Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Base / System / Windows / DependencyPropertyChangedEventArgs.cs / 2 / DependencyPropertyChangedEventArgs.cs
using System; using MS.Internal.WindowsBase; // FriendAccessAllowed namespace System.Windows { ////// Provides data for the various property changed events. /// public struct DependencyPropertyChangedEventArgs { #region Constructors ////// Initializes a new instance of the DependencyPropertyChangedEventArgs class. /// /// /// The property whose value changed. /// /// /// The value of the property before the change. /// /// /// The value of the property after the change. /// public DependencyPropertyChangedEventArgs(DependencyProperty property, object oldValue, object newValue) { _property = property; _metadata = null; _oldEntry = new EffectiveValueEntry(property); _newEntry = _oldEntry; _oldEntry.Value = oldValue; _newEntry.Value = newValue; _flags = 0; _operationType = OperationType.Unknown; IsAValueChange = true; } [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal DependencyPropertyChangedEventArgs(DependencyProperty property, PropertyMetadata metadata, object oldValue, object newValue) { _property = property; _metadata = metadata; _oldEntry = new EffectiveValueEntry(property); _newEntry = _oldEntry; _oldEntry.Value = oldValue; _newEntry.Value = newValue; _flags = 0; _operationType = OperationType.Unknown; IsAValueChange = true; } internal DependencyPropertyChangedEventArgs(DependencyProperty property, PropertyMetadata metadata, object value) { _property = property; _metadata = metadata; _oldEntry = new EffectiveValueEntry(property); _oldEntry.Value = value; _newEntry = _oldEntry; _flags = 0; _operationType = OperationType.Unknown; IsASubPropertyChange = true; } internal DependencyPropertyChangedEventArgs( DependencyProperty property, PropertyMetadata metadata, bool isAValueChange, EffectiveValueEntry oldEntry, EffectiveValueEntry newEntry, OperationType operationType) { _property = property; _metadata = metadata; _oldEntry = oldEntry; _newEntry = newEntry; _flags = 0; _operationType = operationType; IsAValueChange = isAValueChange; // This is when a mutable default is promoted to a local value. On this operation mutable default // value acquires a freezable context. However this value promotion operation is triggered // whenever there has been a sub property change to the mutable default. Eg. Adding a TextEffect // to a TextEffectCollection instance which is the mutable default. Since we missed the sub property // change due to this add, we flip the IsASubPropertyChange bit on the following change caused by // the value promotion to coalesce these operations. IsASubPropertyChange = (operationType == OperationType.ChangeMutableDefaultValue); } #endregion Constructors #region Properties ////// The property whose value changed. /// public DependencyProperty Property { get { return _property; } } ////// Whether or not this change indicates a change to the property value /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal bool IsAValueChange { get { return ReadPrivateFlag(PrivateFlags.IsAValueChange); } set { WritePrivateFlag(PrivateFlags.IsAValueChange, value); } } ////// Whether or not this change indicates a change to the subproperty /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal bool IsASubPropertyChange { get { return ReadPrivateFlag(PrivateFlags.IsASubPropertyChange); } set { WritePrivateFlag(PrivateFlags.IsASubPropertyChange, value); } } ////// Metadata for the property /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal PropertyMetadata Metadata { get { return _metadata; } } ////// Says what operation caused this property change /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal OperationType OperationType { get { return _operationType; } } ////// The old value of the property. /// public object OldValue { get { EffectiveValueEntry oldEntry = OldEntry.GetFlattenedEntry(RequestFlags.FullyResolved); if (oldEntry.IsDeferredReference) { // The