Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / CommonUI / System / Drawing / Advanced / StringFormat.cs / 1 / StringFormat.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Diagnostics; using System; using Microsoft.Win32; using System.Drawing; using System.ComponentModel; using System.Drawing.Text; using System.Drawing.Internal; using System.Runtime.InteropServices; using System.Globalization; ///[StructLayout(LayoutKind.Sequential)] public struct CharacterRange { private int first; private int length; /** * Create a new CharacterRange object */ /// /// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] public CharacterRange(int First, int Length) { this.first = First; this.length = Length; } ////// Initializes a new instance of the ///class /// with the specified coordinates. /// /// /// Gets the First character position of this public int First { get { return first; } set { first = value; } } ///. /// /// /// public int Length { get { return length; } set { length = value; } } public override bool Equals(object obj) { if (obj.GetType() != typeof(CharacterRange)) return false; CharacterRange cr = (CharacterRange)obj; return ((this.first == cr.First) && (this.length == cr.Length)); } ////// Gets the Length of this ///. /// public static bool operator ==(CharacterRange cr1, CharacterRange cr2) { return ((cr1.First == cr2.First) && (cr1.Length == cr2.Length)); } /// public static bool operator !=(CharacterRange cr1, CharacterRange cr2) { return !(cr1 == cr2); } /// public override int GetHashCode() { return this.first << 8 + this.length; } } /** * Represent a Stringformat object */ /// /// /// Encapsulates text layout information (such /// as alignment and linespacing), display manipulations (such as ellipsis insertion /// and national digit substitution) and OpenType features. /// public sealed class StringFormat : MarshalByRefObject, ICloneable, IDisposable { internal IntPtr nativeFormat; private StringFormat(IntPtr format) { nativeFormat = format; } ////// /// Initializes a new instance of the public StringFormat() : this(0, 0) { } ////// class. /// /// /// Initializes a new instance of the public StringFormat(StringFormatFlags options) : this(options, 0) { } ////// class with the specified . /// /// /// Initializes a new instance of the public StringFormat(StringFormatFlags options, int language) { int status = SafeNativeMethods.Gdip.GdipCreateStringFormat(options, language, out nativeFormat); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// class with the specified and language. /// /// /// public StringFormat(StringFormat format) { if (format == null) { throw new ArgumentNullException("format"); } int status = SafeNativeMethods.Gdip.GdipCloneStringFormat(new HandleRef(format, format.nativeFormat), out nativeFormat); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// Initializes a new instance of the ///class from the specified /// existing . /// /// /// Cleans up Windows resources for this /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ///. /// void Dispose(bool disposing) { if (nativeFormat != IntPtr.Zero) { try{ #if DEBUG int status = #endif SafeNativeMethods.Gdip.GdipDeleteStringFormat(new HandleRef(this, nativeFormat)); #if DEBUG Debug.Assert(status == SafeNativeMethods.Gdip.Ok, "GDI+ returned an error status: " + status.ToString(CultureInfo.InvariantCulture)); #endif } catch( Exception ex ){ if( ClientUtils.IsCriticalException( ex ) ) { throw; } Debug.Fail( "Exception thrown during Dispose: " + ex.ToString() ); } finally{ nativeFormat = IntPtr.Zero; } } } /// /// /// Creates an exact copy of this public object Clone() { IntPtr cloneFormat = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCloneStringFormat(new HandleRef(this, nativeFormat), out cloneFormat); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); StringFormat newCloneStringFormat = new StringFormat(cloneFormat); return newCloneStringFormat; } ///. /// /// /// Gets or sets a public StringFormatFlags FormatFlags { get { StringFormatFlags format; int status = SafeNativeMethods.Gdip.GdipGetStringFormatFlags(new HandleRef(this, nativeFormat), out format); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return format; } set { Debug.Assert(nativeFormat != IntPtr.Zero, "NativeFormat is null!"); int status = SafeNativeMethods.Gdip.GdipSetStringFormatFlags(new HandleRef(this, nativeFormat), value); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } } ///that contains