Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / WinForms / Managed / System / WinForms / PropertyGridInternal / CategoryGridEntry.cs / 1 / CategoryGridEntry.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- //#define PAINT_CATEGORY_TRIANGLE /* */ namespace System.Windows.Forms.PropertyGridInternal { using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Collections; using System.Reflection; using System.ComponentModel; using System.ComponentModel.Design; using System.Windows.Forms; using System.Drawing; using Microsoft.Win32; internal class CategoryGridEntry : GridEntry { internal string name; private Brush backBrush = null; private static Hashtable categoryStates = null; [ SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors") // GridEntry classes are internal so we have complete // control over who does what in the constructor. ] public CategoryGridEntry(PropertyGrid ownerGrid, GridEntry peParent,string name, GridEntry[] childGridEntries) : base(ownerGrid, peParent) { this.name = name; #if DEBUG for (int n = 0;n < childGridEntries.Length; n++) { Debug.Assert(childGridEntries[n] != null, "Null item in category subproperty list"); } #endif if (categoryStates == null) { categoryStates = new Hashtable(); } lock (categoryStates) { if (!categoryStates.ContainsKey(name)) { categoryStates.Add(name, true); } } this.IsExpandable = true; for (int i = 0; i < childGridEntries.Length; i++) { childGridEntries[i].ParentGridEntry = this; } this.ChildCollection = new GridEntryCollection(this, childGridEntries); lock (categoryStates) { this.InternalExpanded = (bool)categoryStates[name]; } this.SetFlag(GridEntry.FLAG_LABEL_BOLD,true); } ////// /// Returns true if this GridEntry has a value field in the right hand column. /// internal override bool HasValue { get { return false; } } protected override void Dispose(bool disposing) { if (disposing) { if (backBrush != null) { backBrush.Dispose(); backBrush = null; } if (ChildCollection != null) { ChildCollection = null; } } base.Dispose(disposing); } public override void DisposeChildren() { // categories should never dispose // return; } // we don't want this guy participating in property depth. public override int PropertyDepth { get { return base.PropertyDepth - 1; } } protected override Brush GetBackgroundBrush(Graphics g) { return this.GridEntryHost.GetLineBrush(g); } protected override Color LabelTextColor { get { return ownerGrid.CategoryForeColor; } } public override bool Expandable { get { return !GetFlagSet(FL_EXPANDABLE_FAILED); } } internal override bool InternalExpanded { set { base.InternalExpanded = value; lock (categoryStates) { categoryStates[this.name] = value; } } } public override GridItemType GridItemType { get { return GridItemType.Category; } } public override string HelpKeyword { get { return null; } } public override string PropertyLabel { get { return name; } } internal override int PropertyLabelIndent { get { // we give an extra pixel for breathing room // we want to make sure that we return 0 for property depth here instead of PropertyGridView gridHost = this.GridEntryHost; // we call base.PropertyDepth here because we don't want the subratction to happen. return 1+gridHost.GetOutlineIconSize()+OUTLINE_ICON_PADDING + (base.PropertyDepth * gridHost.GetDefaultOutlineIndent()); } } public override string GetPropertyTextValue(object o) { return ""; } public override Type PropertyType { get { return typeof(void); } } ////// /// Gets the owner of the current value. This is usually the value of the /// root entry, which is the object being browsed /// public override object GetChildValueOwner(GridEntry childEntry) { return ParentGridEntry.GetChildValueOwner(childEntry); } protected