Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Shapes / Line.cs / 1 / Line.cs
//---------------------------------------------------------------------------- // File: Line.cs // // Description: // Implementation of Line shape element. // // History: // 05/30/02 - AdSmith - Created. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows.Shapes; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using System.ComponentModel; using MS.Internal; using System; namespace System.Windows.Shapes { ////// The line shape element /// This element (like all shapes) belongs under a Canvas, /// and will be presented by the parent canvas. /// public sealed class Line : Shape { #region Constructors ////// Instantiates a new instance of a line. /// public Line() { } #endregion Constructors #region Dynamic Properties ////// X1 property /// public static readonly DependencyProperty X1Property = DependencyProperty.Register( "X1", typeof(double), typeof(Line), new FrameworkPropertyMetadata(0d, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(Shape.IsDoubleFinite)); ////// X1 property /// [TypeConverter(typeof(LengthConverter))] public double X1 { get { return (double)GetValue(X1Property); } set { SetValue(X1Property, value); } } ////// Y1 property /// public static readonly DependencyProperty Y1Property = DependencyProperty.Register( "Y1", typeof(double), typeof(Line), new FrameworkPropertyMetadata(0d, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(Shape.IsDoubleFinite)); ////// Y1 property /// [TypeConverter(typeof(LengthConverter))] public double Y1 { get { return (double)GetValue(Y1Property); } set { SetValue(Y1Property, value); } } ////// X2 property /// public static readonly DependencyProperty X2Property = DependencyProperty.Register( "X2", typeof(double), typeof(Line), new FrameworkPropertyMetadata(0d, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(Shape.IsDoubleFinite)); ////// X2 property /// [TypeConverter(typeof(LengthConverter))] public double X2 { get { return (double)GetValue(X2Property); } set { SetValue(X2Property, value); } } ////// Y2 property /// public static readonly DependencyProperty Y2Property = DependencyProperty.Register( "Y2", typeof(double), typeof(Line), new FrameworkPropertyMetadata(0d, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(Shape.IsDoubleFinite)); ////// Y2 property /// [TypeConverter(typeof(LengthConverter))] public double Y2 { get { return (double)GetValue(Y2Property); } set { SetValue(Y2Property, value); } } #endregion Dynamic Properties #region Protected Methods and Properties ////// Get the line that defines this shape /// protected override Geometry DefiningGeometry { get { return _lineGeometry; } } #endregion #region Internal Methods internal override void CacheDefiningGeometry() { Point point1 = new Point(X1, Y1); Point point2 = new Point(X2, Y2); // Create the Line geometry _lineGeometry = new LineGeometry(point1, point2); } #endregion Internal Methods #region Private Methods and Members private LineGeometry _lineGeometry; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // File: Line.cs // // Description: // Implementation of Line shape element. // // History: // 05/30/02 - AdSmith - Created. // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows.Shapes; using System.Diagnostics; using System.Windows.Threading; using System.Windows; using System.Windows.Media; using System.ComponentModel; using MS.Internal; using System; namespace System.Windows.Shapes { ////// The line shape element /// This element (like all shapes) belongs under a Canvas, /// and will be presented by the parent canvas. /// public sealed class Line : Shape { #region Constructors ////// Instantiates a new instance of a line. /// public Line() { } #endregion Constructors #region Dynamic Properties ////// X1 property /// public static readonly DependencyProperty X1Property = DependencyProperty.Register( "X1", typeof(double), typeof(Line), new FrameworkPropertyMetadata(0d, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(Shape.IsDoubleFinite)); ////// X1 property /// [TypeConverter(typeof(LengthConverter))] public double X1 { get { return (double)GetValue(X1Property); } set { SetValue(X1Property, value); } } ////// Y1 property /// public static readonly DependencyProperty Y1Property = DependencyProperty.Register( "Y1", typeof(double), typeof(Line), new