Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / textformatting / TextTabProperties.cs / 1 / TextTabProperties.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2004 // // File: TextTabProperties.cs // // Contents: Definition of tab properties and related types // // Spec: http://team/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 1-2-2004 Worachai Chaoweeraprasit (wchao) // //----------------------------------------------------------------------- using System; using System.Collections; using System.Windows; namespace System.Windows.Media.TextFormatting { ////// Properties of user-defined tab /// public class TextTabProperties { private TextTabAlignment _alignment; private double _location; private int _tabLeader; private int _aligningChar; ////// Construct tab properties /// /// alignment of text at tab location /// tab location /// tab leader /// specific character in text that is aligned at tab location public TextTabProperties( TextTabAlignment alignment, double location, int tabLeader, int aligningChar ) { _alignment = alignment; _location = location; _tabLeader = tabLeader; _aligningChar = aligningChar; } ////// Property to determine how text is aligned at tab location /// public TextTabAlignment Alignment { get { return _alignment; } } ////// Tab location /// public double Location { get { return _location; } } ////// Character used to display tab leader /// public int TabLeader { get { return _tabLeader; } } ////// Specific character in text that is aligned at specified tab location /// public int AligningCharacter { get { return _aligningChar; } } } ////// This property determines how text is aligned at tab location /// public enum TextTabAlignment { ////// Text is left-aligned at tab location /// Left, ////// Text is center-aligned at tab location /// Center, ////// Text is right-aligned at tab location /// Right, ////// Text is aligned at tab location at a specified character /// Character, } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2004 // // File: TextTabProperties.cs // // Contents: Definition of tab properties and related types // // Spec: http://team/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 1-2-2004 Worachai Chaoweeraprasit (wchao) // //----------------------------------------------------------------------- using System; using System.Collections; using System.Windows; namespace System.Windows.Media.TextFormatting { ////// Properties of user-defined tab /// public class TextTabProperties { private TextTabAlignment _alignment; private double _location; private int _tabLeader; private int _aligningChar; ////// Construct tab properties /// /// alignment of text at tab location /// tab location /// tab leader /// specific character in text that is aligned at tab location public TextTabProperties( TextTabAlignment alignment, double location, int tabLeader, int aligningChar ) { _alignment = alignment; _location = location; _tabLeader = tabLeader; _aligningChar = aligningChar; } ////// Property to determine how text is aligned at tab location /// public TextTabAlignment Alignment { get { return _alignment; } } ////// Tab location /// public double Location { get { return _location; } } ////// Character used to display tab leader /// public int TabLeader { get { return _tabLeader; } } ////// Specific character in text that is aligned at specified tab location /// public int AligningCharacter { get { return _aligningChar; } } } ////// This property determines how text is aligned at tab location /// public enum TextTabAlignment { ////// Text is left-aligned at tab location /// Left, ////// Text is center-aligned at tab location /// Center, ////// Text is right-aligned at tab location /// Right, ////// Text is aligned at tab location at a specified character /// Character, } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
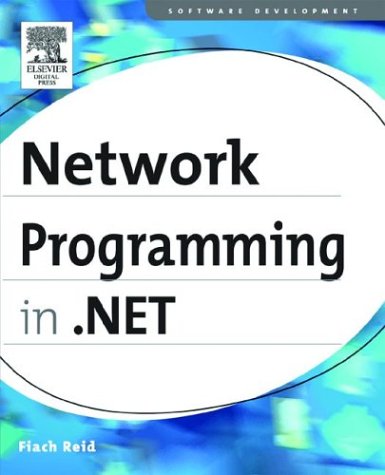
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AnchorEditor.cs
- SingleAnimationBase.cs
- AppSettingsExpressionEditor.cs
- SymbolEqualComparer.cs
- EntitySqlException.cs
- SqlLiftWhereClauses.cs
- MethodImplAttribute.cs
- DBCommandBuilder.cs
- MultipartIdentifier.cs
- MappingMetadataHelper.cs
- WaitHandleCannotBeOpenedException.cs
- HtmlTable.cs
- DataTemplate.cs
- XmlSchemaParticle.cs
- XmlTextAttribute.cs
- SelectedGridItemChangedEvent.cs
- RepeatButton.cs
- EventLogPermissionEntry.cs
- TokenFactoryCredential.cs
- WebConfigurationHost.cs
- EmptyStringExpandableObjectConverter.cs
- PropertyConverter.cs
- SQLDateTime.cs
- HtmlShim.cs
- DataGridViewCellStyle.cs
- DispatcherOperation.cs
- ResourceBinder.cs
- ObfuscateAssemblyAttribute.cs
- TextEditorDragDrop.cs
- DataServiceException.cs
- FixedSOMImage.cs
- MetadataItem_Static.cs
- RecommendedAsConfigurableAttribute.cs
- Interlocked.cs
- MultipartContentParser.cs
- ResourceManager.cs
- GridViewRow.cs
- AuthenticationModuleElementCollection.cs
- DeploymentExceptionMapper.cs
- FormViewUpdateEventArgs.cs
- ImmutableAssemblyCacheEntry.cs
- LinqDataSourceUpdateEventArgs.cs
- SettingsPropertyWrongTypeException.cs
- BuildDependencySet.cs
- SizeChangedEventArgs.cs
- DBCSCodePageEncoding.cs
- CodeMemberMethod.cs
- BitmapData.cs
- Italic.cs
- XNodeNavigator.cs
- SiteMapNodeItem.cs
- PaginationProgressEventArgs.cs
- DataGridViewTopRowAccessibleObject.cs
- BrushMappingModeValidation.cs
- DataTransferEventArgs.cs
- ErrorTableItemStyle.cs
- DynamicEndpoint.cs
- StrokeCollectionConverter.cs
- EntityCommand.cs
- QilFunction.cs
- SamlAuthorizationDecisionStatement.cs
- PageCache.cs
- ArrayItemValue.cs
- PhonemeConverter.cs
- DoubleAnimationBase.cs
- DataStreamFromComStream.cs
- HtmlContainerControl.cs
- Listbox.cs
- CellPartitioner.cs
- CqlLexerHelpers.cs
- SchemaImporterExtension.cs
- PrintPreviewGraphics.cs
- TrackingExtract.cs
- RootBrowserWindowAutomationPeer.cs
- x509store.cs
- XMLUtil.cs
- SoapSchemaImporter.cs
- FacetValues.cs
- Module.cs
- MessageBox.cs
- ContravarianceAdapter.cs
- SoapFormatterSinks.cs
- UrlMappingsModule.cs
- XmlUrlResolver.cs
- InputLangChangeRequestEvent.cs
- Assert.cs
- SplineKeyFrames.cs
- GacUtil.cs
- Point.cs
- FontNamesConverter.cs
- HtmlShim.cs
- EntityDataSourceViewSchema.cs
- ComponentResourceKeyConverter.cs
- WindowsProgressbar.cs
- HierarchicalDataSourceControl.cs
- IgnoreSectionHandler.cs
- DefaultMemberAttribute.cs
- SemaphoreSecurity.cs
- HttpCachePolicyElement.cs
- FontFaceLayoutInfo.cs