Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataEntity / System / Data / Common / CommandTrees / Internal / Util.cs / 1 / Util.cs
using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Globalization; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; namespace System.Data.Common.CommandTrees.Internal { internal static class CommandTreeUtils { internal static ListCreateList (T element) { List retList = new List (); retList.Add(element); return retList; } internal static List CreateList (T element1, T element2) { List retList = new List (); retList.Add(element1); retList.Add(element2); return retList; } internal static System.Collections.ObjectModel.ReadOnlyCollection NewReadOnlyList (IList sourceList) { TElementType[] newList = new TElementType[sourceList.Count]; sourceList.CopyTo(newList, 0); return new System.Collections.ObjectModel.ReadOnlyCollection (newList); } internal static string FormatIndex(string arrayVarName, int idx) { System.Text.StringBuilder builder = new System.Text.StringBuilder(arrayVarName.Length + 10 + 2); return builder.Append(arrayVarName).Append('[').Append(idx).Append(']').ToString(); //return string.Format(CultureInfo.InvariantCulture, "{0}[{1}]", arrayVarName, idx); } internal delegate void CheckNamedListCallback (KeyValuePair element, int index); internal static void CheckNamedList (string listVarName, IList > list, bool bAllowEmpty, CheckNamedListCallback callBack) { if (null == list) { throw EntityUtil.ArgumentNull(listVarName); } if (0 == list.Count && !bAllowEmpty) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Util_CheckListEmptyInvalid, listVarName); } List names = new List (); for (int idx = 0; idx < list.Count; idx++) { KeyValuePair element = list[idx]; string elementName = CommandTreeUtils.FormatIndex(listVarName, idx); // // Check Key for null/empty, Value for null // CommandTreeUtils.CheckNamed (elementName, element); // // Duplicate names are not allowed // int iNameIdx = names.IndexOf(element.Key); if (iNameIdx > -1) { throw EntityUtil.Argument ( System.Data.Entity.Strings.Cqt_Util_CheckListDuplicateName(iNameIdx, idx, element.Key), elementName ); } // // This element is valid, so invoke the callback // if (callBack != null) { callBack(element, idx); } } } internal static void CheckNamed (string strVarName, KeyValuePair element) { if (string.IsNullOrEmpty(element.Key)) { throw EntityUtil.ArgumentNull(string.Format(CultureInfo.InvariantCulture, "{0}.Key", strVarName)); } if (null == element.Value) { throw EntityUtil.ArgumentNull(string.Format(CultureInfo.InvariantCulture, "{0}.Value", strVarName)); } } internal static bool IsValidDataSpace(DataSpace dataSpace) { return (DataSpace.OSpace == dataSpace || DataSpace.CSpace == dataSpace || DataSpace.SSpace == dataSpace); } internal static bool TryGetPrimtiveTypeKind(Type clrType, out PrimitiveTypeKind primitiveTypeKind) { primitiveTypeKind = default(PrimitiveTypeKind); PrimitiveType primitiveType = null; if (ClrProviderManifest.Instance.TryGetPrimitiveType(clrType, out primitiveType)) { primitiveTypeKind = primitiveType.PrimitiveTypeKind; return true; } return false; } #region Expression Flattening Helpers static private readonly HashSet _associativeExpressionKinds = new HashSet (new DbExpressionKind[] { DbExpressionKind.Or, DbExpressionKind.And, DbExpressionKind.Plus, DbExpressionKind.Multiply}); /// /// Creates a flat list of the associative arguments. /// For example, for ((A1 + (A2 - A3)) + A4) it will create A1, (A2 - A3), A4 /// /// ///internal static IEnumerable FlattenAssociativeExpression(DbExpression expression) { return FlattenAssociativeExpression(expression.ExpressionKind, expression); } /// /// Creates a flat list of the associative arguments. /// For example, for ((A1 + (A2 - A3)) + A4) it will create A1, (A2 - A3), A4 /// Only 'unfolds' the given arguments that are of the given expression kind. /// /// /// ///internal static IEnumerable FlattenAssociativeExpression(DbExpressionKind expressionKind, params DbExpression[] arguments) { if (!