Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / MultiTrigger.cs / 1 / MultiTrigger.cs
using System.Collections.Specialized; using System.ComponentModel; using System.IO; using System.Windows.Markup; using MS.Utility; using System; using System.Diagnostics; namespace System.Windows { ////// A multiple Style property conditional dependency driver /// [ContentProperty("Setters")] public sealed class MultiTrigger : TriggerBase, IAddChild { ////// Conditions collection /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public ConditionCollection Conditions { get { // Verify Context Access VerifyAccess(); return _conditions; } } ////// Collection of Setter objects, which describes what to apply /// when this trigger is active. /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public SetterBaseCollection Setters { get { // Verify Context Access VerifyAccess(); if( _setters == null ) { _setters = new SetterBaseCollection(); } return _setters; } } ////// This method is called to Add a Setter object as a child of the Style. /// /// /// The object to add as a child; it must be a Setter or subclass. /// void IAddChild.AddChild (Object value) { // Verify Context Access VerifyAccess(); Setters.Add(Trigger.CheckChildIsSetter(value)); } ////// This method is called by the parser when text appears under the tag in markup. /// As default Styles do not support text, calling this method has no effect. /// /// /// Text to add as a child. /// void IAddChild.AddText (string text) { // Verify Context Access VerifyAccess(); XamlSerializerUtil.ThrowIfNonWhiteSpaceInAddText(text, this); } internal override void Seal() { if (IsSealed) { return; } // Process the _setters collection: Copy values into PropertyValueList and seal the Setter objects. ProcessSettersCollection(_setters); if (_conditions.Count > 0) { // Seal conditions _conditions.Seal(ValueLookupType.Trigger); } // Build conditions array from collection TriggerConditions = new TriggerCondition[_conditions.Count]; for (int i = 0; i < TriggerConditions.Length; i++) { TriggerConditions[i] = new TriggerCondition( _conditions[i].Property, LogicalOp.Equals, _conditions[i].Value, (_conditions[i].SourceName != null) ? _conditions[i].SourceName : StyleHelper.SelfName); } // Set conditions array for all property triggers for (int i = 0; i < PropertyValues.Count; i++) { PropertyValue propertyValue = PropertyValues[i]; propertyValue.Conditions = TriggerConditions; // Put back modified struct PropertyValues[i] = propertyValue; } base.Seal(); } // evaluate the current state of the trigger internal override bool GetCurrentState(DependencyObject container, UncommonFielddataField) { bool retVal = (TriggerConditions.Length > 0); for( int i = 0; retVal && i < TriggerConditions.Length; i++ ) { Debug.Assert( TriggerConditions[i].SourceChildIndex == 0, "This method was created to handle properties on the containing object, more work is needed to handle templated children too." ); retVal = TriggerConditions[i].Match(container.GetValue(TriggerConditions[i].Property)); } return retVal; } private ConditionCollection _conditions = new ConditionCollection(); private SetterBaseCollection _setters = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System.Collections.Specialized; using System.ComponentModel; using System.IO; using System.Windows.Markup; using MS.Utility; using System; using System.Diagnostics; namespace System.Windows { /// /// A multiple Style property conditional dependency driver /// [ContentProperty("Setters")] public sealed class MultiTrigger : TriggerBase, IAddChild { ////// Conditions collection /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public ConditionCollection Conditions { get { // Verify Context Access VerifyAccess(); return _conditions; } } ////// Collection of Setter objects, which describes what to apply /// when this trigger is active. /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public SetterBaseCollection Setters { get { // Verify Context Access VerifyAccess(); if( _setters == null ) { _setters = new SetterBaseCollection(); } return _setters; } } ////// This method is called to Add a Setter object as a child of the Style. /// /// /// The object to add as a child; it must be a Setter or subclass. /// void IAddChild.AddChild (Object value) { // Verify Context Access VerifyAccess(); Setters.Add(Trigger.CheckChildIsSetter(value)); } ////// This method