Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Epm / EpmContentSerializerBase.cs / 1305376 / EpmContentSerializerBase.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Base Classes used for EntityPropertyMappingAttribute related content // serializers // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Common { using System.Collections.Generic; using System.Diagnostics; using System.Linq; #if ASTORIA_CLIENT using System.Xml; #else using System.ServiceModel.Syndication; using System.Data.Services.Providers; #endif ////// What kind of xml nodes to serialize /// internal enum EpmSerializationKind { ////// Serialize only attributes /// Attributes, ////// Serialize only elements /// Elements, ////// Serialize everything /// All } ////// Base visitor class for performing serialization of content whose location in the syndication /// feed is provided through EntityPropertyMappingAttributes /// internal abstract class EpmContentSerializerBase { ////// Constructor decided whether to use syndication or non-syndication sub-tree for target content mappings /// /// Target tree containing mapping information /// Helps in deciding whether to use syndication sub-tree or non-syndication one /// Object to be serialized /// SyndicationItem to which content will be added #if ASTORIA_CLIENT protected EpmContentSerializerBase(EpmTargetTree tree, bool isSyndication, object element, XmlWriter target) #else protected EpmContentSerializerBase(EpmTargetTree tree, bool isSyndication, object element, SyndicationItem target) #endif { this.Root = isSyndication ? tree.SyndicationRoot : tree.NonSyndicationRoot; this.Element = element; this.Target = target; this.Success = false; } ///Root of the target tree containing mapped xml elements/attribute protected EpmTargetPathSegment Root { get; private set; } ///Object whose properties we will read protected object Element { get; private set; } ///Target SyndicationItem on which we are going to add the serialized content #if ASTORIA_CLIENT protected XmlWriter Target #else protected SyndicationItem Target #endif { get; private set; } ///Indicates the success or failure of serialization protected bool Success { get; private set; } #if ASTORIA_CLIENT ///Public interface used by the EpmContentSerializer class internal void Serialize() #else ///Public interface used by the EpmContentSerializer class /// Data Service provider used for rights verification. internal void Serialize(DataServiceProviderWrapper provider) #endif { foreach (EpmTargetPathSegment targetSegment in this.Root.SubSegments) { #if ASTORIA_CLIENT this.Serialize(targetSegment, EpmSerializationKind.All); #else this.Serialize(targetSegment, EpmSerializationKind.All, provider); #endif } this.Success = true; } #if ASTORIA_CLIENT ////// Internal interface to be overridden in the subclasses. /// Goes through each subsegments and invokes itself for the children /// /// Current root segment in the target tree /// Which sub segments to serialize protected virtual void Serialize(EpmTargetPathSegment targetSegment, EpmSerializationKind kind) #else ////// Internal interface to be overridden in the subclasses. /// Goes through each subsegments and invokes itself for the children /// /// Current root segment in the target tree /// Which sub segments to serialize /// Data Service provider used for rights verification. protected virtual void Serialize(EpmTargetPathSegment