Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / xsp / System / Web / Extensions / ui / ScriptControlManager.cs / 1 / ScriptControlManager.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections.ObjectModel; using System.Collections.Generic; using System.Globalization; using System.Text; using System.Web.UI; using System.Web.Resources; using System.Web.Util; using Debug = System.Diagnostics.Debug; internal class ScriptControlManager { private OrderedDictionary> _extenderControls; private bool _pagePreRenderRaised; private OrderedDictionary _scriptControls; private ScriptManager _scriptManager; private bool _scriptReferencesRegistered; public ScriptControlManager(ScriptManager scriptManager) { _scriptManager = scriptManager; } private OrderedDictionary > ExtenderControls { get { if (_extenderControls == null) { _extenderControls = new OrderedDictionary >(); } return _extenderControls; } } private OrderedDictionary ScriptControls { get { if (_scriptControls == null) { _scriptControls = new OrderedDictionary (); } return _scriptControls; } } public void AddScriptReferences(List scriptReferences) { #if DEBUG if (_scriptReferencesRegistered) { Debug.Fail("AddScriptReferences should only be called once per request but it was already called during this request."); } #endif AddScriptReferencesForScriptControls(scriptReferences); AddScriptReferencesForExtenderControls(scriptReferences); _scriptReferencesRegistered = true; } private void AddScriptReferencesForScriptControls(List scriptReferences) { // PERF: Use field directly to avoid creating Dictionary if not already created if (_scriptControls != null) { foreach (IScriptControl scriptControl in _scriptControls.Keys) { AddScriptReferenceForScriptControl(scriptReferences, scriptControl); } } } private static void AddScriptReferenceForScriptControl(List scriptReferences, IScriptControl scriptControl) { IEnumerable scriptControlReferences = scriptControl.GetScriptReferences(); if (scriptControlReferences != null) { Control scriptControlAsControl = (Control)scriptControl; ClientUrlResolverWrapper urlResolverWrapper = null; foreach (ScriptReference scriptControlReference in scriptControlReferences) { if (scriptControlReference != null) { if (urlResolverWrapper == null) { urlResolverWrapper = new ClientUrlResolverWrapper(scriptControlAsControl); } // set containing control on each script reference for client url resolution scriptControlReference.ClientUrlResolver = urlResolverWrapper; scriptControlReference.IsStaticReference = false; scriptControlReference.ContainingControl = scriptControlAsControl; // add to collection of all references scriptReferences.Add(scriptControlReference); } } } } private void AddScriptReferencesForExtenderControls(List scriptReferences) { // PERF: Use field directly to avoid creating Dictionary if not already created if (_extenderControls != null) { foreach (IExtenderControl extenderControl in _extenderControls.Keys) { AddScriptReferenceForExtenderControl(scriptReferences, extenderControl); } } } private static void AddScriptReferenceForExtenderControl(List scriptReferences, IExtenderControl extenderControl) { IEnumerable extenderControlReferences = extenderControl.GetScriptReferences(); if (extenderControlReferences != null) { Control extenderControlAsControl = (Control)extenderControl; ClientUrlResolverWrapper urlResolverWrapper = null; foreach (ScriptReference extenderControlReference in extenderControlReferences) { if (extenderControlReference != null) { if (urlResolverWrapper == null) { urlResolverWrapper = new ClientUrlResolverWrapper(extenderControlAsControl); } // set containing control on each script reference for client url resolution extenderControlReference.ClientUrlResolver = urlResolverWrapper; extenderControlReference.IsStaticReference = false; extenderControlReference.ContainingControl = extenderControlAsControl; // add to collection