formatting information. /// /// /// public void SetMeasurableCharacterRanges(CharacterRange[] ranges) { int status = SafeNativeMethods.Gdip.GdipSetStringFormatMeasurableCharacterRanges(new HandleRef(this, nativeFormat), ranges.Length, ranges); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } // For English, this is horizontal alignment ////// Sets the measure of characters to the specified /// range. /// ////// /// Specifies text alignment information. /// public StringAlignment Alignment { get { StringAlignment alignment = 0; Debug.Assert(nativeFormat != IntPtr.Zero, "NativeFormat is null!"); int status = SafeNativeMethods.Gdip.GdipGetStringFormatAlign(new HandleRef(this, nativeFormat), out alignment); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return alignment; } set { //valid values are 0x0 to 0x2 if (!ClientUtils.IsEnumValid(value, (int)value, (int)StringAlignment.Near, (int)StringAlignment.Far)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(StringAlignment)); } Debug.Assert(nativeFormat != IntPtr.Zero, "NativeFormat is null!"); int status = SafeNativeMethods.Gdip.GdipSetStringFormatAlign(new HandleRef(this, nativeFormat), value); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } } // For English, this is vertical alignment ////// /// Gets or sets the line alignment. /// public StringAlignment LineAlignment { get { StringAlignment alignment = 0; Debug.Assert(nativeFormat != IntPtr.Zero, "NativeFormat is null!"); int status = SafeNativeMethods.Gdip.GdipGetStringFormatLineAlign(new HandleRef(this, nativeFormat), out alignment); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return alignment; } set { if (value<0 || value>StringAlignment.Far) { throw new InvalidEnumArgumentException("value", (int)value, typeof(StringAlignment)); } Debug.Assert(nativeFormat != IntPtr.Zero, "NativeFormat is null!"); int status = SafeNativeMethods.Gdip.GdipSetStringFormatLineAlign(new HandleRef(this, nativeFormat), value); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } } ////// /// public HotkeyPrefix HotkeyPrefix { get { HotkeyPrefix hotkeyPrefix; Debug.Assert(nativeFormat != IntPtr.Zero, "NativeFormat is null!"); int status = SafeNativeMethods.Gdip.GdipGetStringFormatHotkeyPrefix(new HandleRef(this, nativeFormat), out hotkeyPrefix); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return hotkeyPrefix; } set { //valid values are 0x0 to 0x2 if (!ClientUtils.IsEnumValid(value, (int)value, (int)HotkeyPrefix.None, (int)HotkeyPrefix.Hide)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(HotkeyPrefix)); } Debug.Assert(nativeFormat != IntPtr.Zero, "NativeFormat is null!"); int status = SafeNativeMethods.Gdip.GdipSetStringFormatHotkeyPrefix(new HandleRef(this, nativeFormat), value); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } } ////// Gets or sets the ///for this . /// /// /// Sets tab stops for this public void SetTabStops(float firstTabOffset, float[] tabStops) { if (firstTabOffset < 0) throw new ArgumentException(SR.GetString(SR.InvalidArgument, "firstTabOffset", firstTabOffset)); int status = SafeNativeMethods.Gdip.GdipSetStringFormatTabStops(new HandleRef(this, nativeFormat), firstTabOffset, tabStops.Length, tabStops); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///. /// /// /// Gets the tab stops for this public float [] GetTabStops(out float firstTabOffset) { int count = 0; int status = SafeNativeMethods.Gdip.GdipGetStringFormatTabStopCount(new HandleRef(this, nativeFormat), out count); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); float[] tabStops = new float[count]; status = SafeNativeMethods.Gdip.GdipGetStringFormatTabStops(new HandleRef(this, nativeFormat), count, out firstTabOffset, tabStops); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return tabStops; } // String trimming. How to handle more text than can be displayed // in the limits available. ///. /// /// /// Gets or sets the public StringTrimming Trimming { get { StringTrimming trimming; int status = SafeNativeMethods.Gdip.GdipGetStringFormatTrimming(new HandleRef(this, nativeFormat), out trimming); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return trimming; } set { //valid values are 0x0 to 0x5 if (!ClientUtils.IsEnumValid(value, (int)value, (int)StringTrimming.None, (int)StringTrimming.EllipsisPath)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(StringTrimming)); } int status = SafeNativeMethods.Gdip.GdipSetStringFormatTrimming(new