_associativeExpressionKinds.Contains(expressionKind)) { return arguments; } List outputArguments = new List (); foreach (DbExpression argument in arguments) { ExtractAssociativeArguments(expressionKind, outputArguments, argument); } return outputArguments; } /// /// Helper method for FlattenAssociativeExpression. /// Creates a flat list of the associative arguments and appends to the given argument list. /// For example, for ((A1 + (A2 - A3)) + A4) it will add A1, (A2 - A3), A4 to the list. /// Only 'unfolds' the given expression if it is of the given expression kind. /// /// /// /// private static void ExtractAssociativeArguments(DbExpressionKind expressionKind, ListargumentList, DbExpression expression) { if (expression.ExpressionKind != expressionKind) { argumentList.Add(expression); return; } //All associative expressions are binary, thus we must be dealing with a DbBinaryExpresson or // a DbArithmeticExpression with 2 arguments. DbBinaryExpression binaryExpression = expression as DbBinaryExpression; if (binaryExpression != null) { ExtractAssociativeArguments(expressionKind, argumentList, binaryExpression.Left); ExtractAssociativeArguments(expressionKind, argumentList, binaryExpression.Right); return; } DbArithmeticExpression arithExpression = (DbArithmeticExpression)expression; ExtractAssociativeArguments(expressionKind, argumentList, arithExpression.Arguments[0]); ExtractAssociativeArguments(expressionKind, argumentList, arithExpression.Arguments[1]); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Globalization; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees; namespace System.Data.Common.CommandTrees.Internal { internal static class CommandTreeUtils { internal static List CreateList (T element) { List retList = new List (); retList.Add(element); return retList; } internal static List CreateList (T element1, T element2) { List retList = new List (); retList.Add(element1); retList.Add(element2); return retList; } internal static System.Collections.ObjectModel.ReadOnlyCollection NewReadOnlyList (IList sourceList) { TElementType[] newList = new TElementType[sourceList.Count]; sourceList.CopyTo(newList, 0); return new System.Collections.ObjectModel.ReadOnlyCollection (newList); } internal static string FormatIndex(string arrayVarName, int idx) { System.Text.StringBuilder builder = new System.Text.StringBuilder(arrayVarName.Length + 10 + 2); return builder.Append(arrayVarName).Append('[').Append(idx).Append(']').ToString(); //return string.Format(CultureInfo.InvariantCulture, "{0}[{1}]", arrayVarName, idx); } internal delegate void CheckNamedListCallback (KeyValuePair element, int index); internal static void CheckNamedList (string listVarName, IList > list, bool bAllowEmpty, CheckNamedListCallback callBack) { if (null == list) { throw EntityUtil.ArgumentNull(listVarName); } if (0 == list.Count && !bAllowEmpty) { throw EntityUtil.Argument(System.Data.Entity.Strings.Cqt_Util_CheckListEmptyInvalid, listVarName); } List names = new List (); for (int idx = 0; idx < list.Count; idx++) { KeyValuePair element = list[idx]; string elementName = CommandTreeUtils.FormatIndex(listVarName, idx); // // Check Key for null/empty, Value for null // CommandTreeUtils.CheckNamed (elementName, element); // // Duplicate names are not allowed // int iNameIdx = names.IndexOf(element.Key); if (iNameIdx > -1) { throw EntityUtil.Argument ( System.Data.Entity.Strings.Cqt_Util_CheckListDuplicateName(iNameIdx, idx, element.Key), elementName ); } // // This element is valid, so invoke the callback // if (callBack != null) { callBack(element, idx); } } } internal static void CheckNamed (string strVarName, KeyValuePair element) { if (string.IsNullOrEmpty(element.Key)) { throw EntityUtil.ArgumentNull(string.Format(CultureInfo.InvariantCulture, "{0}.Key", strVarName)); } if (null == element.Value) { throw EntityUtil.ArgumentNull(string.Format(CultureInfo.InvariantCulture, "{0}.Value", strVarName)); } } internal static bool IsValidDataSpace(DataSpace dataSpace) { return (DataSpace.OSpace == dataSpace || DataSpace.CSpace == dataSpace || DataSpace.SSpace == dataSpace); } internal static bool TryGetPrimtiveTypeKind(Type clrType, out PrimitiveTypeKind primitiveTypeKind) { primitiveTypeKind = default(PrimitiveTypeKind); PrimitiveType primitiveType = null; if (ClrProviderManifest.Instance.TryGetPrimitiveType(clrType, out primitiveType)) { primitiveTypeKind = primitiveType.PrimitiveTypeKind; return true; } return false; } #region Expression Flattening Helpers static private readonly HashSet _associativeExpressionKinds = new HashSet (new DbExpressionKind[] { DbExpressionKind.Or, DbExpressionKind.And, DbExpressionKind.Plus, DbExpressionKind.Multiply}); /// /// Creates a flat list of the associative arguments. /// For example, for ((A1 + (A2 - A3)) + A4) it will create A1, (A2 - A3), A4 /// /// ///internal static IEnumerable FlattenAssociativeExpression(DbExpression expression) { return FlattenAssociativeExpression(expression.ExpressionKind, expression); } /// /// Creates a flat list of the associative arguments. /// For example, for ((A1 + (A2 - A3)) + A4) it will create A1, (A2 - A3), A4 /// Only 'unfolds' the given arguments that are of the given expression kind. /// /// /// ///internal static IEnumerable FlattenAssociativeExpression(DbExpressionKind expressionKind, params DbExpression[] arguments) { if (!