value for this property was meant to come from a dictionary // and the creation of that value had been deferred until this // time for better performance. Now is the time to actually instantiate // this value by querying it from the dictionary. Once we have the // value we can actually replace the deferred reference marker // with the actual value. oldEntry.Value = ((DeferredReference) oldEntry.Value).GetValue(oldEntry.BaseValueSourceInternal); oldEntry.IsDeferredReference = false; } return oldEntry.Value; } } ////// The entry for the old value (contains value and all modifier info) /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal EffectiveValueEntry OldEntry { get { return _oldEntry; } } ////// The source of the old value /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal BaseValueSourceInternal OldValueSource { get { return _oldEntry.BaseValueSourceInternal; } } ////// Says if the old value was a modified value (coerced, animated, expression) /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal bool IsOldValueModified { get { return _oldEntry.HasModifiers; } } ////// Says if the old value was a deferred value /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal bool IsOldValueDeferred { get { return _oldEntry.IsDeferredReference; } } ////// The new value of the property. /// public object NewValue { get { EffectiveValueEntry newEntry = NewEntry.GetFlattenedEntry(RequestFlags.FullyResolved); if (newEntry.IsDeferredReference) { // The value for this property was meant to come from a dictionary // and the creation of that value had been deferred until this // time for better performance. Now is the time to actually instantiate // this value by querying it from the dictionary. Once we have the // value we can actually replace the deferred reference marker // with the actual value. newEntry.Value = ((DeferredReference) newEntry.Value).GetValue(newEntry.BaseValueSourceInternal); newEntry.IsDeferredReference = false; } return newEntry.Value; } } ////// The entry for the new value (contains value and all modifier info) /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal EffectiveValueEntry NewEntry { get { return _newEntry; } } ////// The source of the new value /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal BaseValueSourceInternal NewValueSource { get { return _newEntry.BaseValueSourceInternal; } } ////// Says if the new value was a modified value (coerced, animated, expression) /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal bool IsNewValueModified { get { return _newEntry.HasModifiers; } } ////// Says if the new value was a deferred value /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal bool IsNewValueDeferred { get { return _newEntry.IsDeferredReference; } } #endregion Properties ////// public override int GetHashCode() { return base.GetHashCode(); } ////// public override bool Equals(object obj) { return Equals((DependencyPropertyChangedEventArgs)obj); } ////// public bool Equals(DependencyPropertyChangedEventArgs args) { return (_property == args._property && _metadata == args._metadata && _oldEntry.Value == args._oldEntry.Value && _newEntry.Value == args._newEntry.Value && _flags == args._flags && _oldEntry.BaseValueSourceInternal == args._oldEntry.BaseValueSourceInternal && _newEntry.BaseValueSourceInternal == args._newEntry.BaseValueSourceInternal && _oldEntry.HasModifiers == args._oldEntry.HasModifiers && _newEntry.HasModifiers == args._newEntry.HasModifiers && _oldEntry.IsDeferredReference == args._oldEntry.IsDeferredReference && _newEntry.IsDeferredReference == args._newEntry.IsDeferredReference && _operationType == args._operationType); } ////// public static bool operator ==(DependencyPropertyChangedEventArgs left, DependencyPropertyChangedEventArgs right) { return left.Equals(right); } ////// public static bool operator !