Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / QueryOperators / Inlined / LongAverageAggregationOperator.cs / 1305376 / LongAverageAggregationOperator.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // LongAverageAggregationOperator.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined average aggregation operator and its enumerator, for longs. /// internal sealed class LongAverageAggregationOperator : InlinedAggregationOperator, double> { //---------------------------------------------------------------------------------------- // Constructs a new instance of an average associative operator. // internal LongAverageAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override double InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator > enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // Throw an error for empty results. if (!enumerator.MoveNext()) { singularExceptionToThrow = new InvalidOperationException(SR.GetString(SR.NoElements)); return default(double); } Pair result = enumerator.Current; // Simply add together the sums and totals. while (enumerator.MoveNext()) { checked { result.First += enumerator.Current.First; result.Second += enumerator.Current.Second; } } // And divide the sum by the total to obtain the final result. return (double)result.First / result.Second; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator , int> CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new LongAverageAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class LongAverageAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator > { private QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal LongAverageAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the average of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref Pair currentElement) { // The temporary result contains the running sum and count, respectively. long sum = 0; long count = 0; QueryOperatorEnumerator source = m_source; long current = default(long); TKey keyUnused = default(TKey); if (source.MoveNext(ref current, ref keyUnused)) { int i = 0; do { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); checked { sum += current; count++; } } while (source.MoveNext(ref current, ref keyUnused)); currentElement = new Pair (sum, count); return true; } return false; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // LongAverageAggregationOperator.cs // // [....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; using System.Diagnostics.Contracts; using System.Threading; namespace System.Linq.Parallel { ////// An inlined average aggregation operator and its enumerator, for longs. /// internal sealed class LongAverageAggregationOperator : InlinedAggregationOperator, double> { //---------------------------------------------------------------------------------------- // Constructs a new instance of an average associative operator. // internal LongAverageAggregationOperator(IEnumerable child) : base(child) { } //--------------------------------------------------------------------------------------- // Executes the entire query tree, and aggregates the intermediate results into the // final result based on the binary operators and final reduction. // // Return Value: // The single result of aggregation. // protected override double InternalAggregate(ref Exception singularExceptionToThrow) { // Because the final reduction is typically much cheaper than the intermediate // reductions over the individual partitions, and because each parallel partition // will do a lot of work to produce a single output element, we prefer to turn off // pipelining, and process the final reductions serially. using (IEnumerator > enumerator = GetEnumerator(ParallelMergeOptions.FullyBuffered, true)) { // Throw an error for empty results. if (!enumerator.MoveNext()) { singularExceptionToThrow = new InvalidOperationException(SR.GetString(SR.NoElements)); return default(double); } Pair result = enumerator.Current; // Simply add together the sums and totals. while (enumerator.MoveNext()) { checked { result.First += enumerator.Current.First; result.Second += enumerator.Current.Second; } } // And divide the sum by the total to obtain the final result. return (double)result.First / result.Second; } } //--------------------------------------------------------------------------------------- // Creates an enumerator that is used internally for the final aggregation step. // protected override QueryOperatorEnumerator , int> CreateEnumerator ( int index, int count, QueryOperatorEnumerator source, object