_NO_MORE_VALUES); } // No GetValue() call pending from this point on // until another call to GetChunk() is made successfully. _pendingGetValue = false; return CorePropertyEnumerator.CurrentValue; } #endregion IManagedFilter methods #region Private methods ////// Generates unique and legal chunk ID. /// To be called prior to returning a chunk. /// ////// 0 is an illegal value, so this function never returns 0. /// After the counter reaches UInt32.MaxValue, it wraps around to 1. /// private uint AllocateChunkID() { if (_chunkID == UInt32.MaxValue) { _chunkID = 1; } else { _chunkID++; } return _chunkID; } #endregion #region Properties ////// Enumerates core properties based on the /// core properties and parameters passed in to Init() call. /// private CorePropertyEnumerator CorePropertyEnumerator { get { if (_corePropertyEnumerator == null) { _corePropertyEnumerator = new CorePropertyEnumerator( _coreProperties, _grfFlags, _aAttributes); } return _corePropertyEnumerator; } } #endregion Properties #region Fields ////// IFilter.Init parameters. /// Used to initialize CorePropertyEnumerator. /// IFILTER_INIT _grfFlags = 0; ManagedFullPropSpec[] _aAttributes = null; ////// Chunk ID for the current chunk. Incremented for /// every next chunk. /// private uint _chunkID = 0; ////// Indicates if GetValue() call is pending /// for the current chunk queried using GetChunk(). /// If not, GetValue() returns FILTER_E_NO_MORE_VALUES. /// private bool _pendingGetValue = false; ////// Enumerator used to iterate over the /// core properties and create chunk out of them. /// private CorePropertyEnumerator _corePropertyEnumerator = null; ////// Core properties being filtered. /// Could be PackageCoreProperties or EncryptedPackageCoreProperties. /// private PackageProperties _coreProperties = null; #endregion Fields } #endregion CorePropertiesFilter #region CorePropertyEnumerator ////// Enumerator for CoreProperties. Used to iterate through the /// properties and obtain their property set GUID, property ID and value. /// internal class CorePropertyEnumerator { #region Constructors ////// Constructor. /// /// CoreProperties to enumerate /// /// if IFILTER_INIT_APPLY_INDEX_ATTRIBUTES is specified, /// this indicates all core properties to be returned unless /// the parameter aAttributes is non-empty. /// /// /// attributes specified corresponding to the properties to filter. /// internal CorePropertyEnumerator(PackageProperties coreProperties, IFILTER_INIT grfFlags, ManagedFullPropSpec[] attributes) { if (attributes != null && attributes.Length > 0) { // // If attruibutes list specified, // return core properties for only those attributes. // _attributes = attributes; } else if ((grfFlags & IFILTER_INIT.IFILTER_INIT_APPLY_INDEX_ATTRIBUTES) == IFILTER_INIT.IFILTER_INIT_APPLY_INDEX_ATTRIBUTES) { // // If no attributes list specified, // but IFILTER_INIT_APPLY_INDEX_ATTRIBUTES is present in grfFlags, // return all core properties. // _attributes = new ManagedFullPropSpec[] { // // SummaryInformation // new ManagedFullPropSpec(FormatId.SummaryInformation, PropertyId.Title), new ManagedFullPropSpec(FormatId.SummaryInformation, PropertyId.Subject), new ManagedFullPropSpec(FormatId.SummaryInformation, PropertyId.Creator), new ManagedFullPropSpec(FormatId.SummaryInformation, PropertyId.Keywords), new ManagedFullPropSpec(FormatId.SummaryInformation, PropertyId.Description), new ManagedFullPropSpec(FormatId.SummaryInformation, PropertyId.LastModifiedBy), new ManagedFullPropSpec(FormatId.SummaryInformation, PropertyId.Revision), new ManagedFullPropSpec(FormatId.SummaryInformation, PropertyId.LastPrinted), new