Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Navigation / NavigationFailedEventArgs.cs / 1 / NavigationFailedEventArgs.cs
//-------------------------------------------------------------------------------------------------- // File: NavigationFailedCancelEventArgs.cs // // Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // // Description: // This event is fired when an error is encountered during a navigation. // The NavigationFailedEventArgs contains the error status code and // the exception that was thrown. By default Handled property is set to false, // which allows the exception to be rethrown. // The event handler can prevent exception from throwing // to the user by setting the Handled property to true // // This event is fired on navigation container and refired on the NavigationApplication // // History: // 06/10/06: huwang Created // //------------------------------------------------------------------------------------------------- using System.ComponentModel; using System.Net; namespace System.Windows.Navigation { ////// Event args for NavigationFailed event /// The NavigationFailedEventArgs contains the exception that was thrown. /// By default Handled property is set to false. /// The event handler can prevent the exception from being throwing to the user by setting /// the Handled property to true /// public class NavigationFailedEventArgs : EventArgs { // Internal constructor internal NavigationFailedEventArgs(Uri uri, Object extraData, Object navigator, WebRequest request, WebResponse response, Exception e) { _uri = uri; _extraData = extraData; _navigator = navigator; _request = request; _response = response; _exception = e; } ////// URI of the markup page to navigate to. /// public Uri Uri { get { return _uri; } } ////// Exposes extra data object which was optionally passed as a parameter to Navigate. /// public Object ExtraData { get { return _extraData; } } ////// The navigator that raised this event /// public object Navigator { get { return _navigator; } } ////// Exposes the WebRequest used to retrieve content. /// public WebRequest WebRequest { get { return _request; } } ////// Exposes the WebResponse used to retrieve content. /// public WebResponse WebResponse { get { return _response; } } ////// Exception that was thrown during the navigation /// public Exception Exception { get { return _exception; } } ////// Returns a boolean flag indicating if or not this event has been handled. /// public bool Handled { get { return _handled; } set { _handled = value; } } Uri _uri; Object _extraData; Object _navigator; WebRequest _request; WebResponse _response; Exception _exception; bool _handled = false; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //-------------------------------------------------------------------------------------------------- // File: NavigationFailedCancelEventArgs.cs // // Copyright (C) 2004 by Microsoft Corporation. All rights reserved. // // Description: // This event is fired when an error is encountered during a navigation. // The NavigationFailedEventArgs contains the error status code and // the exception that was thrown. By default Handled property is set to false, // which allows the exception to be rethrown. // The event handler can prevent exception from throwing // to the user by setting the Handled property to true // // This event is fired on navigation container and refired on the NavigationApplication // // History: // 06/10/06: huwang Created // //------------------------------------------------------------------------------------------------- using System.ComponentModel; using System.Net; namespace System.Windows.Navigation { ////// Event args for NavigationFailed event /// The NavigationFailedEventArgs contains the exception that was thrown. /// By default Handled property is set to false. /// The event handler can prevent the exception from being throwing to the user