Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / MS / Internal / Controls / StickyNote / StickyNoteContentControl.cs / 1 / StickyNoteContentControl.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Implementation of StickyNoteControl's internal TextBox/RichTextBox and InkCanvas helper classes. // // See spec at http://tabletpc/longhorn/Specs/StickyNoteControlSpec.mht // // History: // 03/03/2005 - waynezen - Created // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.IO; using System.Text; using System.Windows; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Ink; using System.Windows.Markup; using System.Xml; namespace MS.Internal.Controls.StickyNote { ////// An abstract class which defines the basic operation for StickyNote content /// internal abstract class StickyNoteContentControl { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors protected StickyNoteContentControl(FrameworkElement innerControl) { SetInnerControl(innerControl); } private StickyNoteContentControl() { } #endregion Constructors //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// Saves the content to an Xml node /// /// public abstract void Save(XmlNode node); ////// Load the content from an Xml node /// /// public abstract void Load(XmlNode node); ////// Clears the current content. /// public abstract void Clear(); #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //-------------------------------------------------------------------- #region Public Properties ////// Checks if the content is empty /// abstract public bool IsEmpty { get; } ////// Returns the content type /// abstract public StickyNoteType Type { get; } ////// Returns the inner control associated to this content. /// public FrameworkElement InnerControl { get { return _innerControl; } } #endregion Public Properties //------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods ////// Sets the internal control. The method also loads the custom style for the control if it's avaliable. /// /// The inner control protected void SetInnerControl(FrameworkElement innerControl) { _innerControl = innerControl; } #endregion Protected Methods //------------------------------------------------------------------- // // Protected Fields // //------------------------------------------------------------------- #region Protected Fields protected FrameworkElement _innerControl; // The maximum size of a byte buffer before its converted to a base64 string. protected const long MaxBufferSize = (Int32.MaxValue / 4) * 3; #endregion Protected Fields } ////// A factory class which creates SticktNote content controls /// internal static class StickyNoteContentControlFactory { //------------------------------------------------------------------- // // Private classes // //-------------------------------------------------------------------- #region Private classes ////// RichTextBox content implementation /// private class StickyNoteRichTextBox : StickyNoteContentControl { //------------------------------------------------------------------- // // Constructors // //-------------------------------------------------------------------- #region Constructors public StickyNoteRichTextBox(RichTextBox rtb) : base(rtb) { // Used to restrict enforce certain data format during pasting DataObject.AddPastingHandler(rtb, new DataObjectPastingEventHandler(OnPastingDataObject)); } #endregion Constructors //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// Clears the inner RichTextBox /// public override void Clear() { ((RichTextBox)InnerControl).Document = new FlowDocument(new Paragraph(new Run())); } ////// Save the RichTextBox data to an Xml node /// /// public override void Save(XmlNode node) { // make constant Debug.Assert(node != null && !IsEmpty); RichTextBox richTextBox = (RichTextBox)InnerControl; TextRange rtbRange = new TextRange(richTextBox.Document.ContentStart, richTextBox.Document.ContentEnd); if (!rtbRange.IsEmpty) { using (MemoryStream buffer = new MemoryStream()) { rtbRange.Save(buffer, DataFormats.Xaml); if (buffer.Length.CompareTo(MaxBufferSize) > 0) throw new InvalidOperationException(SR.Get(SRID.MaximumNoteSizeExceeded)); // Using GetBuffer avoids making a copy of the buffer which isn't necessary // Safe cast because the array's length can never be greater than Int.MaxValue node.InnerText = Convert.ToBase64String(buffer.GetBuffer(), 0, (int)buffer.Length); } } } ////// Load the RichTextBox data from an Xml node /// /// public override void Load(XmlNode node) { Debug.Assert(node != null); RichTextBox richTextBox = (RichTextBox)InnerControl; FlowDocument document = new FlowDocument(); TextRange rtbRange = new