Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / InterOp / Imaging.cs / 1 / Imaging.cs
//------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, All Rights Reserved. // // File: Imaging.cs // //----------------------------------------------------------------------------- using System; using System.Security; using System.Security.Permissions; using MS.Internal; using System.Diagnostics; using System.Windows; using System.Windows.Media.Imaging; using Microsoft.Win32.SafeHandles; using MS.Internal.PresentationCore; // SecurityHelper using System.Windows.Interop; namespace System.Windows.Interop { ////// Managed/Unmanaged Interop for Imaging. /// public static class Imaging { ////// Construct an Bitmap from a HBITMAP. /// /// /// /// /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// Critical - calls critical code, access unmanaged resources /// PublicOK - demands unmanaged code permission /// [SecurityCritical] unsafe public static BitmapSource CreateBitmapSourceFromHBitmap( IntPtr bitmap, IntPtr palette, Int32Rect sourceRect, BitmapSizeOptions sizeOptions) { SecurityHelper.DemandUnmanagedCode(); // CR: dwaynen (1681459) return CriticalCreateBitmapSourceFromHBitmap(bitmap, palette, sourceRect, sizeOptions, WICBitmapAlphaChannelOption.WICBitmapUseAlpha); } ////// Construct an Bitmap from a HBITMAP. /// /// /// /// /// /// ////// Critical - calls critical code, access unmanaged resources /// [SecurityCritical] unsafe internal static BitmapSource CriticalCreateBitmapSourceFromHBitmap( IntPtr bitmap, IntPtr palette, Int32Rect sourceRect, BitmapSizeOptions sizeOptions, WICBitmapAlphaChannelOption alphaOptions) { if (bitmap == IntPtr.Zero) { throw new ArgumentNullException("bitmap"); } return new InteropBitmap(bitmap, palette, sourceRect, sizeOptions, alphaOptions); // use the critical version } ////// Construct an Bitmap from a HICON. /// /// /// /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// Critical - calls critical code, access unmanaged resources /// PublicOK - demands unmanaged code permission /// [SecurityCritical ] unsafe public static BitmapSource CreateBitmapSourceFromHIcon( IntPtr icon, Int32Rect sourceRect, BitmapSizeOptions sizeOptions) { SecurityHelper.DemandUnmanagedCode(); if (icon == IntPtr.Zero) { throw new ArgumentNullException("icon"); } return new InteropBitmap(icon, sourceRect, sizeOptions); } ////// Construct an Bitmap from a section handle. /// /// /// /// /// /// /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// Critical - calls critical code, access unmanaged resources /// PublicOK - demands unmanaged code permission /// [SecurityCritical ] unsafe public static BitmapSource CreateBitmapSourceFromMemorySection( IntPtr section, int pixelWidth, int pixelHeight, Media.PixelFormat format, int stride, int offset) { SecurityHelper.DemandUnmanagedCode(); if (section == IntPtr.Zero) { throw new ArgumentNullException("section"); } return new InteropBitmap(section, pixelWidth, pixelHeight, format, stride, offset); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // Microsoft Avalon // Copyright (c) Microsoft Corporation, All Rights Reserved. // // File: Imaging.cs // //----------------------------------------------------------------------------- using System; using System.Security; using System.Security.Permissions; using MS.Internal; using System.Diagnostics; using System.Windows; using System.Windows.Media.Imaging; using Microsoft.Win32.SafeHandles; using MS.Internal.PresentationCore; // SecurityHelper using System.Windows.Interop; namespace System.Windows.Interop { ////// Managed/Unmanaged Interop for Imaging. /// public static class Imaging { ////// Construct an Bitmap from a HBITMAP. /// /// /// /// /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// Critical - calls critical code, access unmanaged resources /// PublicOK - demands unmanaged code permission /// [SecurityCritical] unsafe public static BitmapSource CreateBitmapSourceFromHBitmap( IntPtr bitmap, IntPtr palette, Int32Rect sourceRect, BitmapSizeOptions sizeOptions) { SecurityHelper.DemandUnmanagedCode(); // CR: dwaynen (1681459) return CriticalCreateBitmapSourceFromHBitmap(bitmap, palette, sourceRect, sizeOptions, WICBitmapAlphaChannelOption.WICBitmapUseAlpha); } ////// Construct an Bitmap from a HBITMAP. /// /// /// /// /// /// ////// Critical - calls critical code, access unmanaged resources /// [SecurityCritical] unsafe internal static BitmapSource CriticalCreateBitmapSourceFromHBitmap( IntPtr bitmap, IntPtr palette, Int32Rect sourceRect, BitmapSizeOptions sizeOptions, WICBitmapAlphaChannelOption alphaOptions) { if (bitmap == IntPtr.Zero) { throw new ArgumentNullException("bitmap"); } return new InteropBitmap(bitmap, palette, sourceRect, sizeOptions, alphaOptions); // use the critical version } ////// Construct an Bitmap from a HICON. /// /// /// /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// Critical - calls critical code, access unmanaged resources /// PublicOK - demands unmanaged code permission /// [SecurityCritical ] unsafe public static BitmapSource CreateBitmapSourceFromHIcon( IntPtr icon, Int32Rect sourceRect, BitmapSizeOptions sizeOptions) { SecurityHelper.DemandUnmanagedCode(); if (icon == IntPtr.Zero) { throw new ArgumentNullException("icon"); } return new InteropBitmap(icon, sourceRect, sizeOptions); } ////// Construct an Bitmap from a section handle. /// /// /// /// /// /// /// ////// Callers must have UnmanagedCode permission to call this API. /// ////// Critical - calls critical code, access unmanaged resources /// PublicOK - demands unmanaged code permission /// [SecurityCritical ] unsafe public static BitmapSource CreateBitmapSourceFromMemorySection( IntPtr section, int pixelWidth, int pixelHeight, Media.PixelFormat format, int stride, int offset) { SecurityHelper.DemandUnmanagedCode(); if (section == IntPtr.Zero) { throw new ArgumentNullException("section"); } return new InteropBitmap(section, pixelWidth, pixelHeight, format, stride, offset); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
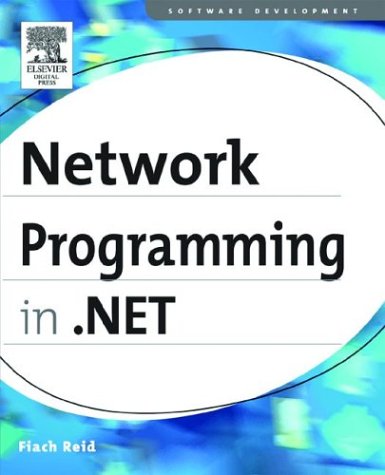
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AssertFilter.cs
- EntityViewGenerationAttribute.cs
- ELinqQueryState.cs
- DLinqTableProvider.cs
- TemplatedControlDesigner.cs
- UnsafeNetInfoNativeMethods.cs
- ObjectManager.cs
- KerberosRequestorSecurityToken.cs
- TlsnegoTokenAuthenticator.cs
- PropVariant.cs
- CryptoApi.cs
- PartitionResolver.cs
- ZipFileInfoCollection.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- ResXResourceSet.cs
- ToolStripScrollButton.cs
- securitycriticaldataClass.cs
- PauseStoryboard.cs
- DiscreteKeyFrames.cs
- EntitySetDataBindingList.cs
- TransactionContext.cs
- ConnectionStringSettingsCollection.cs
- ProgressChangedEventArgs.cs
- ResourceReader.cs
- UnsafePeerToPeerMethods.cs
- CustomError.cs
- SpeechSynthesizer.cs
- SecurityDocument.cs
- MenuCommands.cs
- Html32TextWriter.cs
- InputLanguageEventArgs.cs
- HttpResponseHeader.cs
- RotateTransform.cs
- CookieProtection.cs
- NGCSerializerAsync.cs
- MsmqIntegrationOutputChannel.cs
- OwnerDrawPropertyBag.cs
- RoutingService.cs
- SqlParameter.cs
- HostVisual.cs
- DetailsViewPageEventArgs.cs
- TextTrailingWordEllipsis.cs
- DesignerTransaction.cs
- StoryFragments.cs
- ISAPIWorkerRequest.cs
- SizeAnimationUsingKeyFrames.cs
- TriggerActionCollection.cs
- _HeaderInfo.cs
- TextTrailingWordEllipsis.cs
- XmlSchemas.cs
- DispatcherEventArgs.cs
- BamlLocalizer.cs
- CrossSiteScriptingValidation.cs
- Nullable.cs
- RelationshipEndCollection.cs
- ToolStripItemRenderEventArgs.cs
- ReferencedCollectionType.cs
- GraphicsPathIterator.cs
- NetPeerTcpBindingElement.cs
- ResXResourceReader.cs
- HttpListenerRequest.cs
- TextEndOfLine.cs
- LinkLabelLinkClickedEvent.cs
- WaitingCursor.cs
- InheritanceAttribute.cs
- _DisconnectOverlappedAsyncResult.cs
- ListViewSelectEventArgs.cs
- Int32AnimationUsingKeyFrames.cs
- Site.cs
- EdmPropertyAttribute.cs
- SmtpNegotiateAuthenticationModule.cs
- SyndicationDeserializer.cs
- SQLUtility.cs
- SerializationInfoEnumerator.cs
- FileLevelControlBuilderAttribute.cs
- DragDeltaEventArgs.cs
- StringFormat.cs
- ToolboxItem.cs
- BufferedGraphicsManager.cs
- WeakReference.cs
- ParentQuery.cs
- Matrix3D.cs
- NamespaceEmitter.cs
- XmlSchemaImporter.cs
- CriticalExceptions.cs
- EdmComplexTypeAttribute.cs
- ClientScriptManagerWrapper.cs
- ConfigXmlDocument.cs
- ParagraphVisual.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- RunInstallerAttribute.cs
- XXXInfos.cs
- BamlLocalizer.cs
- XsltArgumentList.cs
- PrinterResolution.cs
- Timeline.cs
- TransformerInfo.cs
- ModelPropertyDescriptor.cs
- WebMethodAttribute.cs
- CellParagraph.cs