override bool CreateChildren(bool diffOldChildren) { return true; } public override string GetTestingInfo() { string str = "object = ("; str += FullLabel; str += "), Category = (" + this.PropertyLabel + ")"; return str; } public override void PaintLabel(System.Drawing.Graphics g, Rectangle rect, Rectangle clipRect, bool selected, bool paintFullLabel) { base.PaintLabel(g, rect, clipRect, false, true); // now draw the focus rect if (selected && hasFocus) { bool bold = ((this.Flags & GridEntry.FLAG_LABEL_BOLD) != 0); Font font = GetFont(bold); int labelWidth = GetLabelTextWidth(this.PropertyLabel, g, font); int indent = PropertyLabelIndent-2; Rectangle focusRect = new Rectangle(indent, rect.Y, labelWidth+3, rect.Height-1); ControlPaint.DrawFocusRectangle(g, focusRect); } // draw the line along the top if (parentPE.GetChildIndex(this) > 0) { g.DrawLine(SystemPens.Control, rect.X-1, rect.Y-1, rect.Width+2, rect.Y - 1); } } #if PAINT_CATEGORY_TRIANGLE private const double TRI_HEIGHT_RATIO = 2.5; private static readonly double TRI_WIDTH_RATIO = .8; #endif public override void PaintOutline(System.Drawing.Graphics g, Rectangle r) { // draw outline pointer triangle. if (Expandable) { bool fExpanded = Expanded; Rectangle outline = OutlineRect; // make sure we're in our bounds outline = Rectangle.Intersect(r, outline); if (outline.IsEmpty) { return; } #if PAINT_CATEGORY_TRIANGLE // bump it over a pixel //outline.Offset(1, 0); //outline.Inflate(-1,-1); // build the triangle, an equalaterial centered around the midpoint of the rect. Point[] points = new Point[3]; int borderWidth = 2; // width is always the length of the sides of the triangle. // Height = (width /2 * (Cos60)) ; Cos60 ~ .86 int triWidth, triHeight; int xOffset, yOffset; if (!fExpanded) { // draw arrow pointing right, remember height is pointing right // 0 // |\ // | \2 // | / // |/ // 1 triWidth = (int)((outline.Height * TRI_WIDTH_RATIO) - (2*borderWidth)); // make sure it's an odd width so our lines will match up if (!(triWidth % 2 == 0)) { triWidth++; } triHeight = (int)Math.Ceil((triWidth/2) * TRI_HEIGHT_RATIO); yOffset = outline.Y + (outline.Height-triWidth)/2; xOffset = outline.X + (outline.Width-triHeight)/2; points[0] = new Point(xOffset, yOffset); points[1] = new Point(xOffset, yOffset + triWidth); points[2] = new Point(xOffset+triHeight, yOffset + (triWidth / 2)); } else { // draw arrow pointing down // 0 -------- 1 // \ / // \ / // \ / // \/ // 2 triWidth = (int)((outline.Width * TRI_WIDTH_RATIO) - (2*borderWidth)); // make sure it's an odd width so our lines will match up if (!(triWidth % 2 == 0)) { triWidth++; } triHeight = (int)Math.Ceil((triWidth/2) * TRI_HEIGHT_RATIO); xOffset = outline.X + (outline.Width-triWidth)/2; yOffset = outline.Y + (outline.Height-triHeight)/2; points[0] = new Point(xOffset, yOffset); points[1] = new Point(xOffset + triWidth, yOffset); points[2] = new Point(xOffset + (triWidth/ 2),yOffset + triHeight); } g.FillPolygon(SystemPens.WindowText, points); #else // draw border area box Brush b; Pen p; bool disposeBrush = false; bool disposePen = false; Color color = GridEntryHost.GetLineColor(); b = new SolidBrush(g.GetNearestColor(color)); disposeBrush = true; color = GridEntryHost.GetTextColor(); p = new Pen(g.GetNearestColor(color)); disposePen = true; g.FillRectangle(b, outline); g.DrawRectangle(p, outline.X, outline.Y, outline.Width - 1, outline.Height - 1); // draw horizontal line for +/- int indent = 2; g.DrawLine(SystemPens.WindowText, outline.X + indent,outline.Y + outline.Height / 2, outline.X + outline.Width - indent - 1,outline.Y + outline.Height/2); // draw vertical line to make a + if (!fExpanded) g.DrawLine(SystemPens.WindowText, outline.X + outline.Width/2, outline.Y + indent, outline.X + outline.Width/2, outline.Y + outline.Height - indent - 1); if (disposePen) p.Dispose(); if (disposeBrush) b.Dispose(); #endif } } public override void PaintValue(object val, System.Drawing.Graphics g, Rectangle rect, Rectangle clipRect, PaintValueFlags paintFlags) { base.PaintValue(val, g, rect, clipRect, paintFlags & ~PaintValueFlags.DrawSelected); // draw the line along the top if (parentPE.GetChildIndex(this) > 0) { g.DrawLine(SystemPens.Control, rect.X-2, rect.Y-1,rect.Width+1, rect.Y-1); } } internal override bool NotifyChildValue(GridEntry pe, int type) { return parentPE.NotifyChildValue(pe, type); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- //#define PAINT_CATEGORY_TRIANGLE /* */ namespace System.Windows.Forms.PropertyGridInternal { using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Collections; using System.Reflection; using System.ComponentModel; using System.ComponentModel.Design; using System.Windows.Forms; using System.Drawing; using Microsoft.Win32; internal class CategoryGridEntry : GridEntry { internal string name; private Brush backBrush = null; private static Hashtable categoryStates = null; [ SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors") // GridEntry classes are internal so we have complete // control over who does what in the constructor. ] public CategoryGridEntry(PropertyGrid ownerGrid, GridEntry peParent,string name, GridEntry[] childGridEntries) : base(ownerGrid, peParent) { this.name = name; #if DEBUG for (int n = 0;n < childGridEntries.Length; n++) { Debug.Assert(childGridEntries[n] != null, "Null item in category subproperty list"); } #endif if (categoryStates == null) { categoryStates = new Hashtable(); } lock (categoryStates) { if (!categoryStates.ContainsKey(name)) { categoryStates.Add(name, true); } } this.IsExpandable = true; for (int i = 0; i < childGridEntries.Length; i++) { childGridEntries[i].ParentGridEntry = this; } this.ChildCollection = new GridEntryCollection(this, childGridEntries); lock (categoryStates) { this.InternalExpanded = (bool)categoryStates[name]; } this.SetFlag(GridEntry.FLAG_LABEL_BOLD,true); } ////// /// Returns true if this GridEntry has a value field in the right hand column. /// internal override bool HasValue { get { return false; } } protected override void Dispose(bool disposing) { if (disposing) { if (backBrush != null) { backBrush.Dispose(); backBrush = null; } if (ChildCollection != null) { ChildCollection = null; } } base.Dispose(disposing); } public override void DisposeChildren() { // categories should never dispose // return; } // we don't want this guy participating in property depth. public override int PropertyDepth { get { return base.PropertyDepth - 1; } } protected override Brush GetBackgroundBrush(Graphics g) { return this.GridEntryHost.GetLineBrush(g); } protected override Color LabelTextColor { get { return ownerGrid.CategoryForeColor; } } public override bool Expandable { get { return !GetFlagSet(FL_EXPANDABLE_FAILED); } } internal override bool InternalExpanded { set { base.InternalExpanded = value; lock (categoryStates) { categoryStates[this.name] = value; } } } public override GridItemType GridItemType { get { return GridItemType.Category; } } public override string HelpKeyword { get { return null; } } public override string PropertyLabel { get { return name; } } internal override int PropertyLabelIndent { get { // we give an extra pixel for breathing room // we want to make sure that we return 0 for property depth here instead of PropertyGridView gridHost = this.GridEntryHost; // we call base.PropertyDepth here because we don't want the subratction to happen. return 