Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / WinForms / Managed / System / WinForms / LinkUtilities.cs / 1 / LinkUtilities.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Windows.Forms; using Microsoft.Win32; using System.Globalization; using System.Security.Permissions; internal class LinkUtilities { // IE fonts and colors static Color ielinkColor = Color.Empty; static Color ieactiveLinkColor = Color.Empty; static Color ievisitedLinkColor = Color.Empty; const string IESettingsRegPath = "Software\\Microsoft\\Internet Explorer\\Settings"; public const string IEMainRegPath = "Software\\Microsoft\\Internet Explorer\\Main"; const string IEAnchorColor = "Anchor Color"; const string IEAnchorColorVisited = "Anchor Color Visited"; const string IEAnchorColorHover = "Anchor Color Hover"; ////// /// Retrieves a named IE color from the registry. There are constants at the top /// of this file of the valid names to retrieve. /// private static Color GetIEColor(string name) { // SECREVIEW : We are just reading the IE color settings from the registry... // // SECUNDONE : This assert doesn't work... assert everything for now... // //new RegistryPermission(RegistryPermissionAccess.Read, "HKCU\\" + IESettingsRegPath).Assert(); new RegistryPermission(PermissionState.Unrestricted).Assert(); try { RegistryKey key = Registry.CurrentUser.OpenSubKey(IESettingsRegPath); if (key != null) { // Since this comes from the registry, be very careful about its contents. // string s = (string)key.GetValue(name); if (s != null) { string[] rgbs = s.Split(new char[] {','}); int[] rgb = new int[3]; int nMax = Math.Min(rgb.Length, rgbs.Length); //NOTE: if we can't parse rgbs[i], rgb[i] will be set to 0. for (int i = 0; i < nMax; i++) { int.TryParse(rgbs[i], out rgb[i]); } return Color.FromArgb(rgb[0], rgb[1], rgb[2]); } } if (string.Equals(name, IEAnchorColor, StringComparison.OrdinalIgnoreCase)) { return Color.Blue; } else if (string.Equals(name, IEAnchorColorVisited, StringComparison.OrdinalIgnoreCase)) { return Color.Purple; } else if (string.Equals(name, IEAnchorColorHover, StringComparison.OrdinalIgnoreCase)) { return Color.Red; } else { return Color.Red; } } finally { System.Security.CodeAccessPermission.RevertAssert(); } } public static Color IELinkColor { get { if (ielinkColor.IsEmpty) { ielinkColor = GetIEColor(IEAnchorColor); } return ielinkColor; } } public static Color IEActiveLinkColor { get { if (ieactiveLinkColor.IsEmpty) { ieactiveLinkColor = GetIEColor(IEAnchorColorHover); } return ieactiveLinkColor; } } public static Color IEVisitedLinkColor { get { if (ievisitedLinkColor.IsEmpty) { ievisitedLinkColor = GetIEColor(IEAnchorColorVisited); } return ievisitedLinkColor; } } ////// /// Retrieves the IE settings for link behavior from the registry. /// public static LinkBehavior GetIELinkBehavior() { // SECREVIEW : We are just reading the IE color settings from the registry... // // SECUNDONE : This assert doesn't work... assert everything for now... // //new RegistryPermission(RegistryPermissionAccess.Read, "HKCU\\" + IEMainRegPath).Assert(); new RegistryPermission(PermissionState.Unrestricted).Assert(); try { RegistryKey key = Registry.CurrentUser.OpenSubKey(IEMainRegPath); if (key != null) { string s = (string)key.GetValue("Anchor Underline"); if (s != null && string.Compare(s, "no", true, CultureInfo.InvariantCulture) == 0) { return LinkBehavior.NeverUnderline; } if (s != null && string.Compare(s, "hover", true, CultureInfo.InvariantCulture) == 0) { return LinkBehavior.HoverUnderline; } else { return LinkBehavior.AlwaysUnderline; } } } finally { System.Security.CodeAccessPermission.RevertAssert(); } return LinkBehavior.AlwaysUnderline; } public static void EnsureLinkFonts(Font baseFont, LinkBehavior link, ref Font linkFont, ref Font hoverLinkFont) { if (linkFont != null && hoverLinkFont != null) { return; } bool underlineLink = true; bool underlineHover = true; if (link == LinkBehavior.SystemDefault) { link = GetIELinkBehavior(); } switch (link) { case LinkBehavior.AlwaysUnderline: underlineLink = true; underlineHover = true; break; case