Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / xsp / System / Web / Security / IMembershipProvider.cs / 2 / IMembershipProvider.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Security { using System.Web; using System.Security.Principal; using System.Collections.Specialized; using System.Web.Configuration; using System.Security.Permissions; using System.Globalization; using System.Security.Cryptography; using System.Runtime.Serialization; using System.Configuration.Provider; using System.Text; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class MembershipProvider : ProviderBase { private const int SALT_SIZE_IN_BYTES = 16; // // Property Section // // Public properties public abstract bool EnablePasswordRetrieval { get; } public abstract bool EnablePasswordReset { get; } public abstract bool RequiresQuestionAndAnswer { get; } public abstract string ApplicationName { get; set; } public abstract int MaxInvalidPasswordAttempts { get; } public abstract int PasswordAttemptWindow { get; } public abstract bool RequiresUniqueEmail { get; } public abstract MembershipPasswordFormat PasswordFormat { get; } public abstract int MinRequiredPasswordLength { get; } public abstract int MinRequiredNonAlphanumericCharacters { get; } public abstract string PasswordStrengthRegularExpression { get; } // // Method Section // public abstract MembershipUser CreateUser( string username, string password, string email, string passwordQuestion, string passwordAnswer, bool isApproved, object providerUserKey, out MembershipCreateStatus status ); public abstract bool ChangePasswordQuestionAndAnswer(string username, string password, string newPasswordQuestion, string newPasswordAnswer); public abstract string GetPassword(string username, string answer); public abstract bool ChangePassword(string username, string oldPassword, string newPassword); public abstract string ResetPassword(string username, string answer); public abstract void UpdateUser(MembershipUser user); public abstract bool ValidateUser(string username, string password); public abstract bool UnlockUser( string userName ); public abstract MembershipUser GetUser( object providerUserKey, bool userIsOnline ); public abstract MembershipUser GetUser(string username, bool userIsOnline); // GetUser() can throw 1 type of exception: // 1. ArgumentException is thrown if: // A. Username is null, is empty, contains commas, or is longer than 256 characters internal MembershipUser GetUser(string username, bool userIsOnline, bool throwOnError) { MembershipUser user = null; try { user = GetUser(username, userIsOnline); } catch (ArgumentException) { if (throwOnError) throw; } return user; } public abstract string GetUserNameByEmail(string email); public abstract bool DeleteUser(string username, bool deleteAllRelatedData); public abstract MembershipUserCollection GetAllUsers(int pageIndex, int pageSize, out int totalRecords); public abstract int GetNumberOfUsersOnline(); public abstract MembershipUserCollection FindUsersByName(string usernameToMatch, int pageIndex, int pageSize, out int totalRecords); public abstract MembershipUserCollection FindUsersByEmail(string emailToMatch, int pageIndex, int pageSize, out int totalRecords); protected virtual byte[] EncryptPassword( byte[] password ) { if (MachineKeySection.IsDecryptionKeyAutogenerated) throw new ProviderException(SR.GetString(SR.Can_not_use_encrypted_passwords_with_autogen_keys)); // DevDiv Bugs 137864: Use IVType.None for compatibility with stored passwords even after SP20 compat mode enabled. // This is the ONLY case IVType.None should be used. return MachineKeySection.EncryptOrDecryptData(true, password, null, 0, password.Length, IVType.None); } protected virtual