targetSegment, EpmSerializationKind kind, DataServiceProviderWrapper provider) #endif { IEnumerablesegmentsToSerialize; switch (kind) { case EpmSerializationKind.Attributes: segmentsToSerialize = targetSegment.SubSegments.Where(s => s.IsAttribute == true); break; case EpmSerializationKind.Elements: segmentsToSerialize = targetSegment.SubSegments.Where(s => s.IsAttribute == false); break; default: Debug.Assert(kind == EpmSerializationKind.All, "Must serialize everything"); segmentsToSerialize = targetSegment.SubSegments; break; } foreach (EpmTargetPathSegment segment in segmentsToSerialize) { #if ASTORIA_CLIENT this.Serialize(segment, kind); #else this.Serialize(segment, kind, provider); #endif } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
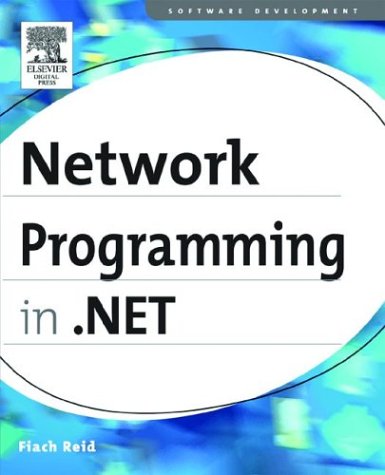
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaValidationException.cs
- ScriptBehaviorDescriptor.cs
- ZipFileInfoCollection.cs
- X509RawDataKeyIdentifierClause.cs
- CompiledQueryCacheEntry.cs
- AnchoredBlock.cs
- WebPartZone.cs
- LookupNode.cs
- DBCommandBuilder.cs
- DbConnectionPoolCounters.cs
- UInt64Storage.cs
- InitializeCorrelation.cs
- HistoryEventArgs.cs
- GridViewUpdatedEventArgs.cs
- ProcessHost.cs
- ScriptMethodAttribute.cs
- HTTPNotFoundHandler.cs
- WebAdminConfigurationHelper.cs
- SecurityTokenValidationException.cs
- ConfigViewGenerator.cs
- SynchronizedPool.cs
- DispatcherProcessingDisabled.cs
- EntityDataSource.cs
- AuthenticationConfig.cs
- FilteredReadOnlyMetadataCollection.cs
- ConfigXmlComment.cs
- CheckBoxFlatAdapter.cs
- DynamicResourceExtension.cs
- CompilationLock.cs
- xmlfixedPageInfo.cs
- CheckBoxAutomationPeer.cs
- SaveFileDialog.cs
- SecurityElement.cs
- Transform3D.cs
- HtmlControlPersistable.cs
- Win32SafeHandles.cs
- FieldAccessException.cs
- UserPersonalizationStateInfo.cs
- AllMembershipCondition.cs
- ChildTable.cs
- XamlTypeMapper.cs
- WebPartMinimizeVerb.cs
- ReadWriteSpinLock.cs
- CaseInsensitiveHashCodeProvider.cs
- ResourceReferenceExpression.cs
- XmlIlTypeHelper.cs
- FrameworkObject.cs
- SystemUnicastIPAddressInformation.cs
- ToolboxComponentsCreatingEventArgs.cs
- BufferedGraphicsManager.cs
- SubMenuStyleCollection.cs
- BamlStream.cs
- LiteralTextParser.cs
- SelectionWordBreaker.cs
- ContentValidator.cs
- DataRowChangeEvent.cs
- AttributeEmitter.cs
- ComPlusDiagnosticTraceSchemas.cs
- RequestCachePolicy.cs
- InvalidDataException.cs
- SqlUtil.cs
- ManagedWndProcTracker.cs
- InternalResources.cs
- Interlocked.cs
- CompoundFileIOPermission.cs
- LinkLabelLinkClickedEvent.cs
- ImpersonationContext.cs
- XmlCDATASection.cs
- CompiledQueryCacheEntry.cs
- JapaneseCalendar.cs
- EntryPointNotFoundException.cs
- XhtmlMobileTextWriter.cs
- FileIOPermission.cs
- VectorAnimation.cs
- TextDecorationUnitValidation.cs
- DataGridCaption.cs
- XmlExpressionDumper.cs
- PenThreadPool.cs
- InvokeProviderWrapper.cs
- Int32Storage.cs
- InheritanceContextHelper.cs
- ErrorLog.cs
- CategoryGridEntry.cs
- ReadOnlyPropertyMetadata.cs
- MethodToken.cs
- RequestDescription.cs
- BuildManagerHost.cs
- SettingsProviderCollection.cs
- Descriptor.cs
- WebPartConnectionsCancelEventArgs.cs
- DesigntimeLicenseContextSerializer.cs
- HyperlinkAutomationPeer.cs
- IISMapPath.cs
- DataControlImageButton.cs
- Literal.cs
- Attributes.cs
- FormsAuthenticationUser.cs
- FollowerQueueCreator.cs
- SystemIPInterfaceProperties.cs
- DateTimeValueSerializerContext.cs