=(DependencyPropertyChangedEventArgs left, DependencyPropertyChangedEventArgs right) { return !left.Equals(right); } #region PrivateMethods private void WritePrivateFlag(PrivateFlags bit, bool value) { if (value) { _flags |= bit; } else { _flags &= ~bit; } } private bool ReadPrivateFlag(PrivateFlags bit) { return (_flags & bit) != 0; } #endregion PrivateMethods #region PrivateDataStructures private enum PrivateFlags : byte { IsAValueChange = 0x01, IsASubPropertyChange = 0x02, } #endregion PrivateDataStructures #region Data private DependencyProperty _property; private PropertyMetadata _metadata; private PrivateFlags _flags; private EffectiveValueEntry _oldEntry; private EffectiveValueEntry _newEntry; private OperationType _operationType; #endregion Data } [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal enum OperationType : byte { Unknown = 0, AddChild = 1, RemoveChild = 2, Inherit = 3, ChangeMutableDefaultValue = 4, } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using MS.Internal.WindowsBase; // FriendAccessAllowed namespace System.Windows { ////// Provides data for the various property changed events. /// public struct DependencyPropertyChangedEventArgs { #region Constructors ////// Initializes a new instance of the DependencyPropertyChangedEventArgs class. /// /// /// The property whose value changed. /// /// /// The value of the property before the change. /// /// /// The value of the property after the change. /// public DependencyPropertyChangedEventArgs(DependencyProperty property, object oldValue, object newValue) { _property = property; _metadata = null; _oldEntry = new EffectiveValueEntry(property); _newEntry = _oldEntry; _oldEntry.Value = oldValue; _newEntry.Value = newValue; _flags = 0; _operationType = OperationType.Unknown; IsAValueChange = true; } [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal DependencyPropertyChangedEventArgs(DependencyProperty property, PropertyMetadata metadata, object oldValue, object newValue) { _property = property; _metadata = metadata; _oldEntry = new EffectiveValueEntry(property); _newEntry = _oldEntry; _oldEntry.Value = oldValue; _newEntry.Value = newValue; _flags = 0; _operationType = OperationType.Unknown; IsAValueChange = true; } internal DependencyPropertyChangedEventArgs(DependencyProperty property, PropertyMetadata metadata, object value) { _property = property; _metadata = metadata; _oldEntry = new EffectiveValueEntry(property); _oldEntry.Value = value; _newEntry = _oldEntry; _flags = 0; _operationType = OperationType.Unknown; IsASubPropertyChange = true; } internal DependencyPropertyChangedEventArgs( DependencyProperty property, PropertyMetadata metadata, bool isAValueChange, EffectiveValueEntry oldEntry, EffectiveValueEntry newEntry, OperationType operationType) { _property = property; _metadata = metadata; _oldEntry = oldEntry; _newEntry = newEntry; _flags = 0; _operationType = operationType; IsAValueChange = isAValueChange; // This is when a mutable default is promoted to a local value. On this operation mutable default // value acquires a freezable context. However this value promotion operation is triggered // whenever there has been a sub property change to the mutable default. Eg. Adding a TextEffect // to a TextEffectCollection instance which is the mutable default. Since we missed the sub property // change due to this add, we flip the IsASubPropertyChange bit on the following change caused by // the value promotion to coalesce these operations. IsASubPropertyChange = (operationType == OperationType.ChangeMutableDefaultValue); } #endregion Constructors #region Properties ////// The property whose value changed. /// public DependencyProperty Property { get { return _property; } } ////// Whether or not this change indicates a change to the property value /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal bool IsAValueChange { get { return ReadPrivateFlag(PrivateFlags.IsAValueChange); } set { WritePrivateFlag(PrivateFlags.IsAValueChange, value); } } ////// Whether or not this change indicates a change to the subproperty /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal bool