TextRange(document.ContentStart, document.ContentEnd); using (MemoryStream buffer = new MemoryStream(Convert.FromBase64String(node.InnerText))) { rtbRange.Load(buffer, DataFormats.Xaml); } richTextBox.Document = document; } #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// A flag whidh indicates if RichTextBox is empty /// public override bool IsEmpty { get { RichTextBox richTextBox = (RichTextBox)InnerControl; TextRange textRange = new TextRange(richTextBox.Document.ContentStart, richTextBox.Document.ContentEnd); return textRange.IsEmpty; } } ////// Returns Text Type /// public override StickyNoteType Type { get { return StickyNoteType.Text; } } #endregion Public Properties //------------------------------------------------------------------- // // Private Methods // //------------------------------------------------------------------- #region Private Methods ////// Serialization of Images isn't working so we restrict the pasting of images (and as a side effect /// all UIElements) into a text StickyNote until the serialization problem is corrected. /// private void OnPastingDataObject(Object sender, DataObjectPastingEventArgs e) { if (e.FormatToApply == DataFormats.Rtf) { UTF8Encoding encoding = new UTF8Encoding(); // Convert the RTF to Avalon content String rtfString = e.DataObject.GetData(DataFormats.Rtf) as String; MemoryStream data = new MemoryStream(encoding.GetBytes(rtfString)); FlowDocument document = new FlowDocument(); TextRange range = new TextRange(document.ContentStart, document.ContentEnd); range.Load(data, DataFormats.Rtf); // Serialize the content without UIElements and make it the preferred content for the paste MemoryStream buffer = new MemoryStream(); range.Save(buffer, DataFormats.Xaml); DataObject dataObject = new DataObject(); dataObject.SetData(DataFormats.Xaml, encoding.GetString(buffer.GetBuffer())); e.DataObject = dataObject; e.FormatToApply = DataFormats.Xaml; } else if (e.FormatToApply == DataFormats.Bitmap || e.FormatToApply == DataFormats.EnhancedMetafile || e.FormatToApply == DataFormats.MetafilePicture || e.FormatToApply == DataFormats.Tiff) { // Cancel all paste operations of stand-alone images e.CancelCommand(); } else if (e.FormatToApply == DataFormats.XamlPackage) { // Choose Xaml without UIElements over a XamlPackage e.FormatToApply = DataFormats.Xaml; } } #endregion Private Methods } ////// InkCanvas content implementation /// private class StickyNoteInkCanvas : StickyNoteContentControl { //-------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors public StickyNoteInkCanvas(InkCanvas canvas) : base(canvas) { } #endregion Constructors //-------------------------------------------------------------------- // // Public Methods // //-------------------------------------------------------------------- #region Public Methods ////// Clears the inner InkCanvas /// public override void Clear() { ( (InkCanvas)InnerControl ).Strokes.Clear(); } ////// Save the stroks data to an Xml node /// /// public override void Save(XmlNode node) { Debug.Assert(node != null && !IsEmpty); StrokeCollection strokes = ((InkCanvas)InnerControl).Strokes; using ( MemoryStream buffer = new MemoryStream() ) { strokes.Save(buffer); if (buffer.Length.CompareTo(MaxBufferSize) > 0) throw new InvalidOperationException(SR.Get(SRID.MaximumNoteSizeExceeded)); // Using GetBuffer avoids making a copy of the buffer which isn't necessary // Safe cast because the array's length can never be greater than Int.MaxValue node.InnerText = Convert.ToBase64String(buffer.GetBuffer(), 0, (int)buffer.Length); } } ////// Load the stroks data from an Xml node /// /// public override void Load(XmlNode node) { Debug.Assert(node != null, "Try to load data from an invalid node"); StrokeCollection strokes = null; if ( string.IsNullOrEmpty(node.InnerText) ) { // Create an empty StrokeCollection strokes = new StrokeCollection(); } else { using ( MemoryStream stream = new MemoryStream(Convert.FromBase64String(node.InnerText)) ) { strokes = new StrokeCollection(stream); } } ((InkCanvas)InnerControl).Strokes = strokes; } #endregion Public Methods //------------------------------------------------------------------- // // Public Properties // //-------------------------------------------------------------------- #region Public Properties ////// A flag which indicates whether the InkCanvas is empty /// public override bool IsEmpty { get { return ( (InkCanvas)InnerControl ).Strokes.Count == 0; } } ////// Returns the Ink type /// public override StickyNoteType Type { get { return StickyNoteType.Ink; } } #endregion Public Properties } #endregion Private classes //------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// A method which creates a specified type content control. /// /// /// ///public static StickyNoteContentControl CreateContentControl(StickyNoteType type, UIElement content) { StickyNoteContentControl contentControl = null; switch ( type ) { case StickyNoteType.Text: { RichTextBox rtb = content as RichTextBox; if (rtb == null) throw new InvalidOperationException(SR.Get(SRID.InvalidStickyNoteTemplate, type, typeof(RichTextBox), SNBConstants.c_ContentControlId)); contentControl = new StickyNoteRichTextBox(rtb); break; } case StickyNoteType.Ink: { InkCanvas canvas = content as InkCanvas; if (canvas == null) throw new InvalidOperationException(SR.Get(SRID.InvalidStickyNoteTemplate, type, typeof(InkCanvas), SNBConstants.c_ContentControlId)); contentControl = new StickyNoteInkCanvas(canvas); break; } } return contentControl; } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Implementation of StickyNoteControl's internal TextBox/RichTextBox and InkCanvas helper classes. // // See spec at http://tabletpc/longhorn/Specs/StickyNoteControlSpec.mht // // History: // 03/03/2005 - waynezen - Created // //--------------------------------------------------------------------------- using System; using System.Diagnostics; using System.IO; using System.Text; using System.Windows; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Ink; using System.Windows.Markup; using System.Xml; namespace MS.Internal.Controls.StickyNote { ////// An abstract class which defines the basic operation for StickyNote content /// internal abstract class StickyNoteContentControl { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors protected StickyNoteContentControl(FrameworkElement innerControl) { SetInnerControl(innerControl); } private StickyNoteContentControl() { } #endregion Constructors //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// Saves the content to an Xml node /// /// public abstract void Save(XmlNode node); ////// Load the content from an Xml node /// /// public abstract void Load(XmlNode node); ////// Clears the current content. /// public abstract void Clear(); #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //-------------------------------------------------------------------- #region Public Properties ////// Checks if the content is empty /// abstract public bool IsEmpty { get; } ////// Returns the content type /// abstract public StickyNoteType Type { get; } ////// Returns the inner control associated to this content. /// public FrameworkElement InnerControl { get { return _innerControl; } } #endregion Public Properties //------------------------------------------------------------------- // // Protected Methods // //-------------------------------------------------------------------- #region Protected Methods ////// Sets the internal control. The method also loads the custom style for the control if it's avaliable. /// /// The inner control protected void SetInnerControl(FrameworkElement innerControl) { _innerControl = innerControl; } #endregion Protected Methods //------------------------------------------------------------------- // // Protected Fields // //------------------------------------------------------------------- #region Protected Fields protected FrameworkElement _innerControl; // The maximum size of a byte buffer before its converted to a base64 string. protected const long MaxBufferSize = (Int32.MaxValue / 4) * 3; #endregion Protected Fields } ////// A factory class which creates SticktNote content controls /// internal static class StickyNoteContentControlFactory { //------------------------------------------------------------------- // // Private classes // //-------------------------------------------------------------------- #region Private classes ////// RichTextBox content implementation /// private class StickyNoteRichTextBox : StickyNoteContentControl { //------------------------------------------------------------------- // // Constructors // //-------------------------------------------------------------------- #region Constructors public StickyNoteRichTextBox(RichTextBox rtb) : base(rtb) { // Used to restrict enforce certain data format during pasting DataObject.AddPastingHandler(rtb, new DataObjectPastingEventHandler(OnPastingDataObject)); } #endregion Constructors //-------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// Clears the inner RichTextBox /// public override void Clear() { ((RichTextBox)InnerControl).Document = new FlowDocument(new Paragraph(new Run())); } ////// Save the RichTextBox data to an Xml node /// /// public override void Save(XmlNode node) { // make constant Debug.Assert(node != null && !IsEmpty); RichTextBox richTextBox = (RichTextBox)InnerControl; TextRange rtbRange = new TextRange(richTextBox.Document.ContentStart, richTextBox.Document.ContentEnd); if (!rtbRange.IsEmpty) { using (MemoryStream buffer = new MemoryStream()) { rtbRange.Save(buffer, DataFormats.Xaml); if (buffer.Length.CompareTo(MaxBufferSize) > 0) throw new InvalidOperationException(SR.Get(SRID.MaximumNoteSizeExceeded)); // Using GetBuffer avoids making a copy of the buffer which isn't necessary // Safe cast because the array's length can never be greater than Int.MaxValue node.InnerText = Convert.ToBase64String(buffer.GetBuffer(), 0, (int)buffer.Length); } } } ////// Load the RichTextBox data from an Xml node /// /// public override void Load(XmlNode node) { Debug.Assert(node != null); RichTextBox richTextBox = (RichTextBox)InnerControl; FlowDocument document = new FlowDocument(); TextRange rtbRange = new TextRange(document.ContentStart, document.ContentEnd); using (MemoryStream buffer = new MemoryStream(Convert.FromBase64String(node.InnerText))) { rtbRange.Load(buffer, DataFormats.Xaml); } richTextBox.Document = document; } #endregion Public Methods //-------------------------------------------------------------------- // // Public Properties // //------------------------------------------------------------------- #region Public Properties ////// A flag whidh indicates if RichTextBox is empty /// public override bool IsEmpty { get { RichTextBox richTextBox = (RichTextBox)InnerControl; TextRange textRange = new TextRange(richTextBox.Document.ContentStart, richTextBox.Document.ContentEnd); return textRange.IsEmpty; } } ////// Returns Text Type /// public override StickyNoteType Type { get { return StickyNoteType.Text; } } #endregion Public Properties //------------------------------------------------------------------- // // Private Methods // //------------------------------------------------------------------- #region Private Methods ////// Serialization of Images isn't working so we restrict the pasting of images (and as a side effect /// all UIElements) into a text StickyNote until the serialization problem is corrected. /// private void OnPastingDataObject(Object sender, DataObjectPastingEventArgs e) { if (e.FormatToApply == DataFormats.Rtf) { UTF8Encoding encoding = new UTF8Encoding(); // Convert the RTF to Avalon content String rtfString = e.DataObject.GetData(DataFormats.Rtf) as String; MemoryStream data = new MemoryStream(encoding.GetBytes(rtfString)); FlowDocument document = new FlowDocument(); TextRange range = new TextRange(document.ContentStart, document.ContentEnd); range.Load(data, DataFormats.Rtf); // Serialize the content without UIElements and make it the preferred content for the paste MemoryStream buffer = new MemoryStream(); range.Save(buffer, DataFormats.Xaml); DataObject dataObject = new DataObject(); dataObject.SetData(DataFormats.Xaml, encoding.GetString(buffer.GetBuffer())); e.DataObject = dataObject; e.FormatToApply = DataFormats.Xaml; } else if (e.FormatToApply == DataFormats.Bitmap || e.FormatToApply == DataFormats.EnhancedMetafile || e.FormatToApply == DataFormats.MetafilePicture || e.FormatToApply == DataFormats.Tiff) { // Cancel all paste operations of stand-alone images e.CancelCommand(); } else if (e.FormatToApply == DataFormats.XamlPackage) { // Choose Xaml without UIElements over a XamlPackage e.FormatToApply = DataFormats.Xaml; } } #endregion Private Methods } ////// InkCanvas content implementation /// private class StickyNoteInkCanvas : StickyNoteContentControl { //-------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors public StickyNoteInkCanvas(InkCanvas canvas) : base(canvas) { } #endregion Constructors //-------------------------------------------------------------------- // // Public Methods // //-------------------------------------------------------------------- #region Public Methods ////// Clears the inner InkCanvas /// public override void Clear() { ( (InkCanvas)InnerControl ).Strokes.Clear(); } ////// Save the stroks data to an Xml node /// /// public override void Save(XmlNode node) { Debug.Assert(node != null && !IsEmpty); StrokeCollection strokes = ((InkCanvas)InnerControl).Strokes; using ( MemoryStream buffer = new MemoryStream() ) { strokes.Save(buffer); if (buffer.Length.CompareTo(MaxBufferSize) > 0) throw new InvalidOperationException(SR.Get(SRID.MaximumNoteSizeExceeded)); // Using GetBuffer avoids making a copy of the buffer which isn't necessary // Safe cast because the array's length can never be greater than Int.MaxValue node.InnerText = Convert.ToBase64String(buffer.GetBuffer(), 