Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / DataServiceStreamResponse.cs / 1305376 / DataServiceStreamResponse.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// This class represents the response as a binary stream of data // and its metadata. // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System.Collections.Generic; using System.Diagnostics; using System.IO; #if !ASTORIA_LIGHT // Data.Services http stack using System.Net; #else using System.Data.Services.Http; #endif ////// Class which represents a stream response from the service. /// public sealed class DataServiceStreamResponse : IDisposable { ///The underlying web response private HttpWebResponse response; ///Lazy initialized cached response headers. private Dictionaryheaders; /// /// Constructor for the response. This method is internal since we don't want users to create instances /// of this class. /// /// The web response to wrap. internal DataServiceStreamResponse(HttpWebResponse response) { Debug.Assert(response != null, "Can't create a stream response object from a null response."); this.response = response; } ////// Returns the content type of the response stream (ex. image/png). /// If the Content-Type header was not present in the response this property /// will return null. /// public string ContentType { get { this.CheckDisposed(); return this.response.Headers[XmlConstants.HttpContentType]; } } ////// Returns the content disposition of the response stream. /// If the Content-Disposition header was not present in the response this property /// will return null. /// public string ContentDisposition { get { this.CheckDisposed(); return this.response.Headers[XmlConstants.HttpContentDisposition]; } } ////// Returns a dictionary containing all the response headers returned from the retrieve request /// to obtain the stream. /// public DictionaryHeaders { get { this.CheckDisposed(); if (this.headers == null) { this.headers = WebUtil.WrapResponseHeaders(this.response); } return this.headers; } } /// /// Returns the stream obtained from the data service. When reading from this stream /// the operations may throw if a network error occurs. This stream is read-only. /// /// Caller must call Dispose/Close on either the returned stream or on the response /// object itself. Otherwise the network connection will be left open and the caller /// might run out of available connections. /// public Stream Stream { get { this.CheckDisposed(); return this.response.GetResponseStream(); } } #region IDisposable Members ////// Disposes all resources held by this class. Namely the network stream. /// public void Dispose() { Util.Dispose(ref this.response); } #endregion ///Checks if the object has already been disposed. If so it throws the ObjectDisposedException. ///If the object has already been disposed. private void CheckDisposed() { if (this.response == null) { Error.ThrowObjectDisposed(this.GetType()); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// This class represents the response as a binary stream of data // and its metadata. // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System.Collections.Generic; using System.Diagnostics; using System.IO; #if !ASTORIA_LIGHT // Data.Services http stack using System.Net; #else using System.Data.Services.Http; #endif ////// Class which represents a stream response from the service. /// public sealed class DataServiceStreamResponse : IDisposable { ///The underlying web response private HttpWebResponse response; ///Lazy initialized cached response headers. private Dictionaryheaders; /// /// Constructor for the response. This method is internal since we don't want users to create instances /// of this class. /// /// The web response to wrap. internal DataServiceStreamResponse(HttpWebResponse response) { Debug.Assert(response != null, "Can't create a stream response object from a null response."); this.response = response; } ////// Returns the content type of the response stream (ex. image/png). /// If the Content-Type header was not present in the response this property /// will return null. /// public string ContentType { get { this.CheckDisposed(); return