IsASubPropertyChange { get { return ReadPrivateFlag(PrivateFlags.IsASubPropertyChange); } set { WritePrivateFlag(PrivateFlags.IsASubPropertyChange, value); } } ////// Metadata for the property /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal PropertyMetadata Metadata { get { return _metadata; } } ////// Says what operation caused this property change /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal OperationType OperationType { get { return _operationType; } } ////// The old value of the property. /// public object OldValue { get { EffectiveValueEntry oldEntry = OldEntry.GetFlattenedEntry(RequestFlags.FullyResolved); if (oldEntry.IsDeferredReference) { // The value for this property was meant to come from a dictionary // and the creation of that value had been deferred until this // time for better performance. Now is the time to actually instantiate // this value by querying it from the dictionary. Once we have the // value we can actually replace the deferred reference marker // with the actual value. oldEntry.Value = ((DeferredReference) oldEntry.Value).GetValue(oldEntry.BaseValueSourceInternal); oldEntry.IsDeferredReference = false; } return oldEntry.Value; } } ////// The entry for the old value (contains value and all modifier info) /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal EffectiveValueEntry OldEntry { get { return _oldEntry; } } ////// The source of the old value /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal BaseValueSourceInternal OldValueSource { get { return _oldEntry.BaseValueSourceInternal; } } ////// Says if the old value was a modified value (coerced, animated, expression) /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal bool IsOldValueModified { get { return _oldEntry.HasModifiers; } } ////// Says if the old value was a deferred value /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal bool IsOldValueDeferred { get { return _oldEntry.IsDeferredReference; } } ////// The new value of the property. /// public object NewValue { get { EffectiveValueEntry newEntry = NewEntry.GetFlattenedEntry(RequestFlags.FullyResolved); if (newEntry.IsDeferredReference) { // The value for this property was meant to come from a dictionary // and the creation of that value had been deferred until this // time for better performance. Now is the time to actually instantiate // this value by querying it from the dictionary. Once we have the // value we can actually replace the deferred reference marker // with the actual value. newEntry.Value = ((DeferredReference) newEntry.Value).GetValue(newEntry.BaseValueSourceInternal); newEntry.IsDeferredReference = false; } return newEntry.Value; } } ////// The entry for the new value (contains value and all modifier info) /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal EffectiveValueEntry NewEntry { get { return _newEntry; } } ////// The source of the new value /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal BaseValueSourceInternal NewValueSource { get { return _newEntry.BaseValueSourceInternal; } } ////// Says if the new value was a modified value (coerced, animated, expression) /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal bool IsNewValueModified { get { return _newEntry.HasModifiers; } } ////// Says if the new value was a deferred value /// [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal bool IsNewValueDeferred { get { return _newEntry.IsDeferredReference; } } #endregion Properties ////// public override int GetHashCode() { return base.GetHashCode(); } ////// public override bool Equals(object obj) { return Equals((DependencyPropertyChangedEventArgs)obj); } ////// public bool Equals(DependencyPropertyChangedEventArgs args) { return (_property == args._property && _metadata == args._metadata && _oldEntry.Value == args._oldEntry.Value && _newEntry.Value == args._newEntry.Value && _flags == args._flags && _oldEntry.BaseValueSourceInternal == args._oldEntry.BaseValueSourceInternal && _newEntry.BaseValueSourceInternal == args._newEntry.BaseValueSourceInternal && _oldEntry.HasModifiers == args._oldEntry.HasModifiers && _newEntry.HasModifiers == args._newEntry.HasModifiers && _oldEntry.IsDeferredReference == args._oldEntry.IsDeferredReference && _newEntry.IsDeferredReference == args._newEntry.IsDeferredReference && _operationType == args._operationType); } ////// public static bool operator ==(DependencyPropertyChangedEventArgs left, DependencyPropertyChangedEventArgs right) { return left.Equals(right); } ////// public static bool operator !