_associativeExpressionKinds.Contains(expressionKind)) { return arguments; } List outputArguments = new List (); foreach (DbExpression argument in arguments) { ExtractAssociativeArguments(expressionKind, outputArguments, argument); } return outputArguments; } /// /// Helper method for FlattenAssociativeExpression. /// Creates a flat list of the associative arguments and appends to the given argument list. /// For example, for ((A1 + (A2 - A3)) + A4) it will add A1, (A2 - A3), A4 to the list. /// Only 'unfolds' the given expression if it is of the given expression kind. /// /// /// /// private static void ExtractAssociativeArguments(DbExpressionKind expressionKind, ListargumentList, DbExpression expression) { if (expression.ExpressionKind != expressionKind) { argumentList.Add(expression); return; } //All associative expressions are binary, thus we must be dealing with a DbBinaryExpresson or // a DbArithmeticExpression with 2 arguments. DbBinaryExpression binaryExpression = expression as DbBinaryExpression; if (binaryExpression != null) { ExtractAssociativeArguments(expressionKind, argumentList, binaryExpression.Left); ExtractAssociativeArguments(expressionKind, argumentList, binaryExpression.Right); return; } DbArithmeticExpression arithExpression = (DbArithmeticExpression)expression; ExtractAssociativeArguments(expressionKind, argumentList, arithExpression.Arguments[0]); ExtractAssociativeArguments(expressionKind, argumentList, arithExpression.Arguments[1]); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
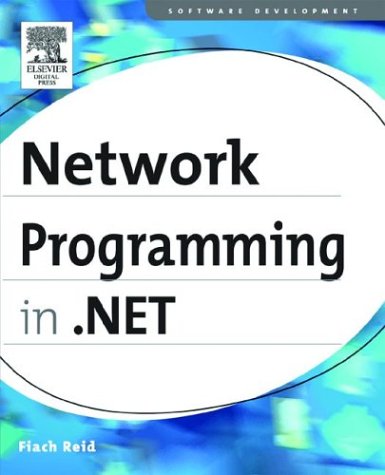
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlNavigatorFilter.cs
- StateMachineExecutionState.cs
- SamlConditions.cs
- PtsPage.cs
- SequenceQuery.cs
- _HTTPDateParse.cs
- Point3DCollection.cs
- RightsManagementEncryptedStream.cs
- CodeArrayIndexerExpression.cs
- MemoryPressure.cs
- TypeSource.cs
- IItemProperties.cs
- FlowDocumentPaginator.cs
- TokenBasedSet.cs
- URL.cs
- SqlStatistics.cs
- AdapterUtil.cs
- DataGridViewCellValidatingEventArgs.cs
- HttpApplicationStateBase.cs
- OpCellTreeNode.cs
- BitStack.cs
- ListViewItem.cs
- MetricEntry.cs
- GlyphRunDrawing.cs
- SettingsPropertyNotFoundException.cs
- ConditionCollection.cs
- GuidelineSet.cs
- HtmlContainerControl.cs
- XmlToDatasetMap.cs
- ListControlDesigner.cs
- EventTrigger.cs
- ClientScriptItemCollection.cs
- SoapSchemaImporter.cs
- UriScheme.cs
- Point4DValueSerializer.cs
- AppearanceEditorPart.cs
- _LoggingObject.cs
- SerialReceived.cs
- XMLDiffLoader.cs
- BufferedGraphicsManager.cs
- VirtualDirectoryMappingCollection.cs
- AVElementHelper.cs
- WorkerRequest.cs
- TextWriterTraceListener.cs
- Main.cs
- TextElementAutomationPeer.cs
- BuildProviderAppliesToAttribute.cs
- RoutedCommand.cs
- BindingCollectionElement.cs
- Parser.cs
- ContentPosition.cs
- PageThemeParser.cs
- SettingsPropertyWrongTypeException.cs
- WebPartDescriptionCollection.cs
- ProxyHwnd.cs
- X509Extension.cs
- KeyInterop.cs
- TransformPatternIdentifiers.cs
- TypeAccessException.cs
- ConfigPathUtility.cs
- DivideByZeroException.cs
- CodeIdentifiers.cs
- TemplatedWizardStep.cs
- OrderingInfo.cs
- DebugTrace.cs
- Vertex.cs
- ConfigurationValidatorBase.cs
- Thread.cs
- DefaultMemberAttribute.cs
- MonthChangedEventArgs.cs
- Listbox.cs
- FigureHelper.cs
- Type.cs
- WorkerRequest.cs
- WindowsPen.cs
- ScriptServiceAttribute.cs
- MenuCommands.cs
- CodeDelegateCreateExpression.cs
- GridViewSortEventArgs.cs
- _DigestClient.cs
- XamlSerializer.cs
- InfoCardClaimCollection.cs
- URLAttribute.cs
- XmlElementCollection.cs
- Utils.cs
- GrammarBuilderRuleRef.cs
- StringKeyFrameCollection.cs
- XPathNavigatorReader.cs
- DataBoundControlAdapter.cs
- IdentifierCollection.cs
- DataServiceRequestException.cs
- TypeReference.cs
- XmlSchemaValidator.cs
- WaitHandleCannotBeOpenedException.cs
- JournalEntryStack.cs
- Thumb.cs
- FileAuthorizationModule.cs
- CommonRemoteMemoryBlock.cs
- RadioButtonAutomationPeer.cs
- Pkcs7Signer.cs