Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / Media / MediaTimeline.cs / 2 / MediaTimeline.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, 2003 // // File: MediaTimeline.cs // //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.ComponentModel; using MS.Internal; using System.Windows.Media.Animation; using System.Windows.Media; using System.Windows.Media.Composition; using System.Windows.Markup; using System.Windows.Threading; using System.Runtime.InteropServices; using System.IO; using System.Collections; using System.Collections.Generic; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { #region MediaTimeline ////// MediaTimeline functions as a template for creating MediaClocks. Whenever /// you create a MediaClock it inherits all of the properties and events of /// the MediaTimeline. Whenever you change a property or register for an /// event on a MediaTimeline, all of those changes get propagated down to /// every MediaClock created off of that MediaTimeline (and any future /// MediaClocks created from it too). /// public partial class MediaTimeline : Timeline, IUriContext { internal const uint LastTimelineFlag = 0x1; #region Constructor ////// Creates a new MediaTimeline. /// /// Source of the media. public MediaTimeline(Uri source) : this() { Source = source; } ////// Creates a new MediaTimeline. /// /// Context used to resolve relative URIs /// Source of the media. internal MediaTimeline(ITypeDescriptorContext context, Uri source) : this() { _context = context; Source = source; } ////// Creates a new MediaTimeline. /// public MediaTimeline() { } ////// Creates a new MediaTimeline. /// /// The value for the BeginTime property public MediaTimeline(NullablebeginTime) : this() { BeginTime = beginTime; } /// /// Creates a new MediaTimeline. /// /// The value for the BeginTime property /// The value for the Duration property public MediaTimeline(NullablebeginTime, Duration duration) : this() { BeginTime = beginTime; Duration = duration; } /// /// Creates a new MediaTimeline. /// /// The value for the BeginTime property /// The value for the Duration property /// The value for the RepeatBehavior property public MediaTimeline(NullablebeginTime, Duration duration, RepeatBehavior repeatBehavior) : this() { BeginTime = beginTime; Duration = duration; RepeatBehavior = repeatBehavior; } #endregion #region IUriContext implementation /// /// Base Uri to use when resolving relative Uri's /// Uri IUriContext.BaseUri { get { return _baseUri; } set { _baseUri = value; } } #endregion #region Timeline ////// Called by the Clock to create a type-specific clock for this /// timeline. /// ////// A clock for this timeline. /// ////// If a derived class overrides this method, it should only create /// and return an object of a class inheriting from Clock. /// protected internal override Clock AllocateClock() { if (Source == null) { throw new InvalidOperationException(SR.Get(SRID.Media_UriNotSpecified)); } MediaClock mediaClock = new MediaClock(this); return mediaClock; } ////// Called by the base Freezable class to make this object /// frozen. /// protected override bool FreezeCore(bool isChecking) { bool canFreeze = base.FreezeCore(isChecking); if (!canFreeze) { return false; } // First, if checking, make sure that we don't have any expressions // on our properties. (base.FreezeCore takes care of animations) if (isChecking) { canFreeze &= !HasExpression(LookupEntry(SourceProperty.GlobalIndex), SourceProperty); } return canFreeze; } ////// Implementation of protected override void CloneCore(Freezable sourceFreezable) { MediaTimeline sourceTimeline = (MediaTimeline) sourceFreezable; base.CloneCore(sourceFreezable); CopyCommon(sourceTimeline); } ///Freezable.CloneCore . ////// Implementation of protected override void CloneCurrentValueCore(Freezable sourceFreezable) { MediaTimeline sourceTimeline = (MediaTimeline) sourceFreezable; base.CloneCurrentValueCore(sourceFreezable); CopyCommon(sourceTimeline); } ///Freezable.CloneCurrentValueCore . ////// Implementation of protected override