Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / WinForms / Managed / System / WinForms / DrawItemEvent.cs / 1 / DrawItemEvent.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.ComponentModel; using System.Drawing; using Microsoft.Win32; ////// /// This event is fired by owner draw Controls, such as ListBoxes and /// ComboBoxes. It contains all the information needed for the user to /// paint the given item, including the item index, the Rectangle in which /// the drawing should be done, and the Graphics object with which the drawing /// should be done. /// public class DrawItemEventArgs : EventArgs { ////// /// The backColor to paint each menu item with. /// private Color backColor; ////// /// The foreColor to paint each menu item with. /// private Color foreColor; ////// /// The font used to draw the item's string. /// private Font font; ////// /// The graphics object with which the drawing should be done. /// private readonly System.Drawing.Graphics graphics; ////// /// The index of the item that should be painted. /// private readonly int index; ////// /// The rectangle outlining the area in which the painting should be /// done. /// private readonly Rectangle rect; ////// /// Miscellaneous state information, such as whether the item is /// "selected", "focused", or some other such information. ComboBoxes /// have one special piece of information which indicates if the item /// being painted is the editable portion of the ComboBox. /// private readonly DrawItemState state; ////// /// Creates a new DrawItemEventArgs with the given parameters. /// public DrawItemEventArgs(Graphics graphics, Font font, Rectangle rect, int index, DrawItemState state) { this.graphics = graphics; this.font = font; this.rect = rect; this.index = index; this.state = state; this.foreColor = SystemColors.WindowText; this.backColor = SystemColors.Window; } ////// /// Creates a new DrawItemEventArgs with the given parameters, including the foreColor and backColor of the control. /// public DrawItemEventArgs(Graphics graphics, Font font, Rectangle rect, int index, DrawItemState state, Color foreColor, Color backColor) { this.graphics = graphics; this.font = font; this.rect = rect; this.index = index; this.state = state; this.foreColor = foreColor; this.backColor = backColor; } ///public Color BackColor { get { if ((state & DrawItemState.Selected) == DrawItemState.Selected) { return SystemColors.Highlight; } return backColor; } } /// /// /// The rectangle outlining the area in which the painting should be /// done. /// public Rectangle Bounds { get { return rect; } } ////// /// A suggested font, usually the parent control's Font property. /// public Font Font { get { return font; } } ////// /// A suggested color drawing: either SystemColors.WindowText or SystemColors.HighlightText, /// depending on whether this item is selected. /// public Color ForeColor { get { if ((state & DrawItemState.Selected) == DrawItemState.Selected) { return SystemColors.HighlightText; } return foreColor; } } ////// /// Graphics object with which painting should be done. /// public Graphics Graphics { get { return graphics; } } ////// /// The index of the item that should be painted. /// public int Index { get { return index; } } ////// /// Miscellaneous state information, such as whether the item is /// "selected", "focused", or some other such information. ComboBoxes /// have one special piece of information which indicates if the item /// being painted is the editable portion of the ComboBox. /// public DrawItemState State { get { return state; } } ////// /// Draws the background of the given rectangle with the color returned from the BackColor property. /// public virtual void DrawBackground() { Brush backBrush = new SolidBrush(BackColor); Graphics.FillRectangle(backBrush, rect); backBrush.Dispose(); } ////// /// Draws a handy focus rect in the given rectangle. /// public virtual void DrawFocusRectangle() { if ((state & DrawItemState.Focus) == DrawItemState.Focus && (state & DrawItemState.NoFocusRect) != DrawItemState.NoFocusRect) ControlPaint.DrawFocusRectangle(Graphics, rect, ForeColor, BackColor); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Diagnostics; using System; using System.ComponentModel; using System.Drawing; using Microsoft.Win32; ////// /// This event is fired by owner draw Controls, such as ListBoxes and /// ComboBoxes. It contains all the information needed for the user to /// paint the given item, including the item index, the Rectangle in which /// the drawing should be done, and the Graphics object with which the drawing /// should be done. /// public class DrawItemEventArgs : EventArgs { ////// /// The backColor to paint each menu item with. /// private Color backColor; ////// /// The foreColor to paint each menu item with. /// private Color foreColor; ////// /// The font used to draw the item's string. /// private Font font; ////// /// The graphics object with which the drawing should be done. /// private readonly System.Drawing.Graphics graphics; ////// /// The index of the item that should be painted. /// private readonly int index; ////// /// The rectangle outlining the area in which the painting should be /// done. /// private readonly Rectangle rect; ////// /// Miscellaneous state information, such as whether the item is /// "selected", "focused", or some other such information. ComboBoxes /// have one special piece of