sharedData, CancellationToken cancellationToken) { return new LongAverageAggregationOperatorEnumerator (source, index, cancellationToken); } //--------------------------------------------------------------------------------------- // This enumerator type encapsulates the intermediary aggregation over the underlying // (possibly partitioned) data source. // private class LongAverageAggregationOperatorEnumerator : InlinedAggregationOperatorEnumerator > { private QueryOperatorEnumerator m_source; // The source data. //---------------------------------------------------------------------------------------- // Instantiates a new aggregation operator. // internal LongAverageAggregationOperatorEnumerator(QueryOperatorEnumerator source, int partitionIndex, CancellationToken cancellationToken) : base(partitionIndex, cancellationToken) { Contract.Assert(source != null); m_source = source; } //--------------------------------------------------------------------------------------- // Tallies up the average of the underlying data source, walking the entire thing the first // time MoveNext is called on this object. // protected override bool MoveNextCore(ref Pair currentElement) { // The temporary result contains the running sum and count, respectively. long sum = 0; long count = 0; QueryOperatorEnumerator source = m_source; long current = default(long); TKey keyUnused = default(TKey); if (source.MoveNext(ref current, ref keyUnused)) { int i = 0; do { if ((i++ & CancellationState.POLL_INTERVAL) == 0) CancellationState.ThrowIfCanceled(m_cancellationToken); checked { sum += current; count++; } } while (source.MoveNext(ref current, ref keyUnused)); currentElement = new Pair (sum, count); return true; } return false; } //---------------------------------------------------------------------------------------- // Dispose of resources associated with the underlying enumerator. // protected override void Dispose(bool disposing) { Contract.Assert(m_source != null); m_source.Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
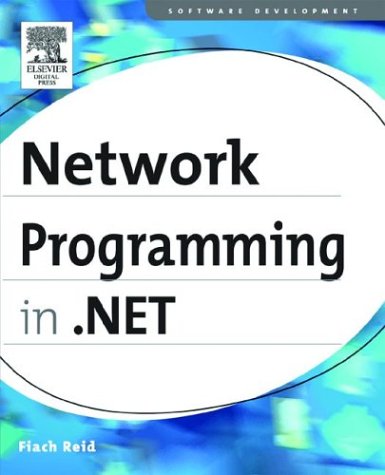
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ILGenerator.cs
- StreamGeometryContext.cs
- DataServiceClientException.cs
- CompilerInfo.cs
- XmlStringTable.cs
- Overlapped.cs
- TypeFieldSchema.cs
- DataBindingCollection.cs
- UserPreferenceChangedEventArgs.cs
- NonClientArea.cs
- OutputScopeManager.cs
- LoginView.cs
- TextContainerChangedEventArgs.cs
- XmlSerializerSection.cs
- Thumb.cs
- WebPartAuthorizationEventArgs.cs
- DataGridViewRowPrePaintEventArgs.cs
- XamlClipboardData.cs
- Int32CollectionValueSerializer.cs
- D3DImage.cs
- SafeReadContext.cs
- CatalogPart.cs
- RemoteWebConfigurationHostServer.cs
- TreeView.cs
- MessageFormatterConverter.cs
- TemplateXamlTreeBuilder.cs
- SqlDependencyListener.cs
- WebPartDisplayModeCancelEventArgs.cs
- Choices.cs
- StorageModelBuildProvider.cs
- StickyNoteContentControl.cs
- PreProcessor.cs
- _UriTypeConverter.cs
- WinEventWrap.cs
- DesignerRegion.cs
- FileLevelControlBuilderAttribute.cs
- XMLSyntaxException.cs
- SoapElementAttribute.cs
- BindUriHelper.cs
- BitVector32.cs
- OracleRowUpdatedEventArgs.cs
- ResXFileRef.cs
- ConsoleTraceListener.cs
- XmlSerializableServices.cs
- DateBoldEvent.cs
- URLIdentityPermission.cs
- EnumValidator.cs
- DbProviderFactories.cs
- MemberJoinTreeNode.cs
- MobileUserControl.cs
- WpfXamlType.cs
- AndCondition.cs
- ArgumentNullException.cs
- CriticalFinalizerObject.cs
- UnconditionalPolicy.cs
- CollectionView.cs
- TextParentUndoUnit.cs
- X500Name.cs
- QilGenerator.cs
- AxisAngleRotation3D.cs
- EncoderNLS.cs
- DocumentGrid.cs
- ActivityAction.cs
- DataGridPreparingCellForEditEventArgs.cs
- ChannelEndpointElementCollection.cs
- DmlSqlGenerator.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- PagesSection.cs
- RawUIStateInputReport.cs
- InvalidProgramException.cs
- TypeInitializationException.cs
- NonClientArea.cs
- Point3D.cs
- XmlDictionary.cs
- DecoderBestFitFallback.cs
- SeverityFilter.cs
- Int16KeyFrameCollection.cs
- ContentValidator.cs
- RadioButtonAutomationPeer.cs
- DataStorage.cs
- PublishLicense.cs
- TextHintingModeValidation.cs
- DropDownList.cs
- AsyncPostBackErrorEventArgs.cs
- XmlNamespaceManager.cs
- newinstructionaction.cs
- Msec.cs
- SafeProcessHandle.cs
- ZeroOpNode.cs
- smtppermission.cs
- RC2CryptoServiceProvider.cs
- SqlRewriteScalarSubqueries.cs
- TransactionScope.cs
- FormatVersion.cs
- TableColumnCollectionInternal.cs
- TableLayoutPanelResizeGlyph.cs
- PrivilegeNotHeldException.cs
- StreamWriter.cs
- PageParserFilter.cs
- NativeActivity.cs