by setting /// the Handled property to true /// public class NavigationFailedEventArgs : EventArgs { // Internal constructor internal NavigationFailedEventArgs(Uri uri, Object extraData, Object navigator, WebRequest request, WebResponse response, Exception e) { _uri = uri; _extraData = extraData; _navigator = navigator; _request = request; _response = response; _exception = e; } ////// URI of the markup page to navigate to. /// public Uri Uri { get { return _uri; } } ////// Exposes extra data object which was optionally passed as a parameter to Navigate. /// public Object ExtraData { get { return _extraData; } } ////// The navigator that raised this event /// public object Navigator { get { return _navigator; } } ////// Exposes the WebRequest used to retrieve content. /// public WebRequest WebRequest { get { return _request; } } ////// Exposes the WebResponse used to retrieve content. /// public WebResponse WebResponse { get { return _response; } } ////// Exception that was thrown during the navigation /// public Exception Exception { get { return _exception; } } ////// Returns a boolean flag indicating if or not this event has been handled. /// public bool Handled { get { return _handled; } set { _handled = value; } } Uri _uri; Object _extraData; Object _navigator; WebRequest _request; WebResponse _response; Exception _exception; bool _handled = false; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
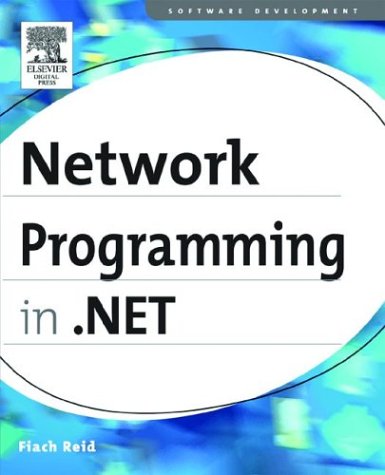
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LocatorPart.cs
- FileLoadException.cs
- HttpConfigurationSystem.cs
- SelectionWordBreaker.cs
- SelectionRangeConverter.cs
- UserControlBuildProvider.cs
- AmbientLight.cs
- CustomPopupPlacement.cs
- ComponentEvent.cs
- ListControl.cs
- PerfCounterSection.cs
- SemaphoreSecurity.cs
- ScaleTransform3D.cs
- Panel.cs
- TextTreeNode.cs
- DataTableReader.cs
- AbstractSvcMapFileLoader.cs
- IPEndPoint.cs
- SignatureToken.cs
- GPPOINTF.cs
- input.cs
- FormsIdentity.cs
- EmbossBitmapEffect.cs
- HandlerFactoryCache.cs
- HttpCacheVary.cs
- FolderLevelBuildProvider.cs
- XmlHierarchicalEnumerable.cs
- LeafCellTreeNode.cs
- unsafenativemethodsother.cs
- SaveFileDialog.cs
- OrderPreservingSpoolingTask.cs
- WorkflowApplicationEventArgs.cs
- SafeMemoryMappedFileHandle.cs
- StrokeRenderer.cs
- ContractAdapter.cs
- TextBoxAutoCompleteSourceConverter.cs
- GlobalProxySelection.cs
- ViewBase.cs
- PriorityChain.cs
- CodeSubDirectory.cs
- compensatingcollection.cs
- COM2ExtendedTypeConverter.cs
- TdsParserStateObject.cs
- Cell.cs
- NonBatchDirectoryCompiler.cs
- ChannelSinkStacks.cs
- ChtmlTextWriter.cs
- D3DImage.cs
- StringExpressionSet.cs
- SqlProfileProvider.cs
- VoiceChangeEventArgs.cs
- InitializerFacet.cs
- CrossAppDomainChannel.cs
- EntityDataSourceDesigner.cs
- WebReferenceOptions.cs
- RijndaelManaged.cs
- X509CertificateInitiatorClientCredential.cs
- ProtocolElementCollection.cs
- FunctionCommandText.cs
- BaseTemplatedMobileComponentEditor.cs
- TargetConverter.cs
- OpCopier.cs
- FunctionImportMapping.cs
- ClientSession.cs
- Query.cs
- CorrelationValidator.cs
- WindowsGrip.cs
- MarkupProperty.cs
- UserCancellationException.cs
- SocketCache.cs
- RotateTransform3D.cs
- RegexRunnerFactory.cs
- StylusPointProperties.cs
- Tokenizer.cs
- XmlReflectionMember.cs
- SelectionRange.cs
- ObjectItemCollection.cs
- CqlBlock.cs
- NullNotAllowedCollection.cs
- TemplateBindingExtensionConverter.cs
- relpropertyhelper.cs
- BamlBinaryWriter.cs
- filewebresponse.cs
- ObjectPersistData.cs
- SubMenuStyleCollectionEditor.cs
- AudioException.cs
- ExpressionBinding.cs
- WebBrowserContainer.cs
- DesignerSerializationManager.cs
- NameTable.cs
- EntityViewGenerator.cs
- LabelTarget.cs
- TabItemWrapperAutomationPeer.cs
- ToolZoneDesigner.cs
- PersonalizationStateInfo.cs
- CheckBox.cs
- BufferedWebEventProvider.cs
- DesignerTextBoxAdapter.cs
- DocumentGridContextMenu.cs
- ConversionHelper.cs