Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / Structures / Constant.cs / 1305376 / Constant.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Common.Utils; using System.Collections.Generic; using System.Text; using System.Data.Mapping.ViewGeneration.CqlGeneration; using System.Diagnostics; using System.Data.Metadata.Edm; using System.Data.Mapping.ViewGeneration.Utils; namespace System.Data.Mapping.ViewGeneration.Structures { // This class denotes a constant that can be stored in multiconstants or // projected in fields internal class Constant : InternalBase { protected Constant() { // Private default constuctor so that no can construct this // object externally other than via subclasses } #region Fields // Two constants to denote nulls and undefined values // Order is important -- it is guaranteed by C# (see Section 10.4.5.1) internal static readonly IEqualityComparerEqualityComparer = new CellConstantComparer(); internal static readonly Constant Null = new Constant(); internal static readonly Constant Undefined = new Constant(); internal static readonly Constant NotNull = NegatedConstant.CreateNotNull(); //Represents scalar constants within a finite set that are not specified explicitly in the domain // Currently only used as a Sentinel node to prevent expression optimization internal static readonly Constant AllOtherConstants = new Constant(); #endregion #region Methods internal bool IsNull() { return EqualityComparer.Equals(this, Constant.Null); } internal bool IsUndefined() { return EqualityComparer.Equals(this, Constant.Undefined); } internal virtual bool IsNotNull() { // Provide a default implementation and let the subclasses // override it return false; // NegatedCellConstant handles it } // effects: Returns true if this contains not null internal virtual bool HasNotNull() { // Provide a default implementation and let the subclasses // override it return false; // NegatedCellConstant handles it } // effects: Modifies builder to contain the appropriate expression // corresponding to this. outputMember is the member to which this constant is directed. // blockAlias is the alias for the SELECT FROM WHERE block where this constant is being projected // if not null. Returns the modified stringbuilder internal virtual StringBuilder AsCql(StringBuilder builder, MemberPath outputMember, string blockAlias) { // Constants will never be simply entity sets -- so we can use Property on the path // constType is the type of this we need to cast to EdmType constType = Helper.GetModelTypeUsage(outputMember.LeafEdmMember).EdmType; Debug.Assert(this != Undefined && this != NotNull, "Generating Cql for undefined slots or not-nulls?"); Debug.Assert(this == Null, "Probably forgot to override this method in a subclass"); // need to cast builder.Append("CAST(NULL AS "); CqlWriter.AppendEscapedTypeName(builder, constType); builder.Append(')'); return builder; } // effects: Returns true if right has the same contents as this protected virtual int GetHash() { Debug.Assert(this == Null || this == Undefined || this == AllOtherConstants, "CellConstants can only be Null, Undefined, or AllOtherConstants"); return 0; } // effects: Returns true if right has the same contents as this protected virtual bool IsEqualTo(Constant right) { Debug.Assert(this == Null || this == Undefined || this == AllOtherConstants, "CellConstants can only be Null, Undefined, or AllOtherConstants"); return this == right; } public override bool Equals(object obj) { Constant cellConst = obj as Constant; if (cellConst == null) { return false; } else { return IsEqualTo(cellConst); } } public override int GetHashCode() { return base.GetHashCode(); } internal virtual string ToUserString() { StringBuilder builder = new StringBuilder(); InternalToString(builder, false); return builder.ToString(); } [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] internal static void ConstantsToUserString(StringBuilder builder, Set constants) { bool isFirst = true; foreach (Constant constant in constants) { if (isFirst == false) { builder.Append(System.Data.Entity.Strings.ViewGen_CommaBlank); } isFirst = false; string constrStr = constant.ToUserString(); builder.Append(constrStr); } } private void InternalToString(StringBuilder builder, bool isInvariant) { string result; if (this == Null) { result = isInvariant ? "NULL" : System.Data.Entity.Strings.ViewGen_Null; } else if (this == Undefined) { Debug.Assert(isInvariant, "We should not emit a message about Undefined constants to the user"); result = "?"; } else if (this == NotNull) { result = isInvariant ? "NOT_NULL" : System.Data.Entity.Strings.ViewGen_NotNull; } else if (this == AllOtherConstants) { result = "AllOtherConstants"; } else { Debug.Fail("No other constants handled by this class"); result = isInvariant ? "FAILURE" : System.Data.Entity.Strings.ViewGen_Failure; } builder.Append(result); } internal override void ToCompactString(StringBuilder builder) { InternalToString(builder, true); } #endregion #region Comparer class private class CellConstantComparer : IEqualityComparer { public bool Equals(Constant left, Constant right) { // Quick check with references if (object.ReferenceEquals(left, right)) { // Gets the Null and Undefined case as well return true; } // One of them is non-null at least. So if the other one is // null, we cannot be equal if (left == null || right == null) { return false; } // Both are non-null at this point return left.IsEqualTo(right); } public int GetHashCode(Constant key) { EntityUtil.CheckArgumentNull(key, "key"); return key.GetHash(); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
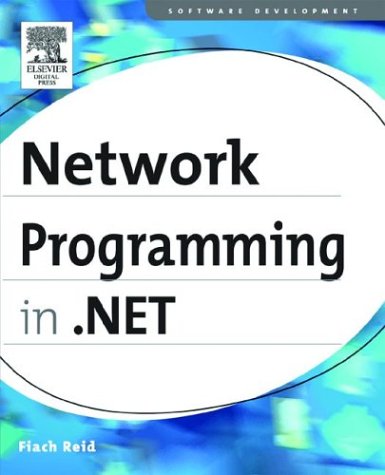
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataPagerFieldCommandEventArgs.cs
- Metafile.cs
- HttpPostedFileBase.cs
- WebPart.cs
- JumpTask.cs
- ReferenceService.cs
- SingleQueryOperator.cs
- LZCodec.cs
- BitArray.cs
- StrongTypingException.cs
- NonSerializedAttribute.cs
- Options.cs
- CodeStatement.cs
- DataGridViewRowConverter.cs
- XamlWriter.cs
- HttpCapabilitiesBase.cs
- ToolboxDataAttribute.cs
- CodeArgumentReferenceExpression.cs
- DataGridTextBoxColumn.cs
- QueryOpcode.cs
- XmlSchemaException.cs
- Rfc2898DeriveBytes.cs
- ExpressionTable.cs
- AddingNewEventArgs.cs
- WebBrowserUriTypeConverter.cs
- Attribute.cs
- NamespaceList.cs
- SoapTransportImporter.cs
- WhiteSpaceTrimStringConverter.cs
- VarInfo.cs
- HtmlInputText.cs
- DesignTimeTemplateParser.cs
- RepeatInfo.cs
- LicenseManager.cs
- ApplicationSecurityInfo.cs
- SafeBitVector32.cs
- GotoExpression.cs
- JumpPath.cs
- EtwTrackingBehavior.cs
- FileChangeNotifier.cs
- Point3DCollectionConverter.cs
- Calendar.cs
- AssemblyCollection.cs
- FamilyTypefaceCollection.cs
- AQNBuilder.cs
- UnicodeEncoding.cs
- MapPathBasedVirtualPathProvider.cs
- DeflateStream.cs
- Transform.cs
- NonceToken.cs
- DescendantOverDescendantQuery.cs
- SizeValueSerializer.cs
- AutoCompleteStringCollection.cs
- SchemaInfo.cs
- OptionalRstParameters.cs
- SessionPageStateSection.cs
- SqlTypeSystemProvider.cs
- ValidatorCompatibilityHelper.cs
- Renderer.cs
- AppSettingsExpressionBuilder.cs
- RecognitionResult.cs
- TemplatedEditableDesignerRegion.cs
- SecurityResources.cs
- TreeNodeStyleCollectionEditor.cs
- DataRowChangeEvent.cs
- NeutralResourcesLanguageAttribute.cs
- FamilyTypefaceCollection.cs
- Util.cs
- Vector3DCollectionValueSerializer.cs
- MaterializeFromAtom.cs
- VisualStyleElement.cs
- RelationshipDetailsRow.cs
- SoundPlayerAction.cs
- AttributeAction.cs
- TextEffect.cs
- TemplatePropertyEntry.cs
- Int64Storage.cs
- HijriCalendar.cs
- Control.cs
- WindowsToolbar.cs
- LogFlushAsyncResult.cs
- PathSegment.cs
- ServerIdentity.cs
- MultiTrigger.cs
- SpeakCompletedEventArgs.cs
- PenContexts.cs
- EntityDataSourceWrapperCollection.cs
- OdbcDataReader.cs
- Variant.cs
- XmlSortKeyAccumulator.cs
- Rect3D.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- FlowDocumentReader.cs
- EncryptedType.cs
- EntityClassGenerator.cs
- SourceElementsCollection.cs
- SemanticResultKey.cs
- RegistryPermission.cs
- CompilationPass2TaskInternal.cs
- UpdatePanel.cs