is called by the parser when text appears under the tag in markup. /// As default Styles do not support text, calling this method has no effect. /// /// /// Text to add as a child. /// void IAddChild.AddText (string text) { // Verify Context Access VerifyAccess(); XamlSerializerUtil.ThrowIfNonWhiteSpaceInAddText(text, this); } internal override void Seal() { if (IsSealed) { return; } // Process the _setters collection: Copy values into PropertyValueList and seal the Setter objects. ProcessSettersCollection(_setters); if (_conditions.Count > 0) { // Seal conditions _conditions.Seal(ValueLookupType.Trigger); } // Build conditions array from collection TriggerConditions = new TriggerCondition[_conditions.Count]; for (int i = 0; i < TriggerConditions.Length; i++) { TriggerConditions[i] = new TriggerCondition( _conditions[i].Property, LogicalOp.Equals, _conditions[i].Value, (_conditions[i].SourceName != null) ? _conditions[i].SourceName : StyleHelper.SelfName); } // Set conditions array for all property triggers for (int i = 0; i < PropertyValues.Count; i++) { PropertyValue propertyValue = PropertyValues[i]; propertyValue.Conditions = TriggerConditions; // Put back modified struct PropertyValues[i] = propertyValue; } base.Seal(); } // evaluate the current state of the trigger internal override bool GetCurrentState(DependencyObject container, UncommonFielddataField) { bool retVal = (TriggerConditions.Length > 0); for( int i = 0; retVal && i < TriggerConditions.Length; i++ ) { Debug.Assert( TriggerConditions[i].SourceChildIndex == 0, "This method was created to handle properties on the containing object, more work is needed to handle templated children too." ); retVal = TriggerConditions[i].Match(container.GetValue(TriggerConditions[i].Property)); } return retVal; } private ConditionCollection _conditions = new ConditionCollection(); private SetterBaseCollection _setters = null; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
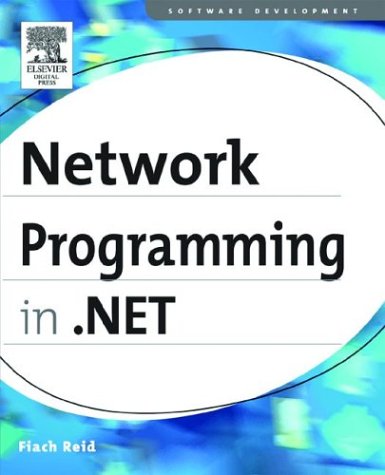
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataContext.cs
- NullableBoolConverter.cs
- ConstraintEnumerator.cs
- EncoderParameter.cs
- InputLangChangeEvent.cs
- XmlNode.cs
- EventSourceCreationData.cs
- BitmapData.cs
- BamlStream.cs
- HtmlInputHidden.cs
- ResponseStream.cs
- CheckBoxDesigner.cs
- DesignerSerializerAttribute.cs
- FormatConvertedBitmap.cs
- ApplicationDirectory.cs
- CheckBoxDesigner.cs
- AvTrace.cs
- StandardRuntimeEnumValidatorAttribute.cs
- EventLogPermissionEntryCollection.cs
- TextEditorSpelling.cs
- ElementsClipboardData.cs
- ValidatingPropertiesEventArgs.cs
- FastPropertyAccessor.cs
- Help.cs
- XmlChildEnumerator.cs
- WinFormsComponentEditor.cs
- DynamicMethod.cs
- WorkflowPageSetupDialog.cs
- PermissionSetTriple.cs
- StrokeNodeData.cs
- DBParameter.cs
- diagnosticsswitches.cs
- ReadOnlyTernaryTree.cs
- EntityDataSourceValidationException.cs
- CellQuery.cs
- TextViewBase.cs
- SubMenuStyle.cs
- QualifierSet.cs
- BaseTreeIterator.cs
- NonDualMessageSecurityOverHttpElement.cs
- EnvironmentPermission.cs
- SoapAttributeAttribute.cs
- FilteredDataSetHelper.cs
- SurrogateSelector.cs
- EntityModelBuildProvider.cs
- SimpleType.cs
- ServiceOperationParameter.cs
- DetailsViewPagerRow.cs
- ConfigurationFileMap.cs
- MeshGeometry3D.cs
- SqlTriggerContext.cs
- SchemaImporterExtensionsSection.cs
- LogEntryHeaderSerializer.cs
- EventManager.cs
- errorpatternmatcher.cs
- UnionCodeGroup.cs
- MD5.cs
- WithParamAction.cs
- ConvertEvent.cs
- errorpatternmatcher.cs
- SecurityTokenAuthenticator.cs
- XamlBrushSerializer.cs
- TagMapInfo.cs
- OdbcInfoMessageEvent.cs
- WindowsToolbar.cs
- StateItem.cs
- XmlSchemaInclude.cs
- CustomDictionarySources.cs
- UserControlFileEditor.cs
- PersistenceContextEnlistment.cs
- propertytag.cs
- Assign.cs
- ListViewInsertionMark.cs
- OleDbError.cs
- NavigationFailedEventArgs.cs
- IdentifierService.cs
- MenuItemStyleCollection.cs
- LineServices.cs
- WindowsRichEdit.cs
- TextRangeBase.cs
- SoapFormatter.cs
- StrongName.cs
- SocketInformation.cs
- ObjectKeyFrameCollection.cs
- __ComObject.cs
- WindowClosedEventArgs.cs
- Parser.cs
- EntityContainer.cs
- ResourceFallbackManager.cs
- EnumType.cs
- BidOverLoads.cs
- Timeline.cs
- XmlSignatureManifest.cs
- ProviderMetadata.cs
- SoapReflectionImporter.cs
- ObjectPersistData.cs
- RequestQueue.cs
- TimeSpanFormat.cs
- DateTimeSerializationSection.cs
- PartBasedPackageProperties.cs