0, (int)buffer.Length); } } ////// Load the stroks data from an Xml node /// /// public override void Load(XmlNode node) { Debug.Assert(node != null, "Try to load data from an invalid node"); StrokeCollection strokes = null; if ( string.IsNullOrEmpty(node.InnerText) ) { // Create an empty StrokeCollection strokes = new StrokeCollection(); } else { using ( MemoryStream stream = new MemoryStream(Convert.FromBase64String(node.InnerText)) ) { strokes = new StrokeCollection(stream); } } ((InkCanvas)InnerControl).Strokes = strokes; } #endregion Public Methods //------------------------------------------------------------------- // // Public Properties // //-------------------------------------------------------------------- #region Public Properties ////// A flag which indicates whether the InkCanvas is empty /// public override bool IsEmpty { get { return ( (InkCanvas)InnerControl ).Strokes.Count == 0; } } ////// Returns the Ink type /// public override StickyNoteType Type { get { return StickyNoteType.Ink; } } #endregion Public Properties } #endregion Private classes //------------------------------------------------------------------- // // Public Methods // //------------------------------------------------------------------- #region Public Methods ////// A method which creates a specified type content control. /// /// /// ///public static StickyNoteContentControl CreateContentControl(StickyNoteType type, UIElement content) { StickyNoteContentControl contentControl = null; switch ( type ) { case StickyNoteType.Text: { RichTextBox rtb = content as RichTextBox; if (rtb == null) throw new InvalidOperationException(SR.Get(SRID.InvalidStickyNoteTemplate, type, typeof(RichTextBox), SNBConstants.c_ContentControlId)); contentControl = new StickyNoteRichTextBox(rtb); break; } case StickyNoteType.Ink: { InkCanvas canvas = content as InkCanvas; if (canvas == null) throw new InvalidOperationException(SR.Get(SRID.InvalidStickyNoteTemplate, type, typeof(InkCanvas), SNBConstants.c_ContentControlId)); contentControl = new StickyNoteInkCanvas(canvas); break; } } return contentControl; } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
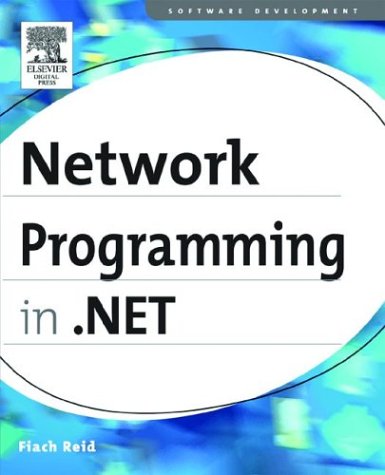
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextCompositionEventArgs.cs
- ObjectDataSourceEventArgs.cs
- IMembershipProvider.cs
- NamedObject.cs
- TableFieldsEditor.cs
- ImportCatalogPart.cs
- MimeTypeAttribute.cs
- WorkflowInstanceContextProvider.cs
- DrawingBrush.cs
- GenerateScriptTypeAttribute.cs
- OperandQuery.cs
- InternalSafeNativeMethods.cs
- Table.cs
- ArrayExtension.cs
- ColumnBinding.cs
- PointValueSerializer.cs
- DataGridTableCollection.cs
- XmlUTF8TextWriter.cs
- XmlnsCache.cs
- ResourceReader.cs
- CacheMemory.cs
- ComNativeDescriptor.cs
- WebPartActionVerb.cs
- RestHandler.cs
- MetadataCache.cs
- PaperSource.cs
- MSAAEventDispatcher.cs
- CodeConstructor.cs
- IriParsingElement.cs
- Point3DIndependentAnimationStorage.cs
- CornerRadius.cs
- ByteConverter.cs
- FilterUserControlBase.cs
- TextEffect.cs
- CompositionTarget.cs
- SessionState.cs
- FontDifferentiator.cs
- ParserOptions.cs
- OutputCacheProfile.cs
- ContextDataSourceView.cs
- TemplateBamlRecordReader.cs
- BatchParser.cs
- ProvidePropertyAttribute.cs
- ColumnTypeConverter.cs
- CultureInfoConverter.cs
- ControlsConfig.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- PointLight.cs
- CornerRadius.cs
- DBCommandBuilder.cs
- DataGridViewCellMouseEventArgs.cs
- GenericsInstances.cs
- EventHandlersStore.cs
- XmlJsonReader.cs
- BitmapScalingModeValidation.cs
- BitmapEffectOutputConnector.cs
- EntityPropertyMappingAttribute.cs
- SerializationEventsCache.cs
- _ShellExpression.cs
- WebResponse.cs
- DocumentGridContextMenu.cs
- ObjectManager.cs
- SourceChangedEventArgs.cs
- ScrollEvent.cs
- AgileSafeNativeMemoryHandle.cs
- SmiRequestExecutor.cs
- HistoryEventArgs.cs
- EngineSiteSapi.cs
- AssociatedControlConverter.cs
- CommandID.cs
- UnsafeNativeMethods.cs
- DPTypeDescriptorContext.cs
- GuidTagList.cs
- XmlLanguage.cs
- DelegateArgumentReference.cs
- XmlSchemaComplexContentExtension.cs
- InkCanvasAutomationPeer.cs
- MemberHolder.cs
- PopupControlService.cs
- IIS7WorkerRequest.cs
- HScrollProperties.cs
- ToolBarPanel.cs
- CmsInterop.cs
- Atom10FormatterFactory.cs
- UserCancellationException.cs
- GenericXmlSecurityTokenAuthenticator.cs
- Tool.cs
- FixedDocumentSequencePaginator.cs
- NameValueSectionHandler.cs
- HelpInfo.cs
- AggregateNode.cs
- CompensateDesigner.cs
- WorkflowServiceBuildProvider.cs
- safesecurityhelperavalon.cs
- SqlInfoMessageEvent.cs
- GatewayDefinition.cs
- DragCompletedEventArgs.cs
- ListViewDataItem.cs
- UiaCoreApi.cs
- XamlFilter.cs