LinkBehavior.HoverUnderline: underlineLink = false; underlineHover = true; break; case LinkBehavior.NeverUnderline: underlineLink = false; underlineHover = false; break; } Font f = baseFont; // We optimize for the "same" value (never & always) to avoid creating an // extra font object. // if (underlineHover == underlineLink) { FontStyle style = f.Style; if (underlineHover) { style |= FontStyle.Underline; } else { style &= ~FontStyle.Underline; } hoverLinkFont = new Font(f, style); linkFont = hoverLinkFont; } else { FontStyle hoverStyle = f.Style; if (underlineHover) { hoverStyle |= FontStyle.Underline; } else { hoverStyle &= ~FontStyle.Underline; } hoverLinkFont = new Font(f, hoverStyle); FontStyle linkStyle = f.Style; if (underlineLink) { linkStyle |= FontStyle.Underline; } else { linkStyle &= ~FontStyle.Underline; } linkFont = new Font(f, linkStyle); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Windows.Forms; using Microsoft.Win32; using System.Globalization; using System.Security.Permissions; internal class LinkUtilities { // IE fonts and colors static Color ielinkColor = Color.Empty; static Color ieactiveLinkColor = Color.Empty; static Color ievisitedLinkColor = Color.Empty; const string IESettingsRegPath = "Software\\Microsoft\\Internet Explorer\\Settings"; public const string IEMainRegPath = "Software\\Microsoft\\Internet Explorer\\Main"; const string IEAnchorColor = "Anchor Color"; const string IEAnchorColorVisited = "Anchor Color Visited"; const string IEAnchorColorHover = "Anchor Color Hover"; ////// /// Retrieves a named IE color from the registry. There are constants at the top /// of this file of the valid names to retrieve. /// private static Color GetIEColor(string name) { // SECREVIEW : We are just reading the IE color settings from the registry... // // SECUNDONE : This assert doesn't work... assert everything for now... // //new RegistryPermission(RegistryPermissionAccess.Read, "HKCU\\" + IESettingsRegPath).Assert(); new RegistryPermission(PermissionState.Unrestricted).Assert(); try { RegistryKey key = Registry.CurrentUser.OpenSubKey(IESettingsRegPath); if (key != null) { // Since this comes from the registry, be very careful about its contents. // string s = (string)key.GetValue(name); if (s != null) { string[] rgbs = s.Split(new char[] {','}); int[] rgb = new int[3]; int nMax = Math.Min(rgb.Length, rgbs.Length); //NOTE: if we can't parse rgbs[i], rgb[i] will be set to 0. for (int i = 0; i < nMax; i++) { int.TryParse(rgbs[i], out rgb[i]); } return Color.FromArgb(rgb[0], rgb[1], rgb[2]); } } if (string.Equals(name, IEAnchorColor, StringComparison.OrdinalIgnoreCase)) { return Color.Blue; } else if (string.Equals(name, IEAnchorColorVisited, StringComparison.OrdinalIgnoreCase)) { return Color.Purple; } else if (string.Equals(name, IEAnchorColorHover, StringComparison.OrdinalIgnoreCase)) { return Color.Red; } else { return Color.Red; } } finally { System.Security.CodeAccessPermission.RevertAssert(); } } public static Color IELinkColor { get { if (ielinkColor.IsEmpty) { ielinkColor = GetIEColor(IEAnchorColor); } return ielinkColor; } } public static Color IEActiveLinkColor { get { if (ieactiveLinkColor.IsEmpty) { ieactiveLinkColor = GetIEColor(IEAnchorColorHover); } return ieactiveLinkColor; } } public static Color IEVisitedLinkColor { get { if (ievisitedLinkColor.IsEmpty) { ievisitedLinkColor = GetIEColor(IEAnchorColorVisited); } return ievisitedLinkColor; } } ////// /// Retrieves the IE settings for link behavior from the registry. /// public static LinkBehavior GetIELinkBehavior() { // SECREVIEW : We are just reading the IE color settings from the registry... // // SECUNDONE : This assert doesn't work... assert everything for now... // //new RegistryPermission(RegistryPermissionAccess.Read, "HKCU\\" + IEMainRegPath).Assert(); new RegistryPermission(PermissionState.Unrestricted).Assert(); try { RegistryKey key = Registry.CurrentUser.OpenSubKey(IEMainRegPath); if (key != null) { string s = (string)key.GetValue("Anchor Underline"); if (s != null && string.Compare(s, "no", true, CultureInfo.InvariantCulture) == 0) { return