1+gridHost.GetOutlineIconSize()+OUTLINE_ICON_PADDING + (base.PropertyDepth * gridHost.GetDefaultOutlineIndent()); } } public override string GetPropertyTextValue(object o) { return ""; } public override Type PropertyType { get { return typeof(void); } } ////// /// Gets the owner of the current value. This is usually the value of the /// root entry, which is the object being browsed /// public override object GetChildValueOwner(GridEntry childEntry) { return ParentGridEntry.GetChildValueOwner(childEntry); } protected override bool CreateChildren(bool diffOldChildren) { return true; } public override string GetTestingInfo() { string str = "object = ("; str += FullLabel; str += "), Category = (" + this.PropertyLabel + ")"; return str; } public override void PaintLabel(System.Drawing.Graphics g, Rectangle rect, Rectangle clipRect, bool selected, bool paintFullLabel) { base.PaintLabel(g, rect, clipRect, false, true); // now draw the focus rect if (selected && hasFocus) { bool bold = ((this.Flags & GridEntry.FLAG_LABEL_BOLD) != 0); Font font = GetFont(bold); int labelWidth = GetLabelTextWidth(this.PropertyLabel, g, font); int indent = PropertyLabelIndent-2; Rectangle focusRect = new Rectangle(indent, rect.Y, labelWidth+3, rect.Height-1); ControlPaint.DrawFocusRectangle(g, focusRect); } // draw the line along the top if (parentPE.GetChildIndex(this) > 0) { g.DrawLine(SystemPens.Control, rect.X-1, rect.Y-1, rect.Width+2, rect.Y - 1); } } #if PAINT_CATEGORY_TRIANGLE private const double TRI_HEIGHT_RATIO = 2.5; private static readonly double TRI_WIDTH_RATIO = .8; #endif public override void PaintOutline(System.Drawing.Graphics g, Rectangle r) { // draw outline pointer triangle. if (Expandable) { bool fExpanded = Expanded; Rectangle outline = OutlineRect; // make sure we're in our bounds outline = Rectangle.Intersect(r, outline); if (outline.IsEmpty) { return; } #if PAINT_CATEGORY_TRIANGLE // bump it over a pixel //outline.Offset(1, 0); //outline.Inflate(-1,-1); // build the triangle, an equalaterial centered around the midpoint of the rect. Point[] points = new Point[3]; int borderWidth = 2; // width is always the length of the sides of the triangle. // Height = (width /2 * (Cos60)) ; Cos60 ~ .86 int triWidth, triHeight; int xOffset, yOffset; if (!fExpanded) { // draw arrow pointing right, remember height is pointing right // 0 // |\ // | \2 // | / // |/ // 1 triWidth = (int)((outline.Height * TRI_WIDTH_RATIO) - (2*borderWidth)); // make sure it's an odd width so our lines will match up if (!(triWidth % 2 == 0)) { triWidth++; } triHeight = (int)Math.Ceil((triWidth/2) * TRI_HEIGHT_RATIO); yOffset = outline.Y + (outline.Height-triWidth)/2; xOffset = outline.X + (outline.Width-triHeight)/2; points[0] = new Point(xOffset, yOffset); points[1] = new Point(xOffset, yOffset + triWidth); points[2] = new Point(xOffset+triHeight, yOffset + (triWidth / 2)); } else { // draw arrow pointing down // 0 -------- 1 // \ / // \ / // \ / // \/ // 2 triWidth = (int)((outline.Width * TRI_WIDTH_RATIO) - (2*borderWidth)); // make sure it's an odd width so our lines will match up if (!(triWidth % 2 == 0)) { triWidth++; } triHeight = (int)Math.Ceil((triWidth/2) * TRI_HEIGHT_RATIO); xOffset = outline.X + (outline.Width-triWidth)/2; yOffset = outline.Y + (outline.Height-triHeight)/2; points[0] = new Point(xOffset, yOffset); points[1] = new Point(xOffset + triWidth, yOffset); points[2] = new Point(xOffset + (triWidth/ 2),yOffset + triHeight); } g.FillPolygon(SystemPens.WindowText, points); #else // draw border area box Brush b; Pen p; bool disposeBrush = false; bool disposePen = false; Color color = GridEntryHost.GetLineColor(); b = new SolidBrush(g.GetNearestColor(color)); disposeBrush = true; color = GridEntryHost.GetTextColor(); p = new Pen(g.GetNearestColor(color)); disposePen = true; g.FillRectangle(b, outline); g.DrawRectangle(p, outline.X, outline.Y, outline.Width - 1, outline.Height - 1); // draw horizontal line for +/- int indent = 2; g.DrawLine(SystemPens.WindowText, outline.X + indent,outline.Y + outline.Height / 2, outline.X + outline.Width - indent - 1,outline.Y + outline.Height/2); // draw vertical line to make a + if (!fExpanded) g.DrawLine(SystemPens.WindowText, outline.X + outline.Width/2, outline.Y + indent, outline.X + outline.Width/2, outline.Y + outline.Height - indent - 1); if (disposePen) p.Dispose(); if (disposeBrush) b.Dispose(); #endif } } public override void PaintValue(object val, System.Drawing.Graphics g, Rectangle rect, Rectangle clipRect, PaintValueFlags paintFlags) { base.PaintValue(val, g, rect, clipRect, paintFlags & ~PaintValueFlags.DrawSelected); // draw the line along the top if (parentPE.GetChildIndex(this) > 0) { g.DrawLine(SystemPens.Control, rect.X-2, rect.Y-1,rect.Width+1, rect.Y-1); } } internal override bool NotifyChildValue(GridEntry pe, int type) { return parentPE.NotifyChildValue(pe, type); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
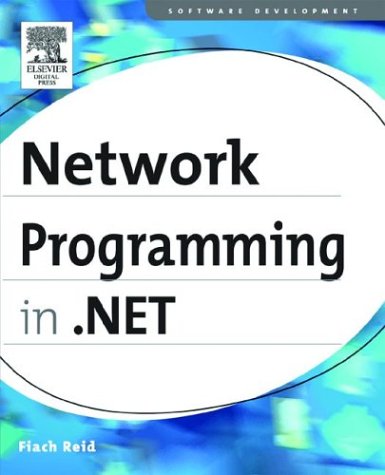
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- QilStrConcatenator.cs
- KeyEvent.cs
- DoubleCollectionValueSerializer.cs
- MouseDevice.cs
- ExpandableObjectConverter.cs
- AsyncResult.cs
- AttachedPropertyDescriptor.cs
- XmlQualifiedName.cs
- EmptyElement.cs
- DescriptionAttribute.cs
- AstNode.cs
- HybridDictionary.cs
- InternalBufferManager.cs
- DrawingBrush.cs
- PasswordBoxAutomationPeer.cs
- IConvertible.cs
- ProfileSettingsCollection.cs
- CheckedListBox.cs
- LoadMessageLogger.cs
- GradientSpreadMethodValidation.cs
- CreateUserWizard.cs
- DataQuery.cs
- DbBuffer.cs
- LongTypeConverter.cs
- HyperLink.cs
- Viewport3DVisual.cs
- FileDialogCustomPlaces.cs
- OciHandle.cs
- SHA256.cs
- DesignerOptions.cs
- Token.cs
- DrawingCollection.cs
- UnsafeNativeMethods.cs
- Int64AnimationBase.cs
- ApplicationServicesHostFactory.cs
- HwndMouseInputProvider.cs
- CompoundFileIOPermission.cs
- AdornerLayer.cs
- StubHelpers.cs
- HttpDebugHandler.cs
- MultiPageTextView.cs
- EntityRecordInfo.cs
- MediaContext.cs
- Int64Animation.cs
- CleanUpVirtualizedItemEventArgs.cs
- InfoCardTrace.cs
- CanonicalFontFamilyReference.cs
- ExpressionBindingCollection.cs
- COAUTHINFO.cs
- TraceRecord.cs
- _DigestClient.cs
- HttpRuntime.cs
- EventOpcode.cs
- SqlDataSourceConnectionPanel.cs
- SizeValueSerializer.cs
- PhotoPrintingIntent.cs
- AuthenticatingEventArgs.cs
- RequestCachePolicy.cs
- DrawingGroup.cs
- AuthorizationRuleCollection.cs
- SoapCommonClasses.cs
- Clause.cs
- CompModSwitches.cs
- CheckBoxStandardAdapter.cs
- MailAddress.cs
- XpsFilter.cs
- DataGridViewTextBoxCell.cs
- ReversePositionQuery.cs
- SingleAnimationBase.cs
- UriPrefixTable.cs
- SqlUserDefinedAggregateAttribute.cs
- DodSequenceMerge.cs
- Function.cs
- WaitHandleCannotBeOpenedException.cs
- serverconfig.cs
- AsymmetricSignatureDeformatter.cs
- DependencyPropertyValueSerializer.cs
- MenuCommands.cs
- DataGridViewCellStyle.cs
- StringUtil.cs
- SecurityChannelListener.cs
- PresentationAppDomainManager.cs
- UniqueIdentifierService.cs
- RequiredAttributeAttribute.cs
- DetailsViewRow.cs
- FormViewPagerRow.cs
- ScriptControlManager.cs
- PtsContext.cs
- XmlChoiceIdentifierAttribute.cs
- StandardBindingReliableSessionElement.cs
- EndpointIdentityConverter.cs
- ArgumentOutOfRangeException.cs
- PointAnimationUsingPath.cs
- DbConnectionFactory.cs
- ForeignConstraint.cs
- XmlValueConverter.cs
- DataGridViewRow.cs
- DataTableCollection.cs
- __TransparentProxy.cs
- SiblingIterators.cs