ManagedFullPropSpec(FormatId.SummaryInformation, PropertyId.DateCreated), new ManagedFullPropSpec(FormatId.SummaryInformation, PropertyId.DateModified), // // DocumentSummaryInformation // new ManagedFullPropSpec(FormatId.DocumentSummaryInformation, PropertyId.Category), new ManagedFullPropSpec(FormatId.DocumentSummaryInformation, PropertyId.Identifier), new ManagedFullPropSpec(FormatId.DocumentSummaryInformation, PropertyId.ContentType), new ManagedFullPropSpec(FormatId.DocumentSummaryInformation, PropertyId.Language), new ManagedFullPropSpec(FormatId.DocumentSummaryInformation, PropertyId.Version), new ManagedFullPropSpec(FormatId.DocumentSummaryInformation, PropertyId.ContentStatus) }; } else { // No core properties to be returned. } _coreProperties = coreProperties; _currentIndex = -1; } #endregion Constructors #region Internal methods ////// Move to next property in the enumeration /// which exists and has a value. /// ///internal bool MoveNext() { if (_attributes == null) { return false; } _currentIndex++; // Move to next existing property with value present. while (_currentIndex < _attributes.Length) { if ( _attributes[_currentIndex].Property.PropType == PropSpecType.Id && CurrentValue != null) { return true; } _currentIndex++; } // End of properties. return false; } #endregion Internal methods #region Internal properties /// /// Property set GUID for current propery. /// internal Guid CurrentGuid { get { ValidateCurrent(); return _attributes[_currentIndex].Guid; } } ////// Property ID for current property. /// internal uint CurrentPropId { get { ValidateCurrent(); return _attributes[_currentIndex].Property.PropId; } } ////// Value for current property. /// internal object CurrentValue { get { ValidateCurrent(); return GetValue(CurrentGuid, CurrentPropId); } } #endregion Internal properties #region Private methods ////// Check if the current entry in enumeration is valid. /// private void ValidateCurrent() { if (_currentIndex < 0 || _currentIndex >= _attributes.Length) { throw new InvalidOperationException( SR.Get(SRID.CorePropertyEnumeratorPositionedOutOfBounds)); } } #endregion #region Private properties ////// Get value for the property corresponding to the /// property set GUID and property ID. /// /// property set GUID /// property ID ////// property value which could be string or DateTime, /// or null if it doesn't exist. /// private object GetValue(Guid guid, uint propId) { if (guid == FormatId.SummaryInformation) { switch (propId) { case PropertyId.Title: return _coreProperties.Title; case PropertyId.Subject: return _coreProperties.Subject; case PropertyId.Creator: return _coreProperties.Creator; case PropertyId.Keywords: return _coreProperties.Keywords; case PropertyId.Description: return _coreProperties.Description; case PropertyId.LastModifiedBy: return _coreProperties.LastModifiedBy; case PropertyId.Revision: return _coreProperties.Revision; case PropertyId.LastPrinted: if (_coreProperties.LastPrinted != null) { return _coreProperties.LastPrinted.Value; } return null; case PropertyId.DateCreated: if (_coreProperties.Created != null) { return _coreProperties.Created.Value; } return null; case PropertyId.DateModified: if (_coreProperties.Modified != null) { return _coreProperties.Modified.Value; } return null; } } else if (guid == FormatId.DocumentSummaryInformation) { switch (propId) { case PropertyId.Category: return _coreProperties.Category; case PropertyId.Identifier: return _coreProperties.Identifier; case PropertyId.ContentType: return _coreProperties.ContentType; case