HandleRef(this, nativeFormat), value); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } } ////// for this . /// /// /// Gets a generic default public static StringFormat GenericDefault { get { IntPtr format; int status = SafeNativeMethods.Gdip.GdipStringFormatGetGenericDefault(out format); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return new StringFormat(format); } } ///. /// Remarks from MSDN: A generic, default StringFormat object has the following characteristics: /// - No string format flags are set. /// - Character alignment and line alignment are set to StringAlignmentNear. /// - Language ID is set to neutral language, which means that the current language associated with the calling thread is used. /// - String digit substitution is set to StringDigitSubstituteUser. /// - Hot key prefix is set to HotkeyPrefixNone. /// - Number of tab stops is set to zero. /// - String trimming is set to StringTrimmingCharacter. /// /// /// Gets a generic typographic public static StringFormat GenericTypographic { get { IntPtr format; int status = SafeNativeMethods.Gdip.GdipStringFormatGetGenericTypographic(out format); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return new StringFormat(format); } } ///. /// Remarks from MSDN: A generic, typographic StringFormat object has the following characteristics: /// - String format flags StringFormatFlagsLineLimit, StringFormatFlagsNoClip, and StringFormatFlagsNoFitBlackBox are set. /// - Character alignment and line alignment are set to StringAlignmentNear. /// - Language ID is set to neutral language, which means that the current language associated with the calling thread is used. /// - String digit substitution is set to StringDigitSubstituteUser. /// - Hot key prefix is set to HotkeyPrefixNone. /// - Number of tab stops is set to zero. /// - String trimming is set to StringTrimmingNone. /// /// /// public void SetDigitSubstitution(int language, StringDigitSubstitute substitute) { int status = SafeNativeMethods.Gdip.GdipSetStringFormatDigitSubstitution(new HandleRef(this, nativeFormat), language, substitute); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// Gets the public StringDigitSubstitute DigitSubstitutionMethod { get { StringDigitSubstitute digitSubstitute; int lang = 0; int status = SafeNativeMethods.Gdip.GdipGetStringFormatDigitSubstitution(new HandleRef(this, nativeFormat), out lang, out digitSubstitute); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return digitSubstitute; } } ////// for this . /// /// /// Gets the language of public int DigitSubstitutionLanguage { get { StringDigitSubstitute digitSubstitute; int language = 0; int status = SafeNativeMethods.Gdip.GdipGetStringFormatDigitSubstitution(new HandleRef(this, nativeFormat), out language, out digitSubstitute); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return language; } } /** * Object cleanup */ ////// for this . /// /// /// Cleans up Windows resources for this /// ~StringFormat() { Dispose(false); } ///. /// /// /// Converts this public override string ToString() { return "[StringFormat, FormatFlags=" + FormatFlags.ToString() + "]"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //to /// a human-readable string. /// // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing { using System.Diagnostics; using System; using Microsoft.Win32; using System.Drawing; using System.ComponentModel; using System.Drawing.Text; using System.Drawing.Internal; using System.Runtime.InteropServices; using System.Globalization; ///[StructLayout(LayoutKind.Sequential)] public struct CharacterRange { private int first; private int length; /** * Create a new CharacterRange object */ /// /// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] public CharacterRange(int First, int Length) { this.first = First; this.length = Length; } ////// Initializes a new instance of the ///class /// with the specified coordinates. /// /// /// Gets the First character position of this public int First { get { return first; } set { first = value; } } ///. /// /// /// public int Length { get { return length; } set { length = value; } } public override bool Equals(object obj) { if (obj.GetType() != typeof(CharacterRange)) return false; CharacterRange cr = (CharacterRange)obj; return ((this.first == cr.First) && (this.length == cr.Length)); } ////// Gets the Length of this ///. /// public static bool operator ==(CharacterRange cr1, CharacterRange cr2) { return ((cr1.First == cr2.First) && (cr1.Length == cr2.Length)); } /// public static bool operator !