of all references scriptReferences.Add(extenderControlReference); } } } } private bool InControlTree(Control targetControl) { for (Control parent = targetControl.Parent; parent != null; parent = parent.Parent) { if (parent == _scriptManager.Page) { return true; } } return false; } public void OnPagePreRender(object sender, EventArgs e) { _pagePreRenderRaised = true; } public void RegisterExtenderControl (TExtenderControl extenderControl, Control targetControl) where TExtenderControl : Control, IExtenderControl { if (extenderControl == null) { throw new ArgumentNullException("extenderControl"); } if (targetControl == null) { throw new ArgumentNullException("targetControl"); } VerifyTargetControlType(extenderControl, targetControl); if (!_pagePreRenderRaised) { throw new InvalidOperationException(AtlasWeb.ScriptControlManager_RegisterExtenderControlTooEarly); } if (_scriptReferencesRegistered) { throw new InvalidOperationException(AtlasWeb.ScriptControlManager_RegisterExtenderControlTooLate); } // A single ExtenderControl may theoretically be registered multiple times List targetControls; if (!ExtenderControls.TryGetValue(extenderControl, out targetControls)) { targetControls = new List (); ExtenderControls[extenderControl] = targetControls; } targetControls.Add(targetControl); } public void RegisterScriptControl (TScriptControl scriptControl) where TScriptControl : Control, IScriptControl { if (scriptControl == null) { throw new ArgumentNullException("scriptControl"); } if (!_pagePreRenderRaised) { throw new InvalidOperationException(AtlasWeb.ScriptControlManager_RegisterScriptControlTooEarly); } if (_scriptReferencesRegistered) { throw new InvalidOperationException(AtlasWeb.ScriptControlManager_RegisterScriptControlTooLate); } // A single ScriptControl may theoretically be registered multiple times int timesRegistered; ScriptControls.TryGetValue(scriptControl, out timesRegistered); timesRegistered++; ScriptControls[scriptControl] = timesRegistered; } public void RegisterScriptDescriptors(IExtenderControl extenderControl) { if (extenderControl == null) { throw new ArgumentNullException("extenderControl"); } Control extenderControlAsControl = extenderControl as Control; if (extenderControlAsControl == null) { throw new ArgumentException( String.Format(CultureInfo.InvariantCulture, AtlasWeb.Common_ArgumentInvalidType, typeof(Control).FullName), "extenderControl"); } List targetControls; if (!ExtenderControls.TryGetValue(extenderControl, out targetControls)) { throw new ArgumentException( String.Format(CultureInfo.InvariantCulture, AtlasWeb.ScriptControlManager_ExtenderControlNotRegistered, extenderControlAsControl.ID), "extenderControl"); } Debug.Assert(targetControls != null && targetControls.Count > 0); // A single ExtenderControl may theoretically be registered multiple times foreach (Control targetControl in targetControls) { // Only register ExtenderControl scripts if the target control is visible and in the control tree. // Else, we assume the target was not rendered. if (targetControl.Visible && InControlTree(targetControl)) { IEnumerable scriptDescriptors = extenderControl.GetScriptDescriptors(targetControl); RegisterScriptsForScriptDescriptors(scriptDescriptors, extenderControlAsControl); } } } public void RegisterScriptDescriptors(IScriptControl scriptControl) { if (scriptControl == null) { throw new ArgumentNullException("scriptControl"); } Control scriptControlAsControl = scriptControl as Control; if (scriptControlAsControl == null) { throw new ArgumentException( String.Format(CultureInfo.InvariantCulture, AtlasWeb.Common_ArgumentInvalidType, typeof(Control).FullName), "scriptControl"); } // Verify that ScriptControl was previously registered int timesRegistered; if (!ScriptControls.TryGetValue(scriptControl, out timesRegistered)) { throw new