Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Objects / CompiledQuery.cs / 1305376 / CompiledQuery.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupowner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Linq.Expressions; using System.Data.Objects.ELinq; using System.Diagnostics; using System.Data.Objects.Internal; using OM = System.Collections.ObjectModel; namespace System.Data.Objects { ////// Caches an ELinq query /// public sealed class CompiledQuery { // NOTE: make sure all changes to this object keep it immutable // so it won't have any thread saftey concerns private readonly LambdaExpression _query; private readonly Guid _cacheToken = Guid.NewGuid(); ////// Constructs a new compiled query instance which hosts the delegate returned to the user /// (one of the Invoke overloads). /// /// Compiled query expression. /// The type of the delegate producing parameter values from CompiledQuery /// delegate arguments. For details, see CompiledQuery.Parameter.CreateObjectParameter. private CompiledQuery(LambdaExpression query) { EntityUtil.CheckArgumentNull(query, "query"); // lockdown the query (all closures become constants) Funcletizer funcletizer = Funcletizer.CreateCompiledQueryLockdownFuncletizer(); FuncrecompiledRequire; _query = (LambdaExpression)funcletizer.Funcletize(query, out recompiledRequire); } /// /// Creates a CompiledQuery delegate from an ELinq expression. /// ///An ObjectContext derived type ///The scalar type of parameter 1. ///The scalar type of parameter 2. ///The scalar type of parameter 3. ///The scalar type of parameter 4. ///The scalar type of parameter 5. ///The scalar type of parameter 6. ///The scalar type of parameter 7. ///The scalar type of parameter 8. ///The scalar type of parameter 9. ///The scalar type of parameter 10. ///The scalar type of parameter 11. ///The scalar type of parameter 12. ///The scalar type of parameter 13. ///The scalar type of parameter 14. ///The scalar type of parameter 15. ///The return type of the delegate. /// The lambda expression to compile. ///The CompiledQuery delegate. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "required for this feature")] public static FuncCompile (Expression > query) where TArg0 : ObjectContext { return new CompiledQuery(query).Invoke ; } /// /// Creates a CompiledQuery delegate from an ELinq expression. /// ///An ObjectContext derived type ///The scalar type of parameter 1. ///The scalar type of parameter 2. ///The scalar type of parameter 3. ///The scalar type of parameter 4. ///The scalar type of parameter 5. ///The scalar type of parameter 6. ///The scalar type of parameter 7. ///The scalar type of parameter 8. ///The scalar type of parameter 9. ///The scalar type of parameter 10. ///The scalar type of parameter 11. ///The scalar type of parameter 12. ///The scalar type of parameter 13. ///The scalar type of parameter 14. ///The return type of the delegate. /// The lambda expression to compile. ///The CompiledQuery delegate. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "required for this feature")] public static FuncCompile (Expression > query) where TArg0 : ObjectContext { return new CompiledQuery(query).Invoke ; } /// /// Creates a CompiledQuery delegate from an ELinq expression. /// ///An ObjectContext derived type ///The scalar type of parameter 1. ///The scalar type of parameter 2. ///The scalar type of parameter 3. ///The scalar type of parameter 4. ///The scalar type of parameter 5. ///The scalar type of parameter 6. ///The scalar type of parameter 7. ///The scalar type of parameter 8. ///The scalar type of parameter 9. ///The scalar type of parameter 10. ///The scalar type of parameter 11. ///The scalar type of parameter 12. ///The scalar type of parameter 13. ///The return type of the delegate. /// The lambda expression to compile. ///The CompiledQuery delegate. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "required for this feature")] public static FuncCompile (Expression > query) where TArg0 : ObjectContext { return new CompiledQuery(query).Invoke ; } /// /// Creates a CompiledQuery delegate from an ELinq expression. /// ///An ObjectContext derived type ///The scalar type of parameter 1. ///The scalar type of parameter 2. ///The scalar type of parameter 3. ///The scalar type of parameter 4. ///The scalar type of parameter 5. ///The scalar type of parameter 6. ///The scalar type of parameter 7. ///The scalar type of parameter 8. ///The scalar type of parameter 9. ///The scalar type of parameter 10. ///The scalar type of parameter 11. ///The scalar type of parameter 12. ///The return type of the delegate. /// The lambda expression to compile. ///The CompiledQuery delegate. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "required for this feature")] public static FuncCompile (Expression > query) where TArg0 : ObjectContext { return new CompiledQuery(query).Invoke ; } /// /// Creates a CompiledQuery delegate from an ELinq expression. /// ///An ObjectContext derived type ///The scalar type of parameter 1. ///The scalar type of parameter 2. ///The scalar type of parameter 3. ///The scalar type of parameter 4. ///The scalar type of parameter 5. ///The scalar type of parameter 6. ///The scalar type of parameter 7. ///The scalar type of parameter 8. ///The scalar type of parameter 9. ///The scalar type of parameter 10. ///The scalar type of parameter 11. ///The return type of the delegate. /// The lambda expression to compile. ///The CompiledQuery delegate. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "required for this feature")] public static FuncCompile (Expression > query) where TArg0 : ObjectContext { return new CompiledQuery(query).Invoke ; } /// /// Creates a CompiledQuery delegate from an ELinq expression. /// ///An ObjectContext derived type ///The scalar type of parameter 1. ///The scalar type of parameter 2. ///The scalar type of parameter 3. ///The scalar type of parameter 4. ///The scalar type of parameter 5. ///The scalar type of parameter 6. ///The scalar type of parameter 7. ///The scalar type of parameter 8. ///The scalar type of parameter 9. ///The scalar type of parameter 10. ///The return type of the delegate. /// The lambda expression to compile. ///The CompiledQuery delegate. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "required for this feature")] public static FuncCompile (Expression > query) where TArg0 : ObjectContext { return new CompiledQuery(query).Invoke ; } /// /// Creates a CompiledQuery delegate from an ELinq expression. /// ///An ObjectContext derived type ///The scalar type of parameter 1. ///The scalar type of parameter 2. ///The scalar type of parameter 3. ///The scalar type of parameter 4. ///The scalar type of parameter 5. ///The scalar type of parameter 6. ///The scalar type of parameter 7. ///The scalar type of parameter 8. ///The scalar type of parameter 9. ///The return type of the delegate. /// The lambda expression to compile. ///The CompiledQuery delegate. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "required for this feature")] public static FuncCompile (Expression > query) where TArg0 : ObjectContext { return new CompiledQuery(query).Invoke ; } /// /// Creates a CompiledQuery delegate from an ELinq expression. /// ///An ObjectContext derived type ///The scalar type of parameter 1. ///The scalar type of parameter 2. ///The scalar type of parameter 3. ///The scalar type of parameter 4. ///The scalar type of parameter 5. ///The scalar type of parameter 6. ///The scalar type of parameter 7. ///The scalar type of parameter 8. ///The return type of the delegate. /// The lambda expression to compile. ///The CompiledQuery delegate. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "required for this feature")] public static FuncCompile (Expression > query) where TArg0 : ObjectContext { return new CompiledQuery(query).Invoke ; } /// /// Creates a CompiledQuery delegate from an ELinq expression. /// ///An ObjectContext derived type ///The scalar type of parameter 1. ///The scalar type of parameter 2. ///The scalar type of parameter 3. ///The scalar type of parameter 4. ///The scalar type of parameter 5. ///The scalar type of parameter 6. ///The scalar type of parameter 7. ///The return type of the delegate. /// The lambda expression to compile. ///The CompiledQuery delegate. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "required for this feature")] public static FuncCompile (Expression > query) where TArg0 : ObjectContext { return new CompiledQuery(query).Invoke ; } /// /// Creates a CompiledQuery delegate from an ELinq expression. /// ///An ObjectContext derived type ///The scalar type of parameter 1. ///The scalar type of parameter 2. ///The scalar type of parameter 3. ///The scalar type of parameter 4. ///The scalar type of parameter 5. ///The scalar type of parameter 6. ///The return type of the delegate. /// The lambda expression to compile. ///The CompiledQuery delegate. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "required for this feature")] public static FuncCompile (Expression > query) where TArg0 : ObjectContext { return new CompiledQuery(query).Invoke ; } /// /// Creates a CompiledQuery delegate from an ELinq expression. /// ///An ObjectContext derived type ///The scalar type of parameter 1. ///The scalar type of parameter 2. ///The scalar type of parameter 3. ///The scalar type of parameter 4. ///The scalar type of parameter 5. ///The return type of the delegate. /// The lambda expression to compile. ///The CompiledQuery delegate. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "required for this feature")] public static FuncCompile (Expression > query) where TArg0 : ObjectContext { return new CompiledQuery(query).Invoke ; } /// /// Creates a CompiledQuery delegate from an ELinq expression. /// ///An ObjectContext derived type ///The scalar type of parameter 1. ///The scalar type of parameter 2. ///The scalar type of parameter 3. ///The scalar type of parameter 4. ///The return type of the delegate. /// The lambda expression to compile. ///The CompiledQuery delegate. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "required for this feature")] public static FuncCompile (Expression > query) where TArg0 : ObjectContext { return new CompiledQuery(query).Invoke ; } /// /// Creates a CompiledQuery delegate from an ELinq expression. /// ///An ObjectContext derived type ///The scalar type of parameter 1. ///The scalar type of parameter 2. ///The scalar type of parameter 3. ///The return type of the delegate. /// The lambda expression to compile. ///The CompiledQuery delegate. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification="required for this feature")] public static FuncCompile (Expression > query) where TArg0 : ObjectContext { return new CompiledQuery(query).Invoke ; } /// /// Creates a CompiledQuery delegate from an ELinq expression. /// ///An ObjectContext derived type ///The scalar type of parameter 1. ///The scalar type of parameter 2. ///The return type of the delegate. /// The lambda expression to compile. ///The CompiledQuery delegate. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "required for this feature")] public static FuncCompile (Expression > query) where TArg0 : ObjectContext { return new CompiledQuery(query).Invoke ; } /// /// Creates a CompiledQuery delegate from an ELinq expression. /// ///An ObjectContext derived type ///The scalar type of parameter 1. ///The return type of the delegate. /// The lambda expression to compile. ///The CompiledQuery delegate. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "required for this feature")] public static FuncCompile (Expression > query) where TArg0 : ObjectContext { return new CompiledQuery(query).Invoke ; } /// /// Creates a CompiledQuery delegate from an ELinq expression. /// ///An ObjectContext derived type ///The return type of the delegate. /// The lambda expression to compile. ///The CompiledQuery delegate. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters", Justification = "required for this feature")] public static FuncCompile (Expression > query) where TArg0 : ObjectContext { return new CompiledQuery(query).Invoke ; } private TResult Invoke (TArg0 arg0) where TArg0 : ObjectContext { EntityUtil.CheckArgumentNull(arg0, "arg0"); // SQLBUDT 447285: Ensure the assembly containing the entity's CLR type is loaded into the workspace. // This method must ensure that the O-Space metadata for TResultType is correctly loaded - it is the equivalent // of a public constructor for compiled queries, since it is returned as a delegate and called as a public entry point. arg0.MetadataWorkspace.ImplicitLoadAssemblyForType(typeof(TResult), System.Reflection.Assembly.GetCallingAssembly()); return ExecuteQuery (arg0); } private TResult Invoke (TArg0 arg0, TArg1 arg1) where TArg0 : ObjectContext { EntityUtil.CheckArgumentNull(arg0, "arg0"); // SQLBUDT 447285: Ensure the assembly containing the entity's CLR type is loaded into the workspace. // This method must ensure that the O-Space metadata for TResultType is correctly loaded - it is the equivalent // of a public constructor for compiled queries, since it is returned as a delegate and called as a public entry point. arg0.MetadataWorkspace.ImplicitLoadAssemblyForType(typeof(TResult), System.Reflection.Assembly.GetCallingAssembly()); return