Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Designer / WinForms / System / WinForms / Design / ListBoxDesigner.cs / 1 / ListBoxDesigner.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ [assembly: System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode", Scope="member", Target="System.Windows.Forms.Design.ListBoxDesigner..ctor()")] namespace System.Windows.Forms.Design { using System.Design; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System; using System.ComponentModel.Design; using System.Windows.Forms; using System.Drawing; using System.Collections; ////// /// This class handles all design time behavior for the list box class. /// It adds a sample item to the list box at design time. /// internal class ListBoxDesigner : ControlDesigner { private DesignerActionListCollection _actionLists; ////// /// Destroys this designer. /// protected override void Dispose(bool disposing) { if (disposing) { // Now, hook the component rename event so we can update the text in the // list box. // IComponentChangeService cs = (IComponentChangeService)GetService(typeof(IComponentChangeService)); if (cs != null) { cs.ComponentRename -= new ComponentRenameEventHandler(this.OnComponentRename); cs.ComponentChanged -= new ComponentChangedEventHandler(this.OnComponentChanged); } } base.Dispose(disposing); } ////// /// Called by the host when we're first initialized. /// public override void Initialize(IComponent component) { base.Initialize(component); AutoResizeHandles = true; // Now, hook the component rename event so we can update the text in the // list box. // IComponentChangeService cs = (IComponentChangeService)GetService(typeof(IComponentChangeService)); if (cs != null) { cs.ComponentRename += new ComponentRenameEventHandler(this.OnComponentRename); cs.ComponentChanged += new ComponentChangedEventHandler(this.OnComponentChanged); } } public override void InitializeNewComponent(IDictionary defaultValues) { base.InitializeNewComponent(defaultValues); // in Whidbey, formattingEnabled is true ((ListBox) this.Component).FormattingEnabled = true; // VSWhidbey 497239 - Setting FormattingEnabled clears the text we set in // OnCreateHandle so let's set it here again. We need to keep setting the text in // OnCreateHandle, otherwise we introduce VSWhidbey 498162. PropertyDescriptor nameProp = TypeDescriptor.GetProperties(Component)["Name"]; if (nameProp != null) { UpdateControlName(nameProp.GetValue(Component).ToString()); } } ////// /// Raised when a component's name changes. Here we update the contents of the list box /// if we are displaying the component's name in it. /// private void OnComponentRename(object sender, ComponentRenameEventArgs e) { if (e.Component == Component) { UpdateControlName(e.NewName); } } ////// /// Raised when ComponentChanges. We listen to this to check if the "Items" propertychanged. /// and if so .. then update the Text within the ListBox. /// private void OnComponentChanged(object sender, ComponentChangedEventArgs e) { if (e.Component == Component && e.Member != null && e.Member.Name == "Items") { PropertyDescriptor nameProp = TypeDescriptor.GetProperties(Component)["Name"]; if (nameProp != null) { UpdateControlName(nameProp.GetValue(Component).ToString()); } } } ////// /// This is called immediately after the control handle has been created. /// protected override void OnCreateHandle() { base.OnCreateHandle(); PropertyDescriptor nameProp = TypeDescriptor.GetProperties(Component)["Name"]; if (nameProp != null) { UpdateControlName(nameProp.GetValue(Component).ToString()); } } ////// /// Updates the name being displayed on this control. This will do nothing if /// the control has items in it. /// private void UpdateControlName(string name) { ListBox lb = (ListBox)Control; if (lb.IsHandleCreated && lb.Items.Count == 0) { NativeMethods.SendMessage(lb.Handle, NativeMethods.LB_RESETCONTENT, 0, 0); NativeMethods.SendMessage(lb.Handle, NativeMethods.LB_ADDSTRING, 0, name); } } public override DesignerActionListCollection ActionLists { get { if (_actionLists == null) { _actionLists = new DesignerActionListCollection(); if (this.Component is CheckedListBox) { _actionLists.Add(new ListControlUnboundActionList(this)); } else { _actionLists.Add(new ListControlBoundActionList(this)); } } return _actionLists; } } } internal class ListControlUnboundActionList : DesignerActionList { private ComponentDesigner _designer; public ListControlUnboundActionList(ComponentDesigner designer) : base(designer.Component) { _designer = designer; } public void InvokeItemsDialog() { EditorServiceContext.EditValue(_designer, Component, "Items"); } public override DesignerActionItemCollection GetSortedActionItems() { DesignerActionItemCollection returnItems = new DesignerActionItemCollection(); returnItems.Add(new DesignerActionMethodItem(this, "InvokeItemsDialog", SR.GetString(SR.ListControlUnboundActionListEditItemsDisplayName), SR.GetString(SR.ItemsCategoryName), SR.GetString(SR.ListControlUnboundActionListEditItemsDescription), true)); return returnItems; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
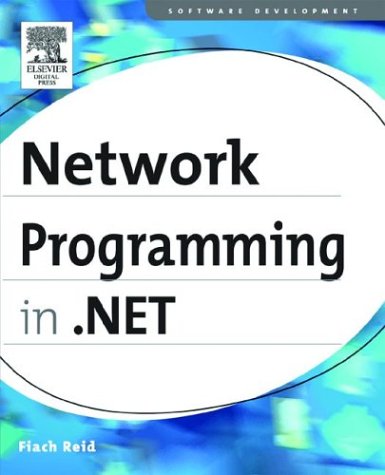
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TransactionWaitAsyncResult.cs
- WebPartRestoreVerb.cs
- NegatedConstant.cs
- ElementAction.cs
- BadImageFormatException.cs
- ImageCodecInfo.cs
- XmlSchemaAnnotated.cs
- SerializationHelper.cs
- HelloMessageCD1.cs
- SoapAttributes.cs
- SignatureDescription.cs
- SqlBuffer.cs
- BulletedList.cs
- XmlNotation.cs
- VoiceChangeEventArgs.cs
- Converter.cs
- Encoder.cs
- ProxyHwnd.cs
- GlyphRun.cs
- IntegrationExceptionEventArgs.cs
- SocketInformation.cs
- Pen.cs
- MailBnfHelper.cs
- DnsCache.cs
- Rect3DConverter.cs
- VisualCollection.cs
- ProviderSettingsCollection.cs
- MsmqOutputSessionChannel.cs
- FrameworkPropertyMetadata.cs
- ManifestSignedXml.cs
- LineSegment.cs
- QueryProcessor.cs
- FamilyCollection.cs
- WorkflowDesigner.cs
- GlyphRun.cs
- ITreeGenerator.cs
- TableAutomationPeer.cs
- ReferencedType.cs
- ColumnWidthChangingEvent.cs
- SqlSupersetValidator.cs
- AutoGeneratedFieldProperties.cs
- AppDomainCompilerProxy.cs
- DropShadowEffect.cs
- XmlLoader.cs
- WindowsFormsHostAutomationPeer.cs
- TypeSystem.cs
- SingleAnimationBase.cs
- WebPartPersonalization.cs
- Internal.cs
- GuidTagList.cs
- ProfileSettingsCollection.cs
- OdbcEnvironment.cs
- DetailsViewModeEventArgs.cs
- Win32.cs
- GenericAuthenticationEventArgs.cs
- QilChoice.cs
- MiniMapControl.xaml.cs
- LongAverageAggregationOperator.cs
- PenThreadPool.cs
- SignedInfo.cs
- ClientFormsAuthenticationMembershipProvider.cs
- UrlParameterWriter.cs
- ServiceManagerHandle.cs
- XmlElementList.cs
- QuaternionIndependentAnimationStorage.cs
- FatalException.cs
- DataServiceRequest.cs
- Terminate.cs
- TextFindEngine.cs
- RunInstallerAttribute.cs
- IntSecurity.cs
- ToolStripRenderEventArgs.cs
- HashSetDebugView.cs
- ReaderWriterLockWrapper.cs
- HtmlInputHidden.cs
- SelectorItemAutomationPeer.cs
- ExcCanonicalXml.cs
- UserControlFileEditor.cs
- PeerNameRecord.cs
- PeerValidationBehavior.cs
- EdmItemCollection.cs
- BasicCellRelation.cs
- KeyboardDevice.cs
- Int32CAMarshaler.cs
- LockedAssemblyCache.cs
- ToolboxDataAttribute.cs
- OutgoingWebRequestContext.cs
- Size.cs
- XmlWriterSettings.cs
- JournalEntryListConverter.cs
- Utils.cs
- MobileTextWriter.cs
- AttachedPropertyBrowsableForChildrenAttribute.cs
- baseaxisquery.cs
- _Win32.cs
- CodeStatementCollection.cs
- InternalResources.cs
- CollectionsUtil.cs
- HwndSourceParameters.cs
- FunctionParameter.cs