Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / ImageBrush.cs / 1 / ImageBrush.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: This file contains the implementation of ImageBrush. // The ImageBrush is a TileBrush which defines its tile content // by use of an ImageSource. // // History: // // 04/29/2003 : adsmith - Created it. // 01/19/2005 : timothyc - Removed SizeViewboxToContent. Moved UpdateResource // to the generated file. //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PresentationCore; using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// ImageBrush - This TileBrush defines its content as an Image /// public sealed partial class ImageBrush : TileBrush { #region Constructors ////// Default constructor for ImageBrush. The resulting Brush has no content. /// public ImageBrush() { // We do this so that the property, when read, is consistent - not that // this will every actually affect drawing. } ////// ImageBrush Constructor where the image is set to the parameter's value /// /// The image source. public ImageBrush(ImageSource image) { ImageSource = image; } #endregion Constructors #region Protected methods ////// This node can introduce graphness /// internal override bool CanIntroduceGraphness() { if (ImageSource == null) { // If we don't have an ImageSource, we can't introduce // graphness right now, if we get an image source later // we'll precompute again. return false; } else { if (ImageSource.CanIntroduceGraphness()) { return true; } else { return false; } } } ////// Obtains the current bounds of the brush's content /// /// Output bounds of content protected override void GetContentBounds(out Rect contentBounds) { // Note, only implemented for DrawingImages. contentBounds = Rect.Empty; DrawingImage di = ImageSource as DrawingImage; if (di != null) { Drawing drawing = di.Drawing; if (drawing != null) { contentBounds = drawing.Bounds; } } } #endregion Protected methods #region Realization Support ////// Precompute is called during the frame preparation phase. Derived classes /// typically check if the brush requires realizations during this phase. /// internal override void Precompute() { ImageSource imageSource = ImageSource; if (imageSource != null) { imageSource.Precompute(); _requiresRealizationUpdates = imageSource.RequiresRealizationUpdates; } else { _requiresRealizationUpdates = false; } } ////// Checks if realization updates are required for this resource. /// internal override bool RequiresRealizationUpdates { get { return _requiresRealizationUpdates; } } ////// Derived classes must override this method and update realizations on dependent /// resources if required. /// internal override void UpdateRealizations(Rect fillShapeBounds, RealizationContext ctx) { if (_requiresRealizationUpdates) { Matrix m; ImageSource imageSource = ImageSource; Debug.Assert(imageSource != null); // Otherwise _requiresRealizationUpdates would be false. GetTileBrushMapping(fillShapeBounds, out m); ctx.TransformStack.Push(ref m, true); imageSource.UpdateRealizations(ctx); ctx.TransformStack.Pop(); } } #endregion private bool _requiresRealizationUpdates; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: This file contains the implementation of ImageBrush. // The ImageBrush is a TileBrush which defines its tile content // by use of an ImageSource. // // History: // // 04/29/2003 : adsmith - Created it. // 01/19/2005 : timothyc - Removed SizeViewboxToContent. Moved UpdateResource // to the generated file. //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PresentationCore; using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// ImageBrush - This TileBrush defines its content as an Image /// public sealed partial class ImageBrush : TileBrush { #region Constructors ////// Default constructor for ImageBrush. The resulting Brush has no content. /// public ImageBrush() { // We do this so that the property, when read, is consistent - not that // this will every actually affect drawing. } ////// ImageBrush Constructor where the image is set to the parameter's value /// /// The image source. public ImageBrush(ImageSource image) { ImageSource = image; } #endregion Constructors #region Protected methods ////// This node can introduce graphness /// internal override bool CanIntroduceGraphness() { if (ImageSource == null) { // If we don't have an ImageSource, we can't introduce // graphness right now, if we get an image source later // we'll precompute again. return false; } else { if (ImageSource.CanIntroduceGraphness()) { return true; } else { return false; } } } ////// Obtains the current bounds of the brush's content /// /// Output bounds of content protected override void GetContentBounds(out Rect contentBounds) { // Note, only implemented for DrawingImages. contentBounds = Rect.Empty; DrawingImage di = ImageSource as DrawingImage; if (di != null) { Drawing drawing = di.Drawing; if (drawing != null) { contentBounds = drawing.Bounds; } } } #endregion Protected methods #region Realization Support ////// Precompute is called during the frame preparation phase. Derived classes /// typically check if the brush requires realizations during this phase. /// internal override void Precompute() { ImageSource imageSource = ImageSource; if (imageSource != null) { imageSource.Precompute(); _requiresRealizationUpdates = imageSource.RequiresRealizationUpdates; } else { _requiresRealizationUpdates = false; } } ////// Checks if realization updates are required for this resource. /// internal override bool RequiresRealizationUpdates { get { return _requiresRealizationUpdates; } } ////// Derived classes must override this method and update realizations on dependent /// resources if required. /// internal override void UpdateRealizations(Rect fillShapeBounds, RealizationContext ctx) { if (_requiresRealizationUpdates) { Matrix m; ImageSource imageSource = ImageSource; Debug.Assert(imageSource != null); // Otherwise _requiresRealizationUpdates would be false. GetTileBrushMapping(fillShapeBounds, out m); ctx.TransformStack.Push(ref m, true); imageSource.UpdateRealizations(ctx); ctx.TransformStack.Pop(); } } #endregion private bool _requiresRealizationUpdates; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
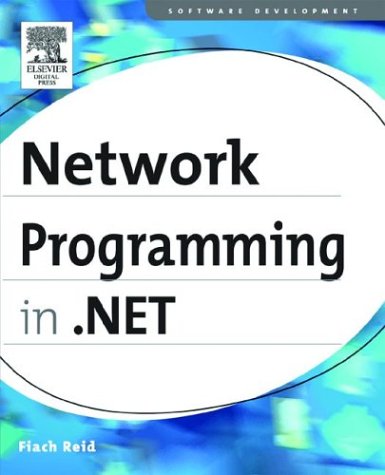
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Buffer.cs
- QilStrConcat.cs
- XmlAnyAttributeAttribute.cs
- RegexCode.cs
- Roles.cs
- TemplateField.cs
- GraphicsPathIterator.cs
- BadImageFormatException.cs
- SqlCacheDependencySection.cs
- EditorPartCollection.cs
- HttpConfigurationContext.cs
- ThaiBuddhistCalendar.cs
- AmbientLight.cs
- AssertSection.cs
- ErrorWrapper.cs
- SHA512Cng.cs
- XmlStreamNodeWriter.cs
- RegexParser.cs
- InkCanvasFeedbackAdorner.cs
- StringPropertyBuilder.cs
- IPPacketInformation.cs
- FieldNameLookup.cs
- SizeChangedInfo.cs
- TableRowCollection.cs
- CacheDependency.cs
- BinaryConverter.cs
- Request.cs
- HtmlControl.cs
- Win32PrintDialog.cs
- RequestContextBase.cs
- DateTimePicker.cs
- BypassElement.cs
- SQLDecimalStorage.cs
- AllMembershipCondition.cs
- ValidationSummary.cs
- DataGridViewRowConverter.cs
- GeometryHitTestResult.cs
- TcpServerChannel.cs
- VersionUtil.cs
- PartialTrustVisibleAssembliesSection.cs
- SortKey.cs
- WindowsIPAddress.cs
- hresults.cs
- TdsEnums.cs
- NumberSubstitution.cs
- GeometryModel3D.cs
- DefaultHttpHandler.cs
- DocumentSchemaValidator.cs
- NamedPermissionSet.cs
- XmlAttributeAttribute.cs
- DataComponentMethodGenerator.cs
- VersionPair.cs
- AuthenticateEventArgs.cs
- MDIWindowDialog.cs
- TableRowCollection.cs
- Help.cs
- IteratorFilter.cs
- XmlSortKeyAccumulator.cs
- precedingsibling.cs
- MimeReturn.cs
- MembershipValidatePasswordEventArgs.cs
- CDSCollectionETWBCLProvider.cs
- InsufficientMemoryException.cs
- XmlSchemaAppInfo.cs
- ContentPathSegment.cs
- MediaCommands.cs
- HttpWriter.cs
- LinqDataSourceDisposeEventArgs.cs
- UriExt.cs
- BindingManagerDataErrorEventArgs.cs
- GeometryCollection.cs
- Inflater.cs
- HyperLink.cs
- DiscoveryClientChannelFactory.cs
- DataGridCaption.cs
- SmiRecordBuffer.cs
- _HeaderInfoTable.cs
- PerfProviderCollection.cs
- ScalarOps.cs
- EntityTemplateFactory.cs
- ListenerElementsCollection.cs
- SqlDataSourceCache.cs
- Int16Storage.cs
- ItemCollection.cs
- Tracking.cs
- UndoEngine.cs
- ServiceBuildProvider.cs
- XmlSchemaValidationException.cs
- XmlStringTable.cs
- FixedSOMTableRow.cs
- ComponentCommands.cs
- TextContainerChangeEventArgs.cs
- OdbcInfoMessageEvent.cs
- JsonQNameDataContract.cs
- ComponentDispatcher.cs
- CheckBoxList.cs
- SendMailErrorEventArgs.cs
- DataTableReaderListener.cs
- ExtendedPropertyDescriptor.cs
- Hex.cs