this.response.Headers[XmlConstants.HttpContentType]; } } ////// Returns the content disposition of the response stream. /// If the Content-Disposition header was not present in the response this property /// will return null. /// public string ContentDisposition { get { this.CheckDisposed(); return this.response.Headers[XmlConstants.HttpContentDisposition]; } } ////// Returns a dictionary containing all the response headers returned from the retrieve request /// to obtain the stream. /// public DictionaryHeaders { get { this.CheckDisposed(); if (this.headers == null) { this.headers = WebUtil.WrapResponseHeaders(this.response); } return this.headers; } } /// /// Returns the stream obtained from the data service. When reading from this stream /// the operations may throw if a network error occurs. This stream is read-only. /// /// Caller must call Dispose/Close on either the returned stream or on the response /// object itself. Otherwise the network connection will be left open and the caller /// might run out of available connections. /// public Stream Stream { get { this.CheckDisposed(); return this.response.GetResponseStream(); } } #region IDisposable Members ////// Disposes all resources held by this class. Namely the network stream. /// public void Dispose() { Util.Dispose(ref this.response); } #endregion ///Checks if the object has already been disposed. If so it throws the ObjectDisposedException. ///If the object has already been disposed. private void CheckDisposed() { if (this.response == null) { Error.ThrowObjectDisposed(this.GetType()); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
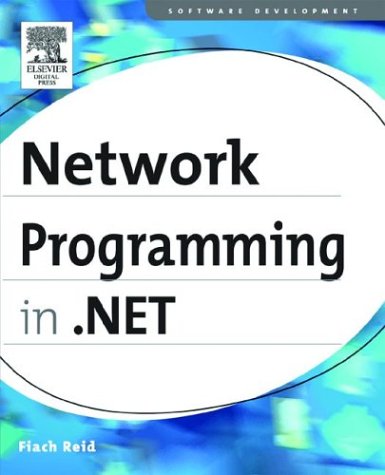
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewComboBoxCell.cs
- MdImport.cs
- PerformanceCounterCategory.cs
- _StreamFramer.cs
- ValidatingReaderNodeData.cs
- SHA256.cs
- ParenthesizePropertyNameAttribute.cs
- ConfigurationErrorsException.cs
- XPathNodePointer.cs
- WebPartsPersonalizationAuthorization.cs
- ConfigUtil.cs
- NativeMethods.cs
- SystemException.cs
- ValueConversionAttribute.cs
- ProcessHost.cs
- SubtreeProcessor.cs
- TableRow.cs
- XamlFigureLengthSerializer.cs
- ScrollEvent.cs
- IxmlLineInfo.cs
- ConnectivityStatus.cs
- WmlObjectListAdapter.cs
- SafeCertificateStore.cs
- MediaTimeline.cs
- MarginCollapsingState.cs
- ADMembershipProvider.cs
- EventEntry.cs
- BadImageFormatException.cs
- ListViewDeleteEventArgs.cs
- SeverityFilter.cs
- DataGridViewColumnDividerDoubleClickEventArgs.cs
- ConfigurationErrorsException.cs
- File.cs
- ExpressionPrefixAttribute.cs
- ListBindingConverter.cs
- XPathAxisIterator.cs
- ArgumentValidation.cs
- ProcessHostFactoryHelper.cs
- BaseValidator.cs
- ScrollableControl.cs
- ListView.cs
- EqualityComparer.cs
- ButtonRenderer.cs
- BuildProviderAppliesToAttribute.cs
- DataGridView.cs
- PropertyChange.cs
- ToolStripTextBox.cs
- FrameworkElementFactoryMarkupObject.cs
- PeerEndPoint.cs
- ConfigurationErrorsException.cs
- UICuesEvent.cs
- followingquery.cs
- keycontainerpermission.cs
- TextTreeText.cs
- PropertyValueUIItem.cs
- PassportIdentity.cs
- DnsCache.cs
- SQlBooleanStorage.cs
- SystemResources.cs
- InputProcessorProfilesLoader.cs
- LongValidatorAttribute.cs
- QilTypeChecker.cs
- ToolStripRendererSwitcher.cs
- WebEventCodes.cs
- XmlSerializerObjectSerializer.cs
- ContainerSelectorBehavior.cs
- DateTimeUtil.cs
- SqlFunctionAttribute.cs
- DesigntimeLicenseContext.cs
- ToolStripPanelRenderEventArgs.cs
- UnlockInstanceCommand.cs
- FolderBrowserDialog.cs
- LocalizableResourceBuilder.cs
- PipeStream.cs
- MarginsConverter.cs
- EventLog.cs
- UpdatePanel.cs
- StrongNameSignatureInformation.cs
- TransactionScope.cs
- QueryPageSettingsEventArgs.cs
- BinaryVersion.cs
- EncoderFallback.cs
- RelationshipManager.cs
- AstTree.cs
- CompositeDataBoundControl.cs
- GeneratedContractType.cs
- SchemaTableColumn.cs
- EntityCommandCompilationException.cs
- PolygonHotSpot.cs
- DashStyle.cs
- DataGridView.cs
- Stream.cs
- CodeTypeMember.cs
- TextRenderer.cs
- ResourcesGenerator.cs
- Cursor.cs
- DataTableClearEvent.cs
- EnumBuilder.cs
- DefaultParameterValueAttribute.cs
- ClientProxyGenerator.cs