ExecuteQuery (arg0, arg1); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2) where TArg0 : ObjectContext { EntityUtil.CheckArgumentNull(arg0, "arg0"); // SQLBUDT 447285: Ensure the assembly containing the entity's CLR type is loaded into the workspace. // This method must ensure that the O-Space metadata for TResultType is correctly loaded - it is the equivalent // of a public constructor for compiled queries, since it is returned as a delegate and called as a public entry point. arg0.MetadataWorkspace.ImplicitLoadAssemblyForType(typeof(TResult), System.Reflection.Assembly.GetCallingAssembly()); return ExecuteQuery (arg0, arg1, arg2); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3) where TArg0 : ObjectContext { EntityUtil.CheckArgumentNull(arg0, "arg0"); // SQLBUDT 447285: Ensure the assembly containing the entity's CLR type is loaded into the workspace. // This method must ensure that the O-Space metadata for TResultType is correctly loaded - it is the equivalent // of a public constructor for compiled queries, since it is returned as a delegate and called as a public entry point. arg0.MetadataWorkspace.ImplicitLoadAssemblyForType(typeof(TResult), System.Reflection.Assembly.GetCallingAssembly()); return ExecuteQuery (arg0, arg1, arg2, arg3); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4) where TArg0 : ObjectContext { EntityUtil.CheckArgumentNull(arg0, "arg0"); // SQLBUDT 447285: Ensure the assembly containing the entity's CLR type is loaded into the workspace. // This method must ensure that the O-Space metadata for TResultType is correctly loaded - it is the equivalent // of a public constructor for compiled queries, since it is returned as a delegate and called as a public entry point. arg0.MetadataWorkspace.ImplicitLoadAssemblyForType(typeof(TResult), System.Reflection.Assembly.GetCallingAssembly()); return ExecuteQuery (arg0, arg1, arg2, arg3, arg4); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5) where TArg0 : ObjectContext { EntityUtil.CheckArgumentNull(arg0, "arg0"); // SQLBUDT 447285: Ensure the assembly containing the entity's CLR type is loaded into the workspace. // This method must ensure that the O-Space metadata for TResultType is correctly loaded - it is the equivalent // of a public constructor for compiled queries, since it is returned as a delegate and called as a public entry point. arg0.MetadataWorkspace.ImplicitLoadAssemblyForType(typeof(TResult), System.Reflection.Assembly.GetCallingAssembly()); return ExecuteQuery (arg0, arg1, arg2, arg3, arg4, arg5); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6) where TArg0 : ObjectContext { EntityUtil.CheckArgumentNull(arg0, "arg0"); // SQLBUDT 447285: Ensure the assembly containing the entity's CLR type is loaded into the workspace. // This method must ensure that the O-Space metadata for TResultType is correctly loaded - it is the equivalent // of a public constructor for compiled queries, since it is returned as a delegate and called as a public entry point. arg0.MetadataWorkspace.ImplicitLoadAssemblyForType(typeof(TResult), System.Reflection.Assembly.GetCallingAssembly()); return ExecuteQuery (arg0, arg1, arg2, arg3, arg4, arg5, arg6); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7) where TArg0 : ObjectContext { EntityUtil.CheckArgumentNull(arg0, "arg0"); // SQLBUDT 447285: Ensure the assembly containing the entity's CLR type is loaded into the workspace. // This method must ensure that the O-Space metadata for TResultType is correctly loaded - it is the equivalent // of a public constructor for compiled queries, since it is returned as a delegate and called as a public entry point. arg0.MetadataWorkspace.ImplicitLoadAssemblyForType(typeof(TResult), System.Reflection.Assembly.GetCallingAssembly()); return ExecuteQuery (arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8) where TArg0 : ObjectContext { EntityUtil.CheckArgumentNull(arg0, "arg0"); // SQLBUDT 447285: Ensure the assembly containing the entity's CLR type is loaded into the workspace. // This method must ensure that the O-Space metadata for TResultType is correctly loaded - it is the equivalent // of a public constructor for compiled queries, since it is returned as a delegate and called as a public entry point. arg0.MetadataWorkspace.ImplicitLoadAssemblyForType(typeof(TResult), System.Reflection.Assembly.GetCallingAssembly()); return ExecuteQuery (arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9) where TArg0 : ObjectContext { EntityUtil.CheckArgumentNull(arg0, "arg0"); // SQLBUDT 447285: Ensure the assembly containing the entity's CLR type is loaded into the workspace. // This method must ensure that the O-Space metadata for TResultType is correctly loaded - it is the equivalent // of a public constructor for compiled queries, since it is returned as a delegate and called as a public entry point. arg0.MetadataWorkspace.ImplicitLoadAssemblyForType(typeof(TResult), System.Reflection.Assembly.GetCallingAssembly()); return ExecuteQuery (arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9, TArg10 arg10) where TArg0 : ObjectContext { EntityUtil.CheckArgumentNull(arg0, "arg0"); // SQLBUDT 447285: Ensure the assembly containing the entity's CLR type is loaded into the workspace. // This method must ensure that the O-Space metadata for TResultType is correctly loaded - it is the equivalent // of a public constructor for compiled queries, since it is returned as a delegate and called as a public entry point. arg0.MetadataWorkspace.ImplicitLoadAssemblyForType(typeof(TResult), System.Reflection.Assembly.GetCallingAssembly()); return ExecuteQuery (arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9, arg10); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9, TArg10 arg10, TArg11 arg11) where TArg0 : ObjectContext { EntityUtil.CheckArgumentNull(arg0, "arg0"); // SQLBUDT 447285: Ensure the assembly containing the entity's CLR type is loaded into the workspace. // This method must ensure that the O-Space metadata for TResultType is correctly loaded - it is the equivalent // of a public constructor for compiled queries, since it is returned as a delegate and called as a public entry point. arg0.MetadataWorkspace.ImplicitLoadAssemblyForType(typeof(TResult), System.Reflection.Assembly.GetCallingAssembly()); return ExecuteQuery (arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9, arg10, arg11); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9, TArg10 arg10, TArg11 arg11, TArg12 arg12) where TArg0 : ObjectContext { EntityUtil.CheckArgumentNull(arg0, "arg0"); // SQLBUDT 447285: Ensure the assembly containing the entity's CLR type is loaded into the workspace. // This method must ensure that the O-Space metadata for TResultType is correctly loaded - it is the equivalent // of a public constructor for compiled queries, since it is returned as a delegate and called as a public entry point. arg0.MetadataWorkspace.ImplicitLoadAssemblyForType(typeof(TResult), System.Reflection.Assembly.GetCallingAssembly()); return ExecuteQuery (arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9, arg10, arg11, arg12); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9, TArg10 arg10, TArg11 arg11, TArg12 arg12, TArg13 arg13) where TArg0 : ObjectContext { EntityUtil.CheckArgumentNull(arg0, "arg0"); // SQLBUDT 447285: Ensure the assembly containing the entity's CLR type is loaded into the workspace. // This method must ensure that the O-Space metadata for TResultType is correctly loaded - it is the equivalent // of a public constructor for compiled queries, since it is returned as a delegate and called as a public entry point. arg0.MetadataWorkspace.ImplicitLoadAssemblyForType(typeof(TResult), System.Reflection.Assembly.GetCallingAssembly()); return ExecuteQuery (arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9, arg10, arg11, arg12, arg13); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9, TArg10 arg10, TArg11 arg11, TArg12 arg12, TArg13 arg13, TArg14 arg14) where TArg0 : ObjectContext { EntityUtil.CheckArgumentNull(arg0, "arg0"); // SQLBUDT 447285: Ensure the assembly containing the entity's CLR type is loaded into the workspace. // This method must ensure that the O-Space metadata for TResultType is correctly loaded - it is the equivalent // of a public constructor for compiled queries, since it is returned as a delegate and called as a public entry point. arg0.MetadataWorkspace.ImplicitLoadAssemblyForType(typeof(TResult), System.Reflection.Assembly.GetCallingAssembly()); return ExecuteQuery (arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9, arg10, arg11, arg12, arg13, arg14); } private TResult Invoke (TArg0 arg0, TArg1 arg1, TArg2 arg2, TArg3 arg3, TArg4 arg4, TArg5 arg5, TArg6 arg6, TArg7 arg7, TArg8 arg8, TArg9 arg9, TArg10 arg10, TArg11 arg11, TArg12 arg12, TArg13 arg13, TArg14 arg14, TArg15 