=(CharacterRange cr1, CharacterRange cr2) { return !(cr1 == cr2); } /// public override int GetHashCode() { return this.first << 8 + this.length; } } /** * Represent a Stringformat object */ /// /// /// Encapsulates text layout information (such /// as alignment and linespacing), display manipulations (such as ellipsis insertion /// and national digit substitution) and OpenType features. /// public sealed class StringFormat : MarshalByRefObject, ICloneable, IDisposable { internal IntPtr nativeFormat; private StringFormat(IntPtr format) { nativeFormat = format; } ////// /// Initializes a new instance of the public StringFormat() : this(0, 0) { } ////// class. /// /// /// Initializes a new instance of the public StringFormat(StringFormatFlags options) : this(options, 0) { } ////// class with the specified . /// /// /// Initializes a new instance of the public StringFormat(StringFormatFlags options, int language) { int status = SafeNativeMethods.Gdip.GdipCreateStringFormat(options, language, out nativeFormat); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// class with the specified and language. /// /// /// public StringFormat(StringFormat format) { if (format == null) { throw new ArgumentNullException("format"); } int status = SafeNativeMethods.Gdip.GdipCloneStringFormat(new HandleRef(format, format.nativeFormat), out nativeFormat); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ////// Initializes a new instance of the ///class from the specified /// existing . /// /// /// Cleans up Windows resources for this /// public void Dispose() { Dispose(true); GC.SuppressFinalize(this); } ///. /// void Dispose(bool disposing) { if (nativeFormat != IntPtr.Zero) { try{ #if DEBUG int status = #endif SafeNativeMethods.Gdip.GdipDeleteStringFormat(new HandleRef(this, nativeFormat)); #if DEBUG Debug.Assert(status == SafeNativeMethods.Gdip.Ok, "GDI+ returned an error status: " + status.ToString(CultureInfo.InvariantCulture)); #endif } catch( Exception ex ){ if( ClientUtils.IsCriticalException( ex ) ) { throw; } Debug.Fail( "Exception thrown during Dispose: " + ex.ToString() ); } finally{ nativeFormat = IntPtr.Zero; } } } /// /// /// Creates an exact copy of this public object Clone() { IntPtr cloneFormat = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCloneStringFormat(new HandleRef(this, nativeFormat), out cloneFormat); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); StringFormat newCloneStringFormat = new StringFormat(cloneFormat); return newCloneStringFormat; } ///. /// /// /// Gets or sets a public StringFormatFlags FormatFlags { get { StringFormatFlags format; int status = SafeNativeMethods.Gdip.GdipGetStringFormatFlags(new HandleRef(this, nativeFormat), out format); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return format; } set { Debug.Assert(nativeFormat != IntPtr.Zero, "NativeFormat is null!"); int status = SafeNativeMethods.Gdip.GdipSetStringFormatFlags(new HandleRef(this, nativeFormat), value); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } } ///that contains formatting information. /// /// /// public void SetMeasurableCharacterRanges(CharacterRange[] ranges) { int status = SafeNativeMethods.Gdip.GdipSetStringFormatMeasurableCharacterRanges(new HandleRef(this, nativeFormat), ranges.Length, ranges); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } // For English, this is horizontal alignment ////// Sets the measure of characters to the specified /// range. /// ////// /// Specifies text alignment information. /// public StringAlignment Alignment { get { StringAlignment alignment = 0; Debug.Assert(nativeFormat != IntPtr.Zero, "NativeFormat is null!"); int status = SafeNativeMethods.Gdip.GdipGetStringFormatAlign(new HandleRef(this, nativeFormat), out alignment); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return alignment; } set { //valid values are 0x0 to 0x2 if (!ClientUtils.IsEnumValid(value, (int)value, (int)StringAlignment.Near, (int)StringAlignment.Far)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(StringAlignment)); } Debug.Assert(nativeFormat != IntPtr.Zero, "NativeFormat is null!"); int status = SafeNativeMethods.Gdip.GdipSetStringFormatAlign(new HandleRef(this, nativeFormat), value); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } } // For English, this is vertical alignment ////// /// Gets or sets the line alignment. /// public StringAlignment