Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Configuration / System / Configuration / ProtectedConfigurationSection.cs / 1 / ProtectedConfigurationSection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Collections; using System.Collections.Specialized; using System.Xml; using System.Globalization; using System.Security.Permissions; public sealed class ProtectedConfigurationSection : ConfigurationSection { internal ProtectedConfigurationProvider GetProviderFromName(string providerName) { ProviderSettings ps = Providers[providerName]; if (ps == null) { throw new Exception(SR.GetString(SR.ProtectedConfigurationProvider_not_found, providerName)); } return InstantiateProvider(ps); } internal ProtectedConfigurationProviderCollection GetAllProviders() { ProtectedConfigurationProviderCollection coll = new ProtectedConfigurationProviderCollection(); foreach(ProviderSettings ps in Providers) { coll.Add(InstantiateProvider(ps)); } return coll; } [PermissionSet(SecurityAction.Assert, Unrestricted=true)] private ProtectedConfigurationProvider CreateAndInitializeProviderWithAssert(Type t, ProviderSettings pn) { ProtectedConfigurationProvider provider = (ProtectedConfigurationProvider)TypeUtil.CreateInstanceWithReflectionPermission(t); NameValueCollection pars = pn.Parameters; NameValueCollection cloneParams = new NameValueCollection(pars.Count); foreach (string key in pars) { cloneParams[key] = pars[key]; } provider.Initialize(pn.Name, cloneParams); return provider; } private ProtectedConfigurationProvider InstantiateProvider(ProviderSettings pn) { Type t = TypeUtil.GetTypeWithReflectionPermission(pn.Type, true); if (!typeof(ProtectedConfigurationProvider).IsAssignableFrom(t)) { throw new Exception(SR.GetString(SR.WrongType_of_Protected_provider)); } // Needs to check APTCA bit. See VSWhidbey 429996. if (!TypeUtil.IsTypeAllowedInConfig(t)) { throw new Exception(SR.GetString(SR.Type_from_untrusted_assembly, t.FullName)); } // Needs to check Assert Fulltrust in order for runtime to work. See VSWhidbey 429996. return CreateAndInitializeProviderWithAssert(t, pn); } internal static string DecryptSection(string encryptedXml, ProtectedConfigurationProvider provider) { XmlDocument doc = new XmlDocument(); doc.LoadXml(encryptedXml); XmlNode resultNode = provider.Decrypt(doc.DocumentElement); return resultNode.OuterXml; } private const string EncryptedSectionTemplate = "<{0} {1}=\"{2}\"> {3} {0}>"; internal static string FormatEncryptedSection(string encryptedXml, string sectionName, string providerName) { return String.Format(CultureInfo.InvariantCulture, EncryptedSectionTemplate, sectionName, // The section to encrypt BaseConfigurationRecord.KEYWORD_PROTECTION_PROVIDER, // protectionProvider keyword providerName, // The provider name encryptedXml // the encrypted xml ); } internal static string EncryptSection(string clearXml, ProtectedConfigurationProvider provider) { XmlDocument xmlDocument = new XmlDocument(); xmlDocument.PreserveWhitespace = true; xmlDocument.LoadXml(clearXml); string sectionName = xmlDocument.DocumentElement.Name; XmlNode encNode = provider.Encrypt(xmlDocument.DocumentElement); return encNode.OuterXml; } ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propProviders = new ConfigurationProperty("providers", typeof(ProtectedProviderSettings), new ProtectedProviderSettings(), ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propDefaultProvider = new ConfigurationProperty("defaultProvider", typeof(string), "RsaProtectedConfigurationProvider", null, ConfigurationProperty.NonEmptyStringValidator, ConfigurationPropertyOptions.None); static ProtectedConfigurationSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propProviders); _properties.Add(_propDefaultProvider); } public