arg15) where TArg0 : ObjectContext { EntityUtil.CheckArgumentNull(arg0, "arg0"); // SQLBUDT 447285: Ensure the assembly containing the entity's CLR type is loaded into the workspace. // This method must ensure that the O-Space metadata for TResultType is correctly loaded - it is the equivalent // of a public constructor for compiled queries, since it is returned as a delegate and called as a public entry point. arg0.MetadataWorkspace.ImplicitLoadAssemblyForType(typeof(TResult), System.Reflection.Assembly.GetCallingAssembly()); return ExecuteQuery (arg0, arg1, arg2, arg3, arg4, arg5, arg6, arg7, arg8, arg9, arg10, arg11, arg12, arg13, arg14, arg15); } private TResult ExecuteQuery (ObjectContext context, params object[] parameterValues) { bool isSingleton; Type elementType = GetElementType(typeof(TResult), out isSingleton); ObjectQueryState queryState = new CompiledELinqQueryState(elementType, context, _query, _cacheToken, parameterValues); System.Collections.IEnumerable query = queryState.CreateQuery(); if (isSingleton) { return ObjectQueryProvider.ExecuteSingle (Enumerable.Cast (query), _query); } else { return (TResult)query; } } /// /// This method is trying to distinguish between a set of types and a singleton type /// It also has the restriction that to be a set of types, it must be assignable from ObjectQuery<T> /// Otherwise we won't be able to cast our query to the set requested. /// /// The type asked for as a result type. /// Is it a set of a type. ///The element type to use private static Type GetElementType(Type resultType, out bool isSingleton) { Type elementType = TypeSystem.GetElementType(resultType); isSingleton = (elementType == resultType || !resultType.IsAssignableFrom(typeof(ObjectQuery<>).MakeGenericType(elementType))); if (isSingleton) { return resultType; } else { return elementType; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
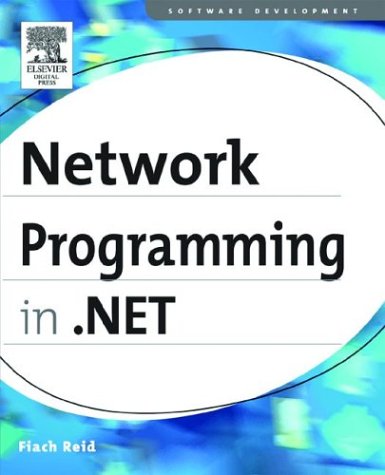
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SystemBrushes.cs
- DbConnectionPoolIdentity.cs
- CheckBoxField.cs
- AudioFormatConverter.cs
- HandlerBase.cs
- XmlUtil.cs
- DbConnectionStringCommon.cs
- FactoryMaker.cs
- FrameworkElement.cs
- WmlImageAdapter.cs
- SqlProvider.cs
- SortedList.cs
- ObjectDataSourceChooseTypePanel.cs
- SRDisplayNameAttribute.cs
- PropertyEmitter.cs
- SetterBase.cs
- NumberFormatInfo.cs
- UIPropertyMetadata.cs
- BorderSidesEditor.cs
- SoapEnumAttribute.cs
- XmlMapping.cs
- PermissionRequestEvidence.cs
- XmlHelper.cs
- TextHidden.cs
- MD5.cs
- ProxyManager.cs
- InputMethodStateChangeEventArgs.cs
- XmlLoader.cs
- WindowsTreeView.cs
- ContainsRowNumberChecker.cs
- xmlformatgeneratorstatics.cs
- TimelineClockCollection.cs
- _ConnectOverlappedAsyncResult.cs
- WindowsRichEditRange.cs
- OperationPerformanceCounters.cs
- CharConverter.cs
- RelativeSource.cs
- ProxyGenerationError.cs
- EdmToObjectNamespaceMap.cs
- XmlElementElement.cs
- CustomActivityDesigner.cs
- XmlTextReaderImplHelpers.cs
- DataGridViewUtilities.cs
- CustomTypeDescriptor.cs
- SecurityKeyIdentifierClause.cs
- ComponentRenameEvent.cs
- AppSettingsSection.cs
- DiagnosticsConfiguration.cs
- XamlGridLengthSerializer.cs
- PerfCounterSection.cs
- NameObjectCollectionBase.cs
- SystemWebExtensionsSectionGroup.cs
- SourceFilter.cs
- NavigationPropertySingletonExpression.cs
- BindingManagerDataErrorEventArgs.cs
- PolicyFactory.cs
- SQLByte.cs
- RepeatInfo.cs
- RuntimeCompatibilityAttribute.cs
- DesignSurface.cs
- ClientTargetCollection.cs
- ItemContainerGenerator.cs
- UserNameSecurityTokenProvider.cs
- NameTable.cs
- ZoneIdentityPermission.cs
- UniqueSet.cs
- RectangleHotSpot.cs
- HttpApplicationStateWrapper.cs
- PathData.cs
- XPathArrayIterator.cs
- IconHelper.cs
- SettingsPropertyIsReadOnlyException.cs
- DataSourceDesigner.cs
- GPRECT.cs
- ValidationEventArgs.cs
- DockAndAnchorLayout.cs
- RemotingConfiguration.cs
- StylusEditingBehavior.cs
- Substitution.cs
- FamilyMap.cs
- AddInStore.cs
- RequestBringIntoViewEventArgs.cs
- SerialReceived.cs
- WebAdminConfigurationHelper.cs
- DES.cs
- ByteAnimation.cs
- SmiSettersStream.cs
- DataSourceView.cs
- TcpActivation.cs
- ThreadNeutralSemaphore.cs
- AnonymousIdentificationModule.cs
- AttributeData.cs
- ConnectionStringsExpressionBuilder.cs
- DoubleIndependentAnimationStorage.cs
- DbReferenceCollection.cs
- ManagementOperationWatcher.cs
- SchemaConstraints.cs
- ProtocolsConfiguration.cs
- XmlWriterDelegator.cs
- ScrollPatternIdentifiers.cs