FrameworkPropertyMetadata(0d, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(Shape.IsDoubleFinite)); ////// Y1 property /// [TypeConverter(typeof(LengthConverter))] public double Y1 { get { return (double)GetValue(Y1Property); } set { SetValue(Y1Property, value); } } ////// X2 property /// public static readonly DependencyProperty X2Property = DependencyProperty.Register( "X2", typeof(double), typeof(Line), new FrameworkPropertyMetadata(0d, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(Shape.IsDoubleFinite)); ////// X2 property /// [TypeConverter(typeof(LengthConverter))] public double X2 { get { return (double)GetValue(X2Property); } set { SetValue(X2Property, value); } } ////// Y2 property /// public static readonly DependencyProperty Y2Property = DependencyProperty.Register( "Y2", typeof(double), typeof(Line), new FrameworkPropertyMetadata(0d, FrameworkPropertyMetadataOptions.AffectsMeasure | FrameworkPropertyMetadataOptions.AffectsRender), new ValidateValueCallback(Shape.IsDoubleFinite)); ////// Y2 property /// [TypeConverter(typeof(LengthConverter))] public double Y2 { get { return (double)GetValue(Y2Property); } set { SetValue(Y2Property, value); } } #endregion Dynamic Properties #region Protected Methods and Properties ////// Get the line that defines this shape /// protected override Geometry DefiningGeometry { get { return _lineGeometry; } } #endregion #region Internal Methods internal override void CacheDefiningGeometry() { Point point1 = new Point(X1, Y1); Point point2 = new Point(X2, Y2); // Create the Line geometry _lineGeometry = new LineGeometry(point1, point2); } #endregion Internal Methods #region Private Methods and Members private LineGeometry _lineGeometry; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
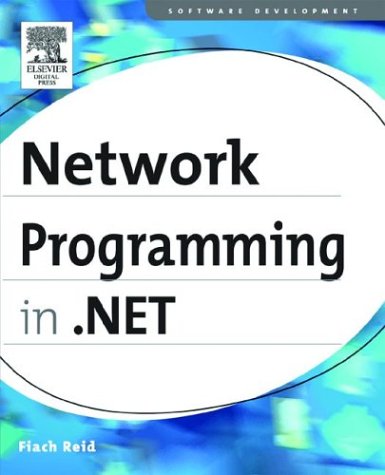
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DoubleConverter.cs
- String.cs
- PageWrapper.cs
- ExpressionNormalizer.cs
- StylusPointPropertyInfo.cs
- RectangleGeometry.cs
- ListBindableAttribute.cs
- StickyNote.cs
- PropertyChangedEventManager.cs
- SQLMoneyStorage.cs
- ElementInit.cs
- HelpPage.cs
- PrintDocument.cs
- SignatureToken.cs
- CompressStream.cs
- OptimalBreakSession.cs
- ClientConvert.cs
- PropertyEmitter.cs
- MailWebEventProvider.cs
- HtmlHead.cs
- SharedUtils.cs
- CallbackHandler.cs
- XmlSchemaCollection.cs
- Int32Collection.cs
- UnsafeNativeMethodsMilCoreApi.cs
- CellRelation.cs
- Asn1Utilities.cs
- LogReserveAndAppendState.cs
- login.cs
- XmlSerializerVersionAttribute.cs
- RangeContentEnumerator.cs
- ContentType.cs
- ASCIIEncoding.cs
- StorageTypeMapping.cs
- CodeIndexerExpression.cs
- WasHttpModulesInstallComponent.cs
- CharacterShapingProperties.cs
- MeasureData.cs
- ListenerConfig.cs
- ReadOnlyDictionary.cs
- UpdatePanelControlTrigger.cs
- EntityCollection.cs
- AnnouncementInnerClientCD1.cs
- ReceiveContextCollection.cs
- SmiMetaData.cs
- DataObjectEventArgs.cs
- DataBoundControlHelper.cs
- SqlMethodAttribute.cs
- DeriveBytes.cs
- TemplatedWizardStep.cs
- FontFaceLayoutInfo.cs
- FormViewCommandEventArgs.cs
- DataControlImageButton.cs
- HtmlInputText.cs
- XmlUrlResolver.cs
- ObjectStateManager.cs
- PersonalizationProviderCollection.cs
- ObjectToIdCache.cs
- TextBoxRenderer.cs
- XmlAttributes.cs
- XmlLanguageConverter.cs
- Model3D.cs
- ActivityExecutorSurrogate.cs
- BackgroundFormatInfo.cs
- CommandArguments.cs
- GridPatternIdentifiers.cs
- XPathNodeList.cs
- _LocalDataStore.cs
- ProtocolsConfigurationEntry.cs
- IItemContainerGenerator.cs
- EndpointBehaviorElementCollection.cs
- HealthMonitoringSectionHelper.cs
- Overlapped.cs
- ContextInformation.cs
- SoapProtocolReflector.cs
- SessionParameter.cs
- LayoutUtils.cs
- ListDataHelper.cs
- PackageDigitalSignatureManager.cs
- QilCloneVisitor.cs
- XmlBinaryReaderSession.cs
- TemplateAction.cs
- DataGridViewIntLinkedList.cs
- AccessDataSourceWizardForm.cs
- ConstantExpression.cs
- PersonalizationDictionary.cs
- GlyphInfoList.cs
- OperationCanceledException.cs
- RelAssertionDirectKeyIdentifierClause.cs
- GridViewColumnCollectionChangedEventArgs.cs
- XmlWrappingReader.cs
- XmlSchemaInferenceException.cs
- MimeTypeMapper.cs
- InfoCardRSAPKCS1SignatureFormatter.cs
- XPathChildIterator.cs
- ValidatorCompatibilityHelper.cs
- VirtualPathProvider.cs
- MimeImporter.cs
- IndependentAnimationStorage.cs
- ErrorHandlingAcceptor.cs