ArgumentException( String.Format(CultureInfo.InvariantCulture, AtlasWeb.ScriptControlManager_ScriptControlNotRegistered, scriptControlAsControl.ID), "scriptControl"); } // A single ScriptControl may theoretically be registered multiple times for (int i = 0; i < timesRegistered; i++) { IEnumerable scriptDescriptors = scriptControl.GetScriptDescriptors(); RegisterScriptsForScriptDescriptors(scriptDescriptors, scriptControlAsControl); } } private void RegisterScriptsForScriptDescriptors(IEnumerable scriptDescriptors, Control control) { if (scriptDescriptors != null) { StringBuilder initBuilder = null; foreach (ScriptDescriptor scriptDescriptor in scriptDescriptors) { if (scriptDescriptor != null) { if (initBuilder == null) { initBuilder = new StringBuilder(); initBuilder.AppendLine("Sys.Application.add_init(function() {"); } initBuilder.Append(" "); initBuilder.AppendLine(scriptDescriptor.GetScript()); // Call into the descriptor to possibly register dispose functionality for async posts scriptDescriptor.RegisterDisposeForDescriptor(_scriptManager, control); } } // If scriptDescriptors enumeration is empty, we don't want to register any script. if (initBuilder != null) { initBuilder.AppendLine("});"); string initScript = initBuilder.ToString(); // DevDiv 35243: Do not use the script itself as the key, since different controls could // possibly register the exact same script, or the same control may want to register the // same script more than once. // Generate a unique script key for every registration. string initScriptKey = _scriptManager.CreateUniqueScriptKey(); _scriptManager.RegisterStartupScriptInternal( control, typeof(ScriptManager), initScriptKey, initScript, true); } } } private static void VerifyTargetControlType ( TExtenderControl extenderControl, Control targetControl) where TExtenderControl : Control, IExtenderControl { Type extenderControlType = extenderControl.GetType(); // Use TargetControlTypeCache instead of directly calling Type.GetCustomAttributes(). // Increases requests/second by nearly 100% in ScriptControlScenario.aspx test. Type[] types = TargetControlTypeCache.GetTargetControlTypes(extenderControlType); if (types.Length == 0) { throw new InvalidOperationException(String.Format(CultureInfo.InvariantCulture, AtlasWeb.ScriptControlManager_NoTargetControlTypes, extenderControlType, typeof(TargetControlTypeAttribute))); } Type targetControlType = targetControl.GetType(); foreach (Type type in types) { if (type.IsAssignableFrom(targetControlType)) { return; } } throw new InvalidOperationException(String.Format(CultureInfo.InvariantCulture, AtlasWeb.ScriptControlManager_TargetControlTypeInvalid, extenderControl.ID, targetControl.ID, extenderControlType, targetControlType)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI { using System; using System.Collections.ObjectModel; using System.Collections.Generic; using System.Globalization; using System.Text; using System.Web.UI; using System.Web.Resources; using System.Web.Util; using Debug = System.Diagnostics.Debug; internal class ScriptControlManager { private OrderedDictionary> _extenderControls; private bool _pagePreRenderRaised; private OrderedDictionary _scriptControls; private ScriptManager _scriptManager; private bool _scriptReferencesRegistered; public ScriptControlManager(ScriptManager scriptManager) { _scriptManager = scriptManager; } private OrderedDictionary > ExtenderControls { get { if (_extenderControls == null) { _extenderControls = new OrderedDictionary >(); } return _extenderControls; } } private OrderedDictionary ScriptControls { get { if (_scriptControls == null) { _scriptControls = new OrderedDictionary (); } return _scriptControls; } } public void AddScriptReferences(List scriptReferences) { #if DEBUG if (_scriptReferencesRegistered) { Debug.Fail("AddScriptReferences should only be called once per request but it was already called during this request."); } #endif