LineAlignment { get { StringAlignment alignment = 0; Debug.Assert(nativeFormat != IntPtr.Zero, "NativeFormat is null!"); int status = SafeNativeMethods.Gdip.GdipGetStringFormatLineAlign(new HandleRef(this, nativeFormat), out alignment); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return alignment; } set { if (value<0 || value>StringAlignment.Far) { throw new InvalidEnumArgumentException("value", (int)value, typeof(StringAlignment)); } Debug.Assert(nativeFormat != IntPtr.Zero, "NativeFormat is null!"); int status = SafeNativeMethods.Gdip.GdipSetStringFormatLineAlign(new HandleRef(this, nativeFormat), value); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } } ////// /// public HotkeyPrefix HotkeyPrefix { get { HotkeyPrefix hotkeyPrefix; Debug.Assert(nativeFormat != IntPtr.Zero, "NativeFormat is null!"); int status = SafeNativeMethods.Gdip.GdipGetStringFormatHotkeyPrefix(new HandleRef(this, nativeFormat), out hotkeyPrefix); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return hotkeyPrefix; } set { //valid values are 0x0 to 0x2 if (!ClientUtils.IsEnumValid(value, (int)value, (int)HotkeyPrefix.None, (int)HotkeyPrefix.Hide)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(HotkeyPrefix)); } Debug.Assert(nativeFormat != IntPtr.Zero, "NativeFormat is null!"); int status = SafeNativeMethods.Gdip.GdipSetStringFormatHotkeyPrefix(new HandleRef(this, nativeFormat), value); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } } ////// Gets or sets the ///for this . /// /// /// Sets tab stops for this public void SetTabStops(float firstTabOffset, float[] tabStops) { if (firstTabOffset < 0) throw new ArgumentException(SR.GetString(SR.InvalidArgument, "firstTabOffset", firstTabOffset)); int status = SafeNativeMethods.Gdip.GdipSetStringFormatTabStops(new HandleRef(this, nativeFormat), firstTabOffset, tabStops.Length, tabStops); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///. /// /// /// Gets the tab stops for this public float [] GetTabStops(out float firstTabOffset) { int count = 0; int status = SafeNativeMethods.Gdip.GdipGetStringFormatTabStopCount(new HandleRef(this, nativeFormat), out count); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); float[] tabStops = new float[count]; status = SafeNativeMethods.Gdip.GdipGetStringFormatTabStops(new HandleRef(this, nativeFormat), count, out firstTabOffset, tabStops); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return tabStops; } // String trimming. How to handle more text than can be displayed // in the limits available. ///. /// /// /// Gets or sets the public StringTrimming Trimming { get { StringTrimming trimming; int status = SafeNativeMethods.Gdip.GdipGetStringFormatTrimming(new HandleRef(this, nativeFormat), out trimming); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return trimming; } set { //valid values are 0x0 to 0x5 if (!ClientUtils.IsEnumValid(value, (int)value, (int)StringTrimming.None, (int)StringTrimming.EllipsisPath)) { throw new InvalidEnumArgumentException("value", (int)value, typeof(StringTrimming)); } int status = SafeNativeMethods.Gdip.GdipSetStringFormatTrimming(new HandleRef(this, nativeFormat), value); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } } ////// for this . /// /// /// Gets a generic default public static StringFormat GenericDefault { get { IntPtr format; int status = SafeNativeMethods.Gdip.GdipStringFormatGetGenericDefault(out format); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return new StringFormat(format); } } ///. /// Remarks from MSDN: A generic, default StringFormat object has the following characteristics: /// - No string format flags are set. /// - Character alignment and line alignment are set to StringAlignmentNear. /// - Language ID is set to neutral language, which means that the current language associated with the calling thread is used. /// - String digit substitution is set to StringDigitSubstituteUser. /// - Hot key prefix is set to HotkeyPrefixNone. /// - Number of tab stops is set to zero. /// - String trimming is set to StringTrimmingCharacter. /// /// /// Gets a generic typographic public static StringFormat GenericTypographic { get { IntPtr format; int status = SafeNativeMethods.Gdip.GdipStringFormatGetGenericTypographic(out format); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return new StringFormat(format); } } ///. /// Remarks from MSDN: A generic, typographic StringFormat object has the following characteristics: /// - String format flags StringFormatFlagsLineLimit, StringFormatFlagsNoClip, and StringFormatFlagsNoFitBlackBox are set. /// - Character alignment and line alignment are set to StringAlignmentNear. /// - Language ID is set to neutral language, which means that the current language associated with the calling thread is used. /// - String digit substitution is set to StringDigitSubstituteUser. /// - Hot key prefix is set to HotkeyPrefixNone. /// - Number of tab stops is set to zero. /// - String trimming is set to StringTrimmingNone. /// /// /// public void SetDigitSubstitution(int language, StringDigitSubstitute substitute) { int status = SafeNativeMethods.Gdip.GdipSetStringFormatDigitSubstitution(new HandleRef(this, nativeFormat), language, substitute); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); } ///[To be supplied.] ////// /// Gets the public StringDigitSubstitute DigitSubstitutionMethod { get { StringDigitSubstitute digitSubstitute; int lang = 0; int status = SafeNativeMethods.Gdip.GdipGetStringFormatDigitSubstitution(new HandleRef(this, nativeFormat), out lang, out digitSubstitute); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return digitSubstitute; } } ////// for this . /// /// /// Gets the language of public int DigitSubstitutionLanguage { get { StringDigitSubstitute digitSubstitute; int language = 0; int status = SafeNativeMethods.Gdip.GdipGetStringFormatDigitSubstitution(new HandleRef(this, nativeFormat), out language, out digitSubstitute); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return language; } } /** * Object cleanup */ ////// for this . /// /// /// Cleans up Windows resources for this /// ~StringFormat() { Dispose(false); } ///. /// /// /// Converts this public override string ToString() { return "[StringFormat, FormatFlags=" + FormatFlags.ToString() + "]"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.to /// a human-readable string. ///
Link Menu
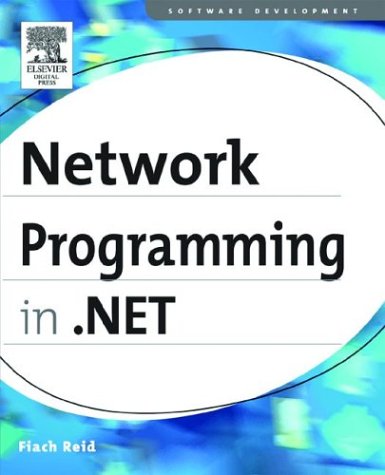
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DbConnectionPoolGroupProviderInfo.cs
- ZipIOEndOfCentralDirectoryBlock.cs
- ServiceCredentialsSecurityTokenManager.cs
- ProtocolViolationException.cs
- GenericEnumerator.cs
- StylusDownEventArgs.cs
- MailDefinition.cs
- PhonemeEventArgs.cs
- HwndProxyElementProvider.cs
- PointAnimationClockResource.cs
- SimpleRecyclingCache.cs
- EditorPartDesigner.cs
- PropertyStore.cs
- CompiledRegexRunner.cs
- AssociatedControlConverter.cs
- MailAddressCollection.cs
- WebPartZoneDesigner.cs
- baseaxisquery.cs
- SuppressMessageAttribute.cs
- UIElement3DAutomationPeer.cs
- DocumentPageHost.cs
- CompositeDesignerAccessibleObject.cs
- UnionCqlBlock.cs
- ColumnMapTranslator.cs
- Control.cs
- IgnoreSectionHandler.cs
- RadialGradientBrush.cs
- PrincipalPermission.cs
- HttpRequest.cs
- MissingMemberException.cs
- ApplicationInfo.cs
- SizeKeyFrameCollection.cs
- PathFigureCollectionValueSerializer.cs
- Vector3D.cs
- LineInfo.cs
- XPathNavigator.cs
- SynchronousReceiveBehavior.cs
- ContentPresenter.cs
- WebSysDescriptionAttribute.cs
- PathFigureCollection.cs
- ECDsaCng.cs
- UIHelper.cs
- XmlSerializerVersionAttribute.cs
- Effect.cs
- DrawItemEvent.cs
- SecUtil.cs
- RewritingValidator.cs
- DiagnosticTraceSource.cs
- StorageEntityTypeMapping.cs
- SemaphoreSlim.cs
- Label.cs
- FormViewUpdateEventArgs.cs
- RegisteredHiddenField.cs
- XmlHierarchicalDataSourceView.cs
- CompressionTransform.cs
- Rotation3DAnimation.cs
- ContainsRowNumberChecker.cs
- ClientTarget.cs
- BufferedGraphicsContext.cs
- cookieexception.cs
- PointCollection.cs
- FormClosedEvent.cs
- EntityDataSourceDesignerHelper.cs
- Constraint.cs
- ManagedIStream.cs
- KeyGesture.cs
- ObjectItemCollectionAssemblyCacheEntry.cs
- AutoResetEvent.cs
- ControlBuilder.cs
- MouseDevice.cs
- GroupPartitionExpr.cs
- SizeLimitedCache.cs
- Reference.cs
- EndpointIdentity.cs
- ScriptComponentDescriptor.cs
- HashSetEqualityComparer.cs
- WeakHashtable.cs
- InputReferenceExpression.cs
- ViewStateException.cs
- DataGridTablesFactory.cs
- EndpointDiscoveryBehavior.cs
- RemoteWebConfigurationHost.cs
- RedistVersionInfo.cs
- PageFunction.cs
- TransformedBitmap.cs
- CheckBoxList.cs
- SqlBinder.cs
- SoapMessage.cs
- InputLanguageProfileNotifySink.cs
- PersonalizationProviderHelper.cs
- Validator.cs
- TreePrinter.cs
- Classification.cs
- EdgeProfileValidation.cs
- GcSettings.cs
- iisPickupDirectory.cs
- HatchBrush.cs
- TableLayout.cs
- SuppressIldasmAttribute.cs
- SpoolingTask.cs