Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Configuration / System / Configuration / ConfigurationSchemaErrors.cs / 1 / ConfigurationSchemaErrors.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Text; internal class ConfigurationSchemaErrors { // Errors with ExceptionAction.Local are logged to this list. // This list is reset when processing of a section is complete. // Errors on this list may be added to the _errorsAll list // when RetrieveAndResetLocalErrors is called. private List_errorsLocal; // Errors with ExceptionAction.Global are logged to this list. private List _errorsGlobal; // All errors related to a config file are logged to this list. // This includes all global errors, all non-specific errors, // and local errors for input that applies to this config file. private List _errorsAll; internal ConfigurationSchemaErrors() {} internal bool HasLocalErrors { get { return ErrorsHelper.GetHasErrors(_errorsLocal); } } internal bool HasGlobalErrors { get { return ErrorsHelper.GetHasErrors(_errorsGlobal); } } private bool HasAllErrors { get { return ErrorsHelper.GetHasErrors(_errorsAll); } } internal int GlobalErrorCount { get { return ErrorsHelper.GetErrorCount(_errorsGlobal); } } // // Add a configuration Error. // internal void AddError(ConfigurationException ce, ExceptionAction action) { switch (action) { case ExceptionAction.Global: ErrorsHelper.AddError(ref _errorsAll, ce); ErrorsHelper.AddError(ref _errorsGlobal, ce); break; case ExceptionAction.NonSpecific: ErrorsHelper.AddError(ref _errorsAll, ce); break; case ExceptionAction.Local: ErrorsHelper.AddError(ref _errorsLocal, ce); break; } } internal void SetSingleGlobalError(ConfigurationException ce) { _errorsAll = null; _errorsLocal = null; _errorsGlobal = null; AddError(ce, ExceptionAction.Global); } internal bool HasErrors(bool ignoreLocal) { if (ignoreLocal) { return HasGlobalErrors; } else { return HasAllErrors; } } // ThrowIfErrors // // Throw if Errors were detected and remembered. // // Parameters: // IgnoreLocal - Should we be using the local errors also to // detemine if we should throw? // // Note: We will always return all the errors, no matter what // IgnoreLocal is. // internal void ThrowIfErrors(bool ignoreLocal) { if (HasErrors(ignoreLocal)) { if (HasGlobalErrors) { // Throw just the global errors, as they invalidate // all other config file parsing. throw new ConfigurationErrorsException(_errorsGlobal); } else { // Throw all errors no matter what throw new ConfigurationErrorsException(_errorsAll); } } } // RetrieveAndResetLocalErrors // // Retrieve the Local Errors, and Reset them to none. // internal List RetrieveAndResetLocalErrors(bool keepLocalErrors) { List list = _errorsLocal; _errorsLocal = null; if (keepLocalErrors) { ErrorsHelper.AddErrors(ref _errorsAll, list); } return list; } // // Add errors that have been saved for a specific section. // internal void AddSavedLocalErrors(ICollection coll) { ErrorsHelper.AddErrors(ref _errorsAll, coll); } // ResetLocalErrors // // Remove all the Local Errors, so we can start from scratch // internal void ResetLocalErrors() { RetrieveAndResetLocalErrors(false); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Text; internal class ConfigurationSchemaErrors { // Errors with ExceptionAction.Local are logged to this list. // This list is reset when processing of a section is complete. // Errors on this list may be added to the _errorsAll list // when RetrieveAndResetLocalErrors is called. private List_errorsLocal; // Errors with ExceptionAction.Global are logged to this list. private List _errorsGlobal; // All errors related to a config file are logged to this list. // This includes all global errors, all non-specific errors, // and local errors for input that applies to this config file. private List _errorsAll; internal ConfigurationSchemaErrors() {} internal bool HasLocalErrors { get { return ErrorsHelper.GetHasErrors(_errorsLocal); } } internal bool HasGlobalErrors { get { return ErrorsHelper.GetHasErrors(_errorsGlobal); } } private bool HasAllErrors { get { return ErrorsHelper.GetHasErrors(_errorsAll); } } internal int GlobalErrorCount { get { return ErrorsHelper.GetErrorCount(_errorsGlobal); } } // // Add a configuration Error. // internal void AddError(ConfigurationException