LinkBehavior.NeverUnderline; } if (s != null && string.Compare(s, "hover", true, CultureInfo.InvariantCulture) == 0) { return LinkBehavior.HoverUnderline; } else { return LinkBehavior.AlwaysUnderline; } } } finally { System.Security.CodeAccessPermission.RevertAssert(); } return LinkBehavior.AlwaysUnderline; } public static void EnsureLinkFonts(Font baseFont, LinkBehavior link, ref Font linkFont, ref Font hoverLinkFont) { if (linkFont != null && hoverLinkFont != null) { return; } bool underlineLink = true; bool underlineHover = true; if (link == LinkBehavior.SystemDefault) { link = GetIELinkBehavior(); } switch (link) { case LinkBehavior.AlwaysUnderline: underlineLink = true; underlineHover = true; break; case LinkBehavior.HoverUnderline: underlineLink = false; underlineHover = true; break; case LinkBehavior.NeverUnderline: underlineLink = false; underlineHover = false; break; } Font f = baseFont; // We optimize for the "same" value (never & always) to avoid creating an // extra font object. // if (underlineHover == underlineLink) { FontStyle style = f.Style; if (underlineHover) { style |= FontStyle.Underline; } else { style &= ~FontStyle.Underline; } hoverLinkFont = new Font(f, style); linkFont = hoverLinkFont; } else { FontStyle hoverStyle = f.Style; if (underlineHover) { hoverStyle |= FontStyle.Underline; } else { hoverStyle &= ~FontStyle.Underline; } hoverLinkFont = new Font(f, hoverStyle); FontStyle linkStyle = f.Style; if (underlineLink) { linkStyle |= FontStyle.Underline; } else { linkStyle &= ~FontStyle.Underline; } linkFont = new Font(f, linkStyle); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
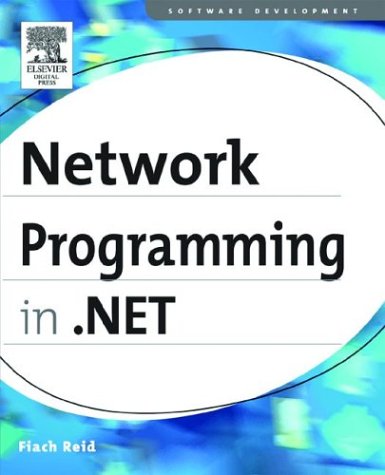
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProfilePropertySettings.cs
- EditorOptionAttribute.cs
- ClickablePoint.cs
- DrawingVisual.cs
- XmlImplementation.cs
- ExternalException.cs
- CollectionChangeEventArgs.cs
- Soap11ServerProtocol.cs
- ObjectStateEntry.cs
- BinaryOperationBinder.cs
- ProxyAttribute.cs
- ReflectionHelper.cs
- ResourcesChangeInfo.cs
- ConfigurationManager.cs
- Cloud.cs
- ProtocolProfile.cs
- RegionData.cs
- Camera.cs
- OneWayChannelFactory.cs
- CaseExpr.cs
- uribuilder.cs
- Container.cs
- SymLanguageType.cs
- SQLBinary.cs
- Vector3dCollection.cs
- DbDeleteCommandTree.cs
- ContractReference.cs
- FlowchartDesignerCommands.cs
- SqlVisitor.cs
- HtmlInputButton.cs
- TextPointerBase.cs
- TrackBar.cs
- SpecularMaterial.cs
- NativeMethodsOther.cs
- Line.cs
- ExtractorMetadata.cs
- VariableQuery.cs
- OleDbWrapper.cs
- WorkflowValidationFailedException.cs
- GridViewRowEventArgs.cs
- TemplatedWizardStep.cs
- DataColumnPropertyDescriptor.cs
- PropertyChangeTracker.cs
- ImageKeyConverter.cs
- StrokeNodeEnumerator.cs
- Single.cs
- ProfileProvider.cs
- DefaultIfEmptyQueryOperator.cs
- TemplateKeyConverter.cs
- SpecialFolderEnumConverter.cs
- QilXmlWriter.cs
- ThreadAttributes.cs
- BrowsableAttribute.cs
- RotateTransform.cs
- InvalidAsynchronousStateException.cs
- RemoteWebConfigurationHost.cs
- HwndKeyboardInputProvider.cs
- FlowLayoutPanel.cs
- UnorderedHashRepartitionStream.cs
- FacetChecker.cs
- DataGridToolTip.cs
- GeneralTransform3DCollection.cs
- DataBoundLiteralControl.cs
- SecurityUtils.cs
- FixUp.cs
- MappingSource.cs
- AsyncContentLoadedEventArgs.cs
- SymLanguageType.cs
- AttributeCollection.cs
- FixUpCollection.cs
- EasingKeyFrames.cs
- XmlEncodedRawTextWriter.cs
- NavigationService.cs
- BinaryConverter.cs
- PolyBezierSegment.cs
- PtsCache.cs
- MimeObjectFactory.cs
- ToolStripItemTextRenderEventArgs.cs
- FrameworkElementFactory.cs
- DataGridViewColumnStateChangedEventArgs.cs
- ClientApiGenerator.cs
- TypeResolvingOptionsAttribute.cs
- COM2Enum.cs
- ElementHostPropertyMap.cs
- ConfigurationValidatorBase.cs
- TreeViewHitTestInfo.cs
- XmlSchemaFacet.cs
- Transaction.cs
- SettingsPropertyValueCollection.cs
- ProvidersHelper.cs
- UpDownBase.cs
- DbConnectionFactory.cs
- ImportFileRequest.cs
- Underline.cs
- ReachSerializationUtils.cs
- SchemaNamespaceManager.cs
- CodeBinaryOperatorExpression.cs
- HierarchicalDataSourceConverter.cs
- DialogResultConverter.cs
- ConfigurationSectionGroup.cs