PropertyId.Language: return _coreProperties.Language; case PropertyId.Version: return _coreProperties.Version; case PropertyId.ContentStatus: return _coreProperties.ContentStatus; } } // Property/value not found. return null; } #endregion Private properties #region Fields ////// Reference to the CorePropeties to enumerate. /// private PackageProperties _coreProperties; ////// Indicates the list of properties to be enumerated. /// private ManagedFullPropSpec[] _attributes = null; ////// Index of the current property in enumeration. /// private int _currentIndex; #endregion Fields } #endregion CorePropertyEnumerator } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implements indexing filters for core properties. // Invoked by PackageFilter and EncryptedPackageFilter // for filtering core properties in Package and // EncryptedPackageEnvelope respectively. // // History: // 07/18/2005: ArindamB: Initial implementation //--------------------------------------------------------------------------- using System; using System.Windows; using System.IO.Packaging; using MS.Internal.Interop; using System.Runtime.InteropServices; using System.Globalization; namespace MS.Internal.IO.Packaging { #region CorePropertiesFilter ////// Class for indexing filters for core properties. /// Implements IManagedFilter to extract property chunks and values /// from CoreProperties. /// internal class CorePropertiesFilter : IManagedFilter { #region Nested types ////// Represents a property chunk. /// private class PropertyChunk : ManagedChunk { internal PropertyChunk(uint chunkId, Guid guid, uint propId) : base( chunkId, CHUNK_BREAKTYPE.CHUNK_EOS, new ManagedFullPropSpec(guid, propId), (uint)CultureInfo.InvariantCulture.LCID, CHUNKSTATE.CHUNK_VALUE ) { } } #endregion Nested types #region Constructor ////// Constructor. /// /// core properties to filter internal CorePropertiesFilter(PackageProperties coreProperties) { if (coreProperties == null) { throw new ArgumentNullException("coreProperties"); } _coreProperties = coreProperties; } #endregion Constructor #region IManagedFilter methods ////// Initialzes the session for this filter. /// /// usage flags /// array of Managed FULLPROPSPEC structs to restrict responses ///IFILTER_FLAGS_NONE. Return value is effectively ignored by the caller. public IFILTER_FLAGS Init(IFILTER_INIT grfFlags, ManagedFullPropSpec[] aAttributes) { // NOTE: Methods parameters have already been validated by XpsFilter. _grfFlags = grfFlags; _aAttributes = aAttributes; // Each call to Init() creates a new enumerator // with parameters corresponding to current Init() call. _corePropertyEnumerator = new CorePropertyEnumerator( _coreProperties, _grfFlags, _aAttributes); return IFILTER_FLAGS.IFILTER_FLAGS_NONE; } ////// Returns description of the next chunk. /// ///Chunk descriptor if there is a next chunk, else null. public ManagedChunk GetChunk() { // No GetValue() call pending from this point on. _pendingGetValue = false; // // Move to the next core property that exists and has a value // and create a chunk descriptor out of it. // if (!CorePropertyEnumerator.MoveNext()) { // End of chunks. return null; } ManagedChunk chunk = new PropertyChunk( AllocateChunkID(), CorePropertyEnumerator.CurrentGuid, CorePropertyEnumerator.CurrentPropId ); // GetValue() call pending from this point on // for the current GetChunk call. _pendingGetValue = true; return chunk; } ////// Gets text content corresponding to current chunk. /// /// ////// Not supported in indexing of core properties. public string GetText(int bufferCharacterCount) { throw new COMException(SR.Get(SRID.FilterGetTextNotSupported), (int)FilterErrorCode.FILTER_E_NO_TEXT); } ////// Gets the property value corresponding to current chunk. /// ///Property value public object GetValue() { // If GetValue() is already called for current chunk, // return error with FILTER_E_NO_MORE_VALUES. if (!