Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / MIT / System / Web / UI / MobileControls / ValidationSummary.cs / 1305376 / ValidationSummary.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design; using System.Drawing; using System.Globalization; using System.Web; using System.Web.UI; using System.Web.UI.Design.WebControls; using System.Web.UI.HtmlControls; using System.Web.UI.WebControls; using System.Diagnostics; using System.Security.Permissions; namespace System.Web.UI.MobileControls { /* * Mobile ValidationSummary class. * The ValidationSummary shows all the validation errors in a Form in a * summary view. * * Copyright (c) 2000 Microsoft Corporation */ ///[ DefaultProperty("FormToValidate"), Designer(typeof(System.Web.UI.Design.MobileControls.ValidationSummaryDesigner)), DesignerAdapter(typeof(System.Web.UI.Design.MobileControls.Adapters.DesignerValidationSummaryAdapter)), ToolboxData("<{0}:ValidationSummary runat=\"server\">{0}:ValidationSummary>"), ToolboxItem("System.Web.UI.Design.WebControlToolboxItem, " + AssemblyRef.SystemDesign) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] [Obsolete("The System.Web.Mobile.dll assembly has been deprecated and should no longer be used. For information about how to develop ASP.NET mobile applications, see http://go.microsoft.com/fwlink/?LinkId=157231.")] public class ValidationSummary : MobileControl { private bool _callValidate = true; /// public ValidationSummary() { StyleReference = Constants.ErrorStyle; } //////////////////////////////////////////////////////////////////////// // Mimic some properties exposed in the original ValidatorSummary. //////////////////////////////////////////////////////////////////////// /// [ Bindable(true), DefaultValue(""), MobileCategory(SR.Category_Appearance), MobileSysDescription(SR.ValidationSummary_HeaderText) ] public String HeaderText { get { String s = (String) ViewState["HeaderText"]; return((s != null) ? s : String.Empty); } set { ViewState["HeaderText"] = value; } } /// [ Bindable(true), DefaultValue(""), MobileCategory(SR.Category_Behavior), MobileSysDescription(SR.ValidationSummary_FormToValidate), TypeConverter(typeof(System.Web.UI.Design.MobileControls.Converters.FormConverter)) ] public String FormToValidate { get { String s = (String) ViewState["FormToValidate"]; return((s != null) ? s : String.Empty); } set { ViewState["FormToValidate"] = value; } } /// [ Bindable(true), DefaultValue(""), MobileCategory(SR.Category_Appearance), MobileSysDescription(SR.ValidationSummary_BackLabel) ] public String BackLabel { get { return ToString(ViewState["BackLabel"]); } set { ViewState["BackLabel"] = value; } } // Designer needs to know the correct default value in order to persist it correctly. /// [ DefaultValue(Constants.ErrorStyle) ] public override String StyleReference { get { return base.StyleReference; } set { base.StyleReference = value; } } /// protected override void OnLoad(EventArgs e) { base.OnLoad(e); // There are cases that we don't want to call the Validate() // method on individual validators that the ValidationSummary is // targeting to. // This first case is when the page is hit at the first time. // And the second case is when FormToValidate is the same as the // form ValidationSummary is on and this form is a postback from // the same form. In this case, the validators' validate() // method should have been called by MobilePage if needed. if (!MobilePage.IsPostBack || String.Compare(Form.UniqueID, FormToValidate, StringComparison.OrdinalIgnoreCase) == 0) { _callValidate = false; } } private void GetErrorValidators_Helper(Control parent, ArrayList errorValidators) { foreach(Control control in parent.Controls) { BaseValidator baseVal = control as