ce, ExceptionAction action) { switch (action) { case ExceptionAction.Global: ErrorsHelper.AddError(ref _errorsAll, ce); ErrorsHelper.AddError(ref _errorsGlobal, ce); break; case ExceptionAction.NonSpecific: ErrorsHelper.AddError(ref _errorsAll, ce); break; case ExceptionAction.Local: ErrorsHelper.AddError(ref _errorsLocal, ce); break; } } internal void SetSingleGlobalError(ConfigurationException ce) { _errorsAll = null; _errorsLocal = null; _errorsGlobal = null; AddError(ce, ExceptionAction.Global); } internal bool HasErrors(bool ignoreLocal) { if (ignoreLocal) { return HasGlobalErrors; } else { return HasAllErrors; } } // ThrowIfErrors // // Throw if Errors were detected and remembered. // // Parameters: // IgnoreLocal - Should we be using the local errors also to // detemine if we should throw? // // Note: We will always return all the errors, no matter what // IgnoreLocal is. // internal void ThrowIfErrors(bool ignoreLocal) { if (HasErrors(ignoreLocal)) { if (HasGlobalErrors) { // Throw just the global errors, as they invalidate // all other config file parsing. throw new ConfigurationErrorsException(_errorsGlobal); } else { // Throw all errors no matter what throw new ConfigurationErrorsException(_errorsAll); } } } // RetrieveAndResetLocalErrors // // Retrieve the Local Errors, and Reset them to none. // internal List RetrieveAndResetLocalErrors(bool keepLocalErrors) { List list = _errorsLocal; _errorsLocal = null; if (keepLocalErrors) { ErrorsHelper.AddErrors(ref _errorsAll, list); } return list; } // // Add errors that have been saved for a specific section. // internal void AddSavedLocalErrors(ICollection coll) { ErrorsHelper.AddErrors(ref _errorsAll, coll); } // ResetLocalErrors // // Remove all the Local Errors, so we can start from scratch // internal void ResetLocalErrors() { RetrieveAndResetLocalErrors(false); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
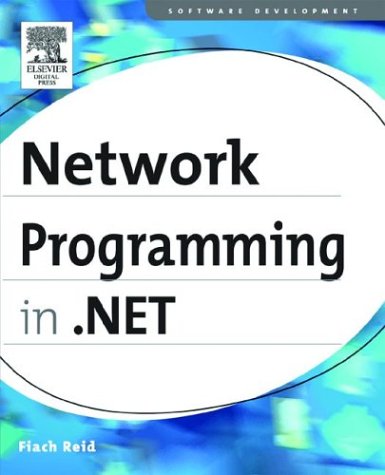
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FormCollection.cs
- DbConnectionOptions.cs
- XmlCountingReader.cs
- QilGeneratorEnv.cs
- QueryCacheManager.cs
- ImageIndexConverter.cs
- DesignerTransactionCloseEvent.cs
- CompletedAsyncResult.cs
- FusionWrap.cs
- FixedStringLookup.cs
- SourceElementsCollection.cs
- TreeBuilder.cs
- InlineUIContainer.cs
- View.cs
- ShaderRenderModeValidation.cs
- TypeReference.cs
- Parser.cs
- CapabilitiesState.cs
- EncodingInfo.cs
- WorkingDirectoryEditor.cs
- SerializationAttributes.cs
- SHA384Cng.cs
- ThreadSafeList.cs
- CalendarDateRange.cs
- HttpCookieCollection.cs
- SchemaNames.cs
- CacheAxisQuery.cs
- ellipse.cs
- XmlReturnReader.cs
- CodeCastExpression.cs
- DependencyStoreSurrogate.cs
- HScrollBar.cs
- UnsafeCollabNativeMethods.cs
- NodeFunctions.cs
- IteratorAsyncResult.cs
- DbProviderManifest.cs
- XmlTextEncoder.cs
- ObjectConverter.cs
- AppSettingsExpressionBuilder.cs
- WebControlsSection.cs
- BindStream.cs
- OuterGlowBitmapEffect.cs
- AttributeTableBuilder.cs
- ListViewDesigner.cs
- LinkUtilities.cs
- DispatcherObject.cs
- Query.cs
- MultiBinding.cs
- DbConnectionPoolIdentity.cs
- DetailsViewInsertedEventArgs.cs
- XmlQueryTypeFactory.cs
- InputLanguageManager.cs
- BasicCellRelation.cs
- querybuilder.cs
- GlyphManager.cs
- _StreamFramer.cs
- SqlUtil.cs
- EncodingNLS.cs
- WindowsToolbarAsMenu.cs
- DBDataPermissionAttribute.cs
- _ServiceNameStore.cs
- RenderData.cs
- DataServiceStreamResponse.cs
- AuthorizationSection.cs
- ManipulationLogic.cs
- EncryptedPackage.cs
- SessionSwitchEventArgs.cs
- SecurityContext.cs
- Color.cs
- KeyTimeConverter.cs
- PageCopyCount.cs
- Visual3D.cs
- ServerProtocol.cs
- MarkupExtensionReturnTypeAttribute.cs
- DocumentNUp.cs
- WebPartConnectionsEventArgs.cs
- PageHandlerFactory.cs
- Constraint.cs
- SHA1Managed.cs
- TabControlEvent.cs
- ColumnWidthChangedEvent.cs
- TextDpi.cs
- DataGridViewCellMouseEventArgs.cs
- DomainUpDown.cs
- TrackingQueryElement.cs
- DbProviderConfigurationHandler.cs
- DynamicResourceExtensionConverter.cs
- ParserStreamGeometryContext.cs
- AutomationIdentifier.cs
- Trigger.cs
- SyndicationCategory.cs
- MenuItem.cs
- WebPartRestoreVerb.cs
- GridViewColumnCollectionChangedEventArgs.cs
- DesignerGeometryHelper.cs
- XmlEnumAttribute.cs
- ListControlConvertEventArgs.cs
- Validator.cs
- PaperSize.cs
- CheckBoxField.cs