byte[] DecryptPassword( byte[] encodedPassword ) { if (MachineKeySection.IsDecryptionKeyAutogenerated) throw new ProviderException(SR.GetString(SR.Can_not_use_encrypted_passwords_with_autogen_keys)); // DevDiv Bugs 137864: Use IVType.None for compatibility with stored passwords even after SP20 compat mode enabled. // This is the ONLY case IVType.None should be used. return MachineKeySection.EncryptOrDecryptData(false, encodedPassword, null, 0, encodedPassword.Length, IVType.None); } internal string EncodePassword(string pass, int passwordFormat, string salt) { if (passwordFormat == 0) // MembershipPasswordFormat.Clear return pass; byte[] bIn = Encoding.Unicode.GetBytes(pass); byte[] bSalt = Convert.FromBase64String(salt); byte[] bAll = new byte[bSalt.Length + bIn.Length]; byte[] bRet = null; Buffer.BlockCopy(bSalt, 0, bAll, 0, bSalt.Length); Buffer.BlockCopy(bIn, 0, bAll, bSalt.Length, bIn.Length); if (passwordFormat == 1) { // MembershipPasswordFormat.Hashed #if !FEATURE_PAL // FEATURE_PAL does not enable cryptography HashAlgorithm s = HashAlgorithm.Create( Membership.HashAlgorithmType ); // If the hash algorithm is null (and came from config), throw a config exception if (s == null) { if ( Membership.IsHashAlgorithmFromMembershipConfig ) { MembershipSection settings = RuntimeConfig.GetAppConfig().Membership; settings.ThrowHashAlgorithmException(); } } bRet = s.ComputeHash(bAll); #endif // !FEATURE_PAL } else { bRet = EncryptPassword( bAll ); } return Convert.ToBase64String(bRet); } internal string UnEncodePassword(string pass, int passwordFormat) { switch (passwordFormat) { case 0: // MembershipPasswordFormat.Clear: return pass; case 1: // MembershipPasswordFormat.Hashed: throw new ProviderException(SR.GetString(SR.Provider_can_not_decode_hashed_password)); default: byte[] bIn = Convert.FromBase64String(pass); byte[] bRet = DecryptPassword( bIn ); if (bRet == null) return null; return Encoding.Unicode.GetString(bRet, SALT_SIZE_IN_BYTES, bRet.Length - SALT_SIZE_IN_BYTES); } } internal string GenerateSalt() { byte[] buf = new byte[SALT_SIZE_IN_BYTES]; (new RNGCryptoServiceProvider()).GetBytes(buf); return Convert.ToBase64String(buf); } // // Event Section // public event MembershipValidatePasswordEventHandler ValidatingPassword { add { _EventHandler += value; } remove { _EventHandler -= value; } } protected virtual void OnValidatingPassword( ValidatePasswordEventArgs e ) { if( _EventHandler != null ) { _EventHandler( this, e ); } } private MembershipValidatePasswordEventHandler _EventHandler; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //[To be supplied.] ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Security { using System.Web; using System.Security.Principal; using System.Collections.Specialized; using System.Web.Configuration; using System.Security.Permissions; using System.Globalization; using System.Security.Cryptography; using System.Runtime.Serialization; using System.Configuration.Provider; using System.Text; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public abstract class MembershipProvider : ProviderBase { private const int SALT_SIZE_IN_BYTES = 16; // // Property Section // // Public properties public abstract bool EnablePasswordRetrieval { get; } public abstract bool EnablePasswordReset { get; } public abstract bool RequiresQuestionAndAnswer { get; } public abstract string ApplicationName { get; set; } public abstract int MaxInvalidPasswordAttempts { get; } public abstract int PasswordAttemptWindow { get; } public abstract bool RequiresUniqueEmail { get; } public abstract MembershipPasswordFormat PasswordFormat { get; } public abstract int MinRequiredPasswordLength { get; } public abstract int MinRequiredNonAlphanumericCharacters { get; } public abstract string