_pendingGetValue) { throw new COMException(SR.Get(SRID.FilterGetValueAlreadyCalledOnCurrentChunk), (int)FilterErrorCode.FILTER_E_NO_MORE_VALUES); } // No GetValue() call pending from this point on // until another call to GetChunk() is made successfully. _pendingGetValue = false; return CorePropertyEnumerator.CurrentValue; } #endregion IManagedFilter methods #region Private methods ////// Generates unique and legal chunk ID. /// To be called prior to returning a chunk. /// ////// 0 is an illegal value, so this function never returns 0. /// After the counter reaches UInt32.MaxValue, it wraps around to 1. /// private uint AllocateChunkID() { if (_chunkID == UInt32.MaxValue) { _chunkID = 1; } else { _chunkID++; } return _chunkID; } #endregion #region Properties ////// Enumerates core properties based on the /// core properties and parameters passed in to Init() call. /// private CorePropertyEnumerator CorePropertyEnumerator { get { if (_corePropertyEnumerator == null) { _corePropertyEnumerator = new CorePropertyEnumerator( _coreProperties, _grfFlags, _aAttributes); } return _corePropertyEnumerator; } } #endregion Properties #region Fields ////// IFilter.Init parameters. /// Used to initialize CorePropertyEnumerator. /// IFILTER_INIT _grfFlags = 0; ManagedFullPropSpec[] _aAttributes = null; ////// Chunk ID for the current chunk. Incremented for /// every next chunk. /// private uint _chunkID = 0; ////// Indicates if GetValue() call is pending /// for the current chunk queried using GetChunk(). /// If not, GetValue() returns FILTER_E_NO_MORE_VALUES. /// private bool _pendingGetValue = false; ////// Enumerator used to iterate over the /// core properties and create chunk out of them. /// private CorePropertyEnumerator _corePropertyEnumerator = null; ////// Core properties being filtered. /// Could be PackageCoreProperties or EncryptedPackageCoreProperties. /// private PackageProperties _coreProperties = null; #endregion Fields } #endregion CorePropertiesFilter #region CorePropertyEnumerator ////// Enumerator for CoreProperties. Used to iterate through the /// properties and obtain their property set GUID, property ID and value. /// internal class CorePropertyEnumerator { #region Constructors ////// Constructor. /// /// CoreProperties to enumerate /// /// if IFILTER_INIT_APPLY_INDEX_ATTRIBUTES is specified, /// this indicates all core properties to be returned unless /// the parameter aAttributes is non-empty. /// /// /// attributes specified corresponding to the properties to filter. /// internal CorePropertyEnumerator(PackageProperties coreProperties, IFILTER_INIT grfFlags, ManagedFullPropSpec[] attributes) { if (attributes != null && attributes.Length > 0) { // // If attruibutes list specified, // return core properties for only those attributes. // _attributes = attributes; } else if ((grfFlags & IFILTER_INIT.IFILTER_INIT_APPLY_INDEX_ATTRIBUTES) == IFILTER_INIT.IFILTER_INIT_APPLY_INDEX_ATTRIBUTES) { // // If no attributes list specified, // but IFILTER_INIT_APPLY_INDEX_ATTRIBUTES is present in grfFlags, // return all core properties. // _attributes = new ManagedFullPropSpec[] { // // SummaryInformation // new ManagedFullPropSpec(FormatId.SummaryInformation, PropertyId.Title), new ManagedFullPropSpec(FormatId.SummaryInformation, PropertyId.Subject), new