Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / RadialGradientBrush.cs / 1305600 / RadialGradientBrush.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: This file contains the implementation of RadialGradientBrush. // The RadialGradientBrush is a GradientBrush which defines its // Gradient as a radial interpolation within an Ellipse. // // History: // 05/08/2003 : [....] - Created it. // 09/21/2004 : timothyc - Added GradientStopCollection constructor. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PresentationCore; using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Reflection; using System.Runtime.InteropServices; using System.Windows; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows.Media { ////// RadialGradientBrush - This GradientBrush defines its Gradient as an interpolation /// within an Ellipse. /// public sealed partial class RadialGradientBrush : GradientBrush { #region Constructors ////// Default constructor for RadialGradientBrush. The resulting brush has no content. /// public RadialGradientBrush() : base() { } ////// RadialGradientBrush Constructor /// Constructs a RadialGradientBrush with two colors specified for GradientStops at /// offsets 0.0 and 1.0. /// /// The Color at offset 0.0. /// The Color at offset 1.0. public RadialGradientBrush(Color startColor, Color endColor) : base() { GradientStops.Add(new GradientStop(startColor, 0.0)); GradientStops.Add(new GradientStop(endColor, 1.0)); } ////// RadialGradientBrush Constructor /// Constructs a RadialGradientBrush with GradientStops set to the passed-in /// collection. /// /// GradientStopCollection to set on this brush. public RadialGradientBrush(GradientStopCollection gradientStopCollection) : base(gradientStopCollection) { } #endregion Constructors ////// Critical: This code acceses an unsafe code block /// TreatAsSafe: This does not expose any data uses all local variables. /// Sending instructions to the channel is considered a safe operation.Also /// all calls to copybytes have been verified so the operation is safe /// [SecurityCritical,SecurityTreatAsSafe] private void ManualUpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { Transform vTransform = Transform; Transform vRelativeTransform = RelativeTransform; GradientStopCollection vGradientStops = GradientStops; DUCE.ResourceHandle hTransform; if (vTransform == null || Object.ReferenceEquals(vTransform, Transform.Identity) ) { hTransform = DUCE.ResourceHandle.Null; } else { hTransform = ((DUCE.IResource)vTransform).GetHandle(channel); } DUCE.ResourceHandle hRelativeTransform; if (vRelativeTransform == null || Object.ReferenceEquals(vRelativeTransform, Transform.Identity) ) { hRelativeTransform = DUCE.ResourceHandle.Null; } else { hRelativeTransform = ((DUCE.IResource)vRelativeTransform).GetHandle(channel); } DUCE.ResourceHandle hOpacityAnimations = GetAnimationResourceHandle(OpacityProperty, channel); DUCE.ResourceHandle hCenterAnimations = GetAnimationResourceHandle(CenterProperty, channel); DUCE.ResourceHandle hRadiusXAnimations = GetAnimationResourceHandle(RadiusXProperty, channel); DUCE.ResourceHandle hRadiusYAnimations = GetAnimationResourceHandle(RadiusYProperty, channel); DUCE.ResourceHandle hGradientOriginAnimations = GetAnimationResourceHandle(GradientOriginProperty, channel); DUCE.MILCMD_RADIALGRADIENTBRUSH data; unsafe { data.Type = MILCMD.MilCmdRadialGradientBrush; data.Handle = _duceResource.GetHandle(channel); double tempOpacity = Opacity; DUCE.CopyBytes((byte*)&data.Opacity, (byte*)&tempOpacity, 8); data.hOpacityAnimations = hOpacityAnimations; data.hTransform = hTransform; data.hRelativeTransform = hRelativeTransform; data.ColorInterpolationMode = ColorInterpolationMode; data.MappingMode = MappingMode; data.SpreadMethod = SpreadMethod; Point tempCenter = Center; DUCE.CopyBytes((byte*)&data.Center, (byte*)&tempCenter, 16); data.hCenterAnimations = hCenterAnimations; double tempRadiusX = RadiusX; DUCE.CopyBytes((byte*)&data.RadiusX, (byte*)&tempRadiusX, 8); data.hRadiusXAnimations = hRadiusXAnimations; double tempRadiusY = RadiusY; DUCE.CopyBytes((byte*)&data.RadiusY, (byte*)&tempRadiusY, 8); data.hRadiusYAnimations = hRadiusYAnimations; Point tempGradientOrigin = GradientOrigin; DUCE.CopyBytes((byte*)&data.GradientOrigin, (byte*)&tempGradientOrigin, 16); data.hGradientOriginAnimations = hGradientOriginAnimations; // NTRAID#Longhorn-1011154-2004/8/12-asecchia GradientStopCollection: Need to enforce upper-limit of gradient stop capacity int count = (vGradientStops == null) ? 0 : vGradientStops.Count; data.GradientStopsSize = (UInt32)(sizeof(DUCE.MIL_GRADIENTSTOP)*count); channel.BeginCommand( (byte*)&data, sizeof(DUCE.MILCMD_RADIALGRADIENTBRUSH), sizeof(DUCE.MIL_GRADIENTSTOP)*count ); for (int i=0; i/// RadialGradientBrush - This GradientBrush defines its Gradient as an interpolation /// within an Ellipse. /// public sealed partial class RadialGradientBrush : GradientBrush { #region Constructors /// /// Default constructor for RadialGradientBrush. The resulting brush has no content. /// public RadialGradientBrush() : base() { } ////// RadialGradientBrush Constructor /// Constructs a RadialGradientBrush with two colors specified for GradientStops at /// offsets 0.0 and 1.0. /// /// The Color at offset 0.0. /// The Color at offset 1.0. public RadialGradientBrush(Color startColor, Color endColor) : base() { GradientStops.Add(new GradientStop(startColor, 0.0)); GradientStops.Add(new GradientStop(endColor, 1.0)); } ////// RadialGradientBrush Constructor /// Constructs a RadialGradientBrush with GradientStops set to the passed-in /// collection. /// /// GradientStopCollection to set on this brush. public RadialGradientBrush(GradientStopCollection gradientStopCollection) : base(gradientStopCollection) { } #endregion Constructors ////// Critical: This code acceses an unsafe code block /// TreatAsSafe: This does not expose any data uses all local variables. /// Sending instructions to the channel is considered a safe operation.Also /// all calls to copybytes have been verified so the operation is safe /// [SecurityCritical,SecurityTreatAsSafe] private void ManualUpdateResource(DUCE.Channel channel, bool skipOnChannelCheck) { // If we're told we can skip the channel check, then we must be on channel Debug.Assert(!skipOnChannelCheck || _duceResource.IsOnChannel(channel)); if (skipOnChannelCheck || _duceResource.IsOnChannel(channel)) { Transform vTransform = Transform; Transform vRelativeTransform = RelativeTransform; GradientStopCollection vGradientStops = GradientStops; DUCE.ResourceHandle hTransform; if (vTransform == null || Object.ReferenceEquals(vTransform, Transform.Identity) ) { hTransform = DUCE.ResourceHandle.Null; } else { hTransform = ((DUCE.IResource)vTransform).GetHandle(channel); } DUCE.ResourceHandle hRelativeTransform; if (vRelativeTransform == null || Object.ReferenceEquals(vRelativeTransform, Transform.Identity) ) { hRelativeTransform = DUCE.ResourceHandle.Null; } else { hRelativeTransform = ((DUCE.IResource)vRelativeTransform).GetHandle(channel); } DUCE.ResourceHandle hOpacityAnimations = GetAnimationResourceHandle(OpacityProperty, channel); DUCE.ResourceHandle hCenterAnimations = GetAnimationResourceHandle(CenterProperty, channel); DUCE.ResourceHandle hRadiusXAnimations = GetAnimationResourceHandle(RadiusXProperty, channel); DUCE.ResourceHandle hRadiusYAnimations = GetAnimationResourceHandle(RadiusYProperty, channel); DUCE.ResourceHandle hGradientOriginAnimations = GetAnimationResourceHandle(GradientOriginProperty, channel); DUCE.MILCMD_RADIALGRADIENTBRUSH data; unsafe { data.Type = MILCMD.MilCmdRadialGradientBrush; data.Handle = _duceResource.GetHandle(channel); double tempOpacity = Opacity; DUCE.CopyBytes((byte*)&data.Opacity, (byte*)&tempOpacity, 8); data.hOpacityAnimations = hOpacityAnimations; data.hTransform = hTransform; data.hRelativeTransform = hRelativeTransform; data.ColorInterpolationMode = ColorInterpolationMode; data.MappingMode = MappingMode; data.SpreadMethod = SpreadMethod; Point tempCenter = Center; DUCE.CopyBytes((byte*)&data.Center, (byte*)&tempCenter, 16); data.hCenterAnimations = hCenterAnimations; double tempRadiusX = RadiusX; DUCE.CopyBytes((byte*)&data.RadiusX, (byte*)&tempRadiusX, 8); data.hRadiusXAnimations = hRadiusXAnimations; double tempRadiusY = RadiusY; DUCE.CopyBytes((byte*)&data.RadiusY, (byte*)&tempRadiusY, 8); data.hRadiusYAnimations = hRadiusYAnimations; Point tempGradientOrigin = GradientOrigin; DUCE.CopyBytes((byte*)&data.GradientOrigin, (byte*)&tempGradientOrigin, 16); data.hGradientOriginAnimations = hGradientOriginAnimations; // NTRAID#Longhorn-1011154-2004/8/12-asecchia GradientStopCollection: Need to enforce upper-limit of gradient stop capacity int count = (vGradientStops == null) ? 0 : vGradientStops.Count; data.GradientStopsSize = (UInt32)(sizeof(DUCE.MIL_GRADIENTSTOP)*count); channel.BeginCommand( (byte*)&data, sizeof(DUCE.MILCMD_RADIALGRADIENTBRUSH), sizeof(DUCE.MIL_GRADIENTSTOP)*count ); for (int i=0; i
Link Menu
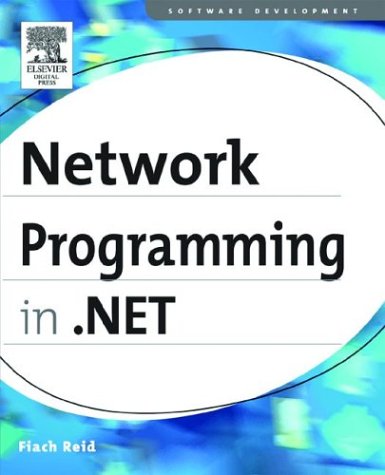
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextBoxLine.cs
- GridViewHeaderRowPresenter.cs
- PersonalizationStateQuery.cs
- SQLStringStorage.cs
- HttpInputStream.cs
- PropertyPath.cs
- TextLineBreak.cs
- PickBranch.cs
- RenderContext.cs
- HostedTcpTransportManager.cs
- SystemResourceKey.cs
- StructuredTypeEmitter.cs
- HyperLinkStyle.cs
- MembershipUser.cs
- Attachment.cs
- EmptyEnumerator.cs
- HtmlTitle.cs
- hwndwrapper.cs
- XmlAtomicValue.cs
- Ppl.cs
- SubMenuStyle.cs
- TagNameToTypeMapper.cs
- SafeViewOfFileHandle.cs
- OleDbParameterCollection.cs
- WebServiceReceiveDesigner.cs
- BinaryObjectInfo.cs
- safex509handles.cs
- ListParaClient.cs
- entitydatasourceentitysetnameconverter.cs
- GrammarBuilder.cs
- SizeLimitedCache.cs
- StringDictionaryWithComparer.cs
- RelatedImageListAttribute.cs
- AuditLogLocation.cs
- NavigatorOutput.cs
- sortedlist.cs
- wgx_exports.cs
- FieldMetadata.cs
- NavigationHelper.cs
- RecognizeCompletedEventArgs.cs
- DefinitionUpdate.cs
- HTMLTextWriter.cs
- ToolBarButtonClickEvent.cs
- CLRBindingWorker.cs
- EventHandlersDesigner.cs
- XamlReaderHelper.cs
- DragDropHelper.cs
- ECDsa.cs
- ConfigurationSchemaErrors.cs
- PackagePartCollection.cs
- _TLSstream.cs
- StandardBindingElement.cs
- RectangleConverter.cs
- CorrelationManager.cs
- TextEditorCopyPaste.cs
- BuildProviderCollection.cs
- TextUtf8RawTextWriter.cs
- TranslateTransform.cs
- KeyTimeConverter.cs
- Substitution.cs
- EntityRecordInfo.cs
- CompareValidator.cs
- NativeMethods.cs
- DesignerPerfEventProvider.cs
- DataReceivedEventArgs.cs
- XmlRawWriterWrapper.cs
- MatrixTransform3D.cs
- VariableQuery.cs
- LocatorPart.cs
- Point.cs
- InstanceNameConverter.cs
- ChannelTokenTypeConverter.cs
- FilteredDataSetHelper.cs
- EqualityComparer.cs
- OdbcStatementHandle.cs
- Triangle.cs
- ToolStripDropDownMenu.cs
- FieldTemplateUserControl.cs
- X509CertificateStore.cs
- DescendentsWalkerBase.cs
- BitmapFrameDecode.cs
- DataGridRelationshipRow.cs
- _NativeSSPI.cs
- SignatureDescription.cs
- ModelUtilities.cs
- PathFigure.cs
- DataGridTablesFactory.cs
- Config.cs
- AdRotator.cs
- DbParameterCollection.cs
- WebPageTraceListener.cs
- Win32SafeHandles.cs
- MappingItemCollection.cs
- SharedConnectionListener.cs
- DataGridViewRowHeightInfoPushedEventArgs.cs
- FaultContext.cs
- DBSqlParserTableCollection.cs
- ConstantProjectedSlot.cs
- controlskin.cs
- XmlEntity.cs