Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / Microsoft / Scripting / Ast / ElementInit.cs / 1305376 / ElementInit.cs
/* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Reflection; using System.Text; using System.Dynamic.Utils; #if SILVERLIGHT using System.Core; #endif namespace System.Linq.Expressions { ////// Represents the initialization of a list. /// public sealed class ElementInit : IArgumentProvider { private MethodInfo _addMethod; private ReadOnlyCollection_arguments; internal ElementInit(MethodInfo addMethod, ReadOnlyCollection arguments) { _addMethod = addMethod; _arguments = arguments; } /// /// Gets the public MethodInfo AddMethod { get { return _addMethod; } } ///used to add elements to the object. /// /// Gets the list of elements to be added to the object. /// public ReadOnlyCollectionArguments { get { return _arguments; } } Expression IArgumentProvider.GetArgument(int index) { return _arguments[index]; } int IArgumentProvider.ArgumentCount { get { return _arguments.Count; } } /// /// Creates a ///representation of the node. /// A public override string ToString() { return ExpressionStringBuilder.ElementInitBindingToString(this); } ///representation of the node. /// Creates a new expression that is like this one, but using the /// supplied children. If all of the children are the same, it will /// return this expression. /// /// Theproperty of the result. /// This expression if no children changed, or an expression with the updated children. public ElementInit Update(IEnumerablearguments) { if (arguments == Arguments) { return this; } return Expression.ElementInit(AddMethod, arguments); } } public partial class Expression { /// /// Creates an /// TheElementInit expression that represents the initialization of a list. ///for the list's Add method. /// An array containing the Expressions to be used to initialize the list. /// The created public static ElementInit ElementInit(MethodInfo addMethod, params Expression[] arguments) { return ElementInit(addMethod, arguments as IEnumerableElementInit expression.); } /// /// Creates an /// TheElementInit expression that represents the initialization of a list. ///for the list's Add method. /// An containing elements to initialize the list. /// The created public static ElementInit ElementInit(MethodInfo addMethod, IEnumerableElementInit expression.arguments) { ContractUtils.RequiresNotNull(addMethod, "addMethod"); ContractUtils.RequiresNotNull(arguments, "arguments"); var argumentsRO = arguments.ToReadOnly(); RequiresCanRead(argumentsRO, "arguments"); ValidateElementInitAddMethodInfo(addMethod); ValidateArgumentTypes(addMethod, ExpressionType.Call, ref argumentsRO); return new ElementInit(addMethod, argumentsRO); } private static void ValidateElementInitAddMethodInfo(MethodInfo addMethod) { ValidateMethodInfo(addMethod); ParameterInfo[] pis = addMethod.GetParametersCached(); if (pis.Length == 0) { throw Error.ElementInitializerMethodWithZeroArgs(); } if (!addMethod.Name.Equals("Add", StringComparison.OrdinalIgnoreCase)) { throw Error.ElementInitializerMethodNotAdd(); } if (addMethod.IsStatic) { throw Error.ElementInitializerMethodStatic(); } foreach (ParameterInfo pi in pis) { if (pi.ParameterType.IsByRef) { throw Error.ElementInitializerMethodNoRefOutParam(pi.Name, addMethod.Name); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Reflection; using System.Text; using System.Dynamic.Utils; #if SILVERLIGHT using System.Core; #endif namespace System.Linq.Expressions { /// /// Represents the initialization of a list. /// public sealed class ElementInit : IArgumentProvider { private MethodInfo _addMethod; private ReadOnlyCollection_arguments; internal ElementInit(MethodInfo addMethod, ReadOnlyCollection arguments) { _addMethod = addMethod; _arguments = arguments; } /// /// Gets the public MethodInfo AddMethod { get { return _addMethod; } } ///used to add elements to the object. /// /// Gets the list of elements to be added to the object. /// public ReadOnlyCollectionArguments { get { return _arguments; } } Expression IArgumentProvider.GetArgument(int index) { return _arguments[index]; } int IArgumentProvider.ArgumentCount { get { return _arguments.Count; } } /// /// Creates a ///representation of the node. /// A public override string ToString() { return ExpressionStringBuilder.ElementInitBindingToString(this); } ///representation of the node. /// Creates a new expression that is like this one, but using the /// supplied