BaseValidator; if (baseVal != null && baseVal.ErrorMessage.Length != 0) { if (_callValidate) { baseVal.Validate(); } if (!baseVal.IsValid) { errorValidators.Add(baseVal); } } GetErrorValidators_Helper(control, errorValidators); } } /// public String[] GetErrorMessages() { String[] errorDescriptions = null; ArrayList errorValidators = new ArrayList(); Form targetForm = ResolveFormReferenceNoThrow(FormToValidate); if (targetForm == null) { throw new ArgumentException( SR.GetString(SR.ValidationSummary_InvalidFormToValidate, FormToValidate, ID)); } // Recursively find all validators with error messages to display. GetErrorValidators_Helper(targetForm, errorValidators); int count = errorValidators.Count; if (count > 0) { // get the messages; errorDescriptions = new String[count]; int iMessage = 0; foreach (BaseValidator val in errorValidators) { Debug.Assert(val != null, "Null reference unexpected!"); Debug.Assert(val.ErrorMessage.Length != 0, "Programmatic error: error message here shouldn't be empty!"); errorDescriptions[iMessage] = String.Copy(val.ErrorMessage); iMessage++; } Debug.Assert(count == iMessage, "Not all messages were found!"); } return errorDescriptions; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
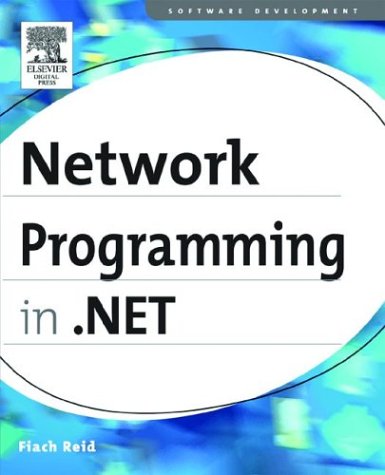
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DeviceContexts.cs
- SingletonConnectionReader.cs
- MsmqAppDomainProtocolHandler.cs
- URLString.cs
- HashRepartitionStream.cs
- GenericPrincipal.cs
- IndentedWriter.cs
- HashHelper.cs
- ExtendedPropertyCollection.cs
- NeutralResourcesLanguageAttribute.cs
- ShapingEngine.cs
- SecureConversationServiceElement.cs
- arabicshape.cs
- UrlMappingsSection.cs
- StringSource.cs
- XamlGridLengthSerializer.cs
- ListViewCancelEventArgs.cs
- VirtualPathUtility.cs
- CodePrimitiveExpression.cs
- Pair.cs
- BoundingRectTracker.cs
- SafeEventLogReadHandle.cs
- SharedRuntimeState.cs
- ColorTransform.cs
- streamingZipPartStream.cs
- PageContentCollection.cs
- formatter.cs
- AssertFilter.cs
- ipaddressinformationcollection.cs
- UnmanagedMemoryStream.cs
- TreeViewImageIndexConverter.cs
- ObjectToken.cs
- ArrayConverter.cs
- XmlSignificantWhitespace.cs
- WmlValidationSummaryAdapter.cs
- ContentType.cs
- GenericUriParser.cs
- StaticExtension.cs
- OneOfScalarConst.cs
- WebPartRestoreVerb.cs
- DocumentReference.cs
- Misc.cs
- RuleInfoComparer.cs
- Vector3DCollection.cs
- CompilerWrapper.cs
- UIAgentAsyncEndRequest.cs
- CodeAssignStatement.cs
- DbConnectionStringBuilder.cs
- Latin1Encoding.cs
- ToolStripSplitStackLayout.cs
- AnimationLayer.cs
- COM2ExtendedBrowsingHandler.cs
- DataGridViewCellCollection.cs
- XmlObjectSerializerWriteContext.cs
- XmlDocument.cs
- Comparer.cs
- FileDataSourceCache.cs
- ControllableStoryboardAction.cs
- ReflectionPermission.cs
- Bitmap.cs
- InputBuffer.cs
- RecognizedWordUnit.cs
- ErrorEventArgs.cs
- CollectionType.cs
- FontNamesConverter.cs
- PeerResolverMode.cs
- QueryReaderSettings.cs
- DataTemplate.cs
- DocumentGrid.cs
- DynamicMetaObject.cs
- SafeNativeMethods.cs
- PerformanceCounterPermissionEntryCollection.cs
- CustomTrackingQuery.cs
- RootCodeDomSerializer.cs
- UIElementIsland.cs
- EncryptedPackageFilter.cs
- RuntimeWrappedException.cs
- TextSpanModifier.cs
- httpserverutility.cs
- EdmToObjectNamespaceMap.cs
- SocketAddress.cs
- DesignerGenericWebPart.cs
- SqlDataSourceCommandEventArgs.cs
- DBCommand.cs
- CheckBox.cs
- ClientSettings.cs
- ExtensionSimplifierMarkupObject.cs
- RemotingServices.cs
- DataGridTable.cs
- KeyGestureValueSerializer.cs
- HtmlShim.cs
- filewebrequest.cs
- SplitterCancelEvent.cs
- ModuleBuilderData.cs
- RadioButtonFlatAdapter.cs
- TypeBuilder.cs
- BitmapEffectInputData.cs
- DataGridViewTopLeftHeaderCell.cs
- InstanceDescriptor.cs
- FloatUtil.cs