void GetAsFrozenCore(Freezable source) { MediaTimeline sourceTimeline = (MediaTimeline) source; base.GetAsFrozenCore(source); CopyCommon(sourceTimeline); } ///Freezable.GetAsFrozenCore . ////// Implementation of protected override void GetCurrentValueAsFrozenCore(Freezable source) { MediaTimeline sourceTimeline = (MediaTimeline) source; base.GetCurrentValueAsFrozenCore(source); CopyCommon(sourceTimeline); } private void CopyCommon(MediaTimeline sourceTimeline) { _context = sourceTimeline._context; _baseUri = sourceTimeline._baseUri; } ///Freezable.GetCurrentValueAsFrozenCore . ////// Creates a new MediaClock using this MediaTimeline. /// ///A new MediaClock. public new MediaClock CreateClock() { return (MediaClock)base.CreateClock(); } ////// Return the duration from a specific clock /// /// /// The Clock whose natural duration is desired. /// ////// A Duration quantity representing the natural duration. /// protected override Duration GetNaturalDurationCore(Clock clock) { MediaClock mc = (MediaClock)clock; if (mc.Player == null) { return Duration.Automatic; } else { return mc.Player.NaturalDuration; } } #endregion #region ToString ////// Persist MediaTimeline in a string /// public override string ToString() { if (null == Source) throw new InvalidOperationException(SR.Get(SRID.Media_UriNotSpecified)); return Source.ToString(); } #endregion internal ITypeDescriptorContext _context = null; private Uri _baseUri = null; } #endregion }; // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
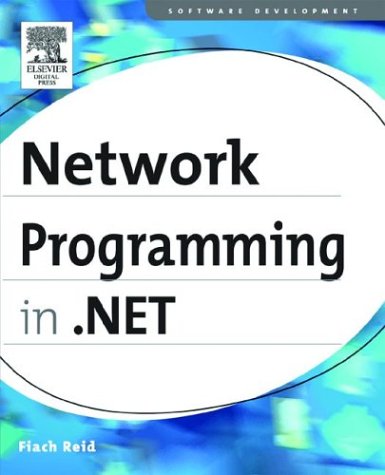
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Accessible.cs
- XPathDocument.cs
- SynchronizationContext.cs
- NativeMethods.cs
- CustomPopupPlacement.cs
- GlobalItem.cs
- StylusOverProperty.cs
- ToolStripContainer.cs
- CalendarItem.cs
- TransformDescriptor.cs
- ADConnectionHelper.cs
- JsonGlobals.cs
- TypeResolvingOptionsAttribute.cs
- HttpResponse.cs
- UriTemplateMatch.cs
- SafeCoTaskMem.cs
- AutoSizeToolBoxItem.cs
- ClassValidator.cs
- XmlSchemaAnnotated.cs
- ProfileGroupSettings.cs
- UniqueID.cs
- ToolStripDropDownClosingEventArgs.cs
- KeyValuePair.cs
- UnsafeNetInfoNativeMethods.cs
- ToolStripContentPanelRenderEventArgs.cs
- DataGridViewDataErrorEventArgs.cs
- StringResourceManager.cs
- SQLConvert.cs
- ListDictionary.cs
- WebControlAdapter.cs
- OdbcConnectionStringbuilder.cs
- WebPartMovingEventArgs.cs
- SqlConnectionFactory.cs
- HttpModuleCollection.cs
- PersonalizationState.cs
- UnmanagedHandle.cs
- DocumentCollection.cs
- WebPartVerb.cs
- FileDialogCustomPlacesCollection.cs
- DocumentPaginator.cs
- Calendar.cs
- NameValueSectionHandler.cs
- JsonServiceDocumentSerializer.cs
- SchemaCollectionPreprocessor.cs
- NetNamedPipeBindingCollectionElement.cs
- SystemSounds.cs
- TextBox.cs
- XmlStreamNodeWriter.cs
- MatrixCamera.cs
- MetaType.cs
- SiteMapHierarchicalDataSourceView.cs
- GradientSpreadMethodValidation.cs
- StringSorter.cs
- RunClient.cs
- XmlSchemaParticle.cs
- DefaultBindingPropertyAttribute.cs
- XmlMtomWriter.cs
- EpmContentSerializerBase.cs
- Rule.cs
- ObjectCloneHelper.cs
- JavaScriptSerializer.cs
- UInt64Storage.cs
- Renderer.cs
- CompiledIdentityConstraint.cs
- ZoneIdentityPermission.cs
- DataTableMappingCollection.cs
- WebHeaderCollection.cs
- ActivityDelegate.cs
- TypeDelegator.cs
- ObjectQuery_EntitySqlExtensions.cs
- ClientRuntimeConfig.cs
- WebBaseEventKeyComparer.cs
- XmlSchemaFacet.cs
- QuaternionConverter.cs
- ConstraintEnumerator.cs
- FrameworkContextData.cs
- OperationFormatStyle.cs
- ComponentCollection.cs
- ColorMatrix.cs
- Base64Stream.cs
- ConfigXmlCDataSection.cs
- CacheOutputQuery.cs
- IIS7UserPrincipal.cs
- ResourceContainer.cs
- RelationshipSet.cs
- UnmanagedMemoryStreamWrapper.cs
- WinFormsSpinner.cs
- StdValidatorsAndConverters.cs
- UnsafeCollabNativeMethods.cs
- DateTimeHelper.cs
- ScriptIgnoreAttribute.cs
- ButtonBase.cs
- IIS7UserPrincipal.cs
- DeploymentExceptionMapper.cs
- WebPartDisplayModeCollection.cs
- EditorPartCollection.cs
- ProviderSettings.cs
- RemotingService.cs
- EmptyControlCollection.cs
- XmlAttribute.cs