information which indicates if the item /// being painted is the editable portion of the ComboBox. /// private readonly DrawItemState state; ////// /// Creates a new DrawItemEventArgs with the given parameters. /// public DrawItemEventArgs(Graphics graphics, Font font, Rectangle rect, int index, DrawItemState state) { this.graphics = graphics; this.font = font; this.rect = rect; this.index = index; this.state = state; this.foreColor = SystemColors.WindowText; this.backColor = SystemColors.Window; } ////// /// Creates a new DrawItemEventArgs with the given parameters, including the foreColor and backColor of the control. /// public DrawItemEventArgs(Graphics graphics, Font font, Rectangle rect, int index, DrawItemState state, Color foreColor, Color backColor) { this.graphics = graphics; this.font = font; this.rect = rect; this.index = index; this.state = state; this.foreColor = foreColor; this.backColor = backColor; } ///public Color BackColor { get { if ((state & DrawItemState.Selected) == DrawItemState.Selected) { return SystemColors.Highlight; } return backColor; } } /// /// /// The rectangle outlining the area in which the painting should be /// done. /// public Rectangle Bounds { get { return rect; } } ////// /// A suggested font, usually the parent control's Font property. /// public Font Font { get { return font; } } ////// /// A suggested color drawing: either SystemColors.WindowText or SystemColors.HighlightText, /// depending on whether this item is selected. /// public Color ForeColor { get { if ((state & DrawItemState.Selected) == DrawItemState.Selected) { return SystemColors.HighlightText; } return foreColor; } } ////// /// Graphics object with which painting should be done. /// public Graphics Graphics { get { return graphics; } } ////// /// The index of the item that should be painted. /// public int Index { get { return index; } } ////// /// Miscellaneous state information, such as whether the item is /// "selected", "focused", or some other such information. ComboBoxes /// have one special piece of information which indicates if the item /// being painted is the editable portion of the ComboBox. /// public DrawItemState State { get { return state; } } ////// /// Draws the background of the given rectangle with the color returned from the BackColor property. /// public virtual void DrawBackground() { Brush backBrush = new SolidBrush(BackColor); Graphics.FillRectangle(backBrush, rect); backBrush.Dispose(); } ////// /// Draws a handy focus rect in the given rectangle. /// public virtual void DrawFocusRectangle() { if ((state & DrawItemState.Focus) == DrawItemState.Focus && (state & DrawItemState.NoFocusRect) != DrawItemState.NoFocusRect) ControlPaint.DrawFocusRectangle(Graphics, rect, ForeColor, BackColor); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
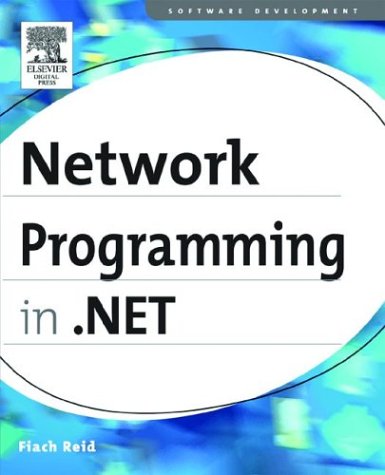
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebPartConnectionCollection.cs
- Process.cs
- DataGridViewColumn.cs
- WebAdminConfigurationHelper.cs
- ArrayMergeHelper.cs
- DynamicResourceExtension.cs
- mil_sdk_version.cs
- XmlnsPrefixAttribute.cs
- XmlKeywords.cs
- CodePropertyReferenceExpression.cs
- ParserExtension.cs
- ImageList.cs
- ReadOnlyDictionary.cs
- AssemblyContextControlItem.cs
- WebConfigurationFileMap.cs
- SchemaTypeEmitter.cs
- XomlCompilerParameters.cs
- ReflectPropertyDescriptor.cs
- InkCanvasAutomationPeer.cs
- ObsoleteAttribute.cs
- Style.cs
- ElementHostPropertyMap.cs
- CollectionDataContractAttribute.cs
- PseudoWebRequest.cs
- DeferredSelectedIndexReference.cs
- XmlHierarchicalDataSourceView.cs
- XpsColorContext.cs
- XsdBuilder.cs
- FaultImportOptions.cs
- WizardStepBase.cs
- GridViewUpdateEventArgs.cs
- HashMembershipCondition.cs
- CancelEventArgs.cs
- BooleanToSelectiveScrollingOrientationConverter.cs
- OleDbRowUpdatedEvent.cs
- DetailsViewInsertEventArgs.cs
- precedingquery.cs
- CompareValidator.cs
- CodeRegionDirective.cs
- ConstraintCollection.cs
- followingsibling.cs
- WebPartManager.cs
- Transform3DCollection.cs
- FixedPage.cs
- TableProviderWrapper.cs
- LinkArea.cs
- StaticTextPointer.cs
- CanonicalXml.cs
- DataGridViewCellConverter.cs
- X509Extension.cs
- MembershipPasswordException.cs
- OLEDB_Util.cs
- ArraySet.cs
- LinqDataSourceEditData.cs
- PersonalizationStateInfoCollection.cs
- UpdateManifestForBrowserApplication.cs
- TextEffectResolver.cs
- BooleanSwitch.cs
- ValidationErrorEventArgs.cs
- OrderedEnumerableRowCollection.cs
- RoleGroup.cs
- HandlerBase.cs
- ReaderWriterLockWrapper.cs
- TypedDataSetSchemaImporterExtension.cs
- ApplicationInterop.cs
- Sql8ConformanceChecker.cs
- Quad.cs
- FontFamilyIdentifier.cs
- StorageSetMapping.cs
- FullTrustAssemblyCollection.cs
- OperationBehaviorAttribute.cs
- XMLUtil.cs
- SspiWrapper.cs
- TreeViewBindingsEditor.cs
- ModelTreeManager.cs
- BitmapImage.cs
- PropertyInfoSet.cs
- LocatorPartList.cs
- AtomPub10ServiceDocumentFormatter.cs
- DeleteHelper.cs
- NativeMethodsOther.cs
- COM2IDispatchConverter.cs
- DateTimeConverter2.cs
- BinHexEncoder.cs
- SaveRecipientRequest.cs
- StoragePropertyMapping.cs
- XmlSchemaExporter.cs
- SortedList.cs
- TemplateInstanceAttribute.cs
- DataGridDetailsPresenterAutomationPeer.cs
- HttpSessionStateBase.cs
- ReadContentAsBinaryHelper.cs
- WebConfigurationHost.cs
- WindowsTitleBar.cs
- SQLMoney.cs
- VisualTreeHelper.cs
- AvTraceFormat.cs
- UnsafeNativeMethods.cs
- ComplexPropertyEntry.cs
- Annotation.cs