AddScriptReferencesForScriptControls(scriptReferences); AddScriptReferencesForExtenderControls(scriptReferences); _scriptReferencesRegistered = true; } private void AddScriptReferencesForScriptControls(List scriptReferences) { // PERF: Use field directly to avoid creating Dictionary if not already created if (_scriptControls != null) { foreach (IScriptControl scriptControl in _scriptControls.Keys) { AddScriptReferenceForScriptControl(scriptReferences, scriptControl); } } } private static void AddScriptReferenceForScriptControl(List scriptReferences, IScriptControl scriptControl) { IEnumerable scriptControlReferences = scriptControl.GetScriptReferences(); if (scriptControlReferences != null) { Control scriptControlAsControl = (Control)scriptControl; ClientUrlResolverWrapper urlResolverWrapper = null; foreach (ScriptReference scriptControlReference in scriptControlReferences) { if (scriptControlReference != null) { if (urlResolverWrapper == null) { urlResolverWrapper = new ClientUrlResolverWrapper(scriptControlAsControl); } // set containing control on each script reference for client url resolution scriptControlReference.ClientUrlResolver = urlResolverWrapper; scriptControlReference.IsStaticReference = false; scriptControlReference.ContainingControl = scriptControlAsControl; // add to collection of all references scriptReferences.Add(scriptControlReference); } } } } private void AddScriptReferencesForExtenderControls(List scriptReferences) { // PERF: Use field directly to avoid creating Dictionary if not already created if (_extenderControls != null) { foreach (IExtenderControl extenderControl in _extenderControls.Keys) { AddScriptReferenceForExtenderControl(scriptReferences, extenderControl); } } } private static void AddScriptReferenceForExtenderControl(List scriptReferences, IExtenderControl extenderControl) { IEnumerable extenderControlReferences = extenderControl.GetScriptReferences(); if (extenderControlReferences != null) { Control extenderControlAsControl = (Control)extenderControl; ClientUrlResolverWrapper urlResolverWrapper = null; foreach (ScriptReference extenderControlReference in extenderControlReferences) { if (extenderControlReference != null) { if (urlResolverWrapper == null) { urlResolverWrapper = new ClientUrlResolverWrapper(extenderControlAsControl); } // set containing control on each script reference for client url resolution extenderControlReference.ClientUrlResolver = urlResolverWrapper; extenderControlReference.IsStaticReference = false; extenderControlReference.ContainingControl = extenderControlAsControl; // add to collection of all references scriptReferences.Add(extenderControlReference); } } } } private bool InControlTree(Control targetControl) { for (Control parent = targetControl.Parent; parent != null; parent = parent.Parent) { if (parent == _scriptManager.Page) { return true; } } return false; } public void OnPagePreRender(object sender, EventArgs e) { _pagePreRenderRaised = true; } public void RegisterExtenderControl (TExtenderControl extenderControl, Control targetControl) where TExtenderControl : Control, IExtenderControl { if (extenderControl == null) { throw new ArgumentNullException("extenderControl"); } if (targetControl == null) { throw new ArgumentNullException("targetControl"); } VerifyTargetControlType(extenderControl, targetControl); if (!_pagePreRenderRaised) { throw new InvalidOperationException(AtlasWeb.ScriptControlManager_RegisterExtenderControlTooEarly); } if (_scriptReferencesRegistered) { throw new InvalidOperationException(AtlasWeb.ScriptControlManager_RegisterExtenderControlTooLate); } // A single ExtenderControl may theoretically be registered multiple times List targetControls; if (!ExtenderControls.TryGetValue(extenderControl, out targetControls)) { targetControls = new List (); ExtenderControls[extenderControl] = targetControls; } targetControls.Add(targetControl); } public void RegisterScriptControl (TScriptControl scriptControl) where TScriptControl : Control, IScriptControl { if (scriptControl == null) { throw new ArgumentNullException("scriptControl"); } if (!