Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / DesignBindingPropertyDescriptor.cs / 1 / DesignBindingPropertyDescriptor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.Design { using System; using System.ComponentModel; ////// /// internal class DesignBindingPropertyDescriptor : PropertyDescriptor { private static TypeConverter designBindingConverter = new DesignBindingConverter(); private PropertyDescriptor property; private bool readOnly; // base.AttributeArray ends up calling the virtual FillAttributes, but we do not override it, so we should be okay. [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Usage", "CA2214:DoNotCallOverridableMethodsInConstructors")] internal DesignBindingPropertyDescriptor(PropertyDescriptor property, Attribute[] attrs, bool readOnly) : base(property.Name, attrs) { this.property = property; this.readOnly = readOnly; if (base.AttributeArray != null && base.AttributeArray.Length > 0) { Attribute[] newAttrs = new Attribute[AttributeArray.Length + 2]; AttributeArray.CopyTo(newAttrs, 0); newAttrs[AttributeArray.Length-1] = NotifyParentPropertyAttribute.Yes; newAttrs[AttributeArray.Length] = RefreshPropertiesAttribute.Repaint; base.AttributeArray = newAttrs; } else { base.AttributeArray = new Attribute[]{NotifyParentPropertyAttribute.Yes, RefreshPropertiesAttribute.Repaint}; } } ///Provides a property descriptor for design time data binding properties. ////// /// public override Type ComponentType { get { return typeof(ControlBindingsCollection); } } ///Gets or sets the type of the component that owns the property. ////// /// public override TypeConverter Converter { get { return designBindingConverter; } } ///Gets or sets the type converter. ////// /// public override bool IsReadOnly { get { return readOnly; } } ///Indicates whether the property is read-only. ////// /// public override Type PropertyType { get { return typeof(DesignBinding); } } ///Gets or sets the type of the property. ////// /// public override bool CanResetValue(object component) { return !GetBinding((ControlBindingsCollection)component, property).IsNull; } ///Gets a value indicating whether the specified component can reset the value /// of the property. ////// /// public override object GetValue(object component) { return GetBinding((ControlBindingsCollection)component, property); } ///Gets a value from the specified component. ////// /// public override void ResetValue(object component) { SetBinding((ControlBindingsCollection)component, property, DesignBinding.Null); } ///Resets the value of the specified component. ////// /// public override void SetValue(object component, object value) { SetBinding((ControlBindingsCollection)component, property, (DesignBinding)value); OnValueChanged(component, EventArgs.Empty); } ///Sets the specified value for the specified component. ////// /// public override bool ShouldSerializeValue(object component) { return false; } private static void SetBinding(ControlBindingsCollection bindings, PropertyDescriptor property, DesignBinding designBinding) { // this means it couldn't be parsed. if (designBinding == null) return; Binding listBinding = bindings[property.Name]; if (listBinding != null) { bindings.Remove(listBinding); } if (!designBinding.IsNull) { bindings.Add(property.Name, designBinding.DataSource, designBinding.DataMember); } } private static DesignBinding GetBinding(ControlBindingsCollection bindings, PropertyDescriptor property) { Binding listBinding = bindings[property.Name]; if (listBinding == null) return DesignBinding.Null; else return new DesignBinding(listBinding.DataSource, listBinding.BindingMemberInfo.BindingMember); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.Indicates whether the specified component should persist the value. ///
Link Menu
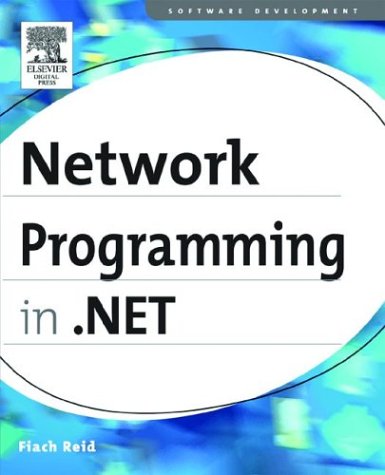
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SettingsAttributes.cs
- NativeMethods.cs
- OrthographicCamera.cs
- InlineObject.cs
- KnownBoxes.cs
- TemplateKeyConverter.cs
- XmlWrappingReader.cs
- Animatable.cs
- HighContrastHelper.cs
- OdbcDataAdapter.cs
- HttpProfileGroupBase.cs
- HandledEventArgs.cs
- Splitter.cs
- Path.cs
- ButtonAutomationPeer.cs
- SByteConverter.cs
- isolationinterop.cs
- PlainXmlDeserializer.cs
- ContentFilePart.cs
- HttpValueCollection.cs
- ToolStripComboBox.cs
- NamedPermissionSet.cs
- TypeUsage.cs
- MediaPlayerState.cs
- ExpanderAutomationPeer.cs
- UnsafeNativeMethods.cs
- DateTimeOffsetAdapter.cs
- XmlAttributeOverrides.cs
- ActivationServices.cs
- HwndTarget.cs
- ButtonField.cs
- ColumnWidthChangedEvent.cs
- path.cs
- SqlConnectionPoolProviderInfo.cs
- XPathSingletonIterator.cs
- XmlBinaryWriter.cs
- SqlFunctionAttribute.cs
- linebase.cs
- Queue.cs
- MobileControl.cs
- SystemThemeKey.cs
- InteropAutomationProvider.cs
- DependencyPropertyChangedEventArgs.cs
- HtmlTableCell.cs
- input.cs
- ActivitiesCollection.cs
- DiagnosticTraceSource.cs
- JournalEntry.cs
- CqlBlock.cs
- EvidenceBase.cs
- Context.cs
- ControlCodeDomSerializer.cs
- METAHEADER.cs
- FileLogRecordHeader.cs
- CodeCommentStatementCollection.cs
- SqlInternalConnectionSmi.cs
- PingOptions.cs
- FormViewPageEventArgs.cs
- CfgArc.cs
- WorkflowEventArgs.cs
- WebBaseEventKeyComparer.cs
- SqlBulkCopy.cs
- DesignerObject.cs
- BaseAsyncResult.cs
- SendKeys.cs
- TypeToken.cs
- LinqDataSourceHelper.cs
- TypeReference.cs
- KeyFrames.cs
- VectorCollectionConverter.cs
- TemplatePropertyEntry.cs
- AspNetCacheProfileAttribute.cs
- WindowsListView.cs
- WinFormsComponentEditor.cs
- FontStyles.cs
- DesignParameter.cs
- NullRuntimeConfig.cs
- EntityDataSourceDesigner.cs
- AccessDataSource.cs
- Int16.cs
- OracleConnectionFactory.cs
- FieldToken.cs
- AutomationProperty.cs
- SemanticAnalyzer.cs
- PreviewPrintController.cs
- WhitespaceRuleLookup.cs
- XmlArrayAttribute.cs
- DocumentViewer.cs
- ETagAttribute.cs
- QuotedPrintableStream.cs
- ListBoxDesigner.cs
- RSAProtectedConfigurationProvider.cs
- WindowPatternIdentifiers.cs
- DataGridPagerStyle.cs
- FontNameEditor.cs
- ConfigXmlElement.cs
- XmlNodeChangedEventManager.cs
- ClientSponsor.cs
- GridSplitter.cs
- Compiler.cs