children. If all of the children are the same, it will /// return this expression. /// /// Theproperty of the result. /// This expression if no children changed, or an expression with the updated children. public ElementInit Update(IEnumerablearguments) { if (arguments == Arguments) { return this; } return Expression.ElementInit(AddMethod, arguments); } } public partial class Expression { /// /// Creates an /// TheElementInit expression that represents the initialization of a list. ///for the list's Add method. /// An array containing the Expressions to be used to initialize the list. /// The created public static ElementInit ElementInit(MethodInfo addMethod, params Expression[] arguments) { return ElementInit(addMethod, arguments as IEnumerableElementInit expression.); } /// /// Creates an /// TheElementInit expression that represents the initialization of a list. ///for the list's Add method. /// An containing elements to initialize the list. /// The created public static ElementInit ElementInit(MethodInfo addMethod, IEnumerableElementInit expression.arguments) { ContractUtils.RequiresNotNull(addMethod, "addMethod"); ContractUtils.RequiresNotNull(arguments, "arguments"); var argumentsRO = arguments.ToReadOnly(); RequiresCanRead(argumentsRO, "arguments"); ValidateElementInitAddMethodInfo(addMethod); ValidateArgumentTypes(addMethod, ExpressionType.Call, ref argumentsRO); return new ElementInit(addMethod, argumentsRO); } private static void ValidateElementInitAddMethodInfo(MethodInfo addMethod) { ValidateMethodInfo(addMethod); ParameterInfo[] pis = addMethod.GetParametersCached(); if (pis.Length == 0) { throw Error.ElementInitializerMethodWithZeroArgs(); } if (!addMethod.Name.Equals("Add", StringComparison.OrdinalIgnoreCase)) { throw Error.ElementInitializerMethodNotAdd(); } if (addMethod.IsStatic) { throw Error.ElementInitializerMethodStatic(); } foreach (ParameterInfo pi in pis) { if (pi.ParameterType.IsByRef) { throw Error.ElementInitializerMethodNoRefOutParam(pi.Name, addMethod.Name); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
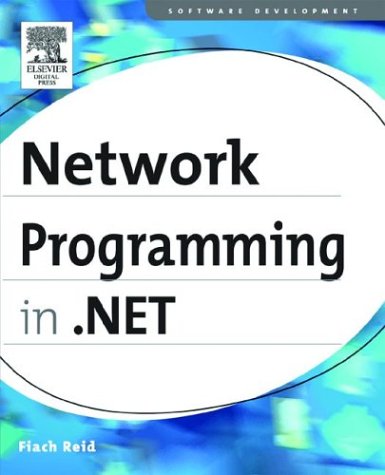
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HelpProvider.cs
- InternalMappingException.cs
- ModuleConfigurationInfo.cs
- UiaCoreApi.cs
- diagnosticsswitches.cs
- CompiledQuery.cs
- EntityContainerEntitySet.cs
- CommonEndpointBehaviorElement.cs
- UnsafePeerToPeerMethods.cs
- RegexCharClass.cs
- SqlDataSourceCache.cs
- ThreadAbortException.cs
- ScrollData.cs
- DataTemplate.cs
- control.ime.cs
- WebPartUserCapability.cs
- LineMetrics.cs
- SqlCharStream.cs
- DetailsViewPageEventArgs.cs
- BadImageFormatException.cs
- Interlocked.cs
- TextParagraphCache.cs
- HtmlPanelAdapter.cs
- TraceSection.cs
- RadioButtonList.cs
- WorkflowRuntimeElement.cs
- GridViewColumnHeader.cs
- DashStyle.cs
- WebPartConnection.cs
- ServiceBehaviorElementCollection.cs
- WebPartVerbCollection.cs
- SerializationFieldInfo.cs
- FormsAuthenticationModule.cs
- DataServiceQueryOfT.cs
- WebSysDescriptionAttribute.cs
- MessageContractImporter.cs
- MDIWindowDialog.cs
- Message.cs
- CodeValidator.cs
- ManifestSignatureInformation.cs
- PermissionSetTriple.cs
- XNameConverter.cs
- _NTAuthentication.cs
- SqlNode.cs
- RoutedEventConverter.cs
- WeakHashtable.cs
- FindCriteriaCD1.cs
- PathTooLongException.cs
- SoapDocumentServiceAttribute.cs
- CompoundFileStorageReference.cs
- Module.cs
- SemanticResultValue.cs
- SQLDecimalStorage.cs
- CompilerErrorCollection.cs
- EarlyBoundInfo.cs
- Errors.cs
- COAUTHINFO.cs
- XmlSyndicationContent.cs
- RegistrationServices.cs
- Quad.cs
- XmlSchemaImport.cs
- MarginCollapsingState.cs
- SerializationInfoEnumerator.cs
- HotSpotCollection.cs
- SafeNativeMethods.cs
- NotSupportedException.cs
- TemplateBindingExpressionConverter.cs
- DecoderReplacementFallback.cs
- PriorityBindingExpression.cs
- CodeSnippetExpression.cs
- ComAdminInterfaces.cs
- NativeMethods.cs
- HttpCacheVary.cs
- DataGridRow.cs
- BCryptNative.cs
- PropertyToken.cs
- MenuAutomationPeer.cs
- PageCache.cs
- DropDownList.cs
- SessionParameter.cs
- OutputCacheModule.cs
- TableCellCollection.cs
- FlowPanelDesigner.cs
- Accessors.cs
- EditorPart.cs
- securestring.cs
- ProfileSection.cs
- Helpers.cs
- EventTrigger.cs
- ResourcePropertyMemberCodeDomSerializer.cs
- DesignerPerfEventProvider.cs
- DrawItemEvent.cs
- SizeChangedInfo.cs
- SmtpSection.cs
- TouchesOverProperty.cs
- DataIdProcessor.cs
- XPathBinder.cs
- _SafeNetHandles.cs
- HttpCookie.cs
- DynamicDataManager.cs