_pagePreRenderRaised) { throw new InvalidOperationException(AtlasWeb.ScriptControlManager_RegisterScriptControlTooEarly); } if (_scriptReferencesRegistered) { throw new InvalidOperationException(AtlasWeb.ScriptControlManager_RegisterScriptControlTooLate); } // A single ScriptControl may theoretically be registered multiple times int timesRegistered; ScriptControls.TryGetValue(scriptControl, out timesRegistered); timesRegistered++; ScriptControls[scriptControl] = timesRegistered; } public void RegisterScriptDescriptors(IExtenderControl extenderControl) { if (extenderControl == null) { throw new ArgumentNullException("extenderControl"); } Control extenderControlAsControl = extenderControl as Control; if (extenderControlAsControl == null) { throw new ArgumentException( String.Format(CultureInfo.InvariantCulture, AtlasWeb.Common_ArgumentInvalidType, typeof(Control).FullName), "extenderControl"); } List targetControls; if (!ExtenderControls.TryGetValue(extenderControl, out targetControls)) { throw new ArgumentException( String.Format(CultureInfo.InvariantCulture, AtlasWeb.ScriptControlManager_ExtenderControlNotRegistered, extenderControlAsControl.ID), "extenderControl"); } Debug.Assert(targetControls != null && targetControls.Count > 0); // A single ExtenderControl may theoretically be registered multiple times foreach (Control targetControl in targetControls) { // Only register ExtenderControl scripts if the target control is visible and in the control tree. // Else, we assume the target was not rendered. if (targetControl.Visible && InControlTree(targetControl)) { IEnumerable scriptDescriptors = extenderControl.GetScriptDescriptors(targetControl); RegisterScriptsForScriptDescriptors(scriptDescriptors, extenderControlAsControl); } } } public void RegisterScriptDescriptors(IScriptControl scriptControl) { if (scriptControl == null) { throw new ArgumentNullException("scriptControl"); } Control scriptControlAsControl = scriptControl as Control; if (scriptControlAsControl == null) { throw new ArgumentException( String.Format(CultureInfo.InvariantCulture, AtlasWeb.Common_ArgumentInvalidType, typeof(Control).FullName), "scriptControl"); } // Verify that ScriptControl was previously registered int timesRegistered; if (!ScriptControls.TryGetValue(scriptControl, out timesRegistered)) { throw new ArgumentException( String.Format(CultureInfo.InvariantCulture, AtlasWeb.ScriptControlManager_ScriptControlNotRegistered, scriptControlAsControl.ID), "scriptControl"); } // A single ScriptControl may theoretically be registered multiple times for (int i = 0; i < timesRegistered; i++) { IEnumerable scriptDescriptors = scriptControl.GetScriptDescriptors(); RegisterScriptsForScriptDescriptors(scriptDescriptors, scriptControlAsControl); } } private void RegisterScriptsForScriptDescriptors(IEnumerable scriptDescriptors, Control control) { if (scriptDescriptors != null) { StringBuilder initBuilder = null; foreach (ScriptDescriptor scriptDescriptor in scriptDescriptors) { if (scriptDescriptor != null) { if (initBuilder == null) { initBuilder = new StringBuilder(); initBuilder.AppendLine("Sys.Application.add_init(function() {"); } initBuilder.Append(" "); initBuilder.AppendLine(scriptDescriptor.GetScript()); // Call into the descriptor to possibly register dispose functionality for async posts scriptDescriptor.RegisterDisposeForDescriptor(_scriptManager, control); } } // If scriptDescriptors enumeration is empty, we don't want to register any script. if (initBuilder != null) { initBuilder.AppendLine("});"); string initScript = initBuilder.ToString(); // DevDiv 35243: Do not use the script itself as the key, since different controls could // possibly register the exact same script, or the same