PasswordStrengthRegularExpression { get; } // // Method Section // public abstract MembershipUser CreateUser( string username, string password, string email, string passwordQuestion, string passwordAnswer, bool isApproved, object providerUserKey, out MembershipCreateStatus status ); public abstract bool ChangePasswordQuestionAndAnswer(string username, string password, string newPasswordQuestion, string newPasswordAnswer); public abstract string GetPassword(string username, string answer); public abstract bool ChangePassword(string username, string oldPassword, string newPassword); public abstract string ResetPassword(string username, string answer); public abstract void UpdateUser(MembershipUser user); public abstract bool ValidateUser(string username, string password); public abstract bool UnlockUser( string userName ); public abstract MembershipUser GetUser( object providerUserKey, bool userIsOnline ); public abstract MembershipUser GetUser(string username, bool userIsOnline); // GetUser() can throw 1 type of exception: // 1. ArgumentException is thrown if: // A. Username is null, is empty, contains commas, or is longer than 256 characters internal MembershipUser GetUser(string username, bool userIsOnline, bool throwOnError) { MembershipUser user = null; try { user = GetUser(username, userIsOnline); } catch (ArgumentException) { if (throwOnError) throw; } return user; } public abstract string GetUserNameByEmail(string email); public abstract bool DeleteUser(string username, bool deleteAllRelatedData); public abstract MembershipUserCollection GetAllUsers(int pageIndex, int pageSize, out int totalRecords); public abstract int GetNumberOfUsersOnline(); public abstract MembershipUserCollection FindUsersByName(string usernameToMatch, int pageIndex, int pageSize, out int totalRecords); public abstract MembershipUserCollection FindUsersByEmail(string emailToMatch, int pageIndex, int pageSize, out int totalRecords); protected virtual byte[] EncryptPassword( byte[] password ) { if (MachineKeySection.IsDecryptionKeyAutogenerated) throw new ProviderException(SR.GetString(SR.Can_not_use_encrypted_passwords_with_autogen_keys)); // DevDiv Bugs 137864: Use IVType.None for compatibility with stored passwords even after SP20 compat mode enabled. // This is the ONLY case IVType.None should be used. return MachineKeySection.EncryptOrDecryptData(true, password, null, 0, password.Length, IVType.None); } protected virtual byte[] DecryptPassword( byte[] encodedPassword ) { if (MachineKeySection.IsDecryptionKeyAutogenerated) throw new ProviderException(SR.GetString(SR.Can_not_use_encrypted_passwords_with_autogen_keys)); // DevDiv Bugs 137864: Use IVType.None for compatibility with stored passwords even after SP20 compat mode enabled. // This is the ONLY case IVType.None should be used. return MachineKeySection.EncryptOrDecryptData(false, encodedPassword, null, 0, encodedPassword.Length, IVType.None); } internal string EncodePassword(string pass, int passwordFormat, string salt) { if (passwordFormat == 0) // MembershipPasswordFormat.Clear return pass; byte[] bIn = Encoding.Unicode.GetBytes(pass); byte[] bSalt = Convert.FromBase64String(salt); byte[] bAll = new byte[bSalt.Length + bIn.Length]; byte[] bRet = null; Buffer.BlockCopy(bSalt, 0, bAll, 0, bSalt.Length); Buffer.BlockCopy(bIn, 0, bAll, bSalt.Length, bIn.Length); if (passwordFormat == 1) { // MembershipPasswordFormat.Hashed #if !FEATURE_PAL // FEATURE_PAL does not enable cryptography HashAlgorithm s = HashAlgorithm.Create( Membership.HashAlgorithmType ); // If the hash algorithm is null (and came from config), throw a config exception if (s == null) { if ( Membership.IsHashAlgorithmFromMembershipConfig ) { MembershipSection settings = RuntimeConfig.GetAppConfig().Membership; settings.ThrowHashAlgorithmException(); } } bRet = s.ComputeHash(bAll); #endif // !FEATURE_PAL } else { bRet = EncryptPassword( bAll ); } return Convert.ToBase64String(bRet); } internal string UnEncodePassword(string pass, int passwordFormat) { switch (passwordFormat) { case 0: // MembershipPasswordFormat.Clear: return pass; case 1: // MembershipPasswordFormat.Hashed: throw new ProviderException(SR.GetString(SR.Provider_can_not_decode_hashed_password)); default: byte[] bIn = Convert.FromBase64String(pass); byte[] bRet = DecryptPassword( bIn ); if (bRet == null) return null; return Encoding.Unicode.GetString(bRet, SALT_SIZE_IN_BYTES, bRet.Length - SALT_SIZE_IN_BYTES); } } internal string GenerateSalt() { byte[] buf = new byte[SALT_SIZE_IN_BYTES]; (new RNGCryptoServiceProvider()).GetBytes(buf); return Convert.ToBase64String(buf); } // // Event Section // public event MembershipValidatePasswordEventHandler ValidatingPassword { add { _EventHandler += value; } remove { _EventHandler -= value; } } protected virtual void OnValidatingPassword( ValidatePasswordEventArgs e ) { if( _EventHandler != null ) { _EventHandler( this, e ); } } private MembershipValidatePasswordEventHandler _EventHandler; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.[To be supplied.] ///
Link Menu
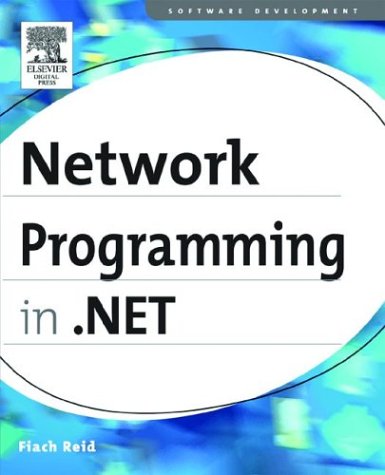
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Unit.cs
- GroupItemAutomationPeer.cs
- ProviderException.cs
- BamlRecordReader.cs
- Pen.cs
- Hash.cs
- TypeToken.cs
- AxParameterData.cs
- HtmlFormWrapper.cs
- IPCCacheManager.cs
- ListViewDeleteEventArgs.cs
- CalendarDay.cs
- SqlFileStream.cs
- SQLGuid.cs
- ObfuscateAssemblyAttribute.cs
- ListViewCommandEventArgs.cs
- ComponentDispatcherThread.cs
- TreeWalker.cs
- AlternateViewCollection.cs
- PriorityChain.cs
- ValidatedControlConverter.cs
- XhtmlBasicLabelAdapter.cs
- CuspData.cs
- CryptoHelper.cs
- SplayTreeNode.cs
- CopyNamespacesAction.cs
- TextAutomationPeer.cs
- ComponentGlyph.cs
- ObjectKeyFrameCollection.cs
- coordinator.cs
- RegexParser.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- InputScopeManager.cs
- _BasicClient.cs
- Events.cs
- StyleXamlTreeBuilder.cs
- ProgressBarHighlightConverter.cs
- SqlCharStream.cs
- ADMembershipUser.cs
- TransformationRules.cs
- InternalSafeNativeMethods.cs
- StickyNoteHelper.cs
- autovalidator.cs
- DiscoveryMessageSequence.cs
- UrlMappingsSection.cs
- CaseStatement.cs
- XmlValueConverter.cs
- TextServicesPropertyRanges.cs
- ExpandCollapseProviderWrapper.cs
- SecondaryIndex.cs
- LeaseManager.cs
- Action.cs
- BmpBitmapEncoder.cs
- OleDbCommandBuilder.cs
- XamlUtilities.cs
- ClientRoleProvider.cs
- PersonalizationEntry.cs
- LoggedException.cs
- COM2PropertyBuilderUITypeEditor.cs
- FormatterConverter.cs
- Assembly.cs
- CompilationSection.cs
- ListViewGroupItemCollection.cs
- ClientWindowsAuthenticationMembershipProvider.cs
- MetadataUtil.cs
- DefaultHttpHandler.cs
- NumericUpDownAcceleration.cs
- BitmapMetadata.cs
- AggregateNode.cs
- ProviderBase.cs
- SignatureDescription.cs
- NegotiateStream.cs
- ListSurrogate.cs
- Rijndael.cs
- WebSysDescriptionAttribute.cs
- XmlAnyAttributeAttribute.cs
- AliasExpr.cs
- XmlAnyElementAttributes.cs
- ExcludePathInfo.cs
- DataServiceEntityAttribute.cs
- StylusButton.cs
- Dump.cs
- ArrayConverter.cs
- AutomationPropertyInfo.cs
- EventProviderWriter.cs
- SuppressMergeCheckAttribute.cs
- HtmlTableCell.cs
- ProcessModelSection.cs
- BufferedStream.cs
- safemediahandle.cs
- CatalogPartChrome.cs
- DbConnectionInternal.cs
- WmlPanelAdapter.cs
- OleDbErrorCollection.cs
- X509SubjectKeyIdentifierClause.cs
- SrgsSemanticInterpretationTag.cs
- CodeDomLoader.cs
- DependentList.cs
- RegexCompilationInfo.cs
- TreeBuilder.cs