ManagedFullPropSpec(FormatId.SummaryInformation, PropertyId.Creator), new ManagedFullPropSpec(FormatId.SummaryInformation, PropertyId.Keywords), new ManagedFullPropSpec(FormatId.SummaryInformation, PropertyId.Description), new ManagedFullPropSpec(FormatId.SummaryInformation, PropertyId.LastModifiedBy), new ManagedFullPropSpec(FormatId.SummaryInformation, PropertyId.Revision), new ManagedFullPropSpec(FormatId.SummaryInformation, PropertyId.LastPrinted), new ManagedFullPropSpec(FormatId.SummaryInformation, PropertyId.DateCreated), new ManagedFullPropSpec(FormatId.SummaryInformation, PropertyId.DateModified), // // DocumentSummaryInformation // new ManagedFullPropSpec(FormatId.DocumentSummaryInformation, PropertyId.Category), new ManagedFullPropSpec(FormatId.DocumentSummaryInformation, PropertyId.Identifier), new ManagedFullPropSpec(FormatId.DocumentSummaryInformation, PropertyId.ContentType), new ManagedFullPropSpec(FormatId.DocumentSummaryInformation, PropertyId.Language), new ManagedFullPropSpec(FormatId.DocumentSummaryInformation, PropertyId.Version), new ManagedFullPropSpec(FormatId.DocumentSummaryInformation, PropertyId.ContentStatus) }; } else { // No core properties to be returned. } _coreProperties = coreProperties; _currentIndex = -1; } #endregion Constructors #region Internal methods ////// Move to next property in the enumeration /// which exists and has a value. /// ///internal bool MoveNext() { if (_attributes == null) { return false; } _currentIndex++; // Move to next existing property with value present. while (_currentIndex < _attributes.Length) { if ( _attributes[_currentIndex].Property.PropType == PropSpecType.Id && CurrentValue != null) { return true; } _currentIndex++; } // End of properties. return false; } #endregion Internal methods #region Internal properties /// /// Property set GUID for current propery. /// internal Guid CurrentGuid { get { ValidateCurrent(); return _attributes[_currentIndex].Guid; } } ////// Property ID for current property. /// internal uint CurrentPropId { get { ValidateCurrent(); return _attributes[_currentIndex].Property.PropId; } } ////// Value for current property. /// internal object CurrentValue { get { ValidateCurrent(); return GetValue(CurrentGuid, CurrentPropId); } } #endregion Internal properties #region Private methods ////// Check if the current entry in enumeration is valid. /// private void ValidateCurrent() { if (_currentIndex < 0 || _currentIndex >= _attributes.Length) { throw new InvalidOperationException( SR.Get(SRID.CorePropertyEnumeratorPositionedOutOfBounds)); } } #endregion #region Private properties ////// Get value for the property corresponding to the /// property set GUID and property ID. /// /// property set GUID /// property ID ////// property value which could be string or DateTime, /// or null if it doesn't exist. /// private object GetValue(Guid guid, uint propId) { if (guid == FormatId.SummaryInformation) { switch (propId) { case PropertyId.Title: return _coreProperties.Title; case PropertyId.Subject: return _coreProperties.Subject; case PropertyId.Creator: return _coreProperties.Creator; case PropertyId.Keywords: return _coreProperties.Keywords; case PropertyId.Description: return _coreProperties.Description; case PropertyId.LastModifiedBy: return _coreProperties.LastModifiedBy; case PropertyId.Revision: return _coreProperties.Revision; case PropertyId.LastPrinted: if (_coreProperties.LastPrinted != null) { return _coreProperties.LastPrinted.Value; } return null; case PropertyId.DateCreated: if (_coreProperties.Created != null) { return _coreProperties.Created.Value; } return