control may want to register the // same script more than once. // Generate a unique script key for every registration. string initScriptKey = _scriptManager.CreateUniqueScriptKey(); _scriptManager.RegisterStartupScriptInternal( control, typeof(ScriptManager), initScriptKey, initScript, true); } } } private static void VerifyTargetControlType ( TExtenderControl extenderControl, Control targetControl) where TExtenderControl : Control, IExtenderControl { Type extenderControlType = extenderControl.GetType(); // Use TargetControlTypeCache instead of directly calling Type.GetCustomAttributes(). // Increases requests/second by nearly 100% in ScriptControlScenario.aspx test. Type[] types = TargetControlTypeCache.GetTargetControlTypes(extenderControlType); if (types.Length == 0) { throw new InvalidOperationException(String.Format(CultureInfo.InvariantCulture, AtlasWeb.ScriptControlManager_NoTargetControlTypes, extenderControlType, typeof(TargetControlTypeAttribute))); } Type targetControlType = targetControl.GetType(); foreach (Type type in types) { if (type.IsAssignableFrom(targetControlType)) { return; } } throw new InvalidOperationException(String.Format(CultureInfo.InvariantCulture, AtlasWeb.ScriptControlManager_TargetControlTypeInvalid, extenderControl.ID, targetControl.ID, extenderControlType, targetControlType)); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
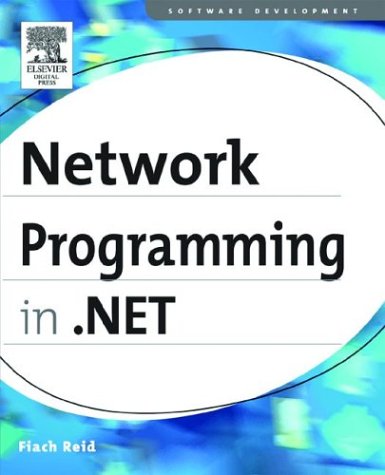
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeVariableDeclarationStatement.cs
- WindowsSysHeader.cs
- NavigationService.cs
- SimpleLine.cs
- BindingCompleteEventArgs.cs
- BaseCAMarshaler.cs
- ReadOnlyDataSourceView.cs
- ShutDownListener.cs
- BamlReader.cs
- Light.cs
- TypedTableHandler.cs
- TreeNode.cs
- Material.cs
- JulianCalendar.cs
- TransformValueSerializer.cs
- CodeTypeParameterCollection.cs
- ChildChangedEventArgs.cs
- LineInfo.cs
- MobilePage.cs
- CodeTypeReferenceExpression.cs
- ThreadAttributes.cs
- EventlogProvider.cs
- WebExceptionStatus.cs
- ResourcePermissionBaseEntry.cs
- XmlSchemaAttributeGroupRef.cs
- GetReadStreamResult.cs
- HierarchicalDataSourceDesigner.cs
- ComPlusTraceRecord.cs
- XmlILAnnotation.cs
- DataGridViewHeaderCell.cs
- MasterPageBuildProvider.cs
- SSmlParser.cs
- ObservableDictionary.cs
- JsonByteArrayDataContract.cs
- CommandCollectionEditor.cs
- MemberMaps.cs
- CreateUserWizardDesigner.cs
- ByteKeyFrameCollection.cs
- httpstaticobjectscollection.cs
- PanelContainerDesigner.cs
- MatchAttribute.cs
- MinMaxParagraphWidth.cs
- HttpPostedFile.cs
- ClientOptions.cs
- WebPartEventArgs.cs
- RadioButtonList.cs
- precedingquery.cs
- EventBuilder.cs
- XmlSchema.cs
- TrimSurroundingWhitespaceAttribute.cs
- SystemUnicastIPAddressInformation.cs
- HttpCachePolicyWrapper.cs
- ConfigXmlDocument.cs
- SerializationStore.cs
- NamedServiceModelExtensionCollectionElement.cs
- AdapterUtil.cs
- PropertyGeneratedEventArgs.cs
- CheckBoxRenderer.cs
- Animatable.cs
- PersonalizationProviderCollection.cs
- SecurityChannelListener.cs
- ApplicationServiceHelper.cs
- NativeActivityContext.cs
- LinkedList.cs
- TemplateBamlRecordReader.cs
- AxHostDesigner.cs
- future.cs
- Vector3DConverter.cs
- Relationship.cs
- KeyProperty.cs
- MatrixConverter.cs
- DelegatingConfigHost.cs
- ListViewContainer.cs
- ObjectSet.cs
- ProtectedConfigurationSection.cs
- AddingNewEventArgs.cs
- SignatureGenerator.cs
- DataControlPagerLinkButton.cs
- AppDomainManager.cs
- AlphabeticalEnumConverter.cs
- Inflater.cs
- PolyBezierSegment.cs
- InputQueue.cs
- DataTemplateSelector.cs
- BasicHttpBinding.cs
- SystemIcmpV4Statistics.cs
- GradientBrush.cs
- XMLDiffLoader.cs
- XsltArgumentList.cs
- HideDisabledControlAdapter.cs
- GridViewEditEventArgs.cs
- LineGeometry.cs
- JsonCollectionDataContract.cs
- MultipartContentParser.cs
- BitVector32.cs
- XmlSchemaInclude.cs
- ConsumerConnectionPointCollection.cs
- Int16.cs
- XmlStringTable.cs
- Hashtable.cs