ProtectedConfigurationSection() { } protected internal override ConfigurationPropertyCollection Properties { get { return _properties; } } private ProtectedProviderSettings _Providers { get { return (ProtectedProviderSettings)base[_propProviders]; } } [ConfigurationProperty("providers")] public ProviderSettingsCollection Providers { get { return _Providers.Providers; } } [ConfigurationProperty("defaultProvider", DefaultValue = "RsaProtectedConfigurationProvider")] public string DefaultProvider { get { return (string)base[_propDefaultProvider]; } set { base[_propDefaultProvider] = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Collections; using System.Collections.Specialized; using System.Xml; using System.Globalization; using System.Security.Permissions; public sealed class ProtectedConfigurationSection : ConfigurationSection { internal ProtectedConfigurationProvider GetProviderFromName(string providerName) { ProviderSettings ps = Providers[providerName]; if (ps == null) { throw new Exception(SR.GetString(SR.ProtectedConfigurationProvider_not_found, providerName)); } return InstantiateProvider(ps); } internal ProtectedConfigurationProviderCollection GetAllProviders() { ProtectedConfigurationProviderCollection coll = new ProtectedConfigurationProviderCollection(); foreach(ProviderSettings ps in Providers) { coll.Add(InstantiateProvider(ps)); } return coll; } [PermissionSet(SecurityAction.Assert, Unrestricted=true)] private ProtectedConfigurationProvider CreateAndInitializeProviderWithAssert(Type t, ProviderSettings pn) { ProtectedConfigurationProvider provider = (ProtectedConfigurationProvider)TypeUtil.CreateInstanceWithReflectionPermission(t); NameValueCollection pars = pn.Parameters; NameValueCollection cloneParams = new NameValueCollection(pars.Count); foreach (string key in pars) { cloneParams[key] = pars[key]; } provider.Initialize(pn.Name, cloneParams); return provider; } private ProtectedConfigurationProvider InstantiateProvider(ProviderSettings pn) { Type t = TypeUtil.GetTypeWithReflectionPermission(pn.Type, true); if (!typeof(ProtectedConfigurationProvider).IsAssignableFrom(t)) { throw new Exception(SR.GetString(SR.WrongType_of_Protected_provider)); } // Needs to check APTCA bit. See VSWhidbey 429996. if (!TypeUtil.IsTypeAllowedInConfig(t)) { throw new Exception(SR.GetString(SR.Type_from_untrusted_assembly, t.FullName)); } // Needs to check Assert Fulltrust in order for runtime to work. See VSWhidbey 429996. return CreateAndInitializeProviderWithAssert(t, pn); } internal static string DecryptSection(string encryptedXml, ProtectedConfigurationProvider provider) { XmlDocument doc = new XmlDocument(); doc.LoadXml(encryptedXml); XmlNode resultNode = provider.Decrypt(doc.DocumentElement); return resultNode.OuterXml; } private const string EncryptedSectionTemplate = "<{0} {1}=\"{2}\"> {3} {0}>"; internal static string FormatEncryptedSection(string encryptedXml, string sectionName, string providerName) { return String.Format(CultureInfo.InvariantCulture, EncryptedSectionTemplate, sectionName, // The section to encrypt BaseConfigurationRecord.KEYWORD_PROTECTION_PROVIDER, // protectionProvider keyword providerName, // The provider name encryptedXml // the encrypted xml ); } internal static string EncryptSection(string clearXml, ProtectedConfigurationProvider provider) { XmlDocument xmlDocument = new XmlDocument(); xmlDocument.PreserveWhitespace = true; xmlDocument.LoadXml(clearXml); string sectionName = xmlDocument.DocumentElement.Name; XmlNode encNode = provider.Encrypt(xmlDocument.DocumentElement); return encNode.OuterXml; } ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// ////////////////////////////////////////////////////////////////////// private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propProviders = new ConfigurationProperty("providers", typeof(ProtectedProviderSettings), new ProtectedProviderSettings(), ConfigurationPropertyOptions.None); private static readonly ConfigurationProperty _propDefaultProvider = new ConfigurationProperty("defaultProvider", typeof(string), "RsaProtectedConfigurationProvider", null, ConfigurationProperty.NonEmptyStringValidator, ConfigurationPropertyOptions.None); static ProtectedConfigurationSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propProviders); _properties.Add(_propDefaultProvider); } public ProtectedConfigurationSection() { } protected internal override ConfigurationPropertyCollection Properties { get { return _properties; } } private ProtectedProviderSettings _Providers { get { return (ProtectedProviderSettings)base[_propProviders]; } } [ConfigurationProperty("providers")] public ProviderSettingsCollection Providers { get { return _Providers.Providers; } } [ConfigurationProperty("defaultProvider", DefaultValue = "RsaProtectedConfigurationProvider")] public string DefaultProvider { get { return (string)base[_propDefaultProvider]; } set { base[_propDefaultProvider] = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
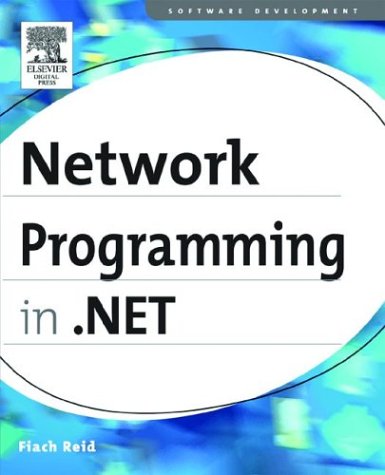
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- GridSplitterAutomationPeer.cs
- CompositionAdorner.cs
- WebUtil.cs
- SingleConverter.cs
- SqlDependencyListener.cs
- ForEachAction.cs
- UDPClient.cs
- ContentElement.cs
- HtmlSelect.cs
- PeerInputChannelListener.cs
- FileFormatException.cs
- SocketAddress.cs
- GregorianCalendar.cs
- DefaultEvaluationContext.cs
- UnsafeNativeMethods.cs
- LexicalChunk.cs
- SafeFileMapViewHandle.cs
- ElementProxy.cs
- Trace.cs
- LinqToSqlWrapper.cs
- NativeMethods.cs
- SerializationException.cs
- OptimizedTemplateContent.cs
- QilPatternFactory.cs
- TemplatePartAttribute.cs
- WorkflowRuntimeSection.cs
- ADRoleFactory.cs
- Unit.cs
- __FastResourceComparer.cs
- SystemIPInterfaceProperties.cs
- SqlDataReaderSmi.cs
- WizardStepBase.cs
- URLString.cs
- UnmanagedMarshal.cs
- ListViewAutomationPeer.cs
- UserValidatedEventArgs.cs
- HttpChannelBindingToken.cs
- StreamReader.cs
- DayRenderEvent.cs
- EntityCollection.cs
- EntityDataSourceChangingEventArgs.cs
- ObjectSet.cs
- Span.cs
- Delegate.cs
- FilterElement.cs
- DataQuery.cs
- printdlgexmarshaler.cs
- InvariantComparer.cs
- ByteConverter.cs
- XmlSchemaAppInfo.cs
- CleanUpVirtualizedItemEventArgs.cs
- addressfiltermode.cs
- SafeNativeMethods.cs
- WebResourceAttribute.cs
- RadioButtonDesigner.cs
- XmlSchemaGroup.cs
- ResourceReader.cs
- DeploymentSection.cs
- DropTarget.cs
- EasingQuaternionKeyFrame.cs
- GenericTypeParameterBuilder.cs
- OleDbException.cs
- OLEDB_Util.cs
- Expander.cs
- SymLanguageVendor.cs
- NonParentingControl.cs
- SymmetricSecurityProtocolFactory.cs
- util.cs
- RolePrincipal.cs
- TemplateField.cs
- SemanticAnalyzer.cs
- Imaging.cs
- InfiniteTimeSpanConverter.cs
- InternalsVisibleToAttribute.cs
- CannotUnloadAppDomainException.cs
- WebBrowserNavigatingEventHandler.cs
- DelimitedListTraceListener.cs
- odbcmetadatacollectionnames.cs
- TextParagraph.cs
- DEREncoding.cs
- MethodBody.cs
- MasterPage.cs
- DBSchemaTable.cs
- ConvertBinder.cs
- DataExpression.cs
- Bitmap.cs
- UriExt.cs
- SynchronizationLockException.cs
- DataGridViewSortCompareEventArgs.cs
- SecurityDescriptor.cs
- ConfigurationSectionCollection.cs
- RsaSecurityTokenAuthenticator.cs
- OrderByBuilder.cs
- clipboard.cs
- PropertyValueUIItem.cs
- TearOffProxy.cs
- PromptStyle.cs
- XmlSchemaAttributeGroup.cs
- StringFunctions.cs
- HttpHandlerActionCollection.cs