null; case PropertyId.DateModified: if (_coreProperties.Modified != null) { return _coreProperties.Modified.Value; } return null; } } else if (guid == FormatId.DocumentSummaryInformation) { switch (propId) { case PropertyId.Category: return _coreProperties.Category; case PropertyId.Identifier: return _coreProperties.Identifier; case PropertyId.ContentType: return _coreProperties.ContentType; case PropertyId.Language: return _coreProperties.Language; case PropertyId.Version: return _coreProperties.Version; case PropertyId.ContentStatus: return _coreProperties.ContentStatus; } } // Property/value not found. return null; } #endregion Private properties #region Fields ////// Reference to the CorePropeties to enumerate. /// private PackageProperties _coreProperties; ////// Indicates the list of properties to be enumerated. /// private ManagedFullPropSpec[] _attributes = null; ////// Index of the current property in enumeration. /// private int _currentIndex; #endregion Fields } #endregion CorePropertyEnumerator } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
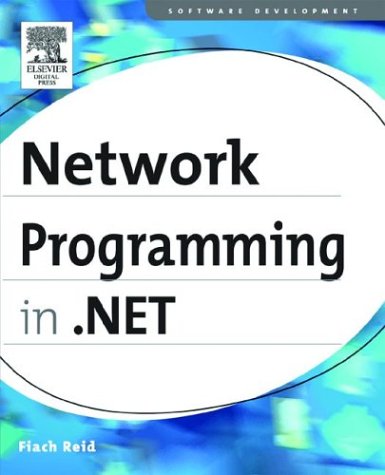
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UInt64.cs
- ExpressionReplacer.cs
- DoubleLinkListEnumerator.cs
- MeshGeometry3D.cs
- SiteMapHierarchicalDataSourceView.cs
- Models.cs
- InputGestureCollection.cs
- LongCountAggregationOperator.cs
- PagerSettings.cs
- PageContentCollection.cs
- SqlRewriteScalarSubqueries.cs
- StackBuilderSink.cs
- ActivityBindForm.cs
- CommandTreeTypeHelper.cs
- BuildProvidersCompiler.cs
- CategoryGridEntry.cs
- FlatButtonAppearance.cs
- MarshalByValueComponent.cs
- WsatStrings.cs
- Window.cs
- ConfigXmlText.cs
- WebRequestModulesSection.cs
- DeploymentSection.cs
- ErrorTolerantObjectWriter.cs
- WorkflowQueuingService.cs
- AutomationProperty.cs
- AutoGeneratedField.cs
- DynamicResourceExtensionConverter.cs
- GlobalizationAssembly.cs
- TreeView.cs
- BigInt.cs
- ReadOnlyCollectionBuilder.cs
- ResizeGrip.cs
- DirectoryLocalQuery.cs
- XPathBinder.cs
- VSWCFServiceContractGenerator.cs
- SequentialWorkflowRootDesigner.cs
- TabletCollection.cs
- TextTreeUndoUnit.cs
- PrintDocument.cs
- EventManager.cs
- ClassicBorderDecorator.cs
- CharStorage.cs
- InkCanvasFeedbackAdorner.cs
- ScriptResourceInfo.cs
- Transform.cs
- ListViewItemCollectionEditor.cs
- ImageBrush.cs
- DeviceFilterEditorDialog.cs
- ValidatorCollection.cs
- DrawListViewSubItemEventArgs.cs
- DelayedRegex.cs
- SHA512CryptoServiceProvider.cs
- ToolboxItemCollection.cs
- XmlHierarchyData.cs
- BitmapEffectvisualstate.cs
- ComProxy.cs
- ProxyElement.cs
- DataGridViewColumnConverter.cs
- CookieHandler.cs
- HttpListenerContext.cs
- StatusBarPanelClickEvent.cs
- GreaterThan.cs
- ActiveDocumentEvent.cs
- DataGridViewCellEventArgs.cs
- SyntaxCheck.cs
- Events.cs
- TraceUtility.cs
- SamlAuthorityBinding.cs
- Pts.cs
- PositiveTimeSpanValidator.cs
- TreeView.cs
- Point3DConverter.cs
- AutoGeneratedField.cs
- RadioButtonAutomationPeer.cs
- StateDesigner.TransitionInfo.cs
- Select.cs
- DataGridBoolColumn.cs
- RemotingException.cs
- TextProperties.cs
- CodeTypeParameterCollection.cs
- GZipObjectSerializer.cs
- SchemaMapping.cs
- HwndKeyboardInputProvider.cs
- UpdateInfo.cs
- DbMetaDataFactory.cs
- ServerValidateEventArgs.cs
- SurrogateSelector.cs
- CodeParameterDeclarationExpression.cs
- ControlBuilder.cs
- DataGridViewRowCancelEventArgs.cs
- DropDownList.cs
- EventHandlers.cs
- DataGridViewCellStyle.cs
- AuthenticationModuleElement.cs
- RowBinding.cs
- basecomparevalidator.cs
- MetadataArtifactLoaderCompositeResource.cs
- ObjectSet.cs
- KoreanLunisolarCalendar.cs