Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / Animation / Generated / DoubleAnimationBase.cs / 1 / DoubleAnimationBase.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- // Allow use of presharp: #pragma warning suppress#pragma warning disable 1634, 1691 using MS.Internal; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; using MS.Internal.PresentationCore; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Animation { /// /// /// public abstract class DoubleAnimationBase : AnimationTimeline { #region Constructors ////// Creates a new DoubleAnimationBase. /// protected DoubleAnimationBase() : base() { } #endregion #region Freezable ////// Creates a copy of this DoubleAnimationBase /// ///The copy public new DoubleAnimationBase Clone() { return (DoubleAnimationBase)base.Clone(); } #endregion #region IAnimation ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public override sealed object GetCurrentValue(object defaultOriginValue, object defaultDestinationValue, AnimationClock animationClock) { // Verify that object arguments are non-null since we are a value type if (defaultOriginValue == null) { throw new ArgumentNullException("defaultOriginValue"); } if (defaultDestinationValue == null) { throw new ArgumentNullException("defaultDestinationValue"); } return GetCurrentValue((Double)defaultOriginValue, (Double)defaultDestinationValue, animationClock); } ////// Returns the type of the target property /// public override sealed Type TargetPropertyType { get { ReadPreamble(); return typeof(Double); } } #endregion #region Methods ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public Double GetCurrentValue(Double defaultOriginValue, Double defaultDestinationValue, AnimationClock animationClock) { ReadPreamble(); if (animationClock == null) { throw new ArgumentNullException("animationClock"); } // We check for null above but presharp doesn't notice so we suppress the // warning here. #pragma warning suppress 6506 if (animationClock.CurrentState == ClockState.Stopped) { return defaultDestinationValue; } /* if (!AnimatedTypeHelpers.IsValidAnimationValueDouble(defaultDestinationValue)) { throw new ArgumentException( SR.Get( SRID.Animation_InvalidBaseValue, defaultDestinationValue, defaultDestinationValue.GetType(), GetType()), "defaultDestinationValue"); } */ return GetCurrentValueCore(defaultOriginValue, defaultDestinationValue, animationClock); } ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// protected abstract Double GetCurrentValueCore(Double defaultOriginValue, Double defaultDestinationValue, AnimationClock animationClock); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- // Allow use of presharp: #pragma warning suppress#pragma warning disable 1634, 1691 using MS.Internal; using System; using System.Collections; using System.ComponentModel; using System.Diagnostics; using System.Windows.Media.Animation; using System.Windows.Media.Media3D; using MS.Internal.PresentationCore; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media.Animation { /// /// /// public abstract class DoubleAnimationBase : AnimationTimeline { #region Constructors ////// Creates a new DoubleAnimationBase. /// protected DoubleAnimationBase() : base() { } #endregion #region Freezable ////// Creates a copy of this DoubleAnimationBase /// ///The copy public new DoubleAnimationBase Clone() { return (DoubleAnimationBase)base.Clone(); } #endregion #region IAnimation ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public override sealed object GetCurrentValue(object defaultOriginValue, object defaultDestinationValue, AnimationClock animationClock) { // Verify that object arguments are non-null since we are a value type if (defaultOriginValue == null) { throw new ArgumentNullException("defaultOriginValue"); } if (defaultDestinationValue == null) { throw new ArgumentNullException("defaultDestinationValue"); } return GetCurrentValue((Double)defaultOriginValue, (Double)defaultDestinationValue, animationClock); } ////// Returns the type of the target property /// public override sealed Type TargetPropertyType { get { ReadPreamble(); return typeof(Double); } } #endregion #region Methods ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// public Double GetCurrentValue(Double defaultOriginValue, Double defaultDestinationValue, AnimationClock animationClock) { ReadPreamble(); if (animationClock == null) { throw new ArgumentNullException("animationClock"); } // We check for null above but presharp doesn't notice so we suppress the // warning here. #pragma warning suppress 6506 if (animationClock.CurrentState == ClockState.Stopped) { return defaultDestinationValue; } /* if (!AnimatedTypeHelpers.IsValidAnimationValueDouble(defaultDestinationValue)) { throw new ArgumentException( SR.Get( SRID.Animation_InvalidBaseValue, defaultDestinationValue, defaultDestinationValue.GetType(), GetType()), "defaultDestinationValue"); } */ return GetCurrentValueCore(defaultOriginValue, defaultDestinationValue, animationClock); } ////// Calculates the value this animation believes should be the current value for the property. /// /// /// This value is the suggested origin value provided to the animation /// to be used if the animation does not have its own concept of a /// start value. If this animation is the first in a composition chain /// this value will be the snapshot value if one is available or the /// base property value if it is not; otherise this value will be the /// value returned by the previous animation in the chain with an /// animationClock that is not Stopped. /// /// /// This value is the suggested destination value provided to the animation /// to be used if the animation does not have its own concept of an /// end value. This value will be the base value if the animation is /// in the first composition layer of animations on a property; /// otherwise this value will be the output value from the previous /// composition layer of animations for the property. /// /// /// This is the animationClock which can generate the CurrentTime or /// CurrentProgress value to be used by the animation to generate its /// output value. /// ////// The value this animation believes should be the current value for the property. /// protected abstract Double GetCurrentValueCore(Double defaultOriginValue, Double defaultDestinationValue, AnimationClock animationClock); #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
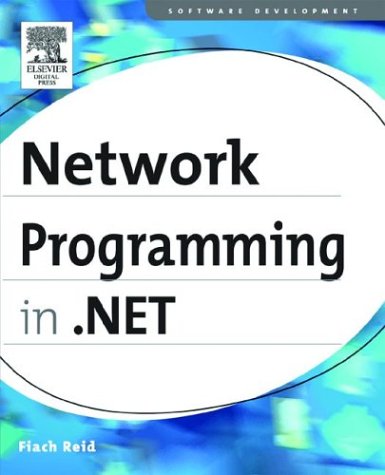
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PngBitmapEncoder.cs
- WindowsAuthenticationEventArgs.cs
- precedingquery.cs
- DataControlHelper.cs
- WindowsPrincipal.cs
- ConfigurationElementProperty.cs
- WebSysDefaultValueAttribute.cs
- SqlParameter.cs
- FlowDocumentReaderAutomationPeer.cs
- PersonalizationAdministration.cs
- SignatureToken.cs
- StringValidatorAttribute.cs
- PermissionToken.cs
- RemoteWebConfigurationHost.cs
- AttributeProviderAttribute.cs
- DataKeyArray.cs
- X509SecurityTokenProvider.cs
- selecteditemcollection.cs
- FixedSchema.cs
- DesignColumnCollection.cs
- HtmlFormWrapper.cs
- MethodBuilderInstantiation.cs
- LookupNode.cs
- RuntimeWrappedException.cs
- ManagementNamedValueCollection.cs
- ServicePointManagerElement.cs
- RawAppCommandInputReport.cs
- FormViewRow.cs
- DesignerView.Commands.cs
- Item.cs
- ApplicationFileCodeDomTreeGenerator.cs
- StackSpiller.cs
- SqlExpressionNullability.cs
- ToolStripManager.cs
- DesignColumnCollection.cs
- LowerCaseStringConverter.cs
- DocumentEventArgs.cs
- Win32MouseDevice.cs
- ToolStripRenderer.cs
- DiscoveryDocumentLinksPattern.cs
- WorkflowMessageEventArgs.cs
- MessageQueuePermission.cs
- NullReferenceException.cs
- Line.cs
- SafeLocalMemHandle.cs
- XpsDocument.cs
- GridViewItemAutomationPeer.cs
- WorkflowTransactionService.cs
- LocationChangedEventArgs.cs
- IdentifierCreationService.cs
- StrongNameMembershipCondition.cs
- UpdateProgress.cs
- Set.cs
- XXXInfos.cs
- SiteMapPath.cs
- WindowsRegion.cs
- DataViewSettingCollection.cs
- Property.cs
- XmlSerializableWriter.cs
- IdentifierCreationService.cs
- SRDisplayNameAttribute.cs
- ProxyHwnd.cs
- MenuItem.cs
- OracleString.cs
- TabControlAutomationPeer.cs
- TextContainerChangeEventArgs.cs
- GeometryDrawing.cs
- SingleTagSectionHandler.cs
- DataBoundLiteralControl.cs
- AccessDataSourceWizardForm.cs
- TextServicesPropertyRanges.cs
- GridViewPageEventArgs.cs
- LocatorPart.cs
- UpDownBase.cs
- ColumnPropertiesGroup.cs
- DEREncoding.cs
- FixedSOMTableCell.cs
- UnSafeCharBuffer.cs
- XmlSchemaInferenceException.cs
- EntityContainer.cs
- GlobalEventManager.cs
- XsdCachingReader.cs
- XmlMapping.cs
- FontStretchConverter.cs
- DbParameterCollectionHelper.cs
- _AutoWebProxyScriptHelper.cs
- CultureSpecificCharacterBufferRange.cs
- CollectionBuilder.cs
- SoapAttributeAttribute.cs
- DataSourceSelectArguments.cs
- InternalConfigEventArgs.cs
- TypeContext.cs
- CompoundFileReference.cs
- VariableBinder.cs
- DataObject.cs
- PeekCompletedEventArgs.cs
- EventLogEntry.cs
- WebBrowserBase.cs
- WebPartCollection.cs
- CodeTypeMember.cs