Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / BCL / System / ArraySegment.cs / 1 / ArraySegment.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: ArraySegment** ** ** Purpose: Convenient wrapper for an array, an offset, and ** a count. Ideally used in streams & collections. ** Net Classes will consume an array of these. ** ** ===========================================================*/ using System.Runtime.InteropServices; namespace System { [Serializable] public struct ArraySegment { private T[] _array; private int _offset; private int _count; public ArraySegment(T[] array) { if (array == null) throw new ArgumentNullException("array"); _array = array; _offset = 0; _count = array.Length; } public ArraySegment(T[] array, int offset, int count) { if (array == null) throw new ArgumentNullException("array"); if (offset < 0) throw new ArgumentOutOfRangeException("offset", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (count < 0) throw new ArgumentOutOfRangeException("count", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (array.Length - offset < count) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidOffLen")); _array = array; _offset = offset; _count = count; } public T[] Array { get { return _array; } } public int Offset { get { return _offset; } } public int Count { get { return _count; } } public override int GetHashCode() { return _array.GetHashCode() ^ _offset ^ _count; } public override bool Equals(Object obj) { if (obj is ArraySegment ) return Equals((ArraySegment )obj); else return false; } public bool Equals(ArraySegment obj) { return obj._array == _array && obj._offset == _offset && obj._count == _count; } public static bool operator ==(ArraySegment a, ArraySegment b) { return a.Equals(b); } public static bool operator !=(ArraySegment a, ArraySegment b) { return !(a == b); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: ArraySegment ** ** ** Purpose: Convenient wrapper for an array, an offset, and ** a count. Ideally used in streams & collections. ** Net Classes will consume an array of these. ** ** ===========================================================*/ using System.Runtime.InteropServices; namespace System { [Serializable] public struct ArraySegment { private T[] _array; private int _offset; private int _count; public ArraySegment(T[] array) { if (array == null) throw new ArgumentNullException("array"); _array = array; _offset = 0; _count = array.Length; } public ArraySegment(T[] array, int offset, int count) { if (array == null) throw new ArgumentNullException("array"); if (offset < 0) throw new ArgumentOutOfRangeException("offset", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (count < 0) throw new ArgumentOutOfRangeException("count", Environment.GetResourceString("ArgumentOutOfRange_NeedNonNegNum")); if (array.Length - offset < count) throw new ArgumentException(Environment.GetResourceString("Argument_InvalidOffLen")); _array = array; _offset = offset; _count = count; } public T[] Array { get { return _array; } } public int Offset { get { return _offset; } } public int Count { get { return _count; } } public override int GetHashCode() { return _array.GetHashCode() ^ _offset ^ _count; } public override bool Equals(Object obj) { if (obj is ArraySegment ) return Equals((ArraySegment )obj); else return false; } public bool Equals(ArraySegment obj) { return obj._array == _array && obj._offset == _offset && obj._count == _count; } public static bool operator ==(ArraySegment a, ArraySegment b) { return a.Equals(b); } public static bool operator !=(ArraySegment a, ArraySegment b) { return !(a == b); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
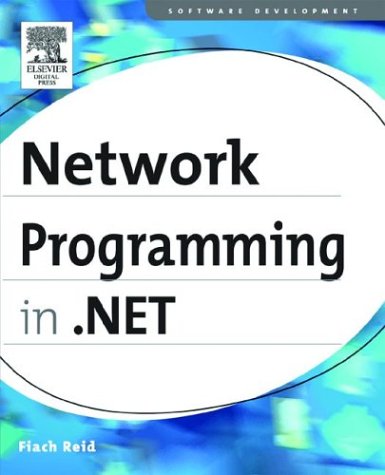
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TaiwanLunisolarCalendar.cs
- PlainXmlSerializer.cs
- CharEnumerator.cs
- DataBinder.cs
- BigIntegerStorage.cs
- FontDriver.cs
- sqlmetadatafactory.cs
- PropertyStore.cs
- Cursors.cs
- MessageSmuggler.cs
- FastEncoderStatics.cs
- NullableDoubleMinMaxAggregationOperator.cs
- Propagator.Evaluator.cs
- SystemUnicastIPAddressInformation.cs
- DataGridViewCellCancelEventArgs.cs
- SHA1.cs
- KeyValueConfigurationCollection.cs
- MethodBuilder.cs
- SecurityPolicySection.cs
- NativeMethods.cs
- Rotation3DAnimationBase.cs
- PointLight.cs
- EventlogProvider.cs
- CssTextWriter.cs
- FusionWrap.cs
- SwitchElementsCollection.cs
- ThreadExceptionEvent.cs
- QuotaExceededException.cs
- StringInfo.cs
- PreloadedPackages.cs
- InternalSafeNativeMethods.cs
- ImageButton.cs
- XamlWrappingReader.cs
- HtmlTextBoxAdapter.cs
- ResourceManager.cs
- ProfileManager.cs
- PipelineComponent.cs
- LoadRetryStrategyFactory.cs
- MissingMemberException.cs
- ControlBuilderAttribute.cs
- WindowsGraphics2.cs
- PathSegmentCollection.cs
- ReplacementText.cs
- SendMessageContent.cs
- CodeRegionDirective.cs
- CodeExpressionStatement.cs
- SimpleApplicationHost.cs
- AuthStoreRoleProvider.cs
- HtmlDocument.cs
- TdsParserSessionPool.cs
- TargetException.cs
- LingerOption.cs
- KeyInstance.cs
- PartialArray.cs
- SqlCharStream.cs
- HttpCachePolicy.cs
- MeasureData.cs
- Line.cs
- AutoFocusStyle.xaml.cs
- TextEditorTables.cs
- SiteIdentityPermission.cs
- DSASignatureFormatter.cs
- CodeDomConfigurationHandler.cs
- GradientSpreadMethodValidation.cs
- EntityParameter.cs
- CommandHelpers.cs
- JsonXmlDataContract.cs
- TypeToStringValueConverter.cs
- TypeConverterAttribute.cs
- ClrPerspective.cs
- TileBrush.cs
- HtmlSelect.cs
- TextTabProperties.cs
- ObjectDataSourceView.cs
- TextDecorationLocationValidation.cs
- EntityProviderFactory.cs
- AsyncResult.cs
- IDictionary.cs
- SchemaNamespaceManager.cs
- ComponentDispatcher.cs
- SimpleHandlerBuildProvider.cs
- SystemSounds.cs
- LocalFileSettingsProvider.cs
- GenericsInstances.cs
- MobileResource.cs
- TransactionContextValidator.cs
- StagingAreaInputItem.cs
- AsyncSerializedWorker.cs
- StoreItemCollection.cs
- PathData.cs
- DataPointer.cs
- EntityClassGenerator.cs
- HtmlTable.cs
- EventMappingSettings.cs
- OpenTypeCommon.cs
- VisualTarget.cs
- JsonUriDataContract.cs
- PrintPageEvent.cs
- SmiTypedGetterSetter.cs
- ProtocolState.cs