=(DependencyPropertyChangedEventArgs left, DependencyPropertyChangedEventArgs right) { return !left.Equals(right); } #region PrivateMethods private void WritePrivateFlag(PrivateFlags bit, bool value) { if (value) { _flags |= bit; } else { _flags &= ~bit; } } private bool ReadPrivateFlag(PrivateFlags bit) { return (_flags & bit) != 0; } #endregion PrivateMethods #region PrivateDataStructures private enum PrivateFlags : byte { IsAValueChange = 0x01, IsASubPropertyChange = 0x02, } #endregion PrivateDataStructures #region Data private DependencyProperty _property; private PropertyMetadata _metadata; private PrivateFlags _flags; private EffectiveValueEntry _oldEntry; private EffectiveValueEntry _newEntry; private OperationType _operationType; #endregion Data } [FriendAccessAllowed] // Built into Base, also used by Core & Framework. internal enum OperationType : byte { Unknown = 0, AddChild = 1, RemoveChild = 2, Inherit = 3, ChangeMutableDefaultValue = 4, } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
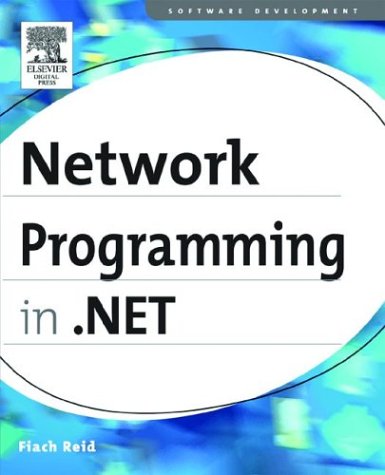
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PeerNameRecordCollection.cs
- GridViewDeleteEventArgs.cs
- OdbcException.cs
- EditingMode.cs
- ColorComboBox.cs
- ListBindableAttribute.cs
- Group.cs
- ProcessingInstructionAction.cs
- DecoratedNameAttribute.cs
- SerializationTrace.cs
- RelationshipSet.cs
- DataTable.cs
- ControlHelper.cs
- XmlDocument.cs
- TextServicesHost.cs
- NamespaceInfo.cs
- EtwTrace.cs
- IISUnsafeMethods.cs
- FamilyTypefaceCollection.cs
- TimeEnumHelper.cs
- UnsafeNativeMethods.cs
- TaskFileService.cs
- ProviderConnectionPointCollection.cs
- DBSqlParserTableCollection.cs
- ping.cs
- BitmapData.cs
- WebScriptMetadataMessage.cs
- FullTextState.cs
- ModuleBuilder.cs
- InvalidPropValue.cs
- ScriptBehaviorDescriptor.cs
- TextSelection.cs
- PointAnimationUsingKeyFrames.cs
- EntityDataSourceEntityTypeFilterItem.cs
- CqlErrorHelper.cs
- FormatterServicesNoSerializableCheck.cs
- GridView.cs
- DesignerInterfaces.cs
- EpmContentSerializer.cs
- IconHelper.cs
- safesecurityhelperavalon.cs
- SoundPlayerAction.cs
- MsmqOutputChannel.cs
- ReadOnlyCollection.cs
- CacheDependency.cs
- PersistenceTypeAttribute.cs
- CompilerCollection.cs
- PrintDocument.cs
- RepeatBehaviorConverter.cs
- Encoder.cs
- SettingsBindableAttribute.cs
- IsolatedStorage.cs
- EFTableProvider.cs
- Compress.cs
- HyperLinkDataBindingHandler.cs
- HierarchicalDataBoundControl.cs
- DataServiceProcessingPipeline.cs
- LayoutEvent.cs
- ScriptIgnoreAttribute.cs
- log.cs
- SpeakInfo.cs
- PageCache.cs
- OrderedDictionary.cs
- ArraySegment.cs
- Hashtable.cs
- ListControlActionList.cs
- CompiledQueryCacheEntry.cs
- UnsafeNativeMethodsPenimc.cs
- HGlobalSafeHandle.cs
- ListViewPagedDataSource.cs
- Rectangle.cs
- SelectionService.cs
- ScrollBar.cs
- AnonymousIdentificationSection.cs
- TabControlEvent.cs
- OdbcCommand.cs
- BulletedList.cs
- TableParagraph.cs
- FileLogRecordStream.cs
- TogglePatternIdentifiers.cs
- TargetException.cs
- TabControlCancelEvent.cs
- FileUpload.cs
- QuaternionConverter.cs
- PeerNameRegistration.cs
- DataMisalignedException.cs
- ByteAnimationUsingKeyFrames.cs
- XpsFilter.cs
- RequestQueryProcessor.cs
- Executor.cs
- RefreshPropertiesAttribute.cs
- SoapSchemaImporter.cs
- RotateTransform3D.cs
- SchemaImporterExtension.cs
- WebPartsSection.cs
- EntityDataSourceContextCreatedEventArgs.cs
- PageAsyncTask.cs